Angular 6: Calling service observer.next from Http Interceptor causes infinite request loop
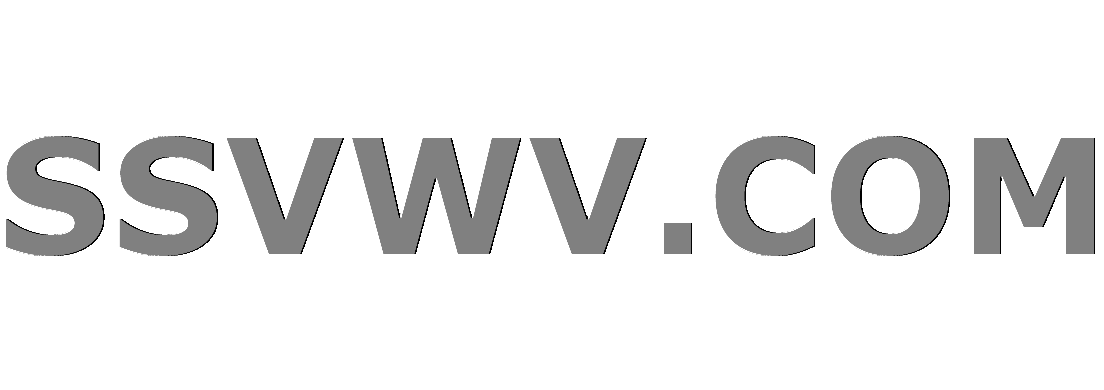
Multi tool use
I'm working on a website with authentication using JWT. I've created a HTTP interceptor class that adds the token to all requests headers and is used for catching 401 errors.
import {Injectable} from '@angular/core';
import {HttpEvent, HttpHandler, HttpInterceptor, HttpRequest} from '@angular/common/http';
import {Observable, of} from 'rxjs';
import {JwtService} from '../service/jwt.service';
import {catchError} from 'rxjs/operators';
import {AlertService} from '../../shared/service/alert.service';
import {Router} from '@angular/router';
import {AlertType} from '../../shared/model/alert.model';
@Injectable()
export class HttpTokenInterceptor implements HttpInterceptor {
constructor(private jwtService: JwtService, private alertService: AlertService, private router: Router) {
}
/**
* Intercept HTTP requests and return a cloned version with added headers
*
* @param req incoming request
* @param next observable next request
*/
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Add headers to all requests
const headersConfig = {
'Accept': 'application/json'
};
// Add token bearer to header when it's available
const token = this.jwtService.getToken();
if (token) {
headersConfig['Authorization'] = `Bearer ${token}`;
headersConfig['Content-Type'] = 'application/json';
}
const request = req.clone({setHeaders: headersConfig});
// Return adjusted http request with added headers
return next.handle(request).pipe(
catchError((error: any) => {
// Unauthorized response
if (error.status === 401) {
this.handleError();
return of(error);
}
throw error;
})
);
}
/**
* Handle http errors
*/
private handleError() {
// Destroy the token
this.jwtService.destroyToken();
// Redirect to login page
this.router.navigate(['/login']);
// This is causing infinite loops in HTTP requests
this.alertService.showAlert({
message: 'Your token is invalid, please login again.',
type: AlertType.Warning
});
}
}
The class uses my JwtToken class to remove the token from the localstorage and redirect the user to the login page using the Angular Router. The showAlert method from the alertService is causing the http request to be repeated infinitely.
I think it's being caused by the Observer implementation in the alert service. But I've tried so many different implementations that I have really no idea what is going wrong.
import {Injectable} from '@angular/core';
import {Alert} from '../model/alert.model';
import {Subject} from 'rxjs';
/**
* Alert Service: Used for showing alerts all over the website
* Callable from all components
*/
@Injectable()
export class AlertService {
public alertEvent: Subject<Alert>;
/**
* AlertService constructor
*/
constructor() {
this.alertEvent = new Subject<Alert>();
}
/**
* Emit event containing an Alert object
*
* @param alert
*/
public showAlert(alert: Alert) {
this.alertEvent.next(alert);
}
}
The alertService class is being used by an alert component that displays all the alert messages. This component is used in two main components: Dashboard & login.
import {Component} from '@angular/core';
import {AlertService} from '../../shared/service/alert.service';
import {Alert} from '../../shared/model/alert.model';
@Component({
selector: '*brand*-alerts',
templateUrl: './alerts.component.html',
})
export class AlertsComponent {
// Keep list in global component
public alerts: Array<Alert> = ;
constructor(private alertService: AlertService) {
// Hook to alertEvents and add to class list
alertService.alertEvent.asObservable().subscribe(alerts => {
// console.log(alerts);
this.alerts.push(alerts);
});
}
}
In the following image is the issue clearly visible:
video of loop
Kind regards.
Edit: solved
In the page that did a request there was a subscription initilialised on the alert service and that caused the http request to fire again. I simply have the alert component being the only subscriber to the alertService now and created a new service for the refresh. The answer from @incNick is indeed a correct implementation. Thanks!
angular jwt angular6 angular-httpclient angular-http-interceptors
add a comment |
I'm working on a website with authentication using JWT. I've created a HTTP interceptor class that adds the token to all requests headers and is used for catching 401 errors.
import {Injectable} from '@angular/core';
import {HttpEvent, HttpHandler, HttpInterceptor, HttpRequest} from '@angular/common/http';
import {Observable, of} from 'rxjs';
import {JwtService} from '../service/jwt.service';
import {catchError} from 'rxjs/operators';
import {AlertService} from '../../shared/service/alert.service';
import {Router} from '@angular/router';
import {AlertType} from '../../shared/model/alert.model';
@Injectable()
export class HttpTokenInterceptor implements HttpInterceptor {
constructor(private jwtService: JwtService, private alertService: AlertService, private router: Router) {
}
/**
* Intercept HTTP requests and return a cloned version with added headers
*
* @param req incoming request
* @param next observable next request
*/
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Add headers to all requests
const headersConfig = {
'Accept': 'application/json'
};
// Add token bearer to header when it's available
const token = this.jwtService.getToken();
if (token) {
headersConfig['Authorization'] = `Bearer ${token}`;
headersConfig['Content-Type'] = 'application/json';
}
const request = req.clone({setHeaders: headersConfig});
// Return adjusted http request with added headers
return next.handle(request).pipe(
catchError((error: any) => {
// Unauthorized response
if (error.status === 401) {
this.handleError();
return of(error);
}
throw error;
})
);
}
/**
* Handle http errors
*/
private handleError() {
// Destroy the token
this.jwtService.destroyToken();
// Redirect to login page
this.router.navigate(['/login']);
// This is causing infinite loops in HTTP requests
this.alertService.showAlert({
message: 'Your token is invalid, please login again.',
type: AlertType.Warning
});
}
}
The class uses my JwtToken class to remove the token from the localstorage and redirect the user to the login page using the Angular Router. The showAlert method from the alertService is causing the http request to be repeated infinitely.
I think it's being caused by the Observer implementation in the alert service. But I've tried so many different implementations that I have really no idea what is going wrong.
import {Injectable} from '@angular/core';
import {Alert} from '../model/alert.model';
import {Subject} from 'rxjs';
/**
* Alert Service: Used for showing alerts all over the website
* Callable from all components
*/
@Injectable()
export class AlertService {
public alertEvent: Subject<Alert>;
/**
* AlertService constructor
*/
constructor() {
this.alertEvent = new Subject<Alert>();
}
/**
* Emit event containing an Alert object
*
* @param alert
*/
public showAlert(alert: Alert) {
this.alertEvent.next(alert);
}
}
The alertService class is being used by an alert component that displays all the alert messages. This component is used in two main components: Dashboard & login.
import {Component} from '@angular/core';
import {AlertService} from '../../shared/service/alert.service';
import {Alert} from '../../shared/model/alert.model';
@Component({
selector: '*brand*-alerts',
templateUrl: './alerts.component.html',
})
export class AlertsComponent {
// Keep list in global component
public alerts: Array<Alert> = ;
constructor(private alertService: AlertService) {
// Hook to alertEvents and add to class list
alertService.alertEvent.asObservable().subscribe(alerts => {
// console.log(alerts);
this.alerts.push(alerts);
});
}
}
In the following image is the issue clearly visible:
video of loop
Kind regards.
Edit: solved
In the page that did a request there was a subscription initilialised on the alert service and that caused the http request to fire again. I simply have the alert component being the only subscriber to the alertService now and created a new service for the refresh. The answer from @incNick is indeed a correct implementation. Thanks!
angular jwt angular6 angular-httpclient angular-http-interceptors
add a comment |
I'm working on a website with authentication using JWT. I've created a HTTP interceptor class that adds the token to all requests headers and is used for catching 401 errors.
import {Injectable} from '@angular/core';
import {HttpEvent, HttpHandler, HttpInterceptor, HttpRequest} from '@angular/common/http';
import {Observable, of} from 'rxjs';
import {JwtService} from '../service/jwt.service';
import {catchError} from 'rxjs/operators';
import {AlertService} from '../../shared/service/alert.service';
import {Router} from '@angular/router';
import {AlertType} from '../../shared/model/alert.model';
@Injectable()
export class HttpTokenInterceptor implements HttpInterceptor {
constructor(private jwtService: JwtService, private alertService: AlertService, private router: Router) {
}
/**
* Intercept HTTP requests and return a cloned version with added headers
*
* @param req incoming request
* @param next observable next request
*/
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Add headers to all requests
const headersConfig = {
'Accept': 'application/json'
};
// Add token bearer to header when it's available
const token = this.jwtService.getToken();
if (token) {
headersConfig['Authorization'] = `Bearer ${token}`;
headersConfig['Content-Type'] = 'application/json';
}
const request = req.clone({setHeaders: headersConfig});
// Return adjusted http request with added headers
return next.handle(request).pipe(
catchError((error: any) => {
// Unauthorized response
if (error.status === 401) {
this.handleError();
return of(error);
}
throw error;
})
);
}
/**
* Handle http errors
*/
private handleError() {
// Destroy the token
this.jwtService.destroyToken();
// Redirect to login page
this.router.navigate(['/login']);
// This is causing infinite loops in HTTP requests
this.alertService.showAlert({
message: 'Your token is invalid, please login again.',
type: AlertType.Warning
});
}
}
The class uses my JwtToken class to remove the token from the localstorage and redirect the user to the login page using the Angular Router. The showAlert method from the alertService is causing the http request to be repeated infinitely.
I think it's being caused by the Observer implementation in the alert service. But I've tried so many different implementations that I have really no idea what is going wrong.
import {Injectable} from '@angular/core';
import {Alert} from '../model/alert.model';
import {Subject} from 'rxjs';
/**
* Alert Service: Used for showing alerts all over the website
* Callable from all components
*/
@Injectable()
export class AlertService {
public alertEvent: Subject<Alert>;
/**
* AlertService constructor
*/
constructor() {
this.alertEvent = new Subject<Alert>();
}
/**
* Emit event containing an Alert object
*
* @param alert
*/
public showAlert(alert: Alert) {
this.alertEvent.next(alert);
}
}
The alertService class is being used by an alert component that displays all the alert messages. This component is used in two main components: Dashboard & login.
import {Component} from '@angular/core';
import {AlertService} from '../../shared/service/alert.service';
import {Alert} from '../../shared/model/alert.model';
@Component({
selector: '*brand*-alerts',
templateUrl: './alerts.component.html',
})
export class AlertsComponent {
// Keep list in global component
public alerts: Array<Alert> = ;
constructor(private alertService: AlertService) {
// Hook to alertEvents and add to class list
alertService.alertEvent.asObservable().subscribe(alerts => {
// console.log(alerts);
this.alerts.push(alerts);
});
}
}
In the following image is the issue clearly visible:
video of loop
Kind regards.
Edit: solved
In the page that did a request there was a subscription initilialised on the alert service and that caused the http request to fire again. I simply have the alert component being the only subscriber to the alertService now and created a new service for the refresh. The answer from @incNick is indeed a correct implementation. Thanks!
angular jwt angular6 angular-httpclient angular-http-interceptors
I'm working on a website with authentication using JWT. I've created a HTTP interceptor class that adds the token to all requests headers and is used for catching 401 errors.
import {Injectable} from '@angular/core';
import {HttpEvent, HttpHandler, HttpInterceptor, HttpRequest} from '@angular/common/http';
import {Observable, of} from 'rxjs';
import {JwtService} from '../service/jwt.service';
import {catchError} from 'rxjs/operators';
import {AlertService} from '../../shared/service/alert.service';
import {Router} from '@angular/router';
import {AlertType} from '../../shared/model/alert.model';
@Injectable()
export class HttpTokenInterceptor implements HttpInterceptor {
constructor(private jwtService: JwtService, private alertService: AlertService, private router: Router) {
}
/**
* Intercept HTTP requests and return a cloned version with added headers
*
* @param req incoming request
* @param next observable next request
*/
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
// Add headers to all requests
const headersConfig = {
'Accept': 'application/json'
};
// Add token bearer to header when it's available
const token = this.jwtService.getToken();
if (token) {
headersConfig['Authorization'] = `Bearer ${token}`;
headersConfig['Content-Type'] = 'application/json';
}
const request = req.clone({setHeaders: headersConfig});
// Return adjusted http request with added headers
return next.handle(request).pipe(
catchError((error: any) => {
// Unauthorized response
if (error.status === 401) {
this.handleError();
return of(error);
}
throw error;
})
);
}
/**
* Handle http errors
*/
private handleError() {
// Destroy the token
this.jwtService.destroyToken();
// Redirect to login page
this.router.navigate(['/login']);
// This is causing infinite loops in HTTP requests
this.alertService.showAlert({
message: 'Your token is invalid, please login again.',
type: AlertType.Warning
});
}
}
The class uses my JwtToken class to remove the token from the localstorage and redirect the user to the login page using the Angular Router. The showAlert method from the alertService is causing the http request to be repeated infinitely.
I think it's being caused by the Observer implementation in the alert service. But I've tried so many different implementations that I have really no idea what is going wrong.
import {Injectable} from '@angular/core';
import {Alert} from '../model/alert.model';
import {Subject} from 'rxjs';
/**
* Alert Service: Used for showing alerts all over the website
* Callable from all components
*/
@Injectable()
export class AlertService {
public alertEvent: Subject<Alert>;
/**
* AlertService constructor
*/
constructor() {
this.alertEvent = new Subject<Alert>();
}
/**
* Emit event containing an Alert object
*
* @param alert
*/
public showAlert(alert: Alert) {
this.alertEvent.next(alert);
}
}
The alertService class is being used by an alert component that displays all the alert messages. This component is used in two main components: Dashboard & login.
import {Component} from '@angular/core';
import {AlertService} from '../../shared/service/alert.service';
import {Alert} from '../../shared/model/alert.model';
@Component({
selector: '*brand*-alerts',
templateUrl: './alerts.component.html',
})
export class AlertsComponent {
// Keep list in global component
public alerts: Array<Alert> = ;
constructor(private alertService: AlertService) {
// Hook to alertEvents and add to class list
alertService.alertEvent.asObservable().subscribe(alerts => {
// console.log(alerts);
this.alerts.push(alerts);
});
}
}
In the following image is the issue clearly visible:
video of loop
Kind regards.
Edit: solved
In the page that did a request there was a subscription initilialised on the alert service and that caused the http request to fire again. I simply have the alert component being the only subscriber to the alertService now and created a new service for the refresh. The answer from @incNick is indeed a correct implementation. Thanks!
angular jwt angular6 angular-httpclient angular-http-interceptors
angular jwt angular6 angular-httpclient angular-http-interceptors
edited Nov 23 '18 at 13:05
asked Nov 22 '18 at 23:09
Casper
153
153
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Sorry of I'm busy on my job, but may my source make helpful.
import { Observable, throwError } from 'rxjs';
import { tap, catchError } from 'rxjs/operators';
...
return httpHandler.handle(request).pipe(
tap((event: HttpEvent<any>) => {
if (event instanceof HttpResponse) {
//this.loadingService.endLoading();
}
},
(err: any) => {
//this.loadingService.endLoading();
}),
catchError((err: any) => {
if (err.status === 401) {
/*
this.modalController.create({
component: LoginComponent,
componentProps: {returnUrl: this.router.url},
showBackdrop: true
}).then(modal => modal.present());
*/
} else {
//this.messageService.showToast(`Some error happen, please try again. (Error-${err.status})`, 'error');
}
return throwError(err);
})
);
I'm return throwError(err) at end.
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
I've tracked it down to the service class. Whenever thethis.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.
– Casper
Nov 23 '18 at 11:25
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438936%2fangular-6-calling-service-observer-next-from-http-interceptor-causes-infinite-r%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sorry of I'm busy on my job, but may my source make helpful.
import { Observable, throwError } from 'rxjs';
import { tap, catchError } from 'rxjs/operators';
...
return httpHandler.handle(request).pipe(
tap((event: HttpEvent<any>) => {
if (event instanceof HttpResponse) {
//this.loadingService.endLoading();
}
},
(err: any) => {
//this.loadingService.endLoading();
}),
catchError((err: any) => {
if (err.status === 401) {
/*
this.modalController.create({
component: LoginComponent,
componentProps: {returnUrl: this.router.url},
showBackdrop: true
}).then(modal => modal.present());
*/
} else {
//this.messageService.showToast(`Some error happen, please try again. (Error-${err.status})`, 'error');
}
return throwError(err);
})
);
I'm return throwError(err) at end.
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
I've tracked it down to the service class. Whenever thethis.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.
– Casper
Nov 23 '18 at 11:25
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
|
show 2 more comments
Sorry of I'm busy on my job, but may my source make helpful.
import { Observable, throwError } from 'rxjs';
import { tap, catchError } from 'rxjs/operators';
...
return httpHandler.handle(request).pipe(
tap((event: HttpEvent<any>) => {
if (event instanceof HttpResponse) {
//this.loadingService.endLoading();
}
},
(err: any) => {
//this.loadingService.endLoading();
}),
catchError((err: any) => {
if (err.status === 401) {
/*
this.modalController.create({
component: LoginComponent,
componentProps: {returnUrl: this.router.url},
showBackdrop: true
}).then(modal => modal.present());
*/
} else {
//this.messageService.showToast(`Some error happen, please try again. (Error-${err.status})`, 'error');
}
return throwError(err);
})
);
I'm return throwError(err) at end.
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
I've tracked it down to the service class. Whenever thethis.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.
– Casper
Nov 23 '18 at 11:25
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
|
show 2 more comments
Sorry of I'm busy on my job, but may my source make helpful.
import { Observable, throwError } from 'rxjs';
import { tap, catchError } from 'rxjs/operators';
...
return httpHandler.handle(request).pipe(
tap((event: HttpEvent<any>) => {
if (event instanceof HttpResponse) {
//this.loadingService.endLoading();
}
},
(err: any) => {
//this.loadingService.endLoading();
}),
catchError((err: any) => {
if (err.status === 401) {
/*
this.modalController.create({
component: LoginComponent,
componentProps: {returnUrl: this.router.url},
showBackdrop: true
}).then(modal => modal.present());
*/
} else {
//this.messageService.showToast(`Some error happen, please try again. (Error-${err.status})`, 'error');
}
return throwError(err);
})
);
I'm return throwError(err) at end.
Sorry of I'm busy on my job, but may my source make helpful.
import { Observable, throwError } from 'rxjs';
import { tap, catchError } from 'rxjs/operators';
...
return httpHandler.handle(request).pipe(
tap((event: HttpEvent<any>) => {
if (event instanceof HttpResponse) {
//this.loadingService.endLoading();
}
},
(err: any) => {
//this.loadingService.endLoading();
}),
catchError((err: any) => {
if (err.status === 401) {
/*
this.modalController.create({
component: LoginComponent,
componentProps: {returnUrl: this.router.url},
showBackdrop: true
}).then(modal => modal.present());
*/
} else {
//this.messageService.showToast(`Some error happen, please try again. (Error-${err.status})`, 'error');
}
return throwError(err);
})
);
I'm return throwError(err) at end.
edited Nov 23 '18 at 12:09
answered Nov 22 '18 at 23:46


incNick
36715
36715
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
I've tracked it down to the service class. Whenever thethis.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.
– Casper
Nov 23 '18 at 11:25
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
|
show 2 more comments
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
I've tracked it down to the service class. Whenever thethis.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.
– Casper
Nov 23 '18 at 11:25
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
This does not seem to be the problem. I did delete the argument anyway since it wasn't used in the method itself. I've edited my OP
– Casper
Nov 23 '18 at 8:28
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Hope it is ok :(
– incNick
Nov 23 '18 at 10:14
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
Unfortunately this does not make a difference. When the request fails with a 401 the user is still routed to the login page. But adding an alert using the alertService still causes the request to retry and send another alert.
– Casper
Nov 23 '18 at 10:44
I've tracked it down to the service class. Whenever the
this.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.– Casper
Nov 23 '18 at 11:25
I've tracked it down to the service class. Whenever the
this.alertEvent.next(alert);
action is called the request gets called again, fails and sends an alert again.– Casper
Nov 23 '18 at 11:25
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
No worries, please only answer if you have the time for it. I'll look into it and come back to you!
– Casper
Nov 23 '18 at 12:55
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438936%2fangular-6-calling-service-observer-next-from-http-interceptor-causes-infinite-r%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
v,G,bdAo9,Fpw9CCinSdifNh8Rd1dtf7