OpenGL ES 2.0 - Blending issue with text (iOS)
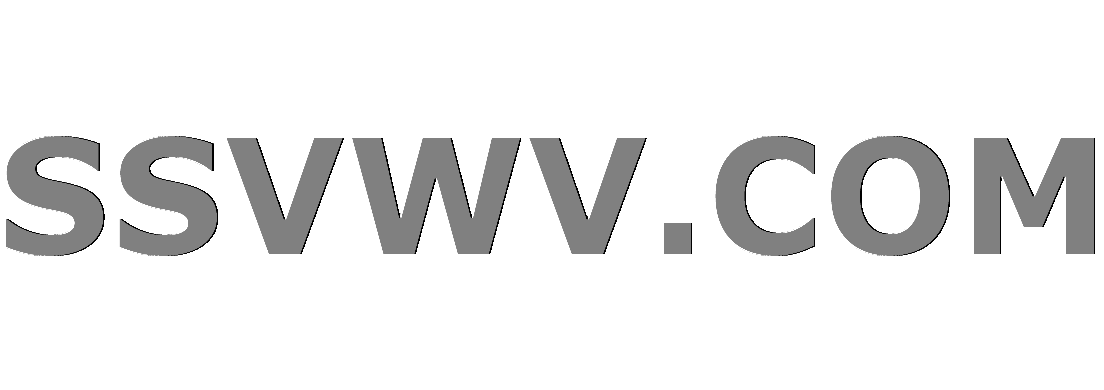
Multi tool use
up vote
0
down vote
favorite
I have grey shadows around texts when I blend them with OpenGL.
Currently, this is my blending function :
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
If I change it to :
glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA);
It works, and I obtain the same result with Gimp :
But, however with GL_ONE, the text can't be faded into the background anymore. It's like it's either transparency 0 or 1.
Also with GL_ONE, a crossfade between 2 pictures will somehow make the result image super bright :
Whereas with GL_SRC_ALPHA it looks normal :
So both solutions have pros and cons. I don't want the grey shadows, but I want to keep the crossfade effect.. Any suggestions would be very appreciated.
This is my fragment shader :
gl_FragColor = (v_Color * texture2D(u_Texture, v_TexCoordinate));
And here's how the textures (text and images) are loaded (premultiplied last):
+ (GLuint)getGLTextureFromCGIImage:(CGImageRef)cgiImage {
size_t width = CGImageGetWidth(cgiImage);
size_t height = CGImageGetHeight(cgiImage);
GLubyte *spriteData = (GLubyte *) calloc(width * height * 4, sizeof(GLubyte));
NSUInteger bytesPerPixel = 4;
NSUInteger bytesPerRow = bytesPerPixel * width;
NSUInteger bitsPerComponent = 8;
CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB();
CGContextRef spriteContext = CGBitmapContextCreate(spriteData,
width,
height,
bitsPerComponent,
bytesPerRow,
colorSpace,
kCGImageAlphaPremultipliedLast | kCGBitmapByteOrder32Big);
CGColorSpaceRelease(colorSpace);
CGContextDrawImage(spriteContext, CGRectMake(0, 0, width, height), cgiImage);
CGContextRelease(spriteContext);
return [GLMediaUtils getGLTextureFromPixelsInFormat:GL_RGBA
width:(int)width
height:(int)height
pixels:spriteData];
}
+ (GLuint)getGLTextureFromPixelsInFormat:(GLenum)format
width:(int)width
height:(int)height
pixels:(void *)pixels {
glActiveTexture(GL_TEXTURE0);
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
GLenum type = GL_UNSIGNED_BYTE;
if(format == GL_RGB) // RGB565
type = GL_UNSIGNED_SHORT_5_6_5;
glTexImage2D(GL_TEXTURE_2D, 0, format, width, height, 0, format, type, pixels);
free(pixels);
glFlush();
return texture;
}
ios opengl-es opengl-es-2.0 blending
add a comment |
up vote
0
down vote
favorite
I have grey shadows around texts when I blend them with OpenGL.
Currently, this is my blending function :
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
If I change it to :
glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA);
It works, and I obtain the same result with Gimp :
But, however with GL_ONE, the text can't be faded into the background anymore. It's like it's either transparency 0 or 1.
Also with GL_ONE, a crossfade between 2 pictures will somehow make the result image super bright :
Whereas with GL_SRC_ALPHA it looks normal :
So both solutions have pros and cons. I don't want the grey shadows, but I want to keep the crossfade effect.. Any suggestions would be very appreciated.
This is my fragment shader :
gl_FragColor = (v_Color * texture2D(u_Texture, v_TexCoordinate));
And here's how the textures (text and images) are loaded (premultiplied last):
+ (GLuint)getGLTextureFromCGIImage:(CGImageRef)cgiImage {
size_t width = CGImageGetWidth(cgiImage);
size_t height = CGImageGetHeight(cgiImage);
GLubyte *spriteData = (GLubyte *) calloc(width * height * 4, sizeof(GLubyte));
NSUInteger bytesPerPixel = 4;
NSUInteger bytesPerRow = bytesPerPixel * width;
NSUInteger bitsPerComponent = 8;
CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB();
CGContextRef spriteContext = CGBitmapContextCreate(spriteData,
width,
height,
bitsPerComponent,
bytesPerRow,
colorSpace,
kCGImageAlphaPremultipliedLast | kCGBitmapByteOrder32Big);
CGColorSpaceRelease(colorSpace);
CGContextDrawImage(spriteContext, CGRectMake(0, 0, width, height), cgiImage);
CGContextRelease(spriteContext);
return [GLMediaUtils getGLTextureFromPixelsInFormat:GL_RGBA
width:(int)width
height:(int)height
pixels:spriteData];
}
+ (GLuint)getGLTextureFromPixelsInFormat:(GLenum)format
width:(int)width
height:(int)height
pixels:(void *)pixels {
glActiveTexture(GL_TEXTURE0);
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
GLenum type = GL_UNSIGNED_BYTE;
if(format == GL_RGB) // RGB565
type = GL_UNSIGNED_SHORT_5_6_5;
glTexImage2D(GL_TEXTURE_2D, 0, format, width, height, 0, format, type, pixels);
free(pixels);
glFlush();
return texture;
}
ios opengl-es opengl-es-2.0 blending
1
what you're looking for is pre-multiplied alpha.
– derhass
Nov 21 at 18:39
@derhass Thanks but what do you mean ? The images are loaded with : "kCGImageAlphaPremultipliedLast"
– Xys
Nov 21 at 18:52
have you tried enabling multisampling when you are using GL_SRC_ALPHA?
– Paritosh Kulkarni
Nov 21 at 19:14
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have grey shadows around texts when I blend them with OpenGL.
Currently, this is my blending function :
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
If I change it to :
glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA);
It works, and I obtain the same result with Gimp :
But, however with GL_ONE, the text can't be faded into the background anymore. It's like it's either transparency 0 or 1.
Also with GL_ONE, a crossfade between 2 pictures will somehow make the result image super bright :
Whereas with GL_SRC_ALPHA it looks normal :
So both solutions have pros and cons. I don't want the grey shadows, but I want to keep the crossfade effect.. Any suggestions would be very appreciated.
This is my fragment shader :
gl_FragColor = (v_Color * texture2D(u_Texture, v_TexCoordinate));
And here's how the textures (text and images) are loaded (premultiplied last):
+ (GLuint)getGLTextureFromCGIImage:(CGImageRef)cgiImage {
size_t width = CGImageGetWidth(cgiImage);
size_t height = CGImageGetHeight(cgiImage);
GLubyte *spriteData = (GLubyte *) calloc(width * height * 4, sizeof(GLubyte));
NSUInteger bytesPerPixel = 4;
NSUInteger bytesPerRow = bytesPerPixel * width;
NSUInteger bitsPerComponent = 8;
CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB();
CGContextRef spriteContext = CGBitmapContextCreate(spriteData,
width,
height,
bitsPerComponent,
bytesPerRow,
colorSpace,
kCGImageAlphaPremultipliedLast | kCGBitmapByteOrder32Big);
CGColorSpaceRelease(colorSpace);
CGContextDrawImage(spriteContext, CGRectMake(0, 0, width, height), cgiImage);
CGContextRelease(spriteContext);
return [GLMediaUtils getGLTextureFromPixelsInFormat:GL_RGBA
width:(int)width
height:(int)height
pixels:spriteData];
}
+ (GLuint)getGLTextureFromPixelsInFormat:(GLenum)format
width:(int)width
height:(int)height
pixels:(void *)pixels {
glActiveTexture(GL_TEXTURE0);
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
GLenum type = GL_UNSIGNED_BYTE;
if(format == GL_RGB) // RGB565
type = GL_UNSIGNED_SHORT_5_6_5;
glTexImage2D(GL_TEXTURE_2D, 0, format, width, height, 0, format, type, pixels);
free(pixels);
glFlush();
return texture;
}
ios opengl-es opengl-es-2.0 blending
I have grey shadows around texts when I blend them with OpenGL.
Currently, this is my blending function :
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
If I change it to :
glBlendFunc(GL_ONE, GL_ONE_MINUS_SRC_ALPHA);
It works, and I obtain the same result with Gimp :
But, however with GL_ONE, the text can't be faded into the background anymore. It's like it's either transparency 0 or 1.
Also with GL_ONE, a crossfade between 2 pictures will somehow make the result image super bright :
Whereas with GL_SRC_ALPHA it looks normal :
So both solutions have pros and cons. I don't want the grey shadows, but I want to keep the crossfade effect.. Any suggestions would be very appreciated.
This is my fragment shader :
gl_FragColor = (v_Color * texture2D(u_Texture, v_TexCoordinate));
And here's how the textures (text and images) are loaded (premultiplied last):
+ (GLuint)getGLTextureFromCGIImage:(CGImageRef)cgiImage {
size_t width = CGImageGetWidth(cgiImage);
size_t height = CGImageGetHeight(cgiImage);
GLubyte *spriteData = (GLubyte *) calloc(width * height * 4, sizeof(GLubyte));
NSUInteger bytesPerPixel = 4;
NSUInteger bytesPerRow = bytesPerPixel * width;
NSUInteger bitsPerComponent = 8;
CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB();
CGContextRef spriteContext = CGBitmapContextCreate(spriteData,
width,
height,
bitsPerComponent,
bytesPerRow,
colorSpace,
kCGImageAlphaPremultipliedLast | kCGBitmapByteOrder32Big);
CGColorSpaceRelease(colorSpace);
CGContextDrawImage(spriteContext, CGRectMake(0, 0, width, height), cgiImage);
CGContextRelease(spriteContext);
return [GLMediaUtils getGLTextureFromPixelsInFormat:GL_RGBA
width:(int)width
height:(int)height
pixels:spriteData];
}
+ (GLuint)getGLTextureFromPixelsInFormat:(GLenum)format
width:(int)width
height:(int)height
pixels:(void *)pixels {
glActiveTexture(GL_TEXTURE0);
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
GLenum type = GL_UNSIGNED_BYTE;
if(format == GL_RGB) // RGB565
type = GL_UNSIGNED_SHORT_5_6_5;
glTexImage2D(GL_TEXTURE_2D, 0, format, width, height, 0, format, type, pixels);
free(pixels);
glFlush();
return texture;
}
ios opengl-es opengl-es-2.0 blending
ios opengl-es opengl-es-2.0 blending
edited Nov 21 at 19:47


genpfault
41.3k95197
41.3k95197
asked Nov 21 at 18:27
Xys
194116
194116
1
what you're looking for is pre-multiplied alpha.
– derhass
Nov 21 at 18:39
@derhass Thanks but what do you mean ? The images are loaded with : "kCGImageAlphaPremultipliedLast"
– Xys
Nov 21 at 18:52
have you tried enabling multisampling when you are using GL_SRC_ALPHA?
– Paritosh Kulkarni
Nov 21 at 19:14
add a comment |
1
what you're looking for is pre-multiplied alpha.
– derhass
Nov 21 at 18:39
@derhass Thanks but what do you mean ? The images are loaded with : "kCGImageAlphaPremultipliedLast"
– Xys
Nov 21 at 18:52
have you tried enabling multisampling when you are using GL_SRC_ALPHA?
– Paritosh Kulkarni
Nov 21 at 19:14
1
1
what you're looking for is pre-multiplied alpha.
– derhass
Nov 21 at 18:39
what you're looking for is pre-multiplied alpha.
– derhass
Nov 21 at 18:39
@derhass Thanks but what do you mean ? The images are loaded with : "kCGImageAlphaPremultipliedLast"
– Xys
Nov 21 at 18:52
@derhass Thanks but what do you mean ? The images are loaded with : "kCGImageAlphaPremultipliedLast"
– Xys
Nov 21 at 18:52
have you tried enabling multisampling when you are using GL_SRC_ALPHA?
– Paritosh Kulkarni
Nov 21 at 19:14
have you tried enabling multisampling when you are using GL_SRC_ALPHA?
– Paritosh Kulkarni
Nov 21 at 19:14
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You're almost there. You need to use pre-multiplied alpha if you want to avoid ghosting from the texture (because GL blending is post-multiplied alpha normally, which causes color bleed across the channels).
Normally GL_ONE
works because the correct alpha has been pre-baked into the texture RGB color channels prior to texture upload. If you start adding custom fading on top of that in the shader then you end up with a desync between the alpha you use for blending in the shader and the alpha that was used for pre-multiplication.
... so you need to add an adjustment to your fragment shader based on the new alpha value to get back to a stable premultiplication value. Something like:
vec4 texColor = texture2D(u_Texture, v_TexCoordinate);
// Scale the texture RGB by the vertex color
texColor.rgb *= v_color.rgb;
// Scale the texture RGBA by the vertex alpha to reinstate premultiplication
gl_FragColor = texColor * v_color.a;
The other approach is to store the font as only a luminance texture. You get grey shadows because the color channels are black outside of the font and texture filtering is mixing white and black. If you know the font color is white you don't need to store RGB
values in a texture at all ... (or if you are lazy just keep the existing texture and flood fill the entire texture RGB channels with white).
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You're almost there. You need to use pre-multiplied alpha if you want to avoid ghosting from the texture (because GL blending is post-multiplied alpha normally, which causes color bleed across the channels).
Normally GL_ONE
works because the correct alpha has been pre-baked into the texture RGB color channels prior to texture upload. If you start adding custom fading on top of that in the shader then you end up with a desync between the alpha you use for blending in the shader and the alpha that was used for pre-multiplication.
... so you need to add an adjustment to your fragment shader based on the new alpha value to get back to a stable premultiplication value. Something like:
vec4 texColor = texture2D(u_Texture, v_TexCoordinate);
// Scale the texture RGB by the vertex color
texColor.rgb *= v_color.rgb;
// Scale the texture RGBA by the vertex alpha to reinstate premultiplication
gl_FragColor = texColor * v_color.a;
The other approach is to store the font as only a luminance texture. You get grey shadows because the color channels are black outside of the font and texture filtering is mixing white and black. If you know the font color is white you don't need to store RGB
values in a texture at all ... (or if you are lazy just keep the existing texture and flood fill the entire texture RGB channels with white).
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
add a comment |
up vote
1
down vote
accepted
You're almost there. You need to use pre-multiplied alpha if you want to avoid ghosting from the texture (because GL blending is post-multiplied alpha normally, which causes color bleed across the channels).
Normally GL_ONE
works because the correct alpha has been pre-baked into the texture RGB color channels prior to texture upload. If you start adding custom fading on top of that in the shader then you end up with a desync between the alpha you use for blending in the shader and the alpha that was used for pre-multiplication.
... so you need to add an adjustment to your fragment shader based on the new alpha value to get back to a stable premultiplication value. Something like:
vec4 texColor = texture2D(u_Texture, v_TexCoordinate);
// Scale the texture RGB by the vertex color
texColor.rgb *= v_color.rgb;
// Scale the texture RGBA by the vertex alpha to reinstate premultiplication
gl_FragColor = texColor * v_color.a;
The other approach is to store the font as only a luminance texture. You get grey shadows because the color channels are black outside of the font and texture filtering is mixing white and black. If you know the font color is white you don't need to store RGB
values in a texture at all ... (or if you are lazy just keep the existing texture and flood fill the entire texture RGB channels with white).
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You're almost there. You need to use pre-multiplied alpha if you want to avoid ghosting from the texture (because GL blending is post-multiplied alpha normally, which causes color bleed across the channels).
Normally GL_ONE
works because the correct alpha has been pre-baked into the texture RGB color channels prior to texture upload. If you start adding custom fading on top of that in the shader then you end up with a desync between the alpha you use for blending in the shader and the alpha that was used for pre-multiplication.
... so you need to add an adjustment to your fragment shader based on the new alpha value to get back to a stable premultiplication value. Something like:
vec4 texColor = texture2D(u_Texture, v_TexCoordinate);
// Scale the texture RGB by the vertex color
texColor.rgb *= v_color.rgb;
// Scale the texture RGBA by the vertex alpha to reinstate premultiplication
gl_FragColor = texColor * v_color.a;
The other approach is to store the font as only a luminance texture. You get grey shadows because the color channels are black outside of the font and texture filtering is mixing white and black. If you know the font color is white you don't need to store RGB
values in a texture at all ... (or if you are lazy just keep the existing texture and flood fill the entire texture RGB channels with white).
You're almost there. You need to use pre-multiplied alpha if you want to avoid ghosting from the texture (because GL blending is post-multiplied alpha normally, which causes color bleed across the channels).
Normally GL_ONE
works because the correct alpha has been pre-baked into the texture RGB color channels prior to texture upload. If you start adding custom fading on top of that in the shader then you end up with a desync between the alpha you use for blending in the shader and the alpha that was used for pre-multiplication.
... so you need to add an adjustment to your fragment shader based on the new alpha value to get back to a stable premultiplication value. Something like:
vec4 texColor = texture2D(u_Texture, v_TexCoordinate);
// Scale the texture RGB by the vertex color
texColor.rgb *= v_color.rgb;
// Scale the texture RGBA by the vertex alpha to reinstate premultiplication
gl_FragColor = texColor * v_color.a;
The other approach is to store the font as only a luminance texture. You get grey shadows because the color channels are black outside of the font and texture filtering is mixing white and black. If you know the font color is white you don't need to store RGB
values in a texture at all ... (or if you are lazy just keep the existing texture and flood fill the entire texture RGB channels with white).
edited Nov 21 at 21:32
answered Nov 21 at 21:27
solidpixel
4,77611120
4,77611120
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
add a comment |
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
Thanks I think it solved everything !
– Xys
Nov 22 at 11:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418408%2fopengl-es-2-0-blending-issue-with-text-ios%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YAET iaab,Ttf,P7wN,TlZYsV8a fMxf 9,76O1cvx4,osROtde,d9U Cz6ecuuA9 v gPRF99nRX8xTWen8uw1pbQ mW,VjbTPhEYZ 7Vp
1
what you're looking for is pre-multiplied alpha.
– derhass
Nov 21 at 18:39
@derhass Thanks but what do you mean ? The images are loaded with : "kCGImageAlphaPremultipliedLast"
– Xys
Nov 21 at 18:52
have you tried enabling multisampling when you are using GL_SRC_ALPHA?
– Paritosh Kulkarni
Nov 21 at 19:14