Consider null and empty records as same in Collectors.groupingBy
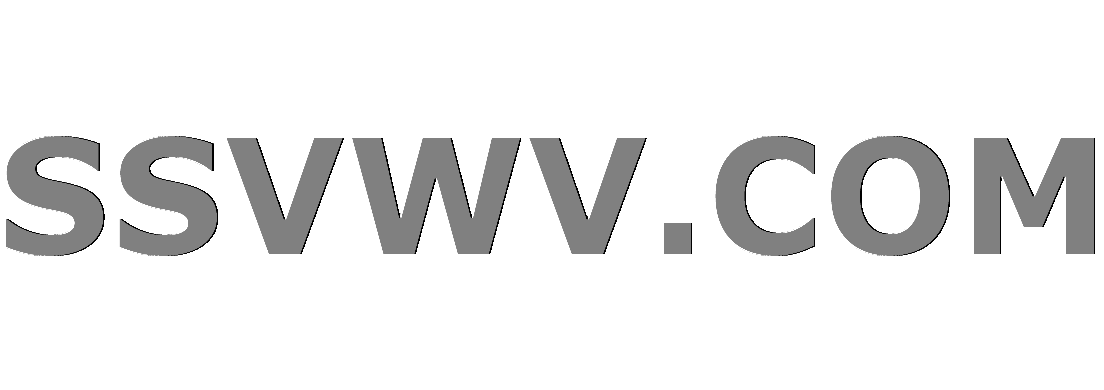
Multi tool use
I have a list of objects where a few records can have empty value property and a few can have null value property. Using Collectors.groupingBy I need both the records to be considered as same.
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
class Code {
private String type;
private String description;
public static void main(String args) {
List<Code> codeList = new ArrayList<>();
Code c = new Code();
c.setDescription("abc");
c.setType("");
codeList.add(c);
Code c1 = new Code();
c1.setDescription("abc");
c1.setType(null);
codeList.add(c1);
Map<String, List<Code>> codeMap = codeList.stream()
.collect(Collectors.groupingBy(code -> getGroupingKey(code)));
System.out.println(codeMap);
System.out.println(codeMap.size());
}
private static String getGroupingKey(Code code) {
return code.getDescription() +
"~" + code.getType();
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
The result of codeMap will have two records since it considers the empty string and the null value in the Type property as different. How can I achieve getting a single record here by considering both the null and empty records as same.
java collectors
add a comment |
I have a list of objects where a few records can have empty value property and a few can have null value property. Using Collectors.groupingBy I need both the records to be considered as same.
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
class Code {
private String type;
private String description;
public static void main(String args) {
List<Code> codeList = new ArrayList<>();
Code c = new Code();
c.setDescription("abc");
c.setType("");
codeList.add(c);
Code c1 = new Code();
c1.setDescription("abc");
c1.setType(null);
codeList.add(c1);
Map<String, List<Code>> codeMap = codeList.stream()
.collect(Collectors.groupingBy(code -> getGroupingKey(code)));
System.out.println(codeMap);
System.out.println(codeMap.size());
}
private static String getGroupingKey(Code code) {
return code.getDescription() +
"~" + code.getType();
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
The result of codeMap will have two records since it considers the empty string and the null value in the Type property as different. How can I achieve getting a single record here by considering both the null and empty records as same.
java collectors
add a comment |
I have a list of objects where a few records can have empty value property and a few can have null value property. Using Collectors.groupingBy I need both the records to be considered as same.
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
class Code {
private String type;
private String description;
public static void main(String args) {
List<Code> codeList = new ArrayList<>();
Code c = new Code();
c.setDescription("abc");
c.setType("");
codeList.add(c);
Code c1 = new Code();
c1.setDescription("abc");
c1.setType(null);
codeList.add(c1);
Map<String, List<Code>> codeMap = codeList.stream()
.collect(Collectors.groupingBy(code -> getGroupingKey(code)));
System.out.println(codeMap);
System.out.println(codeMap.size());
}
private static String getGroupingKey(Code code) {
return code.getDescription() +
"~" + code.getType();
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
The result of codeMap will have two records since it considers the empty string and the null value in the Type property as different. How can I achieve getting a single record here by considering both the null and empty records as same.
java collectors
I have a list of objects where a few records can have empty value property and a few can have null value property. Using Collectors.groupingBy I need both the records to be considered as same.
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
class Code {
private String type;
private String description;
public static void main(String args) {
List<Code> codeList = new ArrayList<>();
Code c = new Code();
c.setDescription("abc");
c.setType("");
codeList.add(c);
Code c1 = new Code();
c1.setDescription("abc");
c1.setType(null);
codeList.add(c1);
Map<String, List<Code>> codeMap = codeList.stream()
.collect(Collectors.groupingBy(code -> getGroupingKey(code)));
System.out.println(codeMap);
System.out.println(codeMap.size());
}
private static String getGroupingKey(Code code) {
return code.getDescription() +
"~" + code.getType();
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
The result of codeMap will have two records since it considers the empty string and the null value in the Type property as different. How can I achieve getting a single record here by considering both the null and empty records as same.
java collectors
java collectors
edited Nov 23 '18 at 3:12


Koray Tugay
8,68826111219
8,68826111219
asked Nov 23 '18 at 3:06
shreya
376
376
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can modify your getGroupingKey
method like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + (code.getType() == null ? "" : code.getType());
}
or like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + Optional.ofNullable(code.getType()).orElse("");
}
or you might as well modify your getType()
method directly as in:
public String getType() {
return type == null ? "" : type;
}
or:
public String getType() {
return Optional.ofNullable(type).orElse("");
}
Either should work the same. Pick one depending on your requirements I guess..
If you add the following toString
method to your Code
class:
@Override
public String toString() {
return "Code{" +
"type='" + type + ''' +
", description='" + description + ''' +
'}';
}
.. with the modified getGroupingKey
method (or the getType
method) the output should be as follows:
{abc~=[Code{type='', description='abc'}, Code{type='null', description='abc'}]}
1
Edit: You can also considering initializing the type to an empty String instead of null
, then you would not need to modify anything:
private String type = "";
That might be an option too..
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440190%2fconsider-null-and-empty-records-as-same-in-collectors-groupingby%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can modify your getGroupingKey
method like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + (code.getType() == null ? "" : code.getType());
}
or like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + Optional.ofNullable(code.getType()).orElse("");
}
or you might as well modify your getType()
method directly as in:
public String getType() {
return type == null ? "" : type;
}
or:
public String getType() {
return Optional.ofNullable(type).orElse("");
}
Either should work the same. Pick one depending on your requirements I guess..
If you add the following toString
method to your Code
class:
@Override
public String toString() {
return "Code{" +
"type='" + type + ''' +
", description='" + description + ''' +
'}';
}
.. with the modified getGroupingKey
method (or the getType
method) the output should be as follows:
{abc~=[Code{type='', description='abc'}, Code{type='null', description='abc'}]}
1
Edit: You can also considering initializing the type to an empty String instead of null
, then you would not need to modify anything:
private String type = "";
That might be an option too..
add a comment |
You can modify your getGroupingKey
method like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + (code.getType() == null ? "" : code.getType());
}
or like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + Optional.ofNullable(code.getType()).orElse("");
}
or you might as well modify your getType()
method directly as in:
public String getType() {
return type == null ? "" : type;
}
or:
public String getType() {
return Optional.ofNullable(type).orElse("");
}
Either should work the same. Pick one depending on your requirements I guess..
If you add the following toString
method to your Code
class:
@Override
public String toString() {
return "Code{" +
"type='" + type + ''' +
", description='" + description + ''' +
'}';
}
.. with the modified getGroupingKey
method (or the getType
method) the output should be as follows:
{abc~=[Code{type='', description='abc'}, Code{type='null', description='abc'}]}
1
Edit: You can also considering initializing the type to an empty String instead of null
, then you would not need to modify anything:
private String type = "";
That might be an option too..
add a comment |
You can modify your getGroupingKey
method like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + (code.getType() == null ? "" : code.getType());
}
or like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + Optional.ofNullable(code.getType()).orElse("");
}
or you might as well modify your getType()
method directly as in:
public String getType() {
return type == null ? "" : type;
}
or:
public String getType() {
return Optional.ofNullable(type).orElse("");
}
Either should work the same. Pick one depending on your requirements I guess..
If you add the following toString
method to your Code
class:
@Override
public String toString() {
return "Code{" +
"type='" + type + ''' +
", description='" + description + ''' +
'}';
}
.. with the modified getGroupingKey
method (or the getType
method) the output should be as follows:
{abc~=[Code{type='', description='abc'}, Code{type='null', description='abc'}]}
1
Edit: You can also considering initializing the type to an empty String instead of null
, then you would not need to modify anything:
private String type = "";
That might be an option too..
You can modify your getGroupingKey
method like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + (code.getType() == null ? "" : code.getType());
}
or like this:
private static String getGroupingKey(Code code) {
return code.getDescription() + "~" + Optional.ofNullable(code.getType()).orElse("");
}
or you might as well modify your getType()
method directly as in:
public String getType() {
return type == null ? "" : type;
}
or:
public String getType() {
return Optional.ofNullable(type).orElse("");
}
Either should work the same. Pick one depending on your requirements I guess..
If you add the following toString
method to your Code
class:
@Override
public String toString() {
return "Code{" +
"type='" + type + ''' +
", description='" + description + ''' +
'}';
}
.. with the modified getGroupingKey
method (or the getType
method) the output should be as follows:
{abc~=[Code{type='', description='abc'}, Code{type='null', description='abc'}]}
1
Edit: You can also considering initializing the type to an empty String instead of null
, then you would not need to modify anything:
private String type = "";
That might be an option too..
edited Nov 23 '18 at 4:17
answered Nov 23 '18 at 3:14


Koray Tugay
8,68826111219
8,68826111219
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440190%2fconsider-null-and-empty-records-as-same-in-collectors-groupingby%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JbMWRKt1nbVgI,ca T1