How can I find a circle angle from x and y axis
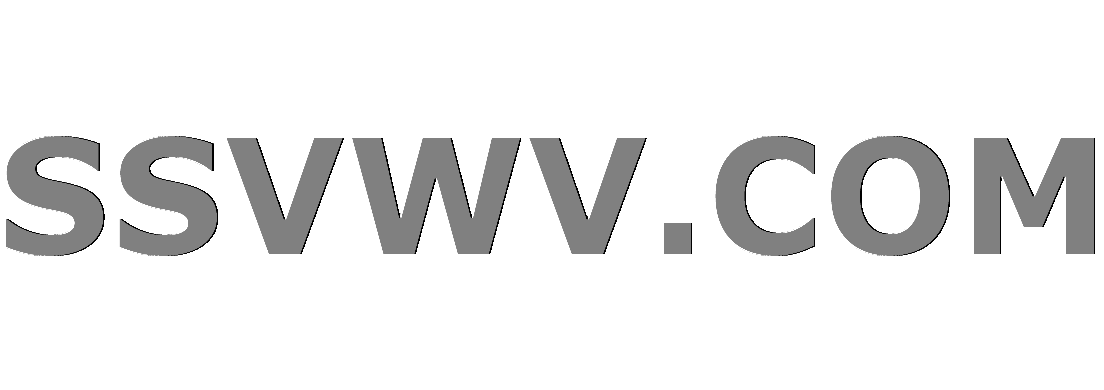
Multi tool use
up vote
0
down vote
favorite
Feel free to mark my question duplicated. Because I know absolute nothing about COS, SIN, and TAN and someone else probably already ask this question.
So, I was try to set the circular progress bar based on x and y axis that can get from gamepad input. The progress bar put it simple is just a Minimum of 0 and maximum of 360.
I did try to search a bit, but my best understanding is that it work with only 180 degree and positive x and y. But the input I get from the controller is and y from -1 to 1 (where x -1 is left and 1 is right, y -1 is bottom and 1 is top)
Here is my code so far.
var controller = Windows.Gaming.Input.Gamepad.Gamepads[0].GetCurrentReading();
x = controller.LeftThumbstickX
y = controller.LeftThumbstickY
//what do I have to do from here?
progress.Value = angle; //?
c# windows math uwp trigonometry
add a comment |
up vote
0
down vote
favorite
Feel free to mark my question duplicated. Because I know absolute nothing about COS, SIN, and TAN and someone else probably already ask this question.
So, I was try to set the circular progress bar based on x and y axis that can get from gamepad input. The progress bar put it simple is just a Minimum of 0 and maximum of 360.
I did try to search a bit, but my best understanding is that it work with only 180 degree and positive x and y. But the input I get from the controller is and y from -1 to 1 (where x -1 is left and 1 is right, y -1 is bottom and 1 is top)
Here is my code so far.
var controller = Windows.Gaming.Input.Gamepad.Gamepads[0].GetCurrentReading();
x = controller.LeftThumbstickX
y = controller.LeftThumbstickY
//what do I have to do from here?
progress.Value = angle; //?
c# windows math uwp trigonometry
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Feel free to mark my question duplicated. Because I know absolute nothing about COS, SIN, and TAN and someone else probably already ask this question.
So, I was try to set the circular progress bar based on x and y axis that can get from gamepad input. The progress bar put it simple is just a Minimum of 0 and maximum of 360.
I did try to search a bit, but my best understanding is that it work with only 180 degree and positive x and y. But the input I get from the controller is and y from -1 to 1 (where x -1 is left and 1 is right, y -1 is bottom and 1 is top)
Here is my code so far.
var controller = Windows.Gaming.Input.Gamepad.Gamepads[0].GetCurrentReading();
x = controller.LeftThumbstickX
y = controller.LeftThumbstickY
//what do I have to do from here?
progress.Value = angle; //?
c# windows math uwp trigonometry
Feel free to mark my question duplicated. Because I know absolute nothing about COS, SIN, and TAN and someone else probably already ask this question.
So, I was try to set the circular progress bar based on x and y axis that can get from gamepad input. The progress bar put it simple is just a Minimum of 0 and maximum of 360.
I did try to search a bit, but my best understanding is that it work with only 180 degree and positive x and y. But the input I get from the controller is and y from -1 to 1 (where x -1 is left and 1 is right, y -1 is bottom and 1 is top)
Here is my code so far.
var controller = Windows.Gaming.Input.Gamepad.Gamepads[0].GetCurrentReading();
x = controller.LeftThumbstickX
y = controller.LeftThumbstickY
//what do I have to do from here?
progress.Value = angle; //?
c# windows math uwp trigonometry
c# windows math uwp trigonometry
asked Nov 21 at 14:09
ToonWK
268
268
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
The trigonometric function atan2
is the tool for this job. In C#, this is implemented by Math.Atan2
:
double angleInRadians = Math.Atan2(y, x);
double angleInDegrees = (180 / Math.PI) * angleInRadians;
Using this formula with (for instance) parameters (1,1)
, you'll get a result of 45.
However, in terms of polar alignment, this angle measures anti-clockwise from "east". To convert this to an angle that measures clockwise from "north":
double compassRadians = Math.PI / 2 - angleInRadians;
double compassDegrees = (180 / Math.PI) * compassRadians;
but now we may encounter negative values, so we can normalize them with the following method:
double normalizeDegrees(double a) => ((a % 360) + 360) % 360; //convert to 0-360
then
var compassAngle = normalizeDegrees(compassDegrees);
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just addif (x == 0 && y == 0) return 0;
or is there something in that formula that can change?
– ToonWK
Nov 21 at 15:52
@ToonWK Yes, I think you'll have to make a special case for0,0
as the maths probably breaks down at 0.
– spender
Nov 21 at 18:44
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters toatan2
withdouble compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
add a comment |
up vote
1
down vote
The method you want is Math.Atan2
. This takes two arguments - the y-value first, then the x-value - and it gives you an angle in radians.
Since you want an angle in degrees, you'll need to convert - the conversion factor is 180 / Math.PI
. So you'll be using something like:
var radiansToDegrees = 180 / Math.PI;
progress.Value = Math.Atan2(y,x) * radiansToDegrees;
Depending exactly what combination of x and y needs to correspond to 0 you might need to add a number of degrees on afterwards. This as-is will give you 0 degrees for x = 1, y = 0, and 90 degrees for x = 0, y = 1, etc.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The trigonometric function atan2
is the tool for this job. In C#, this is implemented by Math.Atan2
:
double angleInRadians = Math.Atan2(y, x);
double angleInDegrees = (180 / Math.PI) * angleInRadians;
Using this formula with (for instance) parameters (1,1)
, you'll get a result of 45.
However, in terms of polar alignment, this angle measures anti-clockwise from "east". To convert this to an angle that measures clockwise from "north":
double compassRadians = Math.PI / 2 - angleInRadians;
double compassDegrees = (180 / Math.PI) * compassRadians;
but now we may encounter negative values, so we can normalize them with the following method:
double normalizeDegrees(double a) => ((a % 360) + 360) % 360; //convert to 0-360
then
var compassAngle = normalizeDegrees(compassDegrees);
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just addif (x == 0 && y == 0) return 0;
or is there something in that formula that can change?
– ToonWK
Nov 21 at 15:52
@ToonWK Yes, I think you'll have to make a special case for0,0
as the maths probably breaks down at 0.
– spender
Nov 21 at 18:44
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters toatan2
withdouble compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
add a comment |
up vote
1
down vote
accepted
The trigonometric function atan2
is the tool for this job. In C#, this is implemented by Math.Atan2
:
double angleInRadians = Math.Atan2(y, x);
double angleInDegrees = (180 / Math.PI) * angleInRadians;
Using this formula with (for instance) parameters (1,1)
, you'll get a result of 45.
However, in terms of polar alignment, this angle measures anti-clockwise from "east". To convert this to an angle that measures clockwise from "north":
double compassRadians = Math.PI / 2 - angleInRadians;
double compassDegrees = (180 / Math.PI) * compassRadians;
but now we may encounter negative values, so we can normalize them with the following method:
double normalizeDegrees(double a) => ((a % 360) + 360) % 360; //convert to 0-360
then
var compassAngle = normalizeDegrees(compassDegrees);
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just addif (x == 0 && y == 0) return 0;
or is there something in that formula that can change?
– ToonWK
Nov 21 at 15:52
@ToonWK Yes, I think you'll have to make a special case for0,0
as the maths probably breaks down at 0.
– spender
Nov 21 at 18:44
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters toatan2
withdouble compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The trigonometric function atan2
is the tool for this job. In C#, this is implemented by Math.Atan2
:
double angleInRadians = Math.Atan2(y, x);
double angleInDegrees = (180 / Math.PI) * angleInRadians;
Using this formula with (for instance) parameters (1,1)
, you'll get a result of 45.
However, in terms of polar alignment, this angle measures anti-clockwise from "east". To convert this to an angle that measures clockwise from "north":
double compassRadians = Math.PI / 2 - angleInRadians;
double compassDegrees = (180 / Math.PI) * compassRadians;
but now we may encounter negative values, so we can normalize them with the following method:
double normalizeDegrees(double a) => ((a % 360) + 360) % 360; //convert to 0-360
then
var compassAngle = normalizeDegrees(compassDegrees);
The trigonometric function atan2
is the tool for this job. In C#, this is implemented by Math.Atan2
:
double angleInRadians = Math.Atan2(y, x);
double angleInDegrees = (180 / Math.PI) * angleInRadians;
Using this formula with (for instance) parameters (1,1)
, you'll get a result of 45.
However, in terms of polar alignment, this angle measures anti-clockwise from "east". To convert this to an angle that measures clockwise from "north":
double compassRadians = Math.PI / 2 - angleInRadians;
double compassDegrees = (180 / Math.PI) * compassRadians;
but now we may encounter negative values, so we can normalize them with the following method:
double normalizeDegrees(double a) => ((a % 360) + 360) % 360; //convert to 0-360
then
var compassAngle = normalizeDegrees(compassDegrees);
edited Nov 21 at 14:35
answered Nov 21 at 14:14


spender
85.8k21157276
85.8k21157276
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just addif (x == 0 && y == 0) return 0;
or is there something in that formula that can change?
– ToonWK
Nov 21 at 15:52
@ToonWK Yes, I think you'll have to make a special case for0,0
as the maths probably breaks down at 0.
– spender
Nov 21 at 18:44
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters toatan2
withdouble compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
add a comment |
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just addif (x == 0 && y == 0) return 0;
or is there something in that formula that can change?
– ToonWK
Nov 21 at 15:52
@ToonWK Yes, I think you'll have to make a special case for0,0
as the maths probably breaks down at 0.
– spender
Nov 21 at 18:44
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters toatan2
withdouble compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just add
if (x == 0 && y == 0) return 0;
or is there something in that formula that can change?– ToonWK
Nov 21 at 15:52
Well that was very insightful answer. Thank you. But I was tested this right now, and it default (0,0) to 90 degree. Even though, I wanted it to be 0. Should I just add
if (x == 0 && y == 0) return 0;
or is there something in that formula that can change?– ToonWK
Nov 21 at 15:52
@ToonWK Yes, I think you'll have to make a special case for
0,0
as the maths probably breaks down at 0.– spender
Nov 21 at 18:44
@ToonWK Yes, I think you'll have to make a special case for
0,0
as the maths probably breaks down at 0.– spender
Nov 21 at 18:44
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters to
atan2
with double compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
@ToonWK Btw, you can avoid some of the maths here by flipping the parameters to
atan2
with double compassRadians = Math.Atan2(x,y);
– spender
Nov 21 at 18:51
add a comment |
up vote
1
down vote
The method you want is Math.Atan2
. This takes two arguments - the y-value first, then the x-value - and it gives you an angle in radians.
Since you want an angle in degrees, you'll need to convert - the conversion factor is 180 / Math.PI
. So you'll be using something like:
var radiansToDegrees = 180 / Math.PI;
progress.Value = Math.Atan2(y,x) * radiansToDegrees;
Depending exactly what combination of x and y needs to correspond to 0 you might need to add a number of degrees on afterwards. This as-is will give you 0 degrees for x = 1, y = 0, and 90 degrees for x = 0, y = 1, etc.
add a comment |
up vote
1
down vote
The method you want is Math.Atan2
. This takes two arguments - the y-value first, then the x-value - and it gives you an angle in radians.
Since you want an angle in degrees, you'll need to convert - the conversion factor is 180 / Math.PI
. So you'll be using something like:
var radiansToDegrees = 180 / Math.PI;
progress.Value = Math.Atan2(y,x) * radiansToDegrees;
Depending exactly what combination of x and y needs to correspond to 0 you might need to add a number of degrees on afterwards. This as-is will give you 0 degrees for x = 1, y = 0, and 90 degrees for x = 0, y = 1, etc.
add a comment |
up vote
1
down vote
up vote
1
down vote
The method you want is Math.Atan2
. This takes two arguments - the y-value first, then the x-value - and it gives you an angle in radians.
Since you want an angle in degrees, you'll need to convert - the conversion factor is 180 / Math.PI
. So you'll be using something like:
var radiansToDegrees = 180 / Math.PI;
progress.Value = Math.Atan2(y,x) * radiansToDegrees;
Depending exactly what combination of x and y needs to correspond to 0 you might need to add a number of degrees on afterwards. This as-is will give you 0 degrees for x = 1, y = 0, and 90 degrees for x = 0, y = 1, etc.
The method you want is Math.Atan2
. This takes two arguments - the y-value first, then the x-value - and it gives you an angle in radians.
Since you want an angle in degrees, you'll need to convert - the conversion factor is 180 / Math.PI
. So you'll be using something like:
var radiansToDegrees = 180 / Math.PI;
progress.Value = Math.Atan2(y,x) * radiansToDegrees;
Depending exactly what combination of x and y needs to correspond to 0 you might need to add a number of degrees on afterwards. This as-is will give you 0 degrees for x = 1, y = 0, and 90 degrees for x = 0, y = 1, etc.
answered Nov 21 at 14:15
T. Linnell
22514
22514
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53413924%2fhow-can-i-find-a-circle-angle-from-x-and-y-axis%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rB6GhHTWrvkJHAv fI4,EXMqM0 ip5qc rAAdtdawKuQ8yObo