Connection string for SQL Server ( local database )
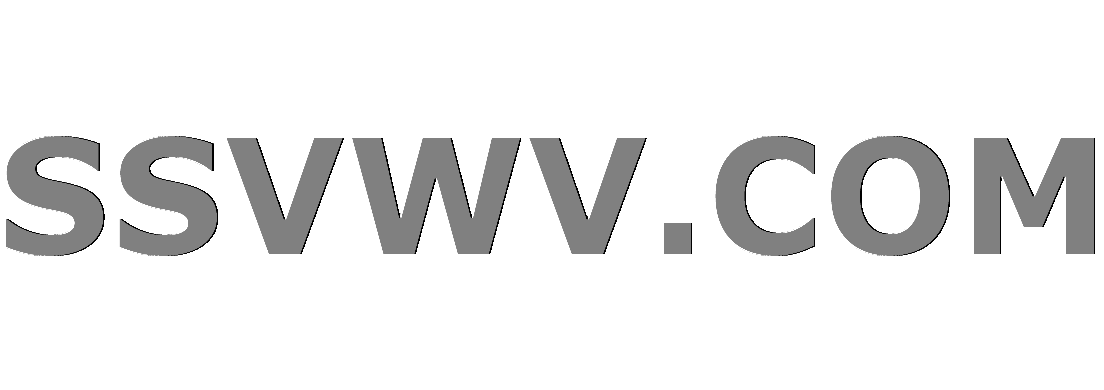
Multi tool use
up vote
2
down vote
favorite
I added a local database to my application in Visual Studio:
database
and I need connection string for it - here it is:
SqlConnection conn = new SqlConnection();
conn.ConnectionString = "Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
When I run that block of code, the whole UI thread stops, like infinite for loop. What is wrong with my connection string?
I'm working with Windows Forms, C#, .NET Framework version 4.5.1
c#

add a comment |
up vote
2
down vote
favorite
I added a local database to my application in Visual Studio:
database
and I need connection string for it - here it is:
SqlConnection conn = new SqlConnection();
conn.ConnectionString = "Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
When I run that block of code, the whole UI thread stops, like infinite for loop. What is wrong with my connection string?
I'm working with Windows Forms, C#, .NET Framework version 4.5.1
c#

1
Going by the screenshot it looks like you're trying to connect to a database file (.mdf) and not to a server instance. ifData Source=<yourcomputername>
doesn't work then try putting the path to the mdf file. E.g.Data Source=C:DataFilters.mdf
. I'm guessing that the reason the UI thread stops is because the default connection timeout is 30 seconds, so the connection has 30 seconds to respond before .net throws an exception.
– Handbag Crab
Nov 21 at 21:03
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I added a local database to my application in Visual Studio:
database
and I need connection string for it - here it is:
SqlConnection conn = new SqlConnection();
conn.ConnectionString = "Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
When I run that block of code, the whole UI thread stops, like infinite for loop. What is wrong with my connection string?
I'm working with Windows Forms, C#, .NET Framework version 4.5.1
c#

I added a local database to my application in Visual Studio:
database
and I need connection string for it - here it is:
SqlConnection conn = new SqlConnection();
conn.ConnectionString = "Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
When I run that block of code, the whole UI thread stops, like infinite for loop. What is wrong with my connection string?
I'm working with Windows Forms, C#, .NET Framework version 4.5.1
c#

c#

edited Nov 21 at 21:42
marc_s
568k12810991249
568k12810991249
asked Nov 21 at 20:46


Aleksa Djuric
132
132
1
Going by the screenshot it looks like you're trying to connect to a database file (.mdf) and not to a server instance. ifData Source=<yourcomputername>
doesn't work then try putting the path to the mdf file. E.g.Data Source=C:DataFilters.mdf
. I'm guessing that the reason the UI thread stops is because the default connection timeout is 30 seconds, so the connection has 30 seconds to respond before .net throws an exception.
– Handbag Crab
Nov 21 at 21:03
add a comment |
1
Going by the screenshot it looks like you're trying to connect to a database file (.mdf) and not to a server instance. ifData Source=<yourcomputername>
doesn't work then try putting the path to the mdf file. E.g.Data Source=C:DataFilters.mdf
. I'm guessing that the reason the UI thread stops is because the default connection timeout is 30 seconds, so the connection has 30 seconds to respond before .net throws an exception.
– Handbag Crab
Nov 21 at 21:03
1
1
Going by the screenshot it looks like you're trying to connect to a database file (.mdf) and not to a server instance. if
Data Source=<yourcomputername>
doesn't work then try putting the path to the mdf file. E.g. Data Source=C:DataFilters.mdf
. I'm guessing that the reason the UI thread stops is because the default connection timeout is 30 seconds, so the connection has 30 seconds to respond before .net throws an exception.– Handbag Crab
Nov 21 at 21:03
Going by the screenshot it looks like you're trying to connect to a database file (.mdf) and not to a server instance. if
Data Source=<yourcomputername>
doesn't work then try putting the path to the mdf file. E.g. Data Source=C:DataFilters.mdf
. I'm guessing that the reason the UI thread stops is because the default connection timeout is 30 seconds, so the connection has 30 seconds to respond before .net throws an exception.– Handbag Crab
Nov 21 at 21:03
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
Part of your problem is you have a trailing '.' in your IP address. Remove that like so:
"Data Source=127.0.0.1;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
Also, I would strongly suggest that you wrap your connection object in a using statement like this:
using (SqlConnection conn = new SqlConnection())
{
conn.ConnectionString =
"Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
}
Lastly, define your connection in a string and pass it into your SqlConnection
object when you instantiate it, like this:
string sqlConnection = "Data Source=127.0.0.1;Initial Catalog=Filter;Integrated Security=SSPI;"
using (SqlConnection conn = new SqlConnection(sqlConnection)
{
conn.Open();
}
This approach does several things for you:
- It makes your code much, much easier to read, and clean.
- It ensures that your connection object will get handled by
Dispose
even if there is an exception thrown in theusing
block. - It is just a good habit to get into early.
More on the SqlConnection
class here, and more on using
can be found here.
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
See the comment above, in your original question. Are you pointing to the right (or any).mdf
file?
– Brian
Nov 21 at 21:05
add a comment |
up vote
1
down vote
Remove the last dot of the IP address.
"Data Source=127.0.0.1.;" +
Should be:
"Data Source=127.0.0.1;" +
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
2
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Part of your problem is you have a trailing '.' in your IP address. Remove that like so:
"Data Source=127.0.0.1;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
Also, I would strongly suggest that you wrap your connection object in a using statement like this:
using (SqlConnection conn = new SqlConnection())
{
conn.ConnectionString =
"Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
}
Lastly, define your connection in a string and pass it into your SqlConnection
object when you instantiate it, like this:
string sqlConnection = "Data Source=127.0.0.1;Initial Catalog=Filter;Integrated Security=SSPI;"
using (SqlConnection conn = new SqlConnection(sqlConnection)
{
conn.Open();
}
This approach does several things for you:
- It makes your code much, much easier to read, and clean.
- It ensures that your connection object will get handled by
Dispose
even if there is an exception thrown in theusing
block. - It is just a good habit to get into early.
More on the SqlConnection
class here, and more on using
can be found here.
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
See the comment above, in your original question. Are you pointing to the right (or any).mdf
file?
– Brian
Nov 21 at 21:05
add a comment |
up vote
1
down vote
accepted
Part of your problem is you have a trailing '.' in your IP address. Remove that like so:
"Data Source=127.0.0.1;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
Also, I would strongly suggest that you wrap your connection object in a using statement like this:
using (SqlConnection conn = new SqlConnection())
{
conn.ConnectionString =
"Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
}
Lastly, define your connection in a string and pass it into your SqlConnection
object when you instantiate it, like this:
string sqlConnection = "Data Source=127.0.0.1;Initial Catalog=Filter;Integrated Security=SSPI;"
using (SqlConnection conn = new SqlConnection(sqlConnection)
{
conn.Open();
}
This approach does several things for you:
- It makes your code much, much easier to read, and clean.
- It ensures that your connection object will get handled by
Dispose
even if there is an exception thrown in theusing
block. - It is just a good habit to get into early.
More on the SqlConnection
class here, and more on using
can be found here.
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
See the comment above, in your original question. Are you pointing to the right (or any).mdf
file?
– Brian
Nov 21 at 21:05
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Part of your problem is you have a trailing '.' in your IP address. Remove that like so:
"Data Source=127.0.0.1;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
Also, I would strongly suggest that you wrap your connection object in a using statement like this:
using (SqlConnection conn = new SqlConnection())
{
conn.ConnectionString =
"Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
}
Lastly, define your connection in a string and pass it into your SqlConnection
object when you instantiate it, like this:
string sqlConnection = "Data Source=127.0.0.1;Initial Catalog=Filter;Integrated Security=SSPI;"
using (SqlConnection conn = new SqlConnection(sqlConnection)
{
conn.Open();
}
This approach does several things for you:
- It makes your code much, much easier to read, and clean.
- It ensures that your connection object will get handled by
Dispose
even if there is an exception thrown in theusing
block. - It is just a good habit to get into early.
More on the SqlConnection
class here, and more on using
can be found here.
Part of your problem is you have a trailing '.' in your IP address. Remove that like so:
"Data Source=127.0.0.1;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
Also, I would strongly suggest that you wrap your connection object in a using statement like this:
using (SqlConnection conn = new SqlConnection())
{
conn.ConnectionString =
"Data Source=127.0.0.1.;" +
"Initial Catalog=Filter;" +
"Integrated Security=SSPI;";
conn.Open();
}
Lastly, define your connection in a string and pass it into your SqlConnection
object when you instantiate it, like this:
string sqlConnection = "Data Source=127.0.0.1;Initial Catalog=Filter;Integrated Security=SSPI;"
using (SqlConnection conn = new SqlConnection(sqlConnection)
{
conn.Open();
}
This approach does several things for you:
- It makes your code much, much easier to read, and clean.
- It ensures that your connection object will get handled by
Dispose
even if there is an exception thrown in theusing
block. - It is just a good habit to get into early.
More on the SqlConnection
class here, and more on using
can be found here.
edited Nov 21 at 22:20
answered Nov 21 at 21:01


Brian
4,58672640
4,58672640
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
See the comment above, in your original question. Are you pointing to the right (or any).mdf
file?
– Brian
Nov 21 at 21:05
add a comment |
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
See the comment above, in your original question. Are you pointing to the right (or any).mdf
file?
– Brian
Nov 21 at 21:05
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
I removed '.' and still don't work
– Aleksa Djuric
Nov 21 at 21:04
See the comment above, in your original question. Are you pointing to the right (or any)
.mdf
file?– Brian
Nov 21 at 21:05
See the comment above, in your original question. Are you pointing to the right (or any)
.mdf
file?– Brian
Nov 21 at 21:05
add a comment |
up vote
1
down vote
Remove the last dot of the IP address.
"Data Source=127.0.0.1.;" +
Should be:
"Data Source=127.0.0.1;" +
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
2
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
add a comment |
up vote
1
down vote
Remove the last dot of the IP address.
"Data Source=127.0.0.1.;" +
Should be:
"Data Source=127.0.0.1;" +
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
2
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
add a comment |
up vote
1
down vote
up vote
1
down vote
Remove the last dot of the IP address.
"Data Source=127.0.0.1.;" +
Should be:
"Data Source=127.0.0.1;" +
Remove the last dot of the IP address.
"Data Source=127.0.0.1.;" +
Should be:
"Data Source=127.0.0.1;" +
edited Nov 21 at 20:50


Brian
4,58672640
4,58672640
answered Nov 21 at 20:49
MLeblanc
46426
46426
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
2
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
add a comment |
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
2
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
Still don't work
– Aleksa Djuric
Nov 21 at 20:59
2
2
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
Make sure sql server is up and running and you can connect to the database using windows authentication with SSMS. If it works, see connectionstrings.com/sql-server for proper syntax
– MLeblanc
Nov 21 at 21:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53420230%2fconnection-string-for-sql-server-local-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KeKmeSCYRIEIQ3LYe4S3qOZd 9Z9qW2xchQAR0eDNrHApVmj6Nb
1
Going by the screenshot it looks like you're trying to connect to a database file (.mdf) and not to a server instance. if
Data Source=<yourcomputername>
doesn't work then try putting the path to the mdf file. E.g.Data Source=C:DataFilters.mdf
. I'm guessing that the reason the UI thread stops is because the default connection timeout is 30 seconds, so the connection has 30 seconds to respond before .net throws an exception.– Handbag Crab
Nov 21 at 21:03