Add capital letters to a list in angular every time the first letter changes
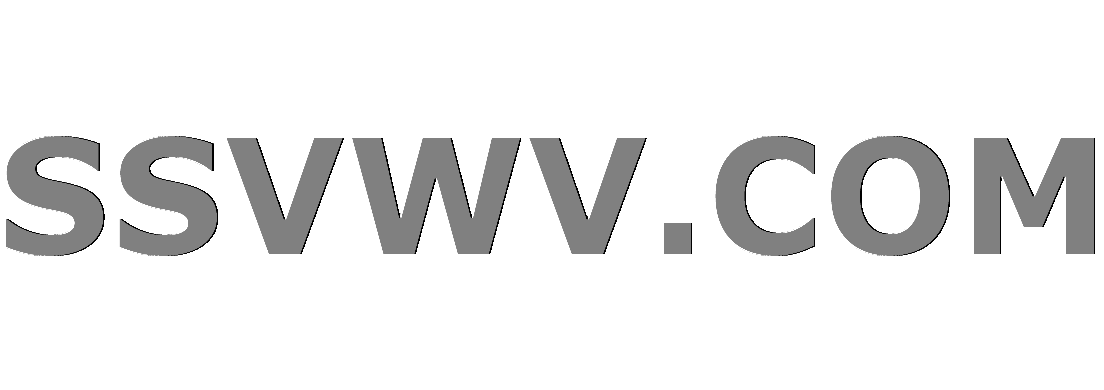
Multi tool use
I have a list of architects.
Every time when an architect begins with a new letter, I should add a single letter, to structure the list of architects:
Example:
A
ABC Architects
Arctical Architects
B
Boston Architects
D
Dutch Architects
etc.
my actual code:
<ul class="architect-list">
<li *ngFor="let architect of architects">
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ul>
How can I add the letters without modifying the list?
Remark: the Letters should not be linked.
angular
add a comment |
I have a list of architects.
Every time when an architect begins with a new letter, I should add a single letter, to structure the list of architects:
Example:
A
ABC Architects
Arctical Architects
B
Boston Architects
D
Dutch Architects
etc.
my actual code:
<ul class="architect-list">
<li *ngFor="let architect of architects">
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ul>
How can I add the letters without modifying the list?
Remark: the Letters should not be linked.
angular
And you can have any number of architects starting with an alphabet right?
– SiddAjmera
Nov 23 '18 at 11:42
add a comment |
I have a list of architects.
Every time when an architect begins with a new letter, I should add a single letter, to structure the list of architects:
Example:
A
ABC Architects
Arctical Architects
B
Boston Architects
D
Dutch Architects
etc.
my actual code:
<ul class="architect-list">
<li *ngFor="let architect of architects">
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ul>
How can I add the letters without modifying the list?
Remark: the Letters should not be linked.
angular
I have a list of architects.
Every time when an architect begins with a new letter, I should add a single letter, to structure the list of architects:
Example:
A
ABC Architects
Arctical Architects
B
Boston Architects
D
Dutch Architects
etc.
my actual code:
<ul class="architect-list">
<li *ngFor="let architect of architects">
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ul>
How can I add the letters without modifying the list?
Remark: the Letters should not be linked.
angular
angular
edited Nov 23 '18 at 14:36


R. Richards
14k93441
14k93441
asked Nov 23 '18 at 11:41
AnotherArtAnotherArt
33
33
And you can have any number of architects starting with an alphabet right?
– SiddAjmera
Nov 23 '18 at 11:42
add a comment |
And you can have any number of architects starting with an alphabet right?
– SiddAjmera
Nov 23 '18 at 11:42
And you can have any number of architects starting with an alphabet right?
– SiddAjmera
Nov 23 '18 at 11:42
And you can have any number of architects starting with an alphabet right?
– SiddAjmera
Nov 23 '18 at 11:42
add a comment |
4 Answers
4
active
oldest
votes
You can create a method that would return to you a boolean to decide if the placeholder alphabet should be displayed or not. This is should do by comparing the first character of each architect name with a property on the Component. It should also make sure to update that property as soon as a name with a new alphabet starts in the list.
Give this a try:
<ul class="architect-list">
<ng-container *ngFor="let architect of architects">
<ng-container *ngIf="checkIfNew(architect.title)">
{{ architect.title.charAt(0).toUpperCase() }}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
</ul>
Component Class:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
currentAlphabet;
architects = [...];
checkIfNew(title: string) {
if(this.currentAlphabet === title.charAt(0).toLowerCase()) {
return false;
} else {
this.currentAlphabet = title.charAt(0).toLowerCase();
return true;
}
}
}
Here's a Sample StackBlitz for your ref.
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
add a comment |
You can achieve this by writing the small function in your Component and calling the same from the HTML. But at the same time this is not the optimal solution from the performance point of view and you need to move this logic to the custom directive. so here is the answer.
The working stackblitz example is here.
code required in component
checkLetter(architect: any, i: number) {
let Initial = '';
if (i === 0) {
Initial = this.architects[0].title.charAt(0);
} else {
if (this.architects[i].title.charAt(0) === this.architects[i - 1].title.charAt(0)) {
Initial = '';
}
else {
Initial = this.architects[i].title.charAt(0);
}
}
return Initial;
}
code required in html
<ul class="architect-list">
<ng-container *ngFor="let architect of architects; let i = index">
<ng-container>
{{checkLetter(architect, i)}}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
add a comment |
Here is a sample stack blitz I through together with a similar array of data.
in your template, you can get the previous item in your array by using the index and subtracting one.
<ul class="genre-list">
<li *ngFor="let genere of musicGeneres; let index = index;">
<div *ngIf="isStartingAlaphet(genere, musicGeneres[index - 1])">
{{ genere[0] }}
</div>
<div [innerHTML]="genere">
</div>
</li>
</ul>
then have a small pure function that checks first if the previous item exists, if it doesn't' then we are on A
and display it. If not then compare the current items first letter to the previous items first letter.
isStartingAlaphet(current, previous){
// if there is no previous one then a
if(!previous){
return current[0]
}
return current[0] !== previous[0]
}
This depends on your list being in Alphabetical order but it also keeps any messy code where your component has to "track" the previous item and rely on impure functions.
To use this type of solution with your more complex array you simply need to pass in the titles:
<div *ngIf="isStartingAlaphet(architect.title, architects[index - 1]?.title)">
{{ architect.title[0] }}
</div>
https://stackblitz.com/edit/angular-mw7tp2
add a comment |
You could use reduce in the component, assuming that the original architect's list is an array:
const objectkeys = Object.keys;
const list = ['Aaarchitext', 'Brand New Architect', 'Clydesdale Architect'];
const newList = this.getReorderedList();
function getReorderedList(): void {
return list.reduce((grp, a, b) => {
if (!grp[a.slice(0,1)]) {
grp[a.slice(0,1)] = ;
}
grp[a.slice(0,1)].push(a);
return grp;
}, {});
}
Then in your template:
<ul class="architect-list">
<ng-container *ngFor="let firstLetter of objectkeys(newList)">
<li>{{ firstLetter }}</li>
<ng-container *ngFor="let architect of newList[firstLetter]">
<div
class="single-architect"
(click)="selectArchitect(architect.title)"
[innerHTML]="architect.title">
</div>
</ng-container>
</ng-container>
</ul>
That should solve your issue. You also only have to call the method just once in the beginning (ie in your ngOnInit()), no constant calling to the component.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446037%2fadd-capital-letters-to-a-list-in-angular-every-time-the-first-letter-changes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can create a method that would return to you a boolean to decide if the placeholder alphabet should be displayed or not. This is should do by comparing the first character of each architect name with a property on the Component. It should also make sure to update that property as soon as a name with a new alphabet starts in the list.
Give this a try:
<ul class="architect-list">
<ng-container *ngFor="let architect of architects">
<ng-container *ngIf="checkIfNew(architect.title)">
{{ architect.title.charAt(0).toUpperCase() }}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
</ul>
Component Class:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
currentAlphabet;
architects = [...];
checkIfNew(title: string) {
if(this.currentAlphabet === title.charAt(0).toLowerCase()) {
return false;
} else {
this.currentAlphabet = title.charAt(0).toLowerCase();
return true;
}
}
}
Here's a Sample StackBlitz for your ref.
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
add a comment |
You can create a method that would return to you a boolean to decide if the placeholder alphabet should be displayed or not. This is should do by comparing the first character of each architect name with a property on the Component. It should also make sure to update that property as soon as a name with a new alphabet starts in the list.
Give this a try:
<ul class="architect-list">
<ng-container *ngFor="let architect of architects">
<ng-container *ngIf="checkIfNew(architect.title)">
{{ architect.title.charAt(0).toUpperCase() }}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
</ul>
Component Class:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
currentAlphabet;
architects = [...];
checkIfNew(title: string) {
if(this.currentAlphabet === title.charAt(0).toLowerCase()) {
return false;
} else {
this.currentAlphabet = title.charAt(0).toLowerCase();
return true;
}
}
}
Here's a Sample StackBlitz for your ref.
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
add a comment |
You can create a method that would return to you a boolean to decide if the placeholder alphabet should be displayed or not. This is should do by comparing the first character of each architect name with a property on the Component. It should also make sure to update that property as soon as a name with a new alphabet starts in the list.
Give this a try:
<ul class="architect-list">
<ng-container *ngFor="let architect of architects">
<ng-container *ngIf="checkIfNew(architect.title)">
{{ architect.title.charAt(0).toUpperCase() }}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
</ul>
Component Class:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
currentAlphabet;
architects = [...];
checkIfNew(title: string) {
if(this.currentAlphabet === title.charAt(0).toLowerCase()) {
return false;
} else {
this.currentAlphabet = title.charAt(0).toLowerCase();
return true;
}
}
}
Here's a Sample StackBlitz for your ref.
You can create a method that would return to you a boolean to decide if the placeholder alphabet should be displayed or not. This is should do by comparing the first character of each architect name with a property on the Component. It should also make sure to update that property as soon as a name with a new alphabet starts in the list.
Give this a try:
<ul class="architect-list">
<ng-container *ngFor="let architect of architects">
<ng-container *ngIf="checkIfNew(architect.title)">
{{ architect.title.charAt(0).toUpperCase() }}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
</ul>
Component Class:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
currentAlphabet;
architects = [...];
checkIfNew(title: string) {
if(this.currentAlphabet === title.charAt(0).toLowerCase()) {
return false;
} else {
this.currentAlphabet = title.charAt(0).toLowerCase();
return true;
}
}
}
Here's a Sample StackBlitz for your ref.
edited Nov 23 '18 at 12:14
answered Nov 23 '18 at 11:50
SiddAjmeraSiddAjmera
13.3k31137
13.3k31137
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
add a comment |
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
@AnotherArt : You should accept this answer.
– Kedar9444
Nov 23 '18 at 12:17
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
Thanks a lot for your help. It works perfect ;DD
– AnotherArt
Nov 24 '18 at 15:03
add a comment |
You can achieve this by writing the small function in your Component and calling the same from the HTML. But at the same time this is not the optimal solution from the performance point of view and you need to move this logic to the custom directive. so here is the answer.
The working stackblitz example is here.
code required in component
checkLetter(architect: any, i: number) {
let Initial = '';
if (i === 0) {
Initial = this.architects[0].title.charAt(0);
} else {
if (this.architects[i].title.charAt(0) === this.architects[i - 1].title.charAt(0)) {
Initial = '';
}
else {
Initial = this.architects[i].title.charAt(0);
}
}
return Initial;
}
code required in html
<ul class="architect-list">
<ng-container *ngFor="let architect of architects; let i = index">
<ng-container>
{{checkLetter(architect, i)}}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
add a comment |
You can achieve this by writing the small function in your Component and calling the same from the HTML. But at the same time this is not the optimal solution from the performance point of view and you need to move this logic to the custom directive. so here is the answer.
The working stackblitz example is here.
code required in component
checkLetter(architect: any, i: number) {
let Initial = '';
if (i === 0) {
Initial = this.architects[0].title.charAt(0);
} else {
if (this.architects[i].title.charAt(0) === this.architects[i - 1].title.charAt(0)) {
Initial = '';
}
else {
Initial = this.architects[i].title.charAt(0);
}
}
return Initial;
}
code required in html
<ul class="architect-list">
<ng-container *ngFor="let architect of architects; let i = index">
<ng-container>
{{checkLetter(architect, i)}}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
add a comment |
You can achieve this by writing the small function in your Component and calling the same from the HTML. But at the same time this is not the optimal solution from the performance point of view and you need to move this logic to the custom directive. so here is the answer.
The working stackblitz example is here.
code required in component
checkLetter(architect: any, i: number) {
let Initial = '';
if (i === 0) {
Initial = this.architects[0].title.charAt(0);
} else {
if (this.architects[i].title.charAt(0) === this.architects[i - 1].title.charAt(0)) {
Initial = '';
}
else {
Initial = this.architects[i].title.charAt(0);
}
}
return Initial;
}
code required in html
<ul class="architect-list">
<ng-container *ngFor="let architect of architects; let i = index">
<ng-container>
{{checkLetter(architect, i)}}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
You can achieve this by writing the small function in your Component and calling the same from the HTML. But at the same time this is not the optimal solution from the performance point of view and you need to move this logic to the custom directive. so here is the answer.
The working stackblitz example is here.
code required in component
checkLetter(architect: any, i: number) {
let Initial = '';
if (i === 0) {
Initial = this.architects[0].title.charAt(0);
} else {
if (this.architects[i].title.charAt(0) === this.architects[i - 1].title.charAt(0)) {
Initial = '';
}
else {
Initial = this.architects[i].title.charAt(0);
}
}
return Initial;
}
code required in html
<ul class="architect-list">
<ng-container *ngFor="let architect of architects; let i = index">
<ng-container>
{{checkLetter(architect, i)}}
</ng-container>
<li>
<div class="single-architect" (click)="selectArchitect(architect.title)" [innerHTML]="architect.title">
</div>
</li>
</ng-container>
answered Nov 23 '18 at 12:04


Kedar9444Kedar9444
37318
37318
add a comment |
add a comment |
Here is a sample stack blitz I through together with a similar array of data.
in your template, you can get the previous item in your array by using the index and subtracting one.
<ul class="genre-list">
<li *ngFor="let genere of musicGeneres; let index = index;">
<div *ngIf="isStartingAlaphet(genere, musicGeneres[index - 1])">
{{ genere[0] }}
</div>
<div [innerHTML]="genere">
</div>
</li>
</ul>
then have a small pure function that checks first if the previous item exists, if it doesn't' then we are on A
and display it. If not then compare the current items first letter to the previous items first letter.
isStartingAlaphet(current, previous){
// if there is no previous one then a
if(!previous){
return current[0]
}
return current[0] !== previous[0]
}
This depends on your list being in Alphabetical order but it also keeps any messy code where your component has to "track" the previous item and rely on impure functions.
To use this type of solution with your more complex array you simply need to pass in the titles:
<div *ngIf="isStartingAlaphet(architect.title, architects[index - 1]?.title)">
{{ architect.title[0] }}
</div>
https://stackblitz.com/edit/angular-mw7tp2
add a comment |
Here is a sample stack blitz I through together with a similar array of data.
in your template, you can get the previous item in your array by using the index and subtracting one.
<ul class="genre-list">
<li *ngFor="let genere of musicGeneres; let index = index;">
<div *ngIf="isStartingAlaphet(genere, musicGeneres[index - 1])">
{{ genere[0] }}
</div>
<div [innerHTML]="genere">
</div>
</li>
</ul>
then have a small pure function that checks first if the previous item exists, if it doesn't' then we are on A
and display it. If not then compare the current items first letter to the previous items first letter.
isStartingAlaphet(current, previous){
// if there is no previous one then a
if(!previous){
return current[0]
}
return current[0] !== previous[0]
}
This depends on your list being in Alphabetical order but it also keeps any messy code where your component has to "track" the previous item and rely on impure functions.
To use this type of solution with your more complex array you simply need to pass in the titles:
<div *ngIf="isStartingAlaphet(architect.title, architects[index - 1]?.title)">
{{ architect.title[0] }}
</div>
https://stackblitz.com/edit/angular-mw7tp2
add a comment |
Here is a sample stack blitz I through together with a similar array of data.
in your template, you can get the previous item in your array by using the index and subtracting one.
<ul class="genre-list">
<li *ngFor="let genere of musicGeneres; let index = index;">
<div *ngIf="isStartingAlaphet(genere, musicGeneres[index - 1])">
{{ genere[0] }}
</div>
<div [innerHTML]="genere">
</div>
</li>
</ul>
then have a small pure function that checks first if the previous item exists, if it doesn't' then we are on A
and display it. If not then compare the current items first letter to the previous items first letter.
isStartingAlaphet(current, previous){
// if there is no previous one then a
if(!previous){
return current[0]
}
return current[0] !== previous[0]
}
This depends on your list being in Alphabetical order but it also keeps any messy code where your component has to "track" the previous item and rely on impure functions.
To use this type of solution with your more complex array you simply need to pass in the titles:
<div *ngIf="isStartingAlaphet(architect.title, architects[index - 1]?.title)">
{{ architect.title[0] }}
</div>
https://stackblitz.com/edit/angular-mw7tp2
Here is a sample stack blitz I through together with a similar array of data.
in your template, you can get the previous item in your array by using the index and subtracting one.
<ul class="genre-list">
<li *ngFor="let genere of musicGeneres; let index = index;">
<div *ngIf="isStartingAlaphet(genere, musicGeneres[index - 1])">
{{ genere[0] }}
</div>
<div [innerHTML]="genere">
</div>
</li>
</ul>
then have a small pure function that checks first if the previous item exists, if it doesn't' then we are on A
and display it. If not then compare the current items first letter to the previous items first letter.
isStartingAlaphet(current, previous){
// if there is no previous one then a
if(!previous){
return current[0]
}
return current[0] !== previous[0]
}
This depends on your list being in Alphabetical order but it also keeps any messy code where your component has to "track" the previous item and rely on impure functions.
To use this type of solution with your more complex array you simply need to pass in the titles:
<div *ngIf="isStartingAlaphet(architect.title, architects[index - 1]?.title)">
{{ architect.title[0] }}
</div>
https://stackblitz.com/edit/angular-mw7tp2
edited Nov 23 '18 at 12:18
answered Nov 23 '18 at 12:13
JoshSommerJoshSommer
1,325814
1,325814
add a comment |
add a comment |
You could use reduce in the component, assuming that the original architect's list is an array:
const objectkeys = Object.keys;
const list = ['Aaarchitext', 'Brand New Architect', 'Clydesdale Architect'];
const newList = this.getReorderedList();
function getReorderedList(): void {
return list.reduce((grp, a, b) => {
if (!grp[a.slice(0,1)]) {
grp[a.slice(0,1)] = ;
}
grp[a.slice(0,1)].push(a);
return grp;
}, {});
}
Then in your template:
<ul class="architect-list">
<ng-container *ngFor="let firstLetter of objectkeys(newList)">
<li>{{ firstLetter }}</li>
<ng-container *ngFor="let architect of newList[firstLetter]">
<div
class="single-architect"
(click)="selectArchitect(architect.title)"
[innerHTML]="architect.title">
</div>
</ng-container>
</ng-container>
</ul>
That should solve your issue. You also only have to call the method just once in the beginning (ie in your ngOnInit()), no constant calling to the component.
add a comment |
You could use reduce in the component, assuming that the original architect's list is an array:
const objectkeys = Object.keys;
const list = ['Aaarchitext', 'Brand New Architect', 'Clydesdale Architect'];
const newList = this.getReorderedList();
function getReorderedList(): void {
return list.reduce((grp, a, b) => {
if (!grp[a.slice(0,1)]) {
grp[a.slice(0,1)] = ;
}
grp[a.slice(0,1)].push(a);
return grp;
}, {});
}
Then in your template:
<ul class="architect-list">
<ng-container *ngFor="let firstLetter of objectkeys(newList)">
<li>{{ firstLetter }}</li>
<ng-container *ngFor="let architect of newList[firstLetter]">
<div
class="single-architect"
(click)="selectArchitect(architect.title)"
[innerHTML]="architect.title">
</div>
</ng-container>
</ng-container>
</ul>
That should solve your issue. You also only have to call the method just once in the beginning (ie in your ngOnInit()), no constant calling to the component.
add a comment |
You could use reduce in the component, assuming that the original architect's list is an array:
const objectkeys = Object.keys;
const list = ['Aaarchitext', 'Brand New Architect', 'Clydesdale Architect'];
const newList = this.getReorderedList();
function getReorderedList(): void {
return list.reduce((grp, a, b) => {
if (!grp[a.slice(0,1)]) {
grp[a.slice(0,1)] = ;
}
grp[a.slice(0,1)].push(a);
return grp;
}, {});
}
Then in your template:
<ul class="architect-list">
<ng-container *ngFor="let firstLetter of objectkeys(newList)">
<li>{{ firstLetter }}</li>
<ng-container *ngFor="let architect of newList[firstLetter]">
<div
class="single-architect"
(click)="selectArchitect(architect.title)"
[innerHTML]="architect.title">
</div>
</ng-container>
</ng-container>
</ul>
That should solve your issue. You also only have to call the method just once in the beginning (ie in your ngOnInit()), no constant calling to the component.
You could use reduce in the component, assuming that the original architect's list is an array:
const objectkeys = Object.keys;
const list = ['Aaarchitext', 'Brand New Architect', 'Clydesdale Architect'];
const newList = this.getReorderedList();
function getReorderedList(): void {
return list.reduce((grp, a, b) => {
if (!grp[a.slice(0,1)]) {
grp[a.slice(0,1)] = ;
}
grp[a.slice(0,1)].push(a);
return grp;
}, {});
}
Then in your template:
<ul class="architect-list">
<ng-container *ngFor="let firstLetter of objectkeys(newList)">
<li>{{ firstLetter }}</li>
<ng-container *ngFor="let architect of newList[firstLetter]">
<div
class="single-architect"
(click)="selectArchitect(architect.title)"
[innerHTML]="architect.title">
</div>
</ng-container>
</ng-container>
</ul>
That should solve your issue. You also only have to call the method just once in the beginning (ie in your ngOnInit()), no constant calling to the component.
answered Nov 23 '18 at 12:24


rrdrrd
2,85831222
2,85831222
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446037%2fadd-capital-letters-to-a-list-in-angular-every-time-the-first-letter-changes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8850pCKFthxLhclazXHwhtTFY,vTgzUhUMwm 0ry3 wS d6AZBl2njjAPS8,SDeNLx7oX
And you can have any number of architects starting with an alphabet right?
– SiddAjmera
Nov 23 '18 at 11:42