Testing window resize handler on React.Component with Jest & Enzyme
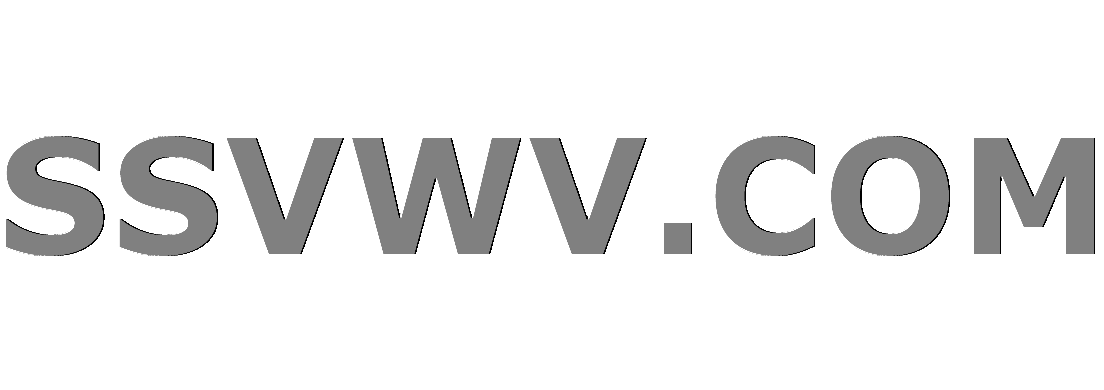
Multi tool use
I'm writing a component that applies some logic on 'resize' event. It basically looks like this:
class MyComponent extends React.Component {
componentDidMount() {
window.addEventListener('resize', this.handleWindowResize);
}
componentWillUnmount() {
window.removeEventListener('resize', this.handleWindowResize);
}
handleWindowResize = () => console.log('handleWindowResize');
}
The test looks like this:
it(`should do some stuff on window resize event`, () => {
const wrapper = mount(<MyComponent />);
const spy = jest.spyOn(wrapper.instance(), 'handleWindowResize');
wrapper.update();
global.dispatchEvent(new Event('resize'));
expect(spy).toHaveBeenCalled();
});
In my test log I get following FAIL:
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
<CircleGraph /> › should do some stuff on window resize event
expect(jest.fn()).toHaveBeenCalled()
Expected mock function to have been called, but it was not called.
171 | global.dispatchEvent(new Event('resize'));
172 |
> 173 | expect(spy).toHaveBeenCalled();
| ^
174 | });
175 | });
176 |
So the original function is invoked during the test (function works without a flaw on the original component), but not on the spy. What am I doing wrong?
Using react 16.6.0, jest-cli 23.6.0, enzyme 3.7.0
[UPDATE]
I've added tested method to the prototype with this.handleWindowResize.bind(this)
in the constructor and wrote my test like that:
it(`should do some stuff on window resize event`, () => {
const spy = jest.spyOn(CircleGraph.prototype, 'handleWindowResize');
const wrapper = shallow(<MyComponent />);
global.dispatchEvent(new Event('resize'));
wrapper.unmount();
expect(spy).toHaveBeenCalled();
});
and the spy finally called. I'm not exactly sure why though...
javascript reactjs window jestjs enzyme
add a comment |
I'm writing a component that applies some logic on 'resize' event. It basically looks like this:
class MyComponent extends React.Component {
componentDidMount() {
window.addEventListener('resize', this.handleWindowResize);
}
componentWillUnmount() {
window.removeEventListener('resize', this.handleWindowResize);
}
handleWindowResize = () => console.log('handleWindowResize');
}
The test looks like this:
it(`should do some stuff on window resize event`, () => {
const wrapper = mount(<MyComponent />);
const spy = jest.spyOn(wrapper.instance(), 'handleWindowResize');
wrapper.update();
global.dispatchEvent(new Event('resize'));
expect(spy).toHaveBeenCalled();
});
In my test log I get following FAIL:
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
<CircleGraph /> › should do some stuff on window resize event
expect(jest.fn()).toHaveBeenCalled()
Expected mock function to have been called, but it was not called.
171 | global.dispatchEvent(new Event('resize'));
172 |
> 173 | expect(spy).toHaveBeenCalled();
| ^
174 | });
175 | });
176 |
So the original function is invoked during the test (function works without a flaw on the original component), but not on the spy. What am I doing wrong?
Using react 16.6.0, jest-cli 23.6.0, enzyme 3.7.0
[UPDATE]
I've added tested method to the prototype with this.handleWindowResize.bind(this)
in the constructor and wrote my test like that:
it(`should do some stuff on window resize event`, () => {
const spy = jest.spyOn(CircleGraph.prototype, 'handleWindowResize');
const wrapper = shallow(<MyComponent />);
global.dispatchEvent(new Event('resize'));
wrapper.unmount();
expect(spy).toHaveBeenCalled();
});
and the spy finally called. I'm not exactly sure why though...
javascript reactjs window jestjs enzyme
how about dispatching event on element itslef?wrapper.first().getDOMNode().dispatchEvent(new Event('resize'))
? taken from stackoverflow.com/questions/45376974/…
– skyboyer
Nov 23 '18 at 15:24
thanks @skyboyer , unfortunately that approach didn't do it for me.
– D-80
Nov 24 '18 at 0:14
add a comment |
I'm writing a component that applies some logic on 'resize' event. It basically looks like this:
class MyComponent extends React.Component {
componentDidMount() {
window.addEventListener('resize', this.handleWindowResize);
}
componentWillUnmount() {
window.removeEventListener('resize', this.handleWindowResize);
}
handleWindowResize = () => console.log('handleWindowResize');
}
The test looks like this:
it(`should do some stuff on window resize event`, () => {
const wrapper = mount(<MyComponent />);
const spy = jest.spyOn(wrapper.instance(), 'handleWindowResize');
wrapper.update();
global.dispatchEvent(new Event('resize'));
expect(spy).toHaveBeenCalled();
});
In my test log I get following FAIL:
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
<CircleGraph /> › should do some stuff on window resize event
expect(jest.fn()).toHaveBeenCalled()
Expected mock function to have been called, but it was not called.
171 | global.dispatchEvent(new Event('resize'));
172 |
> 173 | expect(spy).toHaveBeenCalled();
| ^
174 | });
175 | });
176 |
So the original function is invoked during the test (function works without a flaw on the original component), but not on the spy. What am I doing wrong?
Using react 16.6.0, jest-cli 23.6.0, enzyme 3.7.0
[UPDATE]
I've added tested method to the prototype with this.handleWindowResize.bind(this)
in the constructor and wrote my test like that:
it(`should do some stuff on window resize event`, () => {
const spy = jest.spyOn(CircleGraph.prototype, 'handleWindowResize');
const wrapper = shallow(<MyComponent />);
global.dispatchEvent(new Event('resize'));
wrapper.unmount();
expect(spy).toHaveBeenCalled();
});
and the spy finally called. I'm not exactly sure why though...
javascript reactjs window jestjs enzyme
I'm writing a component that applies some logic on 'resize' event. It basically looks like this:
class MyComponent extends React.Component {
componentDidMount() {
window.addEventListener('resize', this.handleWindowResize);
}
componentWillUnmount() {
window.removeEventListener('resize', this.handleWindowResize);
}
handleWindowResize = () => console.log('handleWindowResize');
}
The test looks like this:
it(`should do some stuff on window resize event`, () => {
const wrapper = mount(<MyComponent />);
const spy = jest.spyOn(wrapper.instance(), 'handleWindowResize');
wrapper.update();
global.dispatchEvent(new Event('resize'));
expect(spy).toHaveBeenCalled();
});
In my test log I get following FAIL:
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
console.log app/components/MyComponent/index.js:32
handleWindowResize
<CircleGraph /> › should do some stuff on window resize event
expect(jest.fn()).toHaveBeenCalled()
Expected mock function to have been called, but it was not called.
171 | global.dispatchEvent(new Event('resize'));
172 |
> 173 | expect(spy).toHaveBeenCalled();
| ^
174 | });
175 | });
176 |
So the original function is invoked during the test (function works without a flaw on the original component), but not on the spy. What am I doing wrong?
Using react 16.6.0, jest-cli 23.6.0, enzyme 3.7.0
[UPDATE]
I've added tested method to the prototype with this.handleWindowResize.bind(this)
in the constructor and wrote my test like that:
it(`should do some stuff on window resize event`, () => {
const spy = jest.spyOn(CircleGraph.prototype, 'handleWindowResize');
const wrapper = shallow(<MyComponent />);
global.dispatchEvent(new Event('resize'));
wrapper.unmount();
expect(spy).toHaveBeenCalled();
});
and the spy finally called. I'm not exactly sure why though...
javascript reactjs window jestjs enzyme
javascript reactjs window jestjs enzyme
edited Nov 24 '18 at 10:05
D-80
asked Nov 23 '18 at 12:05


D-80D-80
11
11
how about dispatching event on element itslef?wrapper.first().getDOMNode().dispatchEvent(new Event('resize'))
? taken from stackoverflow.com/questions/45376974/…
– skyboyer
Nov 23 '18 at 15:24
thanks @skyboyer , unfortunately that approach didn't do it for me.
– D-80
Nov 24 '18 at 0:14
add a comment |
how about dispatching event on element itslef?wrapper.first().getDOMNode().dispatchEvent(new Event('resize'))
? taken from stackoverflow.com/questions/45376974/…
– skyboyer
Nov 23 '18 at 15:24
thanks @skyboyer , unfortunately that approach didn't do it for me.
– D-80
Nov 24 '18 at 0:14
how about dispatching event on element itslef?
wrapper.first().getDOMNode().dispatchEvent(new Event('resize'))
? taken from stackoverflow.com/questions/45376974/…– skyboyer
Nov 23 '18 at 15:24
how about dispatching event on element itslef?
wrapper.first().getDOMNode().dispatchEvent(new Event('resize'))
? taken from stackoverflow.com/questions/45376974/…– skyboyer
Nov 23 '18 at 15:24
thanks @skyboyer , unfortunately that approach didn't do it for me.
– D-80
Nov 24 '18 at 0:14
thanks @skyboyer , unfortunately that approach didn't do it for me.
– D-80
Nov 24 '18 at 0:14
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446427%2ftesting-window-resize-handler-on-react-component-with-jest-enzyme%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446427%2ftesting-window-resize-handler-on-react-component-with-jest-enzyme%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5 AB,tp0,3 zHl iiOAmZyark7dgXwGosMFVxGX1YEHAN zVV,0E,FjKTsD
how about dispatching event on element itslef?
wrapper.first().getDOMNode().dispatchEvent(new Event('resize'))
? taken from stackoverflow.com/questions/45376974/…– skyboyer
Nov 23 '18 at 15:24
thanks @skyboyer , unfortunately that approach didn't do it for me.
– D-80
Nov 24 '18 at 0:14