returned value from AJAX - Bug or Feature?
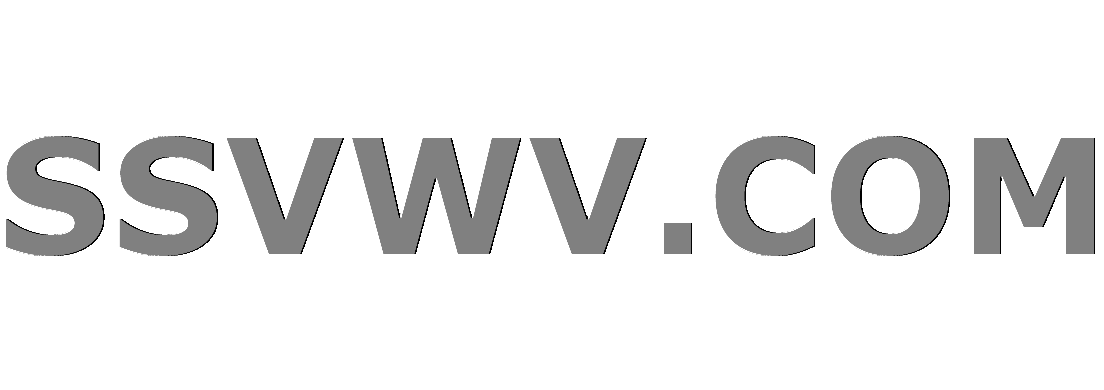
Multi tool use
up vote
0
down vote
favorite
For a long time, I wondered whether one could get data out of the AJAX callback, but the internet said no.
Now, a colleague of mine seems to have found a way to do so, but I wonder whether this is a bug or a feature?
I wrote a little ES7 testcode working inside our tool to confirm it, it looks like this:
async function testWrapper(){
var test = await testPromiseResolve()
console.log("async function was resolved to", test)
}
function testPromiseResolve(){
return $.post('../include/Stornieren/retrieveUserID.php', {
//nothing to transmit
}).then((data) => {
data = JSON.parse(data)
console.log("data from testajax is", data)
return data
})
both console logs return the data correctly (here, just a user ID fetched from the database). So the data must have been extracted from inside the promise returned by the jqueryAJAX $.post.
I want to know whether this behavior is a bug or a feature because if its a bug, I can't really use it inside our code since the day may come where this bug is fixed and then the whole thing comes crashing down on me ^^
Also, I couldn't really test whether this code keeps the functionality of async/await. When using return inside promises (I think I should use resolve/reject instead but I must admit that I haven't fully understood how these work inside jqueryAJAX) I might knock out the promise functionality and therefore, when the code really NEEDS to wait for the completion of an AJAX before proceeding, this might pose a risk for the future reliability of the code when database interactions might take longer etc..
ajax async-await ecmascript-7
|
show 2 more comments
up vote
0
down vote
favorite
For a long time, I wondered whether one could get data out of the AJAX callback, but the internet said no.
Now, a colleague of mine seems to have found a way to do so, but I wonder whether this is a bug or a feature?
I wrote a little ES7 testcode working inside our tool to confirm it, it looks like this:
async function testWrapper(){
var test = await testPromiseResolve()
console.log("async function was resolved to", test)
}
function testPromiseResolve(){
return $.post('../include/Stornieren/retrieveUserID.php', {
//nothing to transmit
}).then((data) => {
data = JSON.parse(data)
console.log("data from testajax is", data)
return data
})
both console logs return the data correctly (here, just a user ID fetched from the database). So the data must have been extracted from inside the promise returned by the jqueryAJAX $.post.
I want to know whether this behavior is a bug or a feature because if its a bug, I can't really use it inside our code since the day may come where this bug is fixed and then the whole thing comes crashing down on me ^^
Also, I couldn't really test whether this code keeps the functionality of async/await. When using return inside promises (I think I should use resolve/reject instead but I must admit that I haven't fully understood how these work inside jqueryAJAX) I might knock out the promise functionality and therefore, when the code really NEEDS to wait for the completion of an AJAX before proceeding, this might pose a risk for the future reliability of the code when database interactions might take longer etc..
ajax async-await ecmascript-7
What do you mean by "data must have been extracted from inside the promise"? Yes, that's exactly whatawait
is supposed to do; it works just like.then()
.
– Bergi
Nov 21 at 10:38
I read in numerous answers on stackoverflow that returning a value from a promise shall be impossible. Basically "what happens in callback, stays in callback" (although we dont call this callback anymore^^). Await expects a promise and once its been delivered, code execution moves on. I even had posted questions here where I was told again and again that a value cant be returned from "inside the promise". Sry if its hard to follow what I mean, but I also find it hard to explain it^^
– JSONBUG123
Nov 21 at 11:06
The whole code that runs after anawait
expression is conceptually in a.then()
callback. That's why anasync function
call must return a promise, it can't return the result immediately either.
– Bergi
Nov 21 at 14:35
ok, but DOES it return the result at all? I mean it does here, somehow, but I wonder whether it actually works like this: "ES7 synchronization functionality + returning the result from the then callback" or if the syntax above just breaks the whole async/await and promise functionality?
– JSONBUG123
Nov 21 at 14:50
No, the call totestWrapper()
in your example does not return the result. It just creates a promise, and will log thetestPromiseResolve()
result later. I does not stop execution, it does not break anything. What you see there is exactly theasync
/await
functionality: it can stop (and defer) the execution inside theasync function
body only.
– Bergi
Nov 21 at 14:53
|
show 2 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
For a long time, I wondered whether one could get data out of the AJAX callback, but the internet said no.
Now, a colleague of mine seems to have found a way to do so, but I wonder whether this is a bug or a feature?
I wrote a little ES7 testcode working inside our tool to confirm it, it looks like this:
async function testWrapper(){
var test = await testPromiseResolve()
console.log("async function was resolved to", test)
}
function testPromiseResolve(){
return $.post('../include/Stornieren/retrieveUserID.php', {
//nothing to transmit
}).then((data) => {
data = JSON.parse(data)
console.log("data from testajax is", data)
return data
})
both console logs return the data correctly (here, just a user ID fetched from the database). So the data must have been extracted from inside the promise returned by the jqueryAJAX $.post.
I want to know whether this behavior is a bug or a feature because if its a bug, I can't really use it inside our code since the day may come where this bug is fixed and then the whole thing comes crashing down on me ^^
Also, I couldn't really test whether this code keeps the functionality of async/await. When using return inside promises (I think I should use resolve/reject instead but I must admit that I haven't fully understood how these work inside jqueryAJAX) I might knock out the promise functionality and therefore, when the code really NEEDS to wait for the completion of an AJAX before proceeding, this might pose a risk for the future reliability of the code when database interactions might take longer etc..
ajax async-await ecmascript-7
For a long time, I wondered whether one could get data out of the AJAX callback, but the internet said no.
Now, a colleague of mine seems to have found a way to do so, but I wonder whether this is a bug or a feature?
I wrote a little ES7 testcode working inside our tool to confirm it, it looks like this:
async function testWrapper(){
var test = await testPromiseResolve()
console.log("async function was resolved to", test)
}
function testPromiseResolve(){
return $.post('../include/Stornieren/retrieveUserID.php', {
//nothing to transmit
}).then((data) => {
data = JSON.parse(data)
console.log("data from testajax is", data)
return data
})
both console logs return the data correctly (here, just a user ID fetched from the database). So the data must have been extracted from inside the promise returned by the jqueryAJAX $.post.
I want to know whether this behavior is a bug or a feature because if its a bug, I can't really use it inside our code since the day may come where this bug is fixed and then the whole thing comes crashing down on me ^^
Also, I couldn't really test whether this code keeps the functionality of async/await. When using return inside promises (I think I should use resolve/reject instead but I must admit that I haven't fully understood how these work inside jqueryAJAX) I might knock out the promise functionality and therefore, when the code really NEEDS to wait for the completion of an AJAX before proceeding, this might pose a risk for the future reliability of the code when database interactions might take longer etc..
async function testWrapper(){
var test = await testPromiseResolve()
console.log("async function was resolved to", test)
}
function testPromiseResolve(){
return $.post('../include/Stornieren/retrieveUserID.php', {
//nothing to transmit
}).then((data) => {
data = JSON.parse(data)
console.log("data from testajax is", data)
return data
})
async function testWrapper(){
var test = await testPromiseResolve()
console.log("async function was resolved to", test)
}
function testPromiseResolve(){
return $.post('../include/Stornieren/retrieveUserID.php', {
//nothing to transmit
}).then((data) => {
data = JSON.parse(data)
console.log("data from testajax is", data)
return data
})
ajax async-await ecmascript-7
ajax async-await ecmascript-7
asked Nov 21 at 8:27
JSONBUG123
487
487
What do you mean by "data must have been extracted from inside the promise"? Yes, that's exactly whatawait
is supposed to do; it works just like.then()
.
– Bergi
Nov 21 at 10:38
I read in numerous answers on stackoverflow that returning a value from a promise shall be impossible. Basically "what happens in callback, stays in callback" (although we dont call this callback anymore^^). Await expects a promise and once its been delivered, code execution moves on. I even had posted questions here where I was told again and again that a value cant be returned from "inside the promise". Sry if its hard to follow what I mean, but I also find it hard to explain it^^
– JSONBUG123
Nov 21 at 11:06
The whole code that runs after anawait
expression is conceptually in a.then()
callback. That's why anasync function
call must return a promise, it can't return the result immediately either.
– Bergi
Nov 21 at 14:35
ok, but DOES it return the result at all? I mean it does here, somehow, but I wonder whether it actually works like this: "ES7 synchronization functionality + returning the result from the then callback" or if the syntax above just breaks the whole async/await and promise functionality?
– JSONBUG123
Nov 21 at 14:50
No, the call totestWrapper()
in your example does not return the result. It just creates a promise, and will log thetestPromiseResolve()
result later. I does not stop execution, it does not break anything. What you see there is exactly theasync
/await
functionality: it can stop (and defer) the execution inside theasync function
body only.
– Bergi
Nov 21 at 14:53
|
show 2 more comments
What do you mean by "data must have been extracted from inside the promise"? Yes, that's exactly whatawait
is supposed to do; it works just like.then()
.
– Bergi
Nov 21 at 10:38
I read in numerous answers on stackoverflow that returning a value from a promise shall be impossible. Basically "what happens in callback, stays in callback" (although we dont call this callback anymore^^). Await expects a promise and once its been delivered, code execution moves on. I even had posted questions here where I was told again and again that a value cant be returned from "inside the promise". Sry if its hard to follow what I mean, but I also find it hard to explain it^^
– JSONBUG123
Nov 21 at 11:06
The whole code that runs after anawait
expression is conceptually in a.then()
callback. That's why anasync function
call must return a promise, it can't return the result immediately either.
– Bergi
Nov 21 at 14:35
ok, but DOES it return the result at all? I mean it does here, somehow, but I wonder whether it actually works like this: "ES7 synchronization functionality + returning the result from the then callback" or if the syntax above just breaks the whole async/await and promise functionality?
– JSONBUG123
Nov 21 at 14:50
No, the call totestWrapper()
in your example does not return the result. It just creates a promise, and will log thetestPromiseResolve()
result later. I does not stop execution, it does not break anything. What you see there is exactly theasync
/await
functionality: it can stop (and defer) the execution inside theasync function
body only.
– Bergi
Nov 21 at 14:53
What do you mean by "data must have been extracted from inside the promise"? Yes, that's exactly what
await
is supposed to do; it works just like .then()
.– Bergi
Nov 21 at 10:38
What do you mean by "data must have been extracted from inside the promise"? Yes, that's exactly what
await
is supposed to do; it works just like .then()
.– Bergi
Nov 21 at 10:38
I read in numerous answers on stackoverflow that returning a value from a promise shall be impossible. Basically "what happens in callback, stays in callback" (although we dont call this callback anymore^^). Await expects a promise and once its been delivered, code execution moves on. I even had posted questions here where I was told again and again that a value cant be returned from "inside the promise". Sry if its hard to follow what I mean, but I also find it hard to explain it^^
– JSONBUG123
Nov 21 at 11:06
I read in numerous answers on stackoverflow that returning a value from a promise shall be impossible. Basically "what happens in callback, stays in callback" (although we dont call this callback anymore^^). Await expects a promise and once its been delivered, code execution moves on. I even had posted questions here where I was told again and again that a value cant be returned from "inside the promise". Sry if its hard to follow what I mean, but I also find it hard to explain it^^
– JSONBUG123
Nov 21 at 11:06
The whole code that runs after an
await
expression is conceptually in a .then()
callback. That's why an async function
call must return a promise, it can't return the result immediately either.– Bergi
Nov 21 at 14:35
The whole code that runs after an
await
expression is conceptually in a .then()
callback. That's why an async function
call must return a promise, it can't return the result immediately either.– Bergi
Nov 21 at 14:35
ok, but DOES it return the result at all? I mean it does here, somehow, but I wonder whether it actually works like this: "ES7 synchronization functionality + returning the result from the then callback" or if the syntax above just breaks the whole async/await and promise functionality?
– JSONBUG123
Nov 21 at 14:50
ok, but DOES it return the result at all? I mean it does here, somehow, but I wonder whether it actually works like this: "ES7 synchronization functionality + returning the result from the then callback" or if the syntax above just breaks the whole async/await and promise functionality?
– JSONBUG123
Nov 21 at 14:50
No, the call to
testWrapper()
in your example does not return the result. It just creates a promise, and will log the testPromiseResolve()
result later. I does not stop execution, it does not break anything. What you see there is exactly the async
/await
functionality: it can stop (and defer) the execution inside the async function
body only.– Bergi
Nov 21 at 14:53
No, the call to
testWrapper()
in your example does not return the result. It just creates a promise, and will log the testPromiseResolve()
result later. I does not stop execution, it does not break anything. What you see there is exactly the async
/await
functionality: it can stop (and defer) the execution inside the async function
body only.– Bergi
Nov 21 at 14:53
|
show 2 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53407897%2freturned-value-from-ajax-bug-or-feature%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CF4Z79aAlMnEGxkR cK,Y9lY9,gYXv R,qo,WSY 1aVGiLyAK4,W2haZ gjbv3 6ACwwuYEic H
What do you mean by "data must have been extracted from inside the promise"? Yes, that's exactly what
await
is supposed to do; it works just like.then()
.– Bergi
Nov 21 at 10:38
I read in numerous answers on stackoverflow that returning a value from a promise shall be impossible. Basically "what happens in callback, stays in callback" (although we dont call this callback anymore^^). Await expects a promise and once its been delivered, code execution moves on. I even had posted questions here where I was told again and again that a value cant be returned from "inside the promise". Sry if its hard to follow what I mean, but I also find it hard to explain it^^
– JSONBUG123
Nov 21 at 11:06
The whole code that runs after an
await
expression is conceptually in a.then()
callback. That's why anasync function
call must return a promise, it can't return the result immediately either.– Bergi
Nov 21 at 14:35
ok, but DOES it return the result at all? I mean it does here, somehow, but I wonder whether it actually works like this: "ES7 synchronization functionality + returning the result from the then callback" or if the syntax above just breaks the whole async/await and promise functionality?
– JSONBUG123
Nov 21 at 14:50
No, the call to
testWrapper()
in your example does not return the result. It just creates a promise, and will log thetestPromiseResolve()
result later. I does not stop execution, it does not break anything. What you see there is exactly theasync
/await
functionality: it can stop (and defer) the execution inside theasync function
body only.– Bergi
Nov 21 at 14:53