problem with images loading on top of already set images swift 4
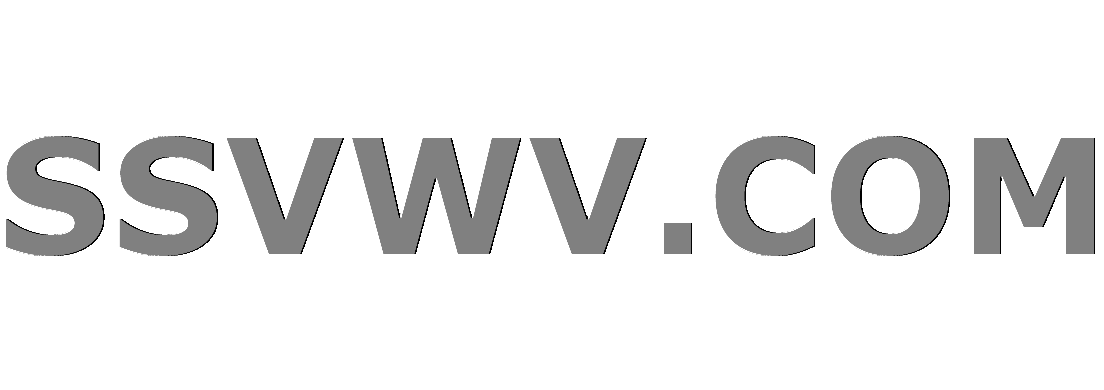
Multi tool use
I'm having an issue in my cellForItemAtIndexPath where I am setting an image to my cell's UIButton but every time I scroll the collectionView's cells, it's placing the image on top of the already set image again and again. I can tell because the shadow of the image is getting thicker and thicker. I'm pulling the images from an array that I created of image literals in that swift file and the correct images are loading so there's no problem there. I'm sure this is a simple fix for most but I can't seem to find an answer anywhere.
Image of my cellForItemAtIndexPath function
My app running before I scroll
App after scrolling a bit
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let collectionImage: UIButton = {
let image = UIButton()
image.setImage(collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal), for: .normal)
image.contentMode = .scaleAspectFit
image.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
return image
}()
collectionImage.imageView?.image = collectionImageArray[imageNumber]
cell.addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: cell.centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: cell.centerYAnchor).isActive = true
print(imageNumber)
return cell
}
uicollectionview swift4 uicollectionviewcell xcode9
add a comment |
I'm having an issue in my cellForItemAtIndexPath where I am setting an image to my cell's UIButton but every time I scroll the collectionView's cells, it's placing the image on top of the already set image again and again. I can tell because the shadow of the image is getting thicker and thicker. I'm pulling the images from an array that I created of image literals in that swift file and the correct images are loading so there's no problem there. I'm sure this is a simple fix for most but I can't seem to find an answer anywhere.
Image of my cellForItemAtIndexPath function
My app running before I scroll
App after scrolling a bit
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let collectionImage: UIButton = {
let image = UIButton()
image.setImage(collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal), for: .normal)
image.contentMode = .scaleAspectFit
image.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
return image
}()
collectionImage.imageView?.image = collectionImageArray[imageNumber]
cell.addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: cell.centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: cell.centerYAnchor).isActive = true
print(imageNumber)
return cell
}
uicollectionview swift4 uicollectionviewcell xcode9
That's the expected behavior: Cells are reused (...dequeueReusableCell...). Check if the subview already exists or design the button in Interface Builder.
– vadian
Nov 23 '18 at 22:07
@vadian I'm only adding the subview once in the cell for index path method. It wouldn't be continuously adding that subview would it? as for interface builder, I'd prefer to do it all in code.
– CodeHurts
Nov 24 '18 at 0:23
Once again, cells are reused. There is a cell pool which contains as many cells (plus 1 or 2) as fit in the table view. So if a cell goes off-screen the cell is put into the pool in the state it left the screen. The next time a new cell is needed the framework takes one from the cell pool. This cell already have the custom button.
– vadian
Nov 24 '18 at 7:29
add a comment |
I'm having an issue in my cellForItemAtIndexPath where I am setting an image to my cell's UIButton but every time I scroll the collectionView's cells, it's placing the image on top of the already set image again and again. I can tell because the shadow of the image is getting thicker and thicker. I'm pulling the images from an array that I created of image literals in that swift file and the correct images are loading so there's no problem there. I'm sure this is a simple fix for most but I can't seem to find an answer anywhere.
Image of my cellForItemAtIndexPath function
My app running before I scroll
App after scrolling a bit
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let collectionImage: UIButton = {
let image = UIButton()
image.setImage(collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal), for: .normal)
image.contentMode = .scaleAspectFit
image.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
return image
}()
collectionImage.imageView?.image = collectionImageArray[imageNumber]
cell.addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: cell.centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: cell.centerYAnchor).isActive = true
print(imageNumber)
return cell
}
uicollectionview swift4 uicollectionviewcell xcode9
I'm having an issue in my cellForItemAtIndexPath where I am setting an image to my cell's UIButton but every time I scroll the collectionView's cells, it's placing the image on top of the already set image again and again. I can tell because the shadow of the image is getting thicker and thicker. I'm pulling the images from an array that I created of image literals in that swift file and the correct images are loading so there's no problem there. I'm sure this is a simple fix for most but I can't seem to find an answer anywhere.
Image of my cellForItemAtIndexPath function
My app running before I scroll
App after scrolling a bit
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let collectionImage: UIButton = {
let image = UIButton()
image.setImage(collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal), for: .normal)
image.contentMode = .scaleAspectFit
image.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
return image
}()
collectionImage.imageView?.image = collectionImageArray[imageNumber]
cell.addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: cell.centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: cell.centerYAnchor).isActive = true
print(imageNumber)
return cell
}
uicollectionview swift4 uicollectionviewcell xcode9
uicollectionview swift4 uicollectionviewcell xcode9
edited Nov 24 '18 at 0:34
CodeHurts
asked Nov 23 '18 at 21:59


CodeHurtsCodeHurts
13
13
That's the expected behavior: Cells are reused (...dequeueReusableCell...). Check if the subview already exists or design the button in Interface Builder.
– vadian
Nov 23 '18 at 22:07
@vadian I'm only adding the subview once in the cell for index path method. It wouldn't be continuously adding that subview would it? as for interface builder, I'd prefer to do it all in code.
– CodeHurts
Nov 24 '18 at 0:23
Once again, cells are reused. There is a cell pool which contains as many cells (plus 1 or 2) as fit in the table view. So if a cell goes off-screen the cell is put into the pool in the state it left the screen. The next time a new cell is needed the framework takes one from the cell pool. This cell already have the custom button.
– vadian
Nov 24 '18 at 7:29
add a comment |
That's the expected behavior: Cells are reused (...dequeueReusableCell...). Check if the subview already exists or design the button in Interface Builder.
– vadian
Nov 23 '18 at 22:07
@vadian I'm only adding the subview once in the cell for index path method. It wouldn't be continuously adding that subview would it? as for interface builder, I'd prefer to do it all in code.
– CodeHurts
Nov 24 '18 at 0:23
Once again, cells are reused. There is a cell pool which contains as many cells (plus 1 or 2) as fit in the table view. So if a cell goes off-screen the cell is put into the pool in the state it left the screen. The next time a new cell is needed the framework takes one from the cell pool. This cell already have the custom button.
– vadian
Nov 24 '18 at 7:29
That's the expected behavior: Cells are reused (...dequeueReusableCell...). Check if the subview already exists or design the button in Interface Builder.
– vadian
Nov 23 '18 at 22:07
That's the expected behavior: Cells are reused (...dequeueReusableCell...). Check if the subview already exists or design the button in Interface Builder.
– vadian
Nov 23 '18 at 22:07
@vadian I'm only adding the subview once in the cell for index path method. It wouldn't be continuously adding that subview would it? as for interface builder, I'd prefer to do it all in code.
– CodeHurts
Nov 24 '18 at 0:23
@vadian I'm only adding the subview once in the cell for index path method. It wouldn't be continuously adding that subview would it? as for interface builder, I'd prefer to do it all in code.
– CodeHurts
Nov 24 '18 at 0:23
Once again, cells are reused. There is a cell pool which contains as many cells (plus 1 or 2) as fit in the table view. So if a cell goes off-screen the cell is put into the pool in the state it left the screen. The next time a new cell is needed the framework takes one from the cell pool. This cell already have the custom button.
– vadian
Nov 24 '18 at 7:29
Once again, cells are reused. There is a cell pool which contains as many cells (plus 1 or 2) as fit in the table view. So if a cell goes off-screen the cell is put into the pool in the state it left the screen. The next time a new cell is needed the framework takes one from the cell pool. This cell already have the custom button.
– vadian
Nov 24 '18 at 7:29
add a comment |
1 Answer
1
active
oldest
votes
If anyone else comes across this issue (or maybe I'm the only dumb one), the problem was that I should have created my UIButton, added the subview, and constrained it inside of the cell class and from the cellForItem AtindexPath method set the image and target handler like this.
class HomeViewCell: UICollectionViewCell {
let collectionImage: UIButton = {
let image = UIButton(type: .custom)
image.contentMode = .scaleAspectFit
return image
}()
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: centerYAnchor).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let image = collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal)
cell.collectionImage.setImage(image, for: .normal)
cell.collectionImage.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
print(imageNumber)
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: view.frame.width, height: view.frame.height)
}
@objc func handleCollectionTap() {
let layout = UICollectionViewFlowLayout()
let cardViewer = CardViewerController(collectionViewLayout: layout)
present(cardViewer, animated: true, completion: nil)
}
Everything is running smoothly now! :)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453336%2fproblem-with-images-loading-on-top-of-already-set-images-swift-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If anyone else comes across this issue (or maybe I'm the only dumb one), the problem was that I should have created my UIButton, added the subview, and constrained it inside of the cell class and from the cellForItem AtindexPath method set the image and target handler like this.
class HomeViewCell: UICollectionViewCell {
let collectionImage: UIButton = {
let image = UIButton(type: .custom)
image.contentMode = .scaleAspectFit
return image
}()
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: centerYAnchor).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let image = collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal)
cell.collectionImage.setImage(image, for: .normal)
cell.collectionImage.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
print(imageNumber)
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: view.frame.width, height: view.frame.height)
}
@objc func handleCollectionTap() {
let layout = UICollectionViewFlowLayout()
let cardViewer = CardViewerController(collectionViewLayout: layout)
present(cardViewer, animated: true, completion: nil)
}
Everything is running smoothly now! :)
add a comment |
If anyone else comes across this issue (or maybe I'm the only dumb one), the problem was that I should have created my UIButton, added the subview, and constrained it inside of the cell class and from the cellForItem AtindexPath method set the image and target handler like this.
class HomeViewCell: UICollectionViewCell {
let collectionImage: UIButton = {
let image = UIButton(type: .custom)
image.contentMode = .scaleAspectFit
return image
}()
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: centerYAnchor).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let image = collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal)
cell.collectionImage.setImage(image, for: .normal)
cell.collectionImage.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
print(imageNumber)
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: view.frame.width, height: view.frame.height)
}
@objc func handleCollectionTap() {
let layout = UICollectionViewFlowLayout()
let cardViewer = CardViewerController(collectionViewLayout: layout)
present(cardViewer, animated: true, completion: nil)
}
Everything is running smoothly now! :)
add a comment |
If anyone else comes across this issue (or maybe I'm the only dumb one), the problem was that I should have created my UIButton, added the subview, and constrained it inside of the cell class and from the cellForItem AtindexPath method set the image and target handler like this.
class HomeViewCell: UICollectionViewCell {
let collectionImage: UIButton = {
let image = UIButton(type: .custom)
image.contentMode = .scaleAspectFit
return image
}()
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: centerYAnchor).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let image = collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal)
cell.collectionImage.setImage(image, for: .normal)
cell.collectionImage.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
print(imageNumber)
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: view.frame.width, height: view.frame.height)
}
@objc func handleCollectionTap() {
let layout = UICollectionViewFlowLayout()
let cardViewer = CardViewerController(collectionViewLayout: layout)
present(cardViewer, animated: true, completion: nil)
}
Everything is running smoothly now! :)
If anyone else comes across this issue (or maybe I'm the only dumb one), the problem was that I should have created my UIButton, added the subview, and constrained it inside of the cell class and from the cellForItem AtindexPath method set the image and target handler like this.
class HomeViewCell: UICollectionViewCell {
let collectionImage: UIButton = {
let image = UIButton(type: .custom)
image.contentMode = .scaleAspectFit
return image
}()
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(collectionImage)
collectionImage.anchor(top: nil, left: nil, bottom: nil, right: nil, paddingTop: 0, paddingLeft: 0, paddingBottom: 0, paddingRight: 0, width: 0, height: 0)
collectionImage.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
collectionImage.centerYAnchor.constraint(equalTo: centerYAnchor).isActive = true
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cellId", for: indexPath) as! HomeViewCell
collectionView.bounces = false
let imageNumber = indexPath.item
let image = collectionImageArray[imageNumber].withRenderingMode(.alwaysOriginal)
cell.collectionImage.setImage(image, for: .normal)
cell.collectionImage.addTarget(self, action: #selector(handleCollectionTap), for: .touchUpInside)
print(imageNumber)
return cell
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: view.frame.width, height: view.frame.height)
}
@objc func handleCollectionTap() {
let layout = UICollectionViewFlowLayout()
let cardViewer = CardViewerController(collectionViewLayout: layout)
present(cardViewer, animated: true, completion: nil)
}
Everything is running smoothly now! :)
answered Nov 24 '18 at 1:47


CodeHurtsCodeHurts
13
13
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453336%2fproblem-with-images-loading-on-top-of-already-set-images-swift-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GT4bcws8fj wkvC 1 M cyz94wKTTLlZS4tbWZUc1QVo n BxfIZ,D FZ3,0dMxLqxoavsGuw 9,a aNwlUCH tDypIDUNnSV x H3p5
That's the expected behavior: Cells are reused (...dequeueReusableCell...). Check if the subview already exists or design the button in Interface Builder.
– vadian
Nov 23 '18 at 22:07
@vadian I'm only adding the subview once in the cell for index path method. It wouldn't be continuously adding that subview would it? as for interface builder, I'd prefer to do it all in code.
– CodeHurts
Nov 24 '18 at 0:23
Once again, cells are reused. There is a cell pool which contains as many cells (plus 1 or 2) as fit in the table view. So if a cell goes off-screen the cell is put into the pool in the state it left the screen. The next time a new cell is needed the framework takes one from the cell pool. This cell already have the custom button.
– vadian
Nov 24 '18 at 7:29