How to override the query in has_many relationship
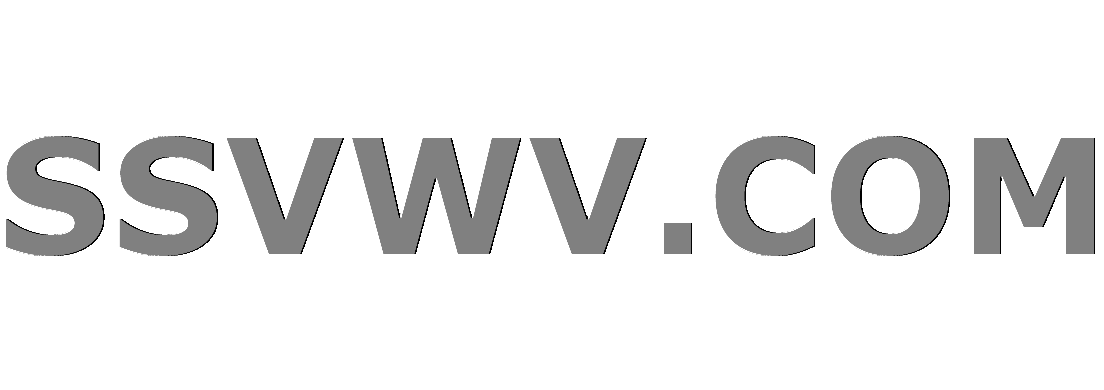
Multi tool use
I need to override the defailt ActiveRecord generated SQL query in a has_many relationship. While finder_sql is deprecated in Rails 4.
In a given legacy application there are multiple relationships between customers and their available discounts. It looks like this:
A Rails 4 scope on discounts to fetch all available discounts for a given customer:
scope :for_customer, lambda { |customer|
where("CustomerNo = '#{customer.CustomerNo}'
OR DiscountGrpCustNo = '#{customer.DiscountGrpCustNo}'
OR PriceListNo = '#{customer.PriceListNo}'")
}
So I wanted to use this scope to define the has_many on the customer.
I found an explanation for using variables on a has_many in a different question here: https://stackoverflow.com/a/2462397/1062276
So I tried:
has_many :discounts,
-> (customer) { for_customer(customer) }
Which resulted in Invalid column name 'customer_id'
due to the following:
> customer.discounts.to_sql
SELECT [KuraasAS].[DiscountAgreementCustomer].* FROM [KuraasAS].[DiscountAgreementCustomer] WHERE [KuraasAS].[DiscountAgreementCustomer].[customer_id] = 12345 AND (CustomerNo = '12345'
OR DiscountGrpCustNo = '5'
OR PriceListNo = '3')
The customer_id column is Rails's assumption. How can I take this out of the query?
ruby-on-rails

add a comment |
I need to override the defailt ActiveRecord generated SQL query in a has_many relationship. While finder_sql is deprecated in Rails 4.
In a given legacy application there are multiple relationships between customers and their available discounts. It looks like this:
A Rails 4 scope on discounts to fetch all available discounts for a given customer:
scope :for_customer, lambda { |customer|
where("CustomerNo = '#{customer.CustomerNo}'
OR DiscountGrpCustNo = '#{customer.DiscountGrpCustNo}'
OR PriceListNo = '#{customer.PriceListNo}'")
}
So I wanted to use this scope to define the has_many on the customer.
I found an explanation for using variables on a has_many in a different question here: https://stackoverflow.com/a/2462397/1062276
So I tried:
has_many :discounts,
-> (customer) { for_customer(customer) }
Which resulted in Invalid column name 'customer_id'
due to the following:
> customer.discounts.to_sql
SELECT [KuraasAS].[DiscountAgreementCustomer].* FROM [KuraasAS].[DiscountAgreementCustomer] WHERE [KuraasAS].[DiscountAgreementCustomer].[customer_id] = 12345 AND (CustomerNo = '12345'
OR DiscountGrpCustNo = '5'
OR PriceListNo = '3')
The customer_id column is Rails's assumption. How can I take this out of the query?
ruby-on-rails

add a comment |
I need to override the defailt ActiveRecord generated SQL query in a has_many relationship. While finder_sql is deprecated in Rails 4.
In a given legacy application there are multiple relationships between customers and their available discounts. It looks like this:
A Rails 4 scope on discounts to fetch all available discounts for a given customer:
scope :for_customer, lambda { |customer|
where("CustomerNo = '#{customer.CustomerNo}'
OR DiscountGrpCustNo = '#{customer.DiscountGrpCustNo}'
OR PriceListNo = '#{customer.PriceListNo}'")
}
So I wanted to use this scope to define the has_many on the customer.
I found an explanation for using variables on a has_many in a different question here: https://stackoverflow.com/a/2462397/1062276
So I tried:
has_many :discounts,
-> (customer) { for_customer(customer) }
Which resulted in Invalid column name 'customer_id'
due to the following:
> customer.discounts.to_sql
SELECT [KuraasAS].[DiscountAgreementCustomer].* FROM [KuraasAS].[DiscountAgreementCustomer] WHERE [KuraasAS].[DiscountAgreementCustomer].[customer_id] = 12345 AND (CustomerNo = '12345'
OR DiscountGrpCustNo = '5'
OR PriceListNo = '3')
The customer_id column is Rails's assumption. How can I take this out of the query?
ruby-on-rails

I need to override the defailt ActiveRecord generated SQL query in a has_many relationship. While finder_sql is deprecated in Rails 4.
In a given legacy application there are multiple relationships between customers and their available discounts. It looks like this:
A Rails 4 scope on discounts to fetch all available discounts for a given customer:
scope :for_customer, lambda { |customer|
where("CustomerNo = '#{customer.CustomerNo}'
OR DiscountGrpCustNo = '#{customer.DiscountGrpCustNo}'
OR PriceListNo = '#{customer.PriceListNo}'")
}
So I wanted to use this scope to define the has_many on the customer.
I found an explanation for using variables on a has_many in a different question here: https://stackoverflow.com/a/2462397/1062276
So I tried:
has_many :discounts,
-> (customer) { for_customer(customer) }
Which resulted in Invalid column name 'customer_id'
due to the following:
> customer.discounts.to_sql
SELECT [KuraasAS].[DiscountAgreementCustomer].* FROM [KuraasAS].[DiscountAgreementCustomer] WHERE [KuraasAS].[DiscountAgreementCustomer].[customer_id] = 12345 AND (CustomerNo = '12345'
OR DiscountGrpCustNo = '5'
OR PriceListNo = '3')
The customer_id column is Rails's assumption. How can I take this out of the query?
ruby-on-rails

ruby-on-rails

asked Nov 22 at 9:34
ringe
632311
632311
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
To override the default generated query in a Rails 4 has_many relationship I did the following:
has_many :discounts,
-> (cu) { unscope(:where).for_customer(cu) }
The method to look up and make use of is unscope
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53427787%2fhow-to-override-the-query-in-has-many-relationship%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To override the default generated query in a Rails 4 has_many relationship I did the following:
has_many :discounts,
-> (cu) { unscope(:where).for_customer(cu) }
The method to look up and make use of is unscope
add a comment |
To override the default generated query in a Rails 4 has_many relationship I did the following:
has_many :discounts,
-> (cu) { unscope(:where).for_customer(cu) }
The method to look up and make use of is unscope
add a comment |
To override the default generated query in a Rails 4 has_many relationship I did the following:
has_many :discounts,
-> (cu) { unscope(:where).for_customer(cu) }
The method to look up and make use of is unscope
To override the default generated query in a Rails 4 has_many relationship I did the following:
has_many :discounts,
-> (cu) { unscope(:where).for_customer(cu) }
The method to look up and make use of is unscope
answered Nov 22 at 9:37
ringe
632311
632311
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53427787%2fhow-to-override-the-query-in-has-many-relationship%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
inmF2ne B7iiwmbPDcc epVqkKAFGODfLWjZ g4PWcirxRZbMGCA7Mg