Enable ContainerRequestFilter without web.xml
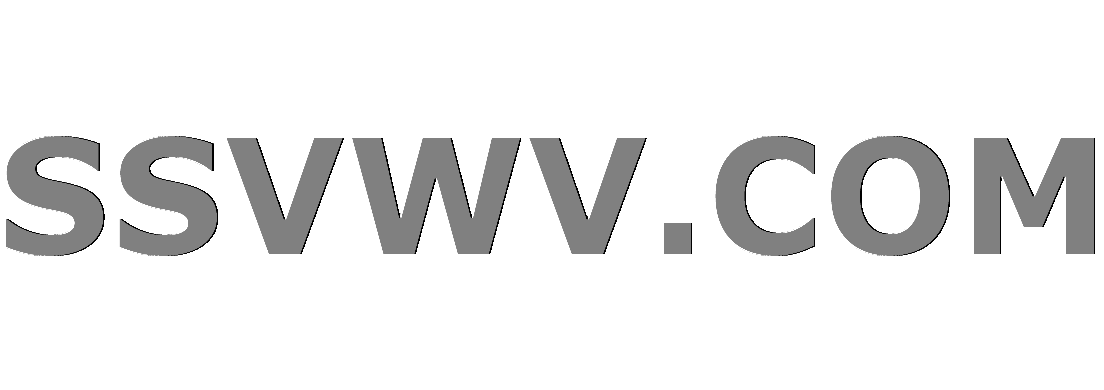
Multi tool use
up vote
0
down vote
favorite
I'm trying to enable basic authentication using Filter. I likes to enable that without using web.xml file. I tried the answer in the question
Use ContainerRequestFilter in Jersey without web.xml
But I can't get clear idea over that.
How to enable filter without web.xml file?
package com.example.filter;
import java.io.IOException;
import java.lang.reflect.Method;
import java.util.Base64;
import java.util.StringTokenizer;
import javax.annotation.security.PermitAll;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerRequestFilter;
import javax.ws.rs.container.ResourceInfo;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.Response;
import com.example.ApiService;
public class AuthFilter implements ContainerRequestFilter {
private HttpServletRequest request;
@Context
private ResourceInfo resourceInfo;
private static final String AUTHORIZATION_PROPERTY = "Authorization";
private static final String AUTHENTICATION_SCHEME = "Basic";
private static final Response ACCESS_DENIED = Response.status(Response.Status.UNAUTHORIZED)
.entity("You cannot access this resource").build();
public boolean isAuthenticated(String authCredentials) {
if (null == authCredentials)
return false;
final String encodedUserPassword = authCredentials.replaceFirst(AUTHENTICATION_SCHEME + " ", "");
String usernameAndPassword = null;
try {
byte decodedBytes = Base64.getDecoder().decode(encodedUserPassword);
usernameAndPassword = new String(decodedBytes, "UTF-8");
} catch (IOException e) {
e.printStackTrace();
}
final StringTokenizer tokenizer = new StringTokenizer(usernameAndPassword, ":");
final String username = tokenizer.nextToken();
if (request.getSession() != null) {
String mobile_number = (String) request.getSession().getAttribute(ApiService.CONTACT_ID_KEY);
if (mobile_number != username) {
return true;
}
}
return false;
}
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
Method method = resourceInfo.getResourceMethod();
if (!method.isAnnotationPresent(PermitAll.class)) {
// Fetch authorization header
final String authorization = requestContext.getHeaderString(AUTHORIZATION_PROPERTY);
// If no authorization information present; block access
if (authorization == null || authorization.isEmpty()) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
if(!isAuthenticated(authorization)) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
}
}
}
And this is my Application class
package com.example;
import java.util.HashMap;
import java.util.Map;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/rest")
public class ApiConfig extends Application {
public Map<String, Object> getProperties() {
Map<String, Object> properties = new HashMap<>();
properties.put("jersey.config.server.provider.packages", "com.example");
return properties;
}
}
Thank you.
java jersey jax-rs tomcat8 requestfiltering
add a comment |
up vote
0
down vote
favorite
I'm trying to enable basic authentication using Filter. I likes to enable that without using web.xml file. I tried the answer in the question
Use ContainerRequestFilter in Jersey without web.xml
But I can't get clear idea over that.
How to enable filter without web.xml file?
package com.example.filter;
import java.io.IOException;
import java.lang.reflect.Method;
import java.util.Base64;
import java.util.StringTokenizer;
import javax.annotation.security.PermitAll;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerRequestFilter;
import javax.ws.rs.container.ResourceInfo;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.Response;
import com.example.ApiService;
public class AuthFilter implements ContainerRequestFilter {
private HttpServletRequest request;
@Context
private ResourceInfo resourceInfo;
private static final String AUTHORIZATION_PROPERTY = "Authorization";
private static final String AUTHENTICATION_SCHEME = "Basic";
private static final Response ACCESS_DENIED = Response.status(Response.Status.UNAUTHORIZED)
.entity("You cannot access this resource").build();
public boolean isAuthenticated(String authCredentials) {
if (null == authCredentials)
return false;
final String encodedUserPassword = authCredentials.replaceFirst(AUTHENTICATION_SCHEME + " ", "");
String usernameAndPassword = null;
try {
byte decodedBytes = Base64.getDecoder().decode(encodedUserPassword);
usernameAndPassword = new String(decodedBytes, "UTF-8");
} catch (IOException e) {
e.printStackTrace();
}
final StringTokenizer tokenizer = new StringTokenizer(usernameAndPassword, ":");
final String username = tokenizer.nextToken();
if (request.getSession() != null) {
String mobile_number = (String) request.getSession().getAttribute(ApiService.CONTACT_ID_KEY);
if (mobile_number != username) {
return true;
}
}
return false;
}
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
Method method = resourceInfo.getResourceMethod();
if (!method.isAnnotationPresent(PermitAll.class)) {
// Fetch authorization header
final String authorization = requestContext.getHeaderString(AUTHORIZATION_PROPERTY);
// If no authorization information present; block access
if (authorization == null || authorization.isEmpty()) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
if(!isAuthenticated(authorization)) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
}
}
}
And this is my Application class
package com.example;
import java.util.HashMap;
import java.util.Map;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/rest")
public class ApiConfig extends Application {
public Map<String, Object> getProperties() {
Map<String, Object> properties = new HashMap<>();
properties.put("jersey.config.server.provider.packages", "com.example");
return properties;
}
}
Thank you.
java jersey jax-rs tomcat8 requestfiltering
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to enable basic authentication using Filter. I likes to enable that without using web.xml file. I tried the answer in the question
Use ContainerRequestFilter in Jersey without web.xml
But I can't get clear idea over that.
How to enable filter without web.xml file?
package com.example.filter;
import java.io.IOException;
import java.lang.reflect.Method;
import java.util.Base64;
import java.util.StringTokenizer;
import javax.annotation.security.PermitAll;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerRequestFilter;
import javax.ws.rs.container.ResourceInfo;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.Response;
import com.example.ApiService;
public class AuthFilter implements ContainerRequestFilter {
private HttpServletRequest request;
@Context
private ResourceInfo resourceInfo;
private static final String AUTHORIZATION_PROPERTY = "Authorization";
private static final String AUTHENTICATION_SCHEME = "Basic";
private static final Response ACCESS_DENIED = Response.status(Response.Status.UNAUTHORIZED)
.entity("You cannot access this resource").build();
public boolean isAuthenticated(String authCredentials) {
if (null == authCredentials)
return false;
final String encodedUserPassword = authCredentials.replaceFirst(AUTHENTICATION_SCHEME + " ", "");
String usernameAndPassword = null;
try {
byte decodedBytes = Base64.getDecoder().decode(encodedUserPassword);
usernameAndPassword = new String(decodedBytes, "UTF-8");
} catch (IOException e) {
e.printStackTrace();
}
final StringTokenizer tokenizer = new StringTokenizer(usernameAndPassword, ":");
final String username = tokenizer.nextToken();
if (request.getSession() != null) {
String mobile_number = (String) request.getSession().getAttribute(ApiService.CONTACT_ID_KEY);
if (mobile_number != username) {
return true;
}
}
return false;
}
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
Method method = resourceInfo.getResourceMethod();
if (!method.isAnnotationPresent(PermitAll.class)) {
// Fetch authorization header
final String authorization = requestContext.getHeaderString(AUTHORIZATION_PROPERTY);
// If no authorization information present; block access
if (authorization == null || authorization.isEmpty()) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
if(!isAuthenticated(authorization)) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
}
}
}
And this is my Application class
package com.example;
import java.util.HashMap;
import java.util.Map;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/rest")
public class ApiConfig extends Application {
public Map<String, Object> getProperties() {
Map<String, Object> properties = new HashMap<>();
properties.put("jersey.config.server.provider.packages", "com.example");
return properties;
}
}
Thank you.
java jersey jax-rs tomcat8 requestfiltering
I'm trying to enable basic authentication using Filter. I likes to enable that without using web.xml file. I tried the answer in the question
Use ContainerRequestFilter in Jersey without web.xml
But I can't get clear idea over that.
How to enable filter without web.xml file?
package com.example.filter;
import java.io.IOException;
import java.lang.reflect.Method;
import java.util.Base64;
import java.util.StringTokenizer;
import javax.annotation.security.PermitAll;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerRequestFilter;
import javax.ws.rs.container.ResourceInfo;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.Response;
import com.example.ApiService;
public class AuthFilter implements ContainerRequestFilter {
private HttpServletRequest request;
@Context
private ResourceInfo resourceInfo;
private static final String AUTHORIZATION_PROPERTY = "Authorization";
private static final String AUTHENTICATION_SCHEME = "Basic";
private static final Response ACCESS_DENIED = Response.status(Response.Status.UNAUTHORIZED)
.entity("You cannot access this resource").build();
public boolean isAuthenticated(String authCredentials) {
if (null == authCredentials)
return false;
final String encodedUserPassword = authCredentials.replaceFirst(AUTHENTICATION_SCHEME + " ", "");
String usernameAndPassword = null;
try {
byte decodedBytes = Base64.getDecoder().decode(encodedUserPassword);
usernameAndPassword = new String(decodedBytes, "UTF-8");
} catch (IOException e) {
e.printStackTrace();
}
final StringTokenizer tokenizer = new StringTokenizer(usernameAndPassword, ":");
final String username = tokenizer.nextToken();
if (request.getSession() != null) {
String mobile_number = (String) request.getSession().getAttribute(ApiService.CONTACT_ID_KEY);
if (mobile_number != username) {
return true;
}
}
return false;
}
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
Method method = resourceInfo.getResourceMethod();
if (!method.isAnnotationPresent(PermitAll.class)) {
// Fetch authorization header
final String authorization = requestContext.getHeaderString(AUTHORIZATION_PROPERTY);
// If no authorization information present; block access
if (authorization == null || authorization.isEmpty()) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
if(!isAuthenticated(authorization)) {
requestContext.abortWith(ACCESS_DENIED);
return;
}
}
}
}
And this is my Application class
package com.example;
import java.util.HashMap;
import java.util.Map;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/rest")
public class ApiConfig extends Application {
public Map<String, Object> getProperties() {
Map<String, Object> properties = new HashMap<>();
properties.put("jersey.config.server.provider.packages", "com.example");
return properties;
}
}
Thank you.
java jersey jax-rs tomcat8 requestfiltering
java jersey jax-rs tomcat8 requestfiltering
asked Nov 21 at 1:56
Chitraveer Akhil
13210
13210
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You need to annotate it with @Provider
. The scanning picks up classes that are annotated with @Provider
and @Path
. You also need to add @Context
for the HttpServletRequest
if you want it injected (you only have it on the ResourceInfo
).
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You need to annotate it with @Provider
. The scanning picks up classes that are annotated with @Provider
and @Path
. You also need to add @Context
for the HttpServletRequest
if you want it injected (you only have it on the ResourceInfo
).
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
add a comment |
up vote
1
down vote
accepted
You need to annotate it with @Provider
. The scanning picks up classes that are annotated with @Provider
and @Path
. You also need to add @Context
for the HttpServletRequest
if you want it injected (you only have it on the ResourceInfo
).
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You need to annotate it with @Provider
. The scanning picks up classes that are annotated with @Provider
and @Path
. You also need to add @Context
for the HttpServletRequest
if you want it injected (you only have it on the ResourceInfo
).
You need to annotate it with @Provider
. The scanning picks up classes that are annotated with @Provider
and @Path
. You also need to add @Context
for the HttpServletRequest
if you want it injected (you only have it on the ResourceInfo
).
answered Nov 21 at 3:48


Paul Samsotha
147k19277462
147k19277462
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
add a comment |
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
Thank you. It works.
– Chitraveer Akhil
Nov 21 at 10:02
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404287%2fenable-containerrequestfilter-without-web-xml%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ODmjLKDfN9MipivRivIQCZcORvlGrmvUlwfSor,x2Ik 6fMzpaOmfLnja,J Gr,hZaVMyuoRaG