input_shape definition in a dense layer when input is an array
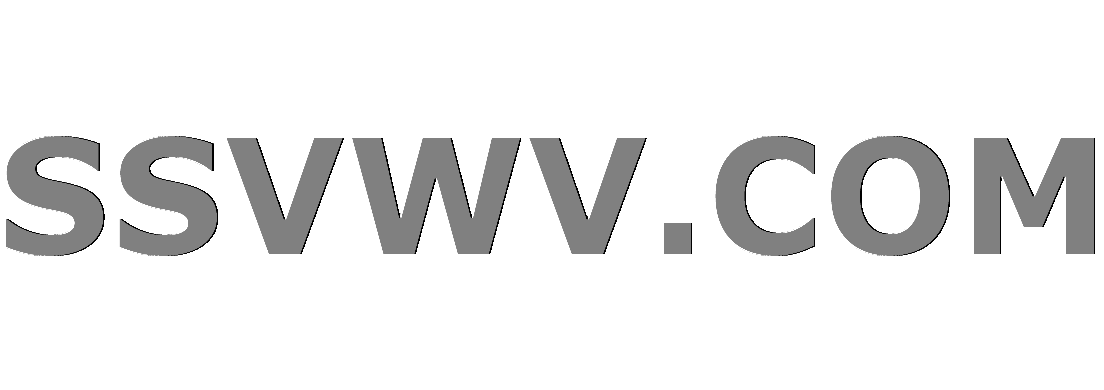
Multi tool use
up vote
0
down vote
favorite
I have an input array, for deep learning classifier, looking like this:
[[ a1, [b1,c1]
[d1,e1]
[f1,g1]]
[ a2, [b2,c2]
[d2,e2]
[f2,g2]]
[h2,i2]]
.......]
So each set has one float and one array (N, 2) where N is not the same for each set.
When googling I noticed that I can input multiple sizes into input_shape value so I tried:
input_shape=(1,(2,None)) /None means undefined size
I tried changing order but it didn't help. Every time I change input_dim to input_shape I have:
TypeError: Error converting shape to a TensorShape: int() argument must be a string, a bytes-like object or a number, not 'tuple'.
How should I define input dim in my case? Thanks!
Code:
classifier = Sequential()
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu', input_shape=(1,(2,None))))
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu'))
classifier.add(Dense(output_dim = 1, init = 'uniform', activation = 'sigmoid'))
classifier.compile(optimizer = 'Adamax', loss = 'binary_crossentropy', metrics = ['accuracy'])
classifier.fit(X_train, y_train, batch_size = 10, epochs = 100)
python tensorflow machine-learning keras deep-learning
add a comment |
up vote
0
down vote
favorite
I have an input array, for deep learning classifier, looking like this:
[[ a1, [b1,c1]
[d1,e1]
[f1,g1]]
[ a2, [b2,c2]
[d2,e2]
[f2,g2]]
[h2,i2]]
.......]
So each set has one float and one array (N, 2) where N is not the same for each set.
When googling I noticed that I can input multiple sizes into input_shape value so I tried:
input_shape=(1,(2,None)) /None means undefined size
I tried changing order but it didn't help. Every time I change input_dim to input_shape I have:
TypeError: Error converting shape to a TensorShape: int() argument must be a string, a bytes-like object or a number, not 'tuple'.
How should I define input dim in my case? Thanks!
Code:
classifier = Sequential()
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu', input_shape=(1,(2,None))))
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu'))
classifier.add(Dense(output_dim = 1, init = 'uniform', activation = 'sigmoid'))
classifier.compile(optimizer = 'Adamax', loss = 'binary_crossentropy', metrics = ['accuracy'])
classifier.fit(X_train, y_train, batch_size = 10, epochs = 100)
python tensorflow machine-learning keras deep-learning
You will need a model with two separate inputs. This is not possible with the Sequential API but requires the Funtional API. Have a look at the docs for some examples. In addition, you will need to pass your second input to a layer that can handle the varying size, such as an LSTM or an Embedding layer.
– sdcbr
9 hours ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have an input array, for deep learning classifier, looking like this:
[[ a1, [b1,c1]
[d1,e1]
[f1,g1]]
[ a2, [b2,c2]
[d2,e2]
[f2,g2]]
[h2,i2]]
.......]
So each set has one float and one array (N, 2) where N is not the same for each set.
When googling I noticed that I can input multiple sizes into input_shape value so I tried:
input_shape=(1,(2,None)) /None means undefined size
I tried changing order but it didn't help. Every time I change input_dim to input_shape I have:
TypeError: Error converting shape to a TensorShape: int() argument must be a string, a bytes-like object or a number, not 'tuple'.
How should I define input dim in my case? Thanks!
Code:
classifier = Sequential()
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu', input_shape=(1,(2,None))))
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu'))
classifier.add(Dense(output_dim = 1, init = 'uniform', activation = 'sigmoid'))
classifier.compile(optimizer = 'Adamax', loss = 'binary_crossentropy', metrics = ['accuracy'])
classifier.fit(X_train, y_train, batch_size = 10, epochs = 100)
python tensorflow machine-learning keras deep-learning
I have an input array, for deep learning classifier, looking like this:
[[ a1, [b1,c1]
[d1,e1]
[f1,g1]]
[ a2, [b2,c2]
[d2,e2]
[f2,g2]]
[h2,i2]]
.......]
So each set has one float and one array (N, 2) where N is not the same for each set.
When googling I noticed that I can input multiple sizes into input_shape value so I tried:
input_shape=(1,(2,None)) /None means undefined size
I tried changing order but it didn't help. Every time I change input_dim to input_shape I have:
TypeError: Error converting shape to a TensorShape: int() argument must be a string, a bytes-like object or a number, not 'tuple'.
How should I define input dim in my case? Thanks!
Code:
classifier = Sequential()
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu', input_shape=(1,(2,None))))
classifier.add(Dense(output_dim = 10, init = 'uniform', activation = 'relu'))
classifier.add(Dense(output_dim = 1, init = 'uniform', activation = 'sigmoid'))
classifier.compile(optimizer = 'Adamax', loss = 'binary_crossentropy', metrics = ['accuracy'])
classifier.fit(X_train, y_train, batch_size = 10, epochs = 100)
python tensorflow machine-learning keras deep-learning
python tensorflow machine-learning keras deep-learning
asked 9 hours ago
Krzychu111
92
92
You will need a model with two separate inputs. This is not possible with the Sequential API but requires the Funtional API. Have a look at the docs for some examples. In addition, you will need to pass your second input to a layer that can handle the varying size, such as an LSTM or an Embedding layer.
– sdcbr
9 hours ago
add a comment |
You will need a model with two separate inputs. This is not possible with the Sequential API but requires the Funtional API. Have a look at the docs for some examples. In addition, you will need to pass your second input to a layer that can handle the varying size, such as an LSTM or an Embedding layer.
– sdcbr
9 hours ago
You will need a model with two separate inputs. This is not possible with the Sequential API but requires the Funtional API. Have a look at the docs for some examples. In addition, you will need to pass your second input to a layer that can handle the varying size, such as an LSTM or an Embedding layer.
– sdcbr
9 hours ago
You will need a model with two separate inputs. This is not possible with the Sequential API but requires the Funtional API. Have a look at the docs for some examples. In addition, you will need to pass your second input to a layer that can handle the varying size, such as an LSTM or an Embedding layer.
– sdcbr
9 hours ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400687%2finput-shape-definition-in-a-dense-layer-when-input-is-an-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cUfqj8,Mm aZ 7D,mSXu
You will need a model with two separate inputs. This is not possible with the Sequential API but requires the Funtional API. Have a look at the docs for some examples. In addition, you will need to pass your second input to a layer that can handle the varying size, such as an LSTM or an Embedding layer.
– sdcbr
9 hours ago