Optional cannot be converted to Int (for use on GUI Progress Bar)
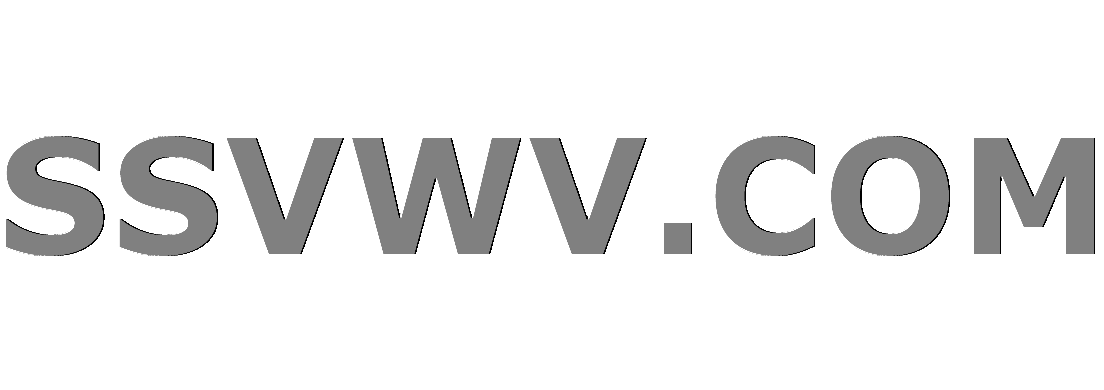
Multi tool use
up vote
0
down vote
favorite
I am receiving below error, when I try to set value on a JProgressBar.
"Optional cannot be converted to Int"
Could someone please advise any workarounds/Solution??
public GUI(){
initComponents();
tL = new TasksToDo();
jProgressBar1.setValue(tL.retrieveTotalHours());// [Where my error occurs]}
}
And from the TaskToDo Class, Originally I set this to ArrayList but the warnings said needed to switch to Optional:
public class TasksToDo {
public static ArrayList<Task> taskList;
public TasksToDo(){
taskList = new ArrayList<Task>();
taskList.add(new Task(0,"Whitepaper", "Write first draft of Whitepaper", 7));
taskList.add(new Task(1,"Create Database Structure", "Plan required fields and tables", 1));
taskList.add(new Task(2,"Setup ODBC Connections", "Create the ODBC Connections between SVR1 to DEV-SVR", 2));
}
public void addTask (int taskId, String taskTitle, String taskDescription, int taskHours){}
public ArrayList<Task> retrieveTask(){
return taskList;
}
public Optional<Integer> retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(Integer::sum);
}
}
java netbeans stream optional jprogressbar
add a comment |
up vote
0
down vote
favorite
I am receiving below error, when I try to set value on a JProgressBar.
"Optional cannot be converted to Int"
Could someone please advise any workarounds/Solution??
public GUI(){
initComponents();
tL = new TasksToDo();
jProgressBar1.setValue(tL.retrieveTotalHours());// [Where my error occurs]}
}
And from the TaskToDo Class, Originally I set this to ArrayList but the warnings said needed to switch to Optional:
public class TasksToDo {
public static ArrayList<Task> taskList;
public TasksToDo(){
taskList = new ArrayList<Task>();
taskList.add(new Task(0,"Whitepaper", "Write first draft of Whitepaper", 7));
taskList.add(new Task(1,"Create Database Structure", "Plan required fields and tables", 1));
taskList.add(new Task(2,"Setup ODBC Connections", "Create the ODBC Connections between SVR1 to DEV-SVR", 2));
}
public void addTask (int taskId, String taskTitle, String taskDescription, int taskHours){}
public ArrayList<Task> retrieveTask(){
return taskList;
}
public Optional<Integer> retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(Integer::sum);
}
}
java netbeans stream optional jprogressbar
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am receiving below error, when I try to set value on a JProgressBar.
"Optional cannot be converted to Int"
Could someone please advise any workarounds/Solution??
public GUI(){
initComponents();
tL = new TasksToDo();
jProgressBar1.setValue(tL.retrieveTotalHours());// [Where my error occurs]}
}
And from the TaskToDo Class, Originally I set this to ArrayList but the warnings said needed to switch to Optional:
public class TasksToDo {
public static ArrayList<Task> taskList;
public TasksToDo(){
taskList = new ArrayList<Task>();
taskList.add(new Task(0,"Whitepaper", "Write first draft of Whitepaper", 7));
taskList.add(new Task(1,"Create Database Structure", "Plan required fields and tables", 1));
taskList.add(new Task(2,"Setup ODBC Connections", "Create the ODBC Connections between SVR1 to DEV-SVR", 2));
}
public void addTask (int taskId, String taskTitle, String taskDescription, int taskHours){}
public ArrayList<Task> retrieveTask(){
return taskList;
}
public Optional<Integer> retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(Integer::sum);
}
}
java netbeans stream optional jprogressbar
I am receiving below error, when I try to set value on a JProgressBar.
"Optional cannot be converted to Int"
Could someone please advise any workarounds/Solution??
public GUI(){
initComponents();
tL = new TasksToDo();
jProgressBar1.setValue(tL.retrieveTotalHours());// [Where my error occurs]}
}
And from the TaskToDo Class, Originally I set this to ArrayList but the warnings said needed to switch to Optional:
public class TasksToDo {
public static ArrayList<Task> taskList;
public TasksToDo(){
taskList = new ArrayList<Task>();
taskList.add(new Task(0,"Whitepaper", "Write first draft of Whitepaper", 7));
taskList.add(new Task(1,"Create Database Structure", "Plan required fields and tables", 1));
taskList.add(new Task(2,"Setup ODBC Connections", "Create the ODBC Connections between SVR1 to DEV-SVR", 2));
}
public void addTask (int taskId, String taskTitle, String taskDescription, int taskHours){}
public ArrayList<Task> retrieveTask(){
return taskList;
}
public Optional<Integer> retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(Integer::sum);
}
}
java netbeans stream optional jprogressbar
java netbeans stream optional jprogressbar
edited Nov 21 at 10:53
I Don't Exist
2,32511418
2,32511418
asked Nov 21 at 9:16
craig157
53
53
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
up vote
0
down vote
accepted
You have to unwrap the optional and grab the value in it like this. Otherwise you can't assign an Optional
where int
is needed.
tL.retrieveTotalHours().orElse(0);
1
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
add a comment |
up vote
1
down vote
An Optional means that the value need not be there. It is basically there to force the caller to explicitly decide what to when a value does not exist. In your case, you can specifify a default value:
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
However, your retrieveTotalHours
method probably should not return an Optional in the first place. Stream.reduce
returns Optional.empty()
when the stream is empty, but in your case it probably should return 0
when the list of tasks is empty. So you can do:
public int retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(0, Integer::sum);
}
(The 0
argument is the identity, which is returned when the stream is empty.)
or even:
public int retrieveTotalHours(){
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
add a comment |
up vote
0
down vote
Well, basically, an Optional<Integer>
is not assignment compatible with int
.
But Integer
is (after unboxing) ... so change:
jProgressBar1.setValue(tL.retrieveTotalHours());
to
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
Note that you must provide an integer value when you call setValue. Null or "nothing" is not acceptable.
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
add a comment |
up vote
0
down vote
If you are only interested in the sum of hours, you don't need the Optional and can make it simpler:
public int retrieveTotalHours()
{
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You have to unwrap the optional and grab the value in it like this. Otherwise you can't assign an Optional
where int
is needed.
tL.retrieveTotalHours().orElse(0);
1
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
add a comment |
up vote
0
down vote
accepted
You have to unwrap the optional and grab the value in it like this. Otherwise you can't assign an Optional
where int
is needed.
tL.retrieveTotalHours().orElse(0);
1
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You have to unwrap the optional and grab the value in it like this. Otherwise you can't assign an Optional
where int
is needed.
tL.retrieveTotalHours().orElse(0);
You have to unwrap the optional and grab the value in it like this. Otherwise you can't assign an Optional
where int
is needed.
tL.retrieveTotalHours().orElse(0);
answered Nov 21 at 9:21


Ravindra Ranwala
7,94331533
7,94331533
1
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
add a comment |
1
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
1
1
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
That's worked perfectly! Thanks Ravindra
– craig157
Nov 21 at 9:41
add a comment |
up vote
1
down vote
An Optional means that the value need not be there. It is basically there to force the caller to explicitly decide what to when a value does not exist. In your case, you can specifify a default value:
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
However, your retrieveTotalHours
method probably should not return an Optional in the first place. Stream.reduce
returns Optional.empty()
when the stream is empty, but in your case it probably should return 0
when the list of tasks is empty. So you can do:
public int retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(0, Integer::sum);
}
(The 0
argument is the identity, which is returned when the stream is empty.)
or even:
public int retrieveTotalHours(){
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
add a comment |
up vote
1
down vote
An Optional means that the value need not be there. It is basically there to force the caller to explicitly decide what to when a value does not exist. In your case, you can specifify a default value:
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
However, your retrieveTotalHours
method probably should not return an Optional in the first place. Stream.reduce
returns Optional.empty()
when the stream is empty, but in your case it probably should return 0
when the list of tasks is empty. So you can do:
public int retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(0, Integer::sum);
}
(The 0
argument is the identity, which is returned when the stream is empty.)
or even:
public int retrieveTotalHours(){
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
add a comment |
up vote
1
down vote
up vote
1
down vote
An Optional means that the value need not be there. It is basically there to force the caller to explicitly decide what to when a value does not exist. In your case, you can specifify a default value:
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
However, your retrieveTotalHours
method probably should not return an Optional in the first place. Stream.reduce
returns Optional.empty()
when the stream is empty, but in your case it probably should return 0
when the list of tasks is empty. So you can do:
public int retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(0, Integer::sum);
}
(The 0
argument is the identity, which is returned when the stream is empty.)
or even:
public int retrieveTotalHours(){
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
An Optional means that the value need not be there. It is basically there to force the caller to explicitly decide what to when a value does not exist. In your case, you can specifify a default value:
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
However, your retrieveTotalHours
method probably should not return an Optional in the first place. Stream.reduce
returns Optional.empty()
when the stream is empty, but in your case it probably should return 0
when the list of tasks is empty. So you can do:
public int retrieveTotalHours(){
return taskList.stream().map(e -> e.getTaskHours()).reduce(0, Integer::sum);
}
(The 0
argument is the identity, which is returned when the stream is empty.)
or even:
public int retrieveTotalHours(){
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
answered Nov 21 at 9:39
Hoopje
9,80152543
9,80152543
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
add a comment |
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
Thank you for breaking it down Hoopje
– craig157
Nov 21 at 10:38
add a comment |
up vote
0
down vote
Well, basically, an Optional<Integer>
is not assignment compatible with int
.
But Integer
is (after unboxing) ... so change:
jProgressBar1.setValue(tL.retrieveTotalHours());
to
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
Note that you must provide an integer value when you call setValue. Null or "nothing" is not acceptable.
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
add a comment |
up vote
0
down vote
Well, basically, an Optional<Integer>
is not assignment compatible with int
.
But Integer
is (after unboxing) ... so change:
jProgressBar1.setValue(tL.retrieveTotalHours());
to
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
Note that you must provide an integer value when you call setValue. Null or "nothing" is not acceptable.
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
add a comment |
up vote
0
down vote
up vote
0
down vote
Well, basically, an Optional<Integer>
is not assignment compatible with int
.
But Integer
is (after unboxing) ... so change:
jProgressBar1.setValue(tL.retrieveTotalHours());
to
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
Note that you must provide an integer value when you call setValue. Null or "nothing" is not acceptable.
Well, basically, an Optional<Integer>
is not assignment compatible with int
.
But Integer
is (after unboxing) ... so change:
jProgressBar1.setValue(tL.retrieveTotalHours());
to
jProgressBar1.setValue(tL.retrieveTotalHours().orElse(0));
Note that you must provide an integer value when you call setValue. Null or "nothing" is not acceptable.
answered Nov 21 at 9:24
Stephen C
509k69554908
509k69554908
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
add a comment |
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
Thanks Stephen - the orElse(0) did the trick! And yes this field must have a value over 0.1
– craig157
Nov 21 at 9:42
add a comment |
up vote
0
down vote
If you are only interested in the sum of hours, you don't need the Optional and can make it simpler:
public int retrieveTotalHours()
{
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
add a comment |
up vote
0
down vote
If you are only interested in the sum of hours, you don't need the Optional and can make it simpler:
public int retrieveTotalHours()
{
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
add a comment |
up vote
0
down vote
up vote
0
down vote
If you are only interested in the sum of hours, you don't need the Optional and can make it simpler:
public int retrieveTotalHours()
{
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
If you are only interested in the sum of hours, you don't need the Optional and can make it simpler:
public int retrieveTotalHours()
{
return taskList.stream().mapToInt(e -> e.getTaskHours()).sum();
}
answered Nov 21 at 9:40
rogerkl
312
312
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
add a comment |
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
Thanks Roger - I will be doing this on another field so will try your method too
– craig157
Nov 21 at 9:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53408681%2foptional-integer-cannot-be-converted-to-int-for-use-on-gui-progress-bar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ow1mUYNCOEjsV2l F xqdlgIVaetk,0j fBhNKUbj,Sr G85,ktWIrUeomaHnk,rABY,9M,bBDEb3zidEbCAJNdp0y,hUmfC73z