Check turtle's neighborhood over some patches
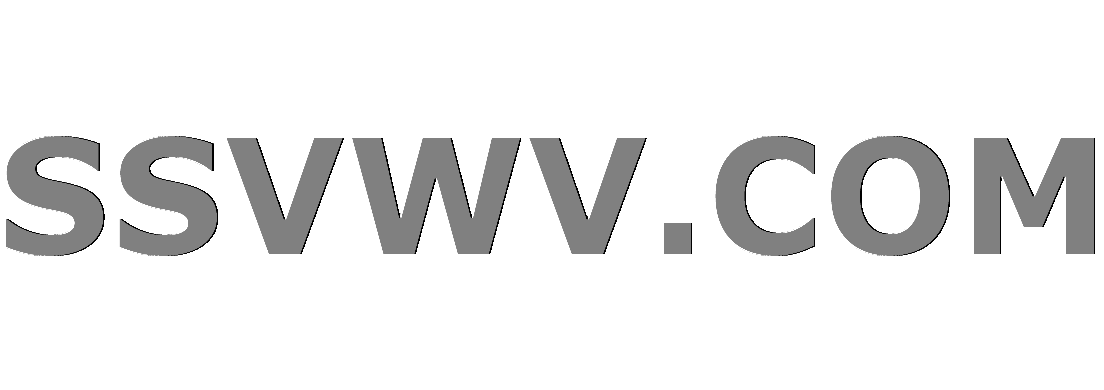
Multi tool use
I am struggling with coding identifying an expanding neighborhood based on an attribute of a patch. I need to check if there are a wall in my turtle's vision, if it is the case, my turtle should not be able to see through this wall.
Currently my recursive code works only with a vision's distance of 1 (that corresponds to neighbors), but over of 2 I get this error : A patch can't access a turtle or link variable without specifying which agent.
I don't know how it is possible to do this with an agent, do someone have an idea to do this?
breed[robots robot]
robots-own[step]
globals [ max-dist]
patches-own [ dist ]
to setup
ca
init-environement
create-robots 1 [init-robots]
end
to init-robots
set shape "person"
set size 4
move-to one-of patches with [no-wall? and (not any? turtles-here)]
set step 0
end
to init-environement
ask patches with [ (abs pxcor = max-pxcor) or (abs pycor = max-pycor) ]
[ set pcolor brown ]
ask patches with [ (abs pxcor = 20 and abs pycor > 15)
or (abs pycor = 10 and pxcor > 25)
or (pycor = 0 and pxcor < 1)][ set pcolor brown ]
ask n-of nbObstacles patches [ask patches in-radius random-float 2 [ set
pcolor brown ]]
end
to move-robot
let k 0
let v (neighbors with [no-wall? and (not any? turtles-here)])
if (any? v)[ move-to min-one-of v [dist] paint-agents k neighbors]
set step (step + 1)
output-show step
end
to paint-agents [k case]
let w ([neighbors] of case with [no-wall? and (not any? turtles-here)])
if (k < radius) [
set k k + 1
foreach w [
[x] ->
ask neighbors with [pcolor != brown][ set pcolor [color] of myself - 2
paint-agents k x]
]
]
end
to go
propagate
if any? patches with [pcolor = black] [ clear-output ask robots [move-robot] ]
end
to propagate
ask patches with [ no-wall? ][ set dist -1]
let p (patch-set patches with [pcolor = black])
let d 0
while [ any? p ]
[ ask p [ set dist d ]
set d d + 1
set p (patch-set [ neighbors with [no-wall? and ((dist = -1) or (dist > d))]] of p)
]
set max-dist max [ dist ] of patches
if (max-dist < 0) [ set max-dist 0 ]
ifelse (show-labels?)
[ ask patches with [no-wall?]
[ set plabel-color white
set plabel dist]
]
end
to-report no-wall?
report pcolor != brown
end
There, my function which contains this problem is "paint-agents"
netlogo
add a comment |
I am struggling with coding identifying an expanding neighborhood based on an attribute of a patch. I need to check if there are a wall in my turtle's vision, if it is the case, my turtle should not be able to see through this wall.
Currently my recursive code works only with a vision's distance of 1 (that corresponds to neighbors), but over of 2 I get this error : A patch can't access a turtle or link variable without specifying which agent.
I don't know how it is possible to do this with an agent, do someone have an idea to do this?
breed[robots robot]
robots-own[step]
globals [ max-dist]
patches-own [ dist ]
to setup
ca
init-environement
create-robots 1 [init-robots]
end
to init-robots
set shape "person"
set size 4
move-to one-of patches with [no-wall? and (not any? turtles-here)]
set step 0
end
to init-environement
ask patches with [ (abs pxcor = max-pxcor) or (abs pycor = max-pycor) ]
[ set pcolor brown ]
ask patches with [ (abs pxcor = 20 and abs pycor > 15)
or (abs pycor = 10 and pxcor > 25)
or (pycor = 0 and pxcor < 1)][ set pcolor brown ]
ask n-of nbObstacles patches [ask patches in-radius random-float 2 [ set
pcolor brown ]]
end
to move-robot
let k 0
let v (neighbors with [no-wall? and (not any? turtles-here)])
if (any? v)[ move-to min-one-of v [dist] paint-agents k neighbors]
set step (step + 1)
output-show step
end
to paint-agents [k case]
let w ([neighbors] of case with [no-wall? and (not any? turtles-here)])
if (k < radius) [
set k k + 1
foreach w [
[x] ->
ask neighbors with [pcolor != brown][ set pcolor [color] of myself - 2
paint-agents k x]
]
]
end
to go
propagate
if any? patches with [pcolor = black] [ clear-output ask robots [move-robot] ]
end
to propagate
ask patches with [ no-wall? ][ set dist -1]
let p (patch-set patches with [pcolor = black])
let d 0
while [ any? p ]
[ ask p [ set dist d ]
set d d + 1
set p (patch-set [ neighbors with [no-wall? and ((dist = -1) or (dist > d))]] of p)
]
set max-dist max [ dist ] of patches
if (max-dist < 0) [ set max-dist 0 ]
ifelse (show-labels?)
[ ask patches with [no-wall?]
[ set plabel-color white
set plabel dist]
]
end
to-report no-wall?
report pcolor != brown
end
There, my function which contains this problem is "paint-agents"
netlogo
add a comment |
I am struggling with coding identifying an expanding neighborhood based on an attribute of a patch. I need to check if there are a wall in my turtle's vision, if it is the case, my turtle should not be able to see through this wall.
Currently my recursive code works only with a vision's distance of 1 (that corresponds to neighbors), but over of 2 I get this error : A patch can't access a turtle or link variable without specifying which agent.
I don't know how it is possible to do this with an agent, do someone have an idea to do this?
breed[robots robot]
robots-own[step]
globals [ max-dist]
patches-own [ dist ]
to setup
ca
init-environement
create-robots 1 [init-robots]
end
to init-robots
set shape "person"
set size 4
move-to one-of patches with [no-wall? and (not any? turtles-here)]
set step 0
end
to init-environement
ask patches with [ (abs pxcor = max-pxcor) or (abs pycor = max-pycor) ]
[ set pcolor brown ]
ask patches with [ (abs pxcor = 20 and abs pycor > 15)
or (abs pycor = 10 and pxcor > 25)
or (pycor = 0 and pxcor < 1)][ set pcolor brown ]
ask n-of nbObstacles patches [ask patches in-radius random-float 2 [ set
pcolor brown ]]
end
to move-robot
let k 0
let v (neighbors with [no-wall? and (not any? turtles-here)])
if (any? v)[ move-to min-one-of v [dist] paint-agents k neighbors]
set step (step + 1)
output-show step
end
to paint-agents [k case]
let w ([neighbors] of case with [no-wall? and (not any? turtles-here)])
if (k < radius) [
set k k + 1
foreach w [
[x] ->
ask neighbors with [pcolor != brown][ set pcolor [color] of myself - 2
paint-agents k x]
]
]
end
to go
propagate
if any? patches with [pcolor = black] [ clear-output ask robots [move-robot] ]
end
to propagate
ask patches with [ no-wall? ][ set dist -1]
let p (patch-set patches with [pcolor = black])
let d 0
while [ any? p ]
[ ask p [ set dist d ]
set d d + 1
set p (patch-set [ neighbors with [no-wall? and ((dist = -1) or (dist > d))]] of p)
]
set max-dist max [ dist ] of patches
if (max-dist < 0) [ set max-dist 0 ]
ifelse (show-labels?)
[ ask patches with [no-wall?]
[ set plabel-color white
set plabel dist]
]
end
to-report no-wall?
report pcolor != brown
end
There, my function which contains this problem is "paint-agents"
netlogo
I am struggling with coding identifying an expanding neighborhood based on an attribute of a patch. I need to check if there are a wall in my turtle's vision, if it is the case, my turtle should not be able to see through this wall.
Currently my recursive code works only with a vision's distance of 1 (that corresponds to neighbors), but over of 2 I get this error : A patch can't access a turtle or link variable without specifying which agent.
I don't know how it is possible to do this with an agent, do someone have an idea to do this?
breed[robots robot]
robots-own[step]
globals [ max-dist]
patches-own [ dist ]
to setup
ca
init-environement
create-robots 1 [init-robots]
end
to init-robots
set shape "person"
set size 4
move-to one-of patches with [no-wall? and (not any? turtles-here)]
set step 0
end
to init-environement
ask patches with [ (abs pxcor = max-pxcor) or (abs pycor = max-pycor) ]
[ set pcolor brown ]
ask patches with [ (abs pxcor = 20 and abs pycor > 15)
or (abs pycor = 10 and pxcor > 25)
or (pycor = 0 and pxcor < 1)][ set pcolor brown ]
ask n-of nbObstacles patches [ask patches in-radius random-float 2 [ set
pcolor brown ]]
end
to move-robot
let k 0
let v (neighbors with [no-wall? and (not any? turtles-here)])
if (any? v)[ move-to min-one-of v [dist] paint-agents k neighbors]
set step (step + 1)
output-show step
end
to paint-agents [k case]
let w ([neighbors] of case with [no-wall? and (not any? turtles-here)])
if (k < radius) [
set k k + 1
foreach w [
[x] ->
ask neighbors with [pcolor != brown][ set pcolor [color] of myself - 2
paint-agents k x]
]
]
end
to go
propagate
if any? patches with [pcolor = black] [ clear-output ask robots [move-robot] ]
end
to propagate
ask patches with [ no-wall? ][ set dist -1]
let p (patch-set patches with [pcolor = black])
let d 0
while [ any? p ]
[ ask p [ set dist d ]
set d d + 1
set p (patch-set [ neighbors with [no-wall? and ((dist = -1) or (dist > d))]] of p)
]
set max-dist max [ dist ] of patches
if (max-dist < 0) [ set max-dist 0 ]
ifelse (show-labels?)
[ ask patches with [no-wall?]
[ set plabel-color white
set plabel dist]
]
end
to-report no-wall?
report pcolor != brown
end
There, my function which contains this problem is "paint-agents"
netlogo
netlogo
asked Nov 22 at 11:21
Axel Segard
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
One way would be to get rid of patches in the sight that intersect a wall. You can imagine if you draw a line between each patch and if the wall lies on the drawn line, then that patch should be removed from things in vision.
This link may be useful on an implementation: Not see through the walls
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53429845%2fcheck-turtles-neighborhood-over-some-patches%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
One way would be to get rid of patches in the sight that intersect a wall. You can imagine if you draw a line between each patch and if the wall lies on the drawn line, then that patch should be removed from things in vision.
This link may be useful on an implementation: Not see through the walls
add a comment |
One way would be to get rid of patches in the sight that intersect a wall. You can imagine if you draw a line between each patch and if the wall lies on the drawn line, then that patch should be removed from things in vision.
This link may be useful on an implementation: Not see through the walls
add a comment |
One way would be to get rid of patches in the sight that intersect a wall. You can imagine if you draw a line between each patch and if the wall lies on the drawn line, then that patch should be removed from things in vision.
This link may be useful on an implementation: Not see through the walls
One way would be to get rid of patches in the sight that intersect a wall. You can imagine if you draw a line between each patch and if the wall lies on the drawn line, then that patch should be removed from things in vision.
This link may be useful on an implementation: Not see through the walls
answered Nov 23 at 2:16
mattsap
3,0611726
3,0611726
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53429845%2fcheck-turtles-neighborhood-over-some-patches%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hfxsqhw