How to extend/overwrite an exported type in Typescript
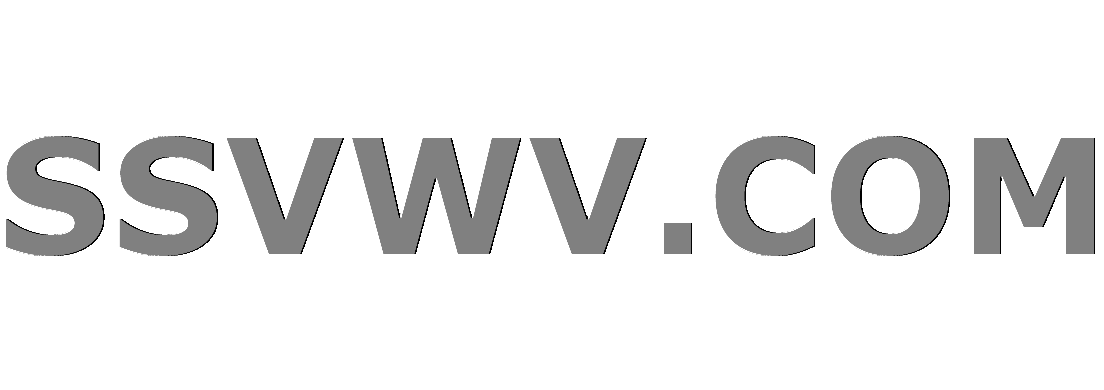
Multi tool use
I'm using @fortawesome
and @fortawesome/react-fontawesome
. @fortawesome/fontawesome-common-types
defines IconDefinition
as
export interface IconLookup {
prefix: IconPrefix;
// IconName is defined in the code that will be generated at build time and bundled with this file.
iconName: IconName;
}
export interface IconDefinition extends IconLookup {
icon: any;
}
export type IconProp = IconLookup | /*...*/;
export type IconName = /* a lot of concatenated strings */;
@fortawesome/react-fontawesome
defines
export function FontAwesomeIcon(props: Props): JSX.Element;
export interface Props {
icon: IconProp;
/* ... */
}
I wanted to add my own SVGs and use them in the icon
property of <FontAwesomeIcon />
.
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
type CustomIconName = 'myCustomIcon' /* | ... */;
interface CustomIconDefinition extends Pick<IconDefinition, Exclude<keyof IconDefinition, 'iconName'>> {
prefix: 'fab',
iconName: CustomIconName;
}
const MyCustomIcon: CustomIconDefinition = /* ... */;
export default function() {
let icon: any = MyCustomIcon;
return <FontAwesomeIcon icon={icon} />;
}
This works so long as icon
is of type any
.
I'd like to remove CustomIconDefinition
and overwrite or extend the IconName
definition to include MyCustomIcon
.
I thought to do something like:
import { IconName as VanillaIconName } from '@fortawesome/fontawesome-common-types';
declare module "@fortawesome/fontawesome-common-types" {
export type IconName = VanillaIconName | MyCustomIcon;
}
This results in Duplicate identifier 'IconName'. index.d.ts(16,13): 'IconName' was also declared here.
I suspect even if I get around this, it's going to have an error because IconName = VanillaIconName
introduces a circular definition.
I also thought to overwrite the FontAwesomeIcon
definition, but I didn't get far on that one because icon: IconProp
is several types deep. I suppose I could create my own CustomFontAwesomeIcon extends FontAwesomeIcon
, but this isn't ideal.
typescript
add a comment |
I'm using @fortawesome
and @fortawesome/react-fontawesome
. @fortawesome/fontawesome-common-types
defines IconDefinition
as
export interface IconLookup {
prefix: IconPrefix;
// IconName is defined in the code that will be generated at build time and bundled with this file.
iconName: IconName;
}
export interface IconDefinition extends IconLookup {
icon: any;
}
export type IconProp = IconLookup | /*...*/;
export type IconName = /* a lot of concatenated strings */;
@fortawesome/react-fontawesome
defines
export function FontAwesomeIcon(props: Props): JSX.Element;
export interface Props {
icon: IconProp;
/* ... */
}
I wanted to add my own SVGs and use them in the icon
property of <FontAwesomeIcon />
.
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
type CustomIconName = 'myCustomIcon' /* | ... */;
interface CustomIconDefinition extends Pick<IconDefinition, Exclude<keyof IconDefinition, 'iconName'>> {
prefix: 'fab',
iconName: CustomIconName;
}
const MyCustomIcon: CustomIconDefinition = /* ... */;
export default function() {
let icon: any = MyCustomIcon;
return <FontAwesomeIcon icon={icon} />;
}
This works so long as icon
is of type any
.
I'd like to remove CustomIconDefinition
and overwrite or extend the IconName
definition to include MyCustomIcon
.
I thought to do something like:
import { IconName as VanillaIconName } from '@fortawesome/fontawesome-common-types';
declare module "@fortawesome/fontawesome-common-types" {
export type IconName = VanillaIconName | MyCustomIcon;
}
This results in Duplicate identifier 'IconName'. index.d.ts(16,13): 'IconName' was also declared here.
I suspect even if I get around this, it's going to have an error because IconName = VanillaIconName
introduces a circular definition.
I also thought to overwrite the FontAwesomeIcon
definition, but I didn't get far on that one because icon: IconProp
is several types deep. I suppose I could create my own CustomFontAwesomeIcon extends FontAwesomeIcon
, but this isn't ideal.
typescript
This might be helpful. github.com/Microsoft/TypeScript/issues/28078 I spent 20-minutes trying to get it to work,though, without success. Your mileage may be better.
– Shaun Luttin
Nov 24 '18 at 4:31
Unless you can find a hack like that, it is not possible to augment a type alias nor to change the type of an interface property.
– Shaun Luttin
Nov 24 '18 at 5:12
add a comment |
I'm using @fortawesome
and @fortawesome/react-fontawesome
. @fortawesome/fontawesome-common-types
defines IconDefinition
as
export interface IconLookup {
prefix: IconPrefix;
// IconName is defined in the code that will be generated at build time and bundled with this file.
iconName: IconName;
}
export interface IconDefinition extends IconLookup {
icon: any;
}
export type IconProp = IconLookup | /*...*/;
export type IconName = /* a lot of concatenated strings */;
@fortawesome/react-fontawesome
defines
export function FontAwesomeIcon(props: Props): JSX.Element;
export interface Props {
icon: IconProp;
/* ... */
}
I wanted to add my own SVGs and use them in the icon
property of <FontAwesomeIcon />
.
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
type CustomIconName = 'myCustomIcon' /* | ... */;
interface CustomIconDefinition extends Pick<IconDefinition, Exclude<keyof IconDefinition, 'iconName'>> {
prefix: 'fab',
iconName: CustomIconName;
}
const MyCustomIcon: CustomIconDefinition = /* ... */;
export default function() {
let icon: any = MyCustomIcon;
return <FontAwesomeIcon icon={icon} />;
}
This works so long as icon
is of type any
.
I'd like to remove CustomIconDefinition
and overwrite or extend the IconName
definition to include MyCustomIcon
.
I thought to do something like:
import { IconName as VanillaIconName } from '@fortawesome/fontawesome-common-types';
declare module "@fortawesome/fontawesome-common-types" {
export type IconName = VanillaIconName | MyCustomIcon;
}
This results in Duplicate identifier 'IconName'. index.d.ts(16,13): 'IconName' was also declared here.
I suspect even if I get around this, it's going to have an error because IconName = VanillaIconName
introduces a circular definition.
I also thought to overwrite the FontAwesomeIcon
definition, but I didn't get far on that one because icon: IconProp
is several types deep. I suppose I could create my own CustomFontAwesomeIcon extends FontAwesomeIcon
, but this isn't ideal.
typescript
I'm using @fortawesome
and @fortawesome/react-fontawesome
. @fortawesome/fontawesome-common-types
defines IconDefinition
as
export interface IconLookup {
prefix: IconPrefix;
// IconName is defined in the code that will be generated at build time and bundled with this file.
iconName: IconName;
}
export interface IconDefinition extends IconLookup {
icon: any;
}
export type IconProp = IconLookup | /*...*/;
export type IconName = /* a lot of concatenated strings */;
@fortawesome/react-fontawesome
defines
export function FontAwesomeIcon(props: Props): JSX.Element;
export interface Props {
icon: IconProp;
/* ... */
}
I wanted to add my own SVGs and use them in the icon
property of <FontAwesomeIcon />
.
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
type CustomIconName = 'myCustomIcon' /* | ... */;
interface CustomIconDefinition extends Pick<IconDefinition, Exclude<keyof IconDefinition, 'iconName'>> {
prefix: 'fab',
iconName: CustomIconName;
}
const MyCustomIcon: CustomIconDefinition = /* ... */;
export default function() {
let icon: any = MyCustomIcon;
return <FontAwesomeIcon icon={icon} />;
}
This works so long as icon
is of type any
.
I'd like to remove CustomIconDefinition
and overwrite or extend the IconName
definition to include MyCustomIcon
.
I thought to do something like:
import { IconName as VanillaIconName } from '@fortawesome/fontawesome-common-types';
declare module "@fortawesome/fontawesome-common-types" {
export type IconName = VanillaIconName | MyCustomIcon;
}
This results in Duplicate identifier 'IconName'. index.d.ts(16,13): 'IconName' was also declared here.
I suspect even if I get around this, it's going to have an error because IconName = VanillaIconName
introduces a circular definition.
I also thought to overwrite the FontAwesomeIcon
definition, but I didn't get far on that one because icon: IconProp
is several types deep. I suppose I could create my own CustomFontAwesomeIcon extends FontAwesomeIcon
, but this isn't ideal.
typescript
typescript
asked Nov 24 '18 at 2:19


dx_over_dtdx_over_dt
1,60842447
1,60842447
This might be helpful. github.com/Microsoft/TypeScript/issues/28078 I spent 20-minutes trying to get it to work,though, without success. Your mileage may be better.
– Shaun Luttin
Nov 24 '18 at 4:31
Unless you can find a hack like that, it is not possible to augment a type alias nor to change the type of an interface property.
– Shaun Luttin
Nov 24 '18 at 5:12
add a comment |
This might be helpful. github.com/Microsoft/TypeScript/issues/28078 I spent 20-minutes trying to get it to work,though, without success. Your mileage may be better.
– Shaun Luttin
Nov 24 '18 at 4:31
Unless you can find a hack like that, it is not possible to augment a type alias nor to change the type of an interface property.
– Shaun Luttin
Nov 24 '18 at 5:12
This might be helpful. github.com/Microsoft/TypeScript/issues/28078 I spent 20-minutes trying to get it to work,though, without success. Your mileage may be better.
– Shaun Luttin
Nov 24 '18 at 4:31
This might be helpful. github.com/Microsoft/TypeScript/issues/28078 I spent 20-minutes trying to get it to work,though, without success. Your mileage may be better.
– Shaun Luttin
Nov 24 '18 at 4:31
Unless you can find a hack like that, it is not possible to augment a type alias nor to change the type of an interface property.
– Shaun Luttin
Nov 24 '18 at 5:12
Unless you can find a hack like that, it is not possible to augment a type alias nor to change the type of an interface property.
– Shaun Luttin
Nov 24 '18 at 5:12
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454644%2fhow-to-extend-overwrite-an-exported-type-in-typescript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454644%2fhow-to-extend-overwrite-an-exported-type-in-typescript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Q0p,Xjd7GWlQ7QHGiIOfMRpGwewc1
This might be helpful. github.com/Microsoft/TypeScript/issues/28078 I spent 20-minutes trying to get it to work,though, without success. Your mileage may be better.
– Shaun Luttin
Nov 24 '18 at 4:31
Unless you can find a hack like that, it is not possible to augment a type alias nor to change the type of an interface property.
– Shaun Luttin
Nov 24 '18 at 5:12