Most frequent and least duplicate frequent number in array
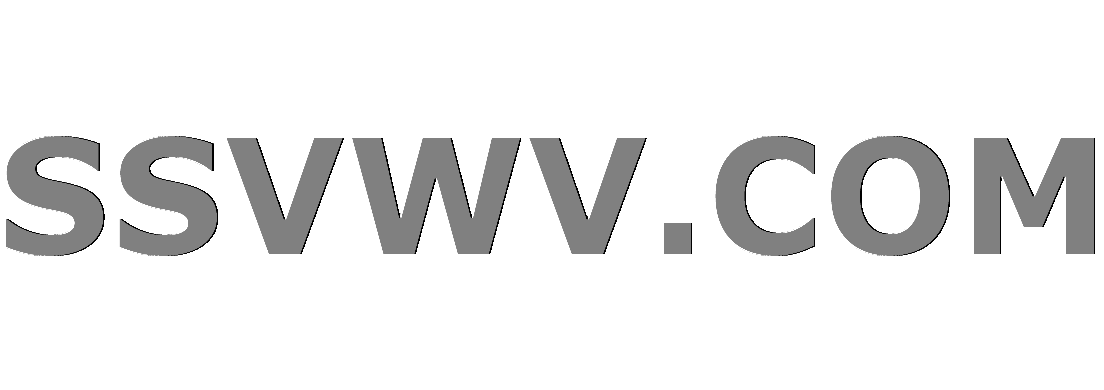
Multi tool use
I'm trying to find the most frequent number and the least frequent duplicate number in an array E.g
[7, 5, 6, 4, 6, 5, 5, 8, 7, 0, 7, 5, 2, 9, 7, 9, 3, 4, 6]
These are the duplicate number in the array above:
- "7" appears (4 times).
- "5" appears (4 times).
- "6" appears (3 times).
- "4" appears (2 times).
"7" and "5" are the most frequent number,
"4" is the least frequent duplicate.
When I tried to code, I was able to get the number 7, but then I don't know how to implement the least frequent.
This is the code I wrote:
String numbers = "7564655870752979346".split("");
String elements = "";
int count = 0;
for (String tempElement : numbers) {
int tempCount = 0;
for (n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
System.out.println("Frequent number is: " + elements + " It appeared " + count+" times");
My solution above only prints out 7, and I don't know how to check for the least duplicate.
java arrays duplicates frequency
add a comment |
I'm trying to find the most frequent number and the least frequent duplicate number in an array E.g
[7, 5, 6, 4, 6, 5, 5, 8, 7, 0, 7, 5, 2, 9, 7, 9, 3, 4, 6]
These are the duplicate number in the array above:
- "7" appears (4 times).
- "5" appears (4 times).
- "6" appears (3 times).
- "4" appears (2 times).
"7" and "5" are the most frequent number,
"4" is the least frequent duplicate.
When I tried to code, I was able to get the number 7, but then I don't know how to implement the least frequent.
This is the code I wrote:
String numbers = "7564655870752979346".split("");
String elements = "";
int count = 0;
for (String tempElement : numbers) {
int tempCount = 0;
for (n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
System.out.println("Frequent number is: " + elements + " It appeared " + count+" times");
My solution above only prints out 7, and I don't know how to check for the least duplicate.
java arrays duplicates frequency
add a comment |
I'm trying to find the most frequent number and the least frequent duplicate number in an array E.g
[7, 5, 6, 4, 6, 5, 5, 8, 7, 0, 7, 5, 2, 9, 7, 9, 3, 4, 6]
These are the duplicate number in the array above:
- "7" appears (4 times).
- "5" appears (4 times).
- "6" appears (3 times).
- "4" appears (2 times).
"7" and "5" are the most frequent number,
"4" is the least frequent duplicate.
When I tried to code, I was able to get the number 7, but then I don't know how to implement the least frequent.
This is the code I wrote:
String numbers = "7564655870752979346".split("");
String elements = "";
int count = 0;
for (String tempElement : numbers) {
int tempCount = 0;
for (n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
System.out.println("Frequent number is: " + elements + " It appeared " + count+" times");
My solution above only prints out 7, and I don't know how to check for the least duplicate.
java arrays duplicates frequency
I'm trying to find the most frequent number and the least frequent duplicate number in an array E.g
[7, 5, 6, 4, 6, 5, 5, 8, 7, 0, 7, 5, 2, 9, 7, 9, 3, 4, 6]
These are the duplicate number in the array above:
- "7" appears (4 times).
- "5" appears (4 times).
- "6" appears (3 times).
- "4" appears (2 times).
"7" and "5" are the most frequent number,
"4" is the least frequent duplicate.
When I tried to code, I was able to get the number 7, but then I don't know how to implement the least frequent.
This is the code I wrote:
String numbers = "7564655870752979346".split("");
String elements = "";
int count = 0;
for (String tempElement : numbers) {
int tempCount = 0;
for (n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
System.out.println("Frequent number is: " + elements + " It appeared " + count+" times");
My solution above only prints out 7, and I don't know how to check for the least duplicate.
java arrays duplicates frequency
java arrays duplicates frequency
edited Dec 18 '18 at 21:57
king amada
asked Nov 24 '18 at 2:34


king amadaking amada
349
349
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Write a function to find Min Repeated number(started from 2)
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement : numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if(count == counter) {
return elements;
}
}
if(count < counter) {
return "";
}
return elements;
}
A loop over numbers:
String x = "";
int c = 2;
do {
x = findMin(numbers, c ++);
} while(x == "");
The whole code will be
public class X {
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if (count == counter) {
return elements;
}
}
if (count < counter) {
return "";
}
return elements;
}
public static void main(String args) {
String numbers = "756655874075297346".split("");
String elements = "";
int count = 0;
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
String x = "";
int c = 2;
do {
x = findMin(numbers, c++);
} while (x == "");
System.out.println("Frequent number is: " + elements + " It appeared " + count + " times");
System.out.println("Min Frequent number is: " + x + " It appeared " + (c - 1) + " times");
}
}
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
add a comment |
Solution:
- Count the number of times each digit appears. Use an array of counts, one for each distinct digit.
- Find the position in the array of counts with count == 2.
Implementation ... is for you to do. But you will need to find a way to convert a String
containing one digit into an integer. (Hint: search the javadocs!)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454707%2fmost-frequent-and-least-duplicate-frequent-number-in-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Write a function to find Min Repeated number(started from 2)
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement : numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if(count == counter) {
return elements;
}
}
if(count < counter) {
return "";
}
return elements;
}
A loop over numbers:
String x = "";
int c = 2;
do {
x = findMin(numbers, c ++);
} while(x == "");
The whole code will be
public class X {
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if (count == counter) {
return elements;
}
}
if (count < counter) {
return "";
}
return elements;
}
public static void main(String args) {
String numbers = "756655874075297346".split("");
String elements = "";
int count = 0;
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
String x = "";
int c = 2;
do {
x = findMin(numbers, c++);
} while (x == "");
System.out.println("Frequent number is: " + elements + " It appeared " + count + " times");
System.out.println("Min Frequent number is: " + x + " It appeared " + (c - 1) + " times");
}
}
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
add a comment |
Write a function to find Min Repeated number(started from 2)
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement : numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if(count == counter) {
return elements;
}
}
if(count < counter) {
return "";
}
return elements;
}
A loop over numbers:
String x = "";
int c = 2;
do {
x = findMin(numbers, c ++);
} while(x == "");
The whole code will be
public class X {
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if (count == counter) {
return elements;
}
}
if (count < counter) {
return "";
}
return elements;
}
public static void main(String args) {
String numbers = "756655874075297346".split("");
String elements = "";
int count = 0;
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
String x = "";
int c = 2;
do {
x = findMin(numbers, c++);
} while (x == "");
System.out.println("Frequent number is: " + elements + " It appeared " + count + " times");
System.out.println("Min Frequent number is: " + x + " It appeared " + (c - 1) + " times");
}
}
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
add a comment |
Write a function to find Min Repeated number(started from 2)
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement : numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if(count == counter) {
return elements;
}
}
if(count < counter) {
return "";
}
return elements;
}
A loop over numbers:
String x = "";
int c = 2;
do {
x = findMin(numbers, c ++);
} while(x == "");
The whole code will be
public class X {
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if (count == counter) {
return elements;
}
}
if (count < counter) {
return "";
}
return elements;
}
public static void main(String args) {
String numbers = "756655874075297346".split("");
String elements = "";
int count = 0;
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
String x = "";
int c = 2;
do {
x = findMin(numbers, c++);
} while (x == "");
System.out.println("Frequent number is: " + elements + " It appeared " + count + " times");
System.out.println("Min Frequent number is: " + x + " It appeared " + (c - 1) + " times");
}
}
Write a function to find Min Repeated number(started from 2)
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement : numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if(count == counter) {
return elements;
}
}
if(count < counter) {
return "";
}
return elements;
}
A loop over numbers:
String x = "";
int c = 2;
do {
x = findMin(numbers, c ++);
} while(x == "");
The whole code will be
public class X {
public static String findMin(String numbers, int counter) {
int count = 0;
String elements = "";
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > counter) {
count = 0;
break;
}
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
if (count == counter) {
return elements;
}
}
if (count < counter) {
return "";
}
return elements;
}
public static void main(String args) {
String numbers = "756655874075297346".split("");
String elements = "";
int count = 0;
for (String tempElement: numbers) {
int tempCount = 0;
for (int n = 0; n < numbers.length; n++) {
if (numbers[n].equals(tempElement)) {
tempCount++;
if (tempCount > count) {
elements = tempElement;
// System.out.println(elements);
count = tempCount;
}
}
}
}
String x = "";
int c = 2;
do {
x = findMin(numbers, c++);
} while (x == "");
System.out.println("Frequent number is: " + elements + " It appeared " + count + " times");
System.out.println("Min Frequent number is: " + x + " It appeared " + (c - 1) + " times");
}
}
answered Nov 24 '18 at 5:17


Saeed.AtaeeSaeed.Ataee
3,65811531
3,65811531
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
add a comment |
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
Thanks for the answer, but when I tried to use this number "3620035681863138685" It prints out 3 as the most frequent, whilst "8" and "6" appeared as many times as the "3". How can I then make all the highest frequency appear and I select the one that is higher in the case of the above number, 8 would higher as it's higher than 6 and 3.
– king amada
Nov 24 '18 at 6:06
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
For that case, The solution I suggest is to count all numbers' Repetition and store them in an array and then choose the numbers you want from that array. @kingamada
– Saeed.Ataee
Nov 24 '18 at 6:38
add a comment |
Solution:
- Count the number of times each digit appears. Use an array of counts, one for each distinct digit.
- Find the position in the array of counts with count == 2.
Implementation ... is for you to do. But you will need to find a way to convert a String
containing one digit into an integer. (Hint: search the javadocs!)
add a comment |
Solution:
- Count the number of times each digit appears. Use an array of counts, one for each distinct digit.
- Find the position in the array of counts with count == 2.
Implementation ... is for you to do. But you will need to find a way to convert a String
containing one digit into an integer. (Hint: search the javadocs!)
add a comment |
Solution:
- Count the number of times each digit appears. Use an array of counts, one for each distinct digit.
- Find the position in the array of counts with count == 2.
Implementation ... is for you to do. But you will need to find a way to convert a String
containing one digit into an integer. (Hint: search the javadocs!)
Solution:
- Count the number of times each digit appears. Use an array of counts, one for each distinct digit.
- Find the position in the array of counts with count == 2.
Implementation ... is for you to do. But you will need to find a way to convert a String
containing one digit into an integer. (Hint: search the javadocs!)
edited Nov 24 '18 at 3:06
answered Nov 24 '18 at 2:59
Stephen CStephen C
516k70565921
516k70565921
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454707%2fmost-frequent-and-least-duplicate-frequent-number-in-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cF mt8U,oeZe,ZSaS9,tJZ gxw01Z,tPNGaP1tWFqB,3QTHRHGgN3a