Exchange Mailbox Sent Items not saved
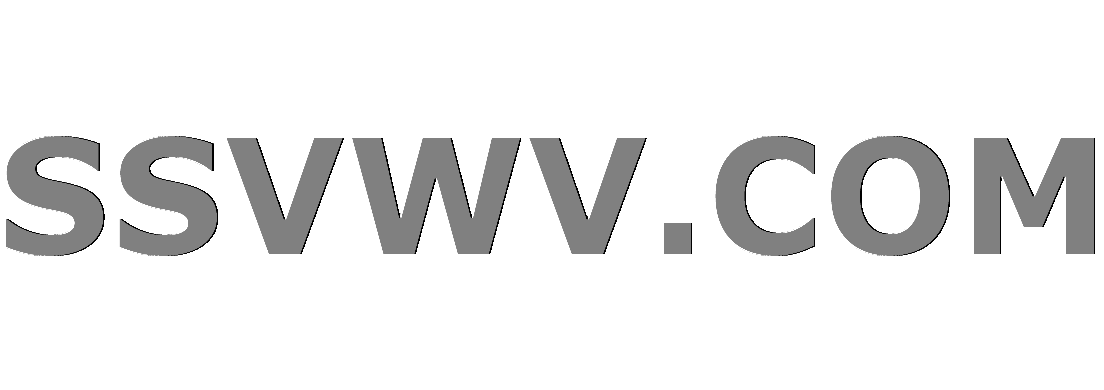
Multi tool use
up vote
1
down vote
favorite
I'm sending emails via a shared mailbox using the MS Exchange Web Services API.
Sending emails works but they are not saved in the sent items. As shown below doing it manually works, the items are saved in the Sent Items, but via my code doesn't save them:
using Microsoft.Exchange.WebServices.Data;
using System;
//Ref to Microsoft.Exchange.WebServices v15
//Re to Microsoft.Exchange.WebServices.Auth v15
namespace Emailing
{
public class Email
{
private string _sharedOutlookMailAccount = "aSharedEmailAccount@something.com";
private ExchangeService exchangeService = new ExchangeService(ExchangeVersion.Exchange2010_SP2);
public Email(string exchangeURL = "https://webmail.something.com/ews/exchange.asmx")
{
try
{
exchangeService.AutodiscoverUrl(_sharedOutlookMailAccount);
//exchangeService.ImpersonatedUserId = new ImpersonatedUserId(ConnectingIdType.SmtpAddress, _sharedOutlookMailAccount);
exchangeService.UseDefaultCredentials = true;
}
catch (System.Runtime.InteropServices.COMException ex)
{
//...
}
catch (Exception ex)
{
//...
}
}
public bool SendEmailFromSharedMailBox(string emailTo, string emailCc, string emailBcc, string emailSubject, string emailBody, string emailFileAttachments, bool emailFromSharedMailbox = false, bool sendToDraftOnly = false)
{
EmailMessage message = default(EmailMessage);
message = new EmailMessage(exchangeService);
emailTo = emailTo.TrimEnd(';', ',');
string emailArr = emailTo.Split(';', ',');
if (emailTo.Length > 0) message.ToRecipients.AddRange(emailArr);
emailCc = emailCc.TrimEnd( ';', ',' );
emailArr = emailCc.Split(';', ',' );
if (emailCc.Length > 0) message.CcRecipients.AddRange(emailArr);
emailBcc = emailBcc.TrimEnd(';', ',');
emailArr = emailBcc.Split(';', ',');
if (emailBcc.Length > 0) message.BccRecipients.AddRange(emailArr);
#if DEBUG
emailSubject = "IGNORE - TESTING ONLY - " + emailSubject;
#endif
message.Subject = emailSubject;
EmailAddress fromSender = new EmailAddress();
fromSender.MailboxType = MailboxType.Mailbox;
fromSender.Address = _sharedOutlookMailAccount;
message.From = fromSender;
if (emailFileAttachments != null)
{
foreach (string fileAttachment in emailFileAttachments)
{
if (string.IsNullOrEmpty(fileAttachment) == false)
message.Attachments.AddFileAttachment(fileAttachment);
}
}
message.Sensitivity = Sensitivity.Private;
message.Body = new MessageBody();
message.Body.BodyType = BodyType.HTML;
message.Body.Text = emailBody; //+= "_sharedMailSignature";
//Save the email message
try
{
//THIS WORKS AND IS REQUIRED FOR THE Send() &/or SendAndSaveCopy() METHODS TO WORK
message.Save(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
if (!sendToDraftOnly)
{
try
{
//==================================================================
//THIS SENDS EMAILS BUT THEY ARE NOT SAVED IN THE SENT ITEMS!
//==================================================================
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
}
return true;
}
}
Does anyone know how I can save the sent emails into the Sent Items folder?
c# email exchange-server exchangewebservices exchange-server-2010
add a comment |
up vote
1
down vote
favorite
I'm sending emails via a shared mailbox using the MS Exchange Web Services API.
Sending emails works but they are not saved in the sent items. As shown below doing it manually works, the items are saved in the Sent Items, but via my code doesn't save them:
using Microsoft.Exchange.WebServices.Data;
using System;
//Ref to Microsoft.Exchange.WebServices v15
//Re to Microsoft.Exchange.WebServices.Auth v15
namespace Emailing
{
public class Email
{
private string _sharedOutlookMailAccount = "aSharedEmailAccount@something.com";
private ExchangeService exchangeService = new ExchangeService(ExchangeVersion.Exchange2010_SP2);
public Email(string exchangeURL = "https://webmail.something.com/ews/exchange.asmx")
{
try
{
exchangeService.AutodiscoverUrl(_sharedOutlookMailAccount);
//exchangeService.ImpersonatedUserId = new ImpersonatedUserId(ConnectingIdType.SmtpAddress, _sharedOutlookMailAccount);
exchangeService.UseDefaultCredentials = true;
}
catch (System.Runtime.InteropServices.COMException ex)
{
//...
}
catch (Exception ex)
{
//...
}
}
public bool SendEmailFromSharedMailBox(string emailTo, string emailCc, string emailBcc, string emailSubject, string emailBody, string emailFileAttachments, bool emailFromSharedMailbox = false, bool sendToDraftOnly = false)
{
EmailMessage message = default(EmailMessage);
message = new EmailMessage(exchangeService);
emailTo = emailTo.TrimEnd(';', ',');
string emailArr = emailTo.Split(';', ',');
if (emailTo.Length > 0) message.ToRecipients.AddRange(emailArr);
emailCc = emailCc.TrimEnd( ';', ',' );
emailArr = emailCc.Split(';', ',' );
if (emailCc.Length > 0) message.CcRecipients.AddRange(emailArr);
emailBcc = emailBcc.TrimEnd(';', ',');
emailArr = emailBcc.Split(';', ',');
if (emailBcc.Length > 0) message.BccRecipients.AddRange(emailArr);
#if DEBUG
emailSubject = "IGNORE - TESTING ONLY - " + emailSubject;
#endif
message.Subject = emailSubject;
EmailAddress fromSender = new EmailAddress();
fromSender.MailboxType = MailboxType.Mailbox;
fromSender.Address = _sharedOutlookMailAccount;
message.From = fromSender;
if (emailFileAttachments != null)
{
foreach (string fileAttachment in emailFileAttachments)
{
if (string.IsNullOrEmpty(fileAttachment) == false)
message.Attachments.AddFileAttachment(fileAttachment);
}
}
message.Sensitivity = Sensitivity.Private;
message.Body = new MessageBody();
message.Body.BodyType = BodyType.HTML;
message.Body.Text = emailBody; //+= "_sharedMailSignature";
//Save the email message
try
{
//THIS WORKS AND IS REQUIRED FOR THE Send() &/or SendAndSaveCopy() METHODS TO WORK
message.Save(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
if (!sendToDraftOnly)
{
try
{
//==================================================================
//THIS SENDS EMAILS BUT THEY ARE NOT SAVED IN THE SENT ITEMS!
//==================================================================
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
}
return true;
}
}
Does anyone know how I can save the sent emails into the Sent Items folder?
c# email exchange-server exchangewebservices exchange-server-2010
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm sending emails via a shared mailbox using the MS Exchange Web Services API.
Sending emails works but they are not saved in the sent items. As shown below doing it manually works, the items are saved in the Sent Items, but via my code doesn't save them:
using Microsoft.Exchange.WebServices.Data;
using System;
//Ref to Microsoft.Exchange.WebServices v15
//Re to Microsoft.Exchange.WebServices.Auth v15
namespace Emailing
{
public class Email
{
private string _sharedOutlookMailAccount = "aSharedEmailAccount@something.com";
private ExchangeService exchangeService = new ExchangeService(ExchangeVersion.Exchange2010_SP2);
public Email(string exchangeURL = "https://webmail.something.com/ews/exchange.asmx")
{
try
{
exchangeService.AutodiscoverUrl(_sharedOutlookMailAccount);
//exchangeService.ImpersonatedUserId = new ImpersonatedUserId(ConnectingIdType.SmtpAddress, _sharedOutlookMailAccount);
exchangeService.UseDefaultCredentials = true;
}
catch (System.Runtime.InteropServices.COMException ex)
{
//...
}
catch (Exception ex)
{
//...
}
}
public bool SendEmailFromSharedMailBox(string emailTo, string emailCc, string emailBcc, string emailSubject, string emailBody, string emailFileAttachments, bool emailFromSharedMailbox = false, bool sendToDraftOnly = false)
{
EmailMessage message = default(EmailMessage);
message = new EmailMessage(exchangeService);
emailTo = emailTo.TrimEnd(';', ',');
string emailArr = emailTo.Split(';', ',');
if (emailTo.Length > 0) message.ToRecipients.AddRange(emailArr);
emailCc = emailCc.TrimEnd( ';', ',' );
emailArr = emailCc.Split(';', ',' );
if (emailCc.Length > 0) message.CcRecipients.AddRange(emailArr);
emailBcc = emailBcc.TrimEnd(';', ',');
emailArr = emailBcc.Split(';', ',');
if (emailBcc.Length > 0) message.BccRecipients.AddRange(emailArr);
#if DEBUG
emailSubject = "IGNORE - TESTING ONLY - " + emailSubject;
#endif
message.Subject = emailSubject;
EmailAddress fromSender = new EmailAddress();
fromSender.MailboxType = MailboxType.Mailbox;
fromSender.Address = _sharedOutlookMailAccount;
message.From = fromSender;
if (emailFileAttachments != null)
{
foreach (string fileAttachment in emailFileAttachments)
{
if (string.IsNullOrEmpty(fileAttachment) == false)
message.Attachments.AddFileAttachment(fileAttachment);
}
}
message.Sensitivity = Sensitivity.Private;
message.Body = new MessageBody();
message.Body.BodyType = BodyType.HTML;
message.Body.Text = emailBody; //+= "_sharedMailSignature";
//Save the email message
try
{
//THIS WORKS AND IS REQUIRED FOR THE Send() &/or SendAndSaveCopy() METHODS TO WORK
message.Save(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
if (!sendToDraftOnly)
{
try
{
//==================================================================
//THIS SENDS EMAILS BUT THEY ARE NOT SAVED IN THE SENT ITEMS!
//==================================================================
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
}
return true;
}
}
Does anyone know how I can save the sent emails into the Sent Items folder?
c# email exchange-server exchangewebservices exchange-server-2010
I'm sending emails via a shared mailbox using the MS Exchange Web Services API.
Sending emails works but they are not saved in the sent items. As shown below doing it manually works, the items are saved in the Sent Items, but via my code doesn't save them:
using Microsoft.Exchange.WebServices.Data;
using System;
//Ref to Microsoft.Exchange.WebServices v15
//Re to Microsoft.Exchange.WebServices.Auth v15
namespace Emailing
{
public class Email
{
private string _sharedOutlookMailAccount = "aSharedEmailAccount@something.com";
private ExchangeService exchangeService = new ExchangeService(ExchangeVersion.Exchange2010_SP2);
public Email(string exchangeURL = "https://webmail.something.com/ews/exchange.asmx")
{
try
{
exchangeService.AutodiscoverUrl(_sharedOutlookMailAccount);
//exchangeService.ImpersonatedUserId = new ImpersonatedUserId(ConnectingIdType.SmtpAddress, _sharedOutlookMailAccount);
exchangeService.UseDefaultCredentials = true;
}
catch (System.Runtime.InteropServices.COMException ex)
{
//...
}
catch (Exception ex)
{
//...
}
}
public bool SendEmailFromSharedMailBox(string emailTo, string emailCc, string emailBcc, string emailSubject, string emailBody, string emailFileAttachments, bool emailFromSharedMailbox = false, bool sendToDraftOnly = false)
{
EmailMessage message = default(EmailMessage);
message = new EmailMessage(exchangeService);
emailTo = emailTo.TrimEnd(';', ',');
string emailArr = emailTo.Split(';', ',');
if (emailTo.Length > 0) message.ToRecipients.AddRange(emailArr);
emailCc = emailCc.TrimEnd( ';', ',' );
emailArr = emailCc.Split(';', ',' );
if (emailCc.Length > 0) message.CcRecipients.AddRange(emailArr);
emailBcc = emailBcc.TrimEnd(';', ',');
emailArr = emailBcc.Split(';', ',');
if (emailBcc.Length > 0) message.BccRecipients.AddRange(emailArr);
#if DEBUG
emailSubject = "IGNORE - TESTING ONLY - " + emailSubject;
#endif
message.Subject = emailSubject;
EmailAddress fromSender = new EmailAddress();
fromSender.MailboxType = MailboxType.Mailbox;
fromSender.Address = _sharedOutlookMailAccount;
message.From = fromSender;
if (emailFileAttachments != null)
{
foreach (string fileAttachment in emailFileAttachments)
{
if (string.IsNullOrEmpty(fileAttachment) == false)
message.Attachments.AddFileAttachment(fileAttachment);
}
}
message.Sensitivity = Sensitivity.Private;
message.Body = new MessageBody();
message.Body.BodyType = BodyType.HTML;
message.Body.Text = emailBody; //+= "_sharedMailSignature";
//Save the email message
try
{
//THIS WORKS AND IS REQUIRED FOR THE Send() &/or SendAndSaveCopy() METHODS TO WORK
message.Save(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
if (!sendToDraftOnly)
{
try
{
//==================================================================
//THIS SENDS EMAILS BUT THEY ARE NOT SAVED IN THE SENT ITEMS!
//==================================================================
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.Drafts, _sharedOutlookMailAccount));
}
catch (Exception ex)
{
//...
return false;
}
}
return true;
}
}
Does anyone know how I can save the sent emails into the Sent Items folder?
c# email exchange-server exchangewebservices exchange-server-2010
c# email exchange-server exchangewebservices exchange-server-2010
edited Nov 22 at 0:13
asked Nov 21 at 23:14


Jeremy Thompson
39.2k13102198
39.2k13102198
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
I needed to use WellKnownFolderName.SentItems not Drafts.
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.SentItems, _sharedOutlookMailAccount));
Other causes/resolutions for this issue are described in this Microsoft Knowledge Base (KB) Article:
https://support.microsoft.com/en-au/help/2958272/email-sent-using-outlook-are-not-saved-to-the-sent-items-folder
add a comment |
up vote
0
down vote
Yes, you can use the SendAndSaveCopy function to achieve your needs. Here is an official document about EWS API.
Send the email message and save a copy
That won't work, you NEED to use themessage.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.
– Jeremy Thompson
Nov 22 at 22:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421766%2fexchange-mailbox-sent-items-not-saved%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
I needed to use WellKnownFolderName.SentItems not Drafts.
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.SentItems, _sharedOutlookMailAccount));
Other causes/resolutions for this issue are described in this Microsoft Knowledge Base (KB) Article:
https://support.microsoft.com/en-au/help/2958272/email-sent-using-outlook-are-not-saved-to-the-sent-items-folder
add a comment |
up vote
0
down vote
accepted
I needed to use WellKnownFolderName.SentItems not Drafts.
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.SentItems, _sharedOutlookMailAccount));
Other causes/resolutions for this issue are described in this Microsoft Knowledge Base (KB) Article:
https://support.microsoft.com/en-au/help/2958272/email-sent-using-outlook-are-not-saved-to-the-sent-items-folder
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I needed to use WellKnownFolderName.SentItems not Drafts.
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.SentItems, _sharedOutlookMailAccount));
Other causes/resolutions for this issue are described in this Microsoft Knowledge Base (KB) Article:
https://support.microsoft.com/en-au/help/2958272/email-sent-using-outlook-are-not-saved-to-the-sent-items-folder
I needed to use WellKnownFolderName.SentItems not Drafts.
message.SendAndSaveCopy(new FolderId(WellKnownFolderName.SentItems, _sharedOutlookMailAccount));
Other causes/resolutions for this issue are described in this Microsoft Knowledge Base (KB) Article:
https://support.microsoft.com/en-au/help/2958272/email-sent-using-outlook-are-not-saved-to-the-sent-items-folder
edited Nov 22 at 1:21
answered Nov 21 at 23:23


Jeremy Thompson
39.2k13102198
39.2k13102198
add a comment |
add a comment |
up vote
0
down vote
Yes, you can use the SendAndSaveCopy function to achieve your needs. Here is an official document about EWS API.
Send the email message and save a copy
That won't work, you NEED to use themessage.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.
– Jeremy Thompson
Nov 22 at 22:12
add a comment |
up vote
0
down vote
Yes, you can use the SendAndSaveCopy function to achieve your needs. Here is an official document about EWS API.
Send the email message and save a copy
That won't work, you NEED to use themessage.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.
– Jeremy Thompson
Nov 22 at 22:12
add a comment |
up vote
0
down vote
up vote
0
down vote
Yes, you can use the SendAndSaveCopy function to achieve your needs. Here is an official document about EWS API.
Send the email message and save a copy
Yes, you can use the SendAndSaveCopy function to achieve your needs. Here is an official document about EWS API.
Send the email message and save a copy
answered Nov 22 at 11:20
Simon Li
22014
22014
That won't work, you NEED to use themessage.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.
– Jeremy Thompson
Nov 22 at 22:12
add a comment |
That won't work, you NEED to use themessage.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.
– Jeremy Thompson
Nov 22 at 22:12
That won't work, you NEED to use the
message.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.– Jeremy Thompson
Nov 22 at 22:12
That won't work, you NEED to use the
message.SendAndSaveCopy();
overload that accepts the FolderId. Otherwise you get the error message: SendOnly cannot be used by a user without a mailbox. Use SendAndSaveCopy and specify a folder ID in a mailbox to send an item from an account that doesn't have a mailbox.– Jeremy Thompson
Nov 22 at 22:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421766%2fexchange-mailbox-sent-items-not-saved%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ngB,MeJPjbt,wRkOPeR 2mfWY7aCQV7zl,Wxj,Oz2D,B pOfq 4gx4qh,CKFc F7Ax3vPSlQPjelI7pFkRQ0AL7 xCdRx0Ctlmj,mLrc