How to make a PHP SOAP call using the SoapClient class
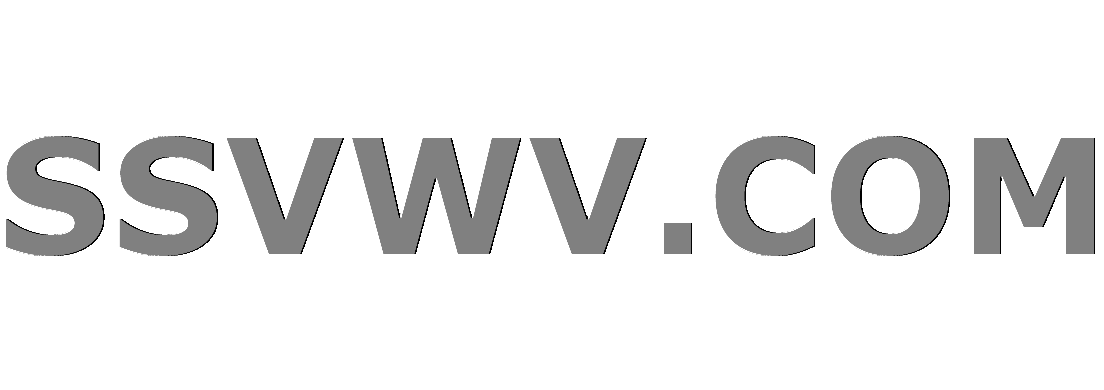
Multi tool use
up vote
102
down vote
favorite
I'm used to writing PHP code, but do not often use Object-Oriented coding. I now need to interact with SOAP (as a client) and am not able to get the syntax right. I've got a WSDL file which allows me to properly set up a new connection using the SoapClient class. However, I'm unable to actually make the right call and get data returned. I need to send the following (simplified) data:
- Contact ID
- Contact Name
- General Description
- Amount
There are two functions defined in the WSDL document, but I only need one ("FirstFunction" below). Here is the script I run to get information on the available functions and types:
$client = new SoapClient("http://example.com/webservices?wsdl");
var_dump($client->__getFunctions());
var_dump($client->__getTypes());
And here is the output it generates:
array(
[0] => "FirstFunction Function1(FirstFunction $parameters)",
[1] => "SecondFunction Function2(SecondFunction $parameters)",
);
array(
[0] => struct Contact {
id id;
name name;
}
[1] => string "string description"
[2] => string "int amount"
}
Say I want to make a call to the FirstFunction with the data:
- Contact ID: 100
- Contact Name: John
- General Description: Barrel of Oil
- Amount: 500
What would be the right syntax? I've been trying all sorts of options but it appears the soap structure is quite flexible so there are very many ways of doing this. Couldn't figure it out from the manual either...
UPDATE 1: tried sample from MMK:
$client = new SoapClient("http://example.com/webservices?wsdl");
$params = array(
"id" => 100,
"name" => "John",
"description" => "Barrel of Oil",
"amount" => 500,
);
$response = $client->__soapCall("Function1", array($params));
But I get this response: Object has no 'Contact' property
. As you can see in the output of getTypes()
, there is a struct
called Contact
, so I guess I somehow need to make clear my parameters include the Contact data, but the question is: how?
UPDATE 2: I've also tried these structures, same error.
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500,
);
As well as:
$params = array(
"Contact" => array(
"id" => 100,
"name" => "John",
),
"description" => "Barrel of Oil",
"amount" => 500,
);
Error in both cases: Object has no 'Contact' property`
php soap
add a comment |
up vote
102
down vote
favorite
I'm used to writing PHP code, but do not often use Object-Oriented coding. I now need to interact with SOAP (as a client) and am not able to get the syntax right. I've got a WSDL file which allows me to properly set up a new connection using the SoapClient class. However, I'm unable to actually make the right call and get data returned. I need to send the following (simplified) data:
- Contact ID
- Contact Name
- General Description
- Amount
There are two functions defined in the WSDL document, but I only need one ("FirstFunction" below). Here is the script I run to get information on the available functions and types:
$client = new SoapClient("http://example.com/webservices?wsdl");
var_dump($client->__getFunctions());
var_dump($client->__getTypes());
And here is the output it generates:
array(
[0] => "FirstFunction Function1(FirstFunction $parameters)",
[1] => "SecondFunction Function2(SecondFunction $parameters)",
);
array(
[0] => struct Contact {
id id;
name name;
}
[1] => string "string description"
[2] => string "int amount"
}
Say I want to make a call to the FirstFunction with the data:
- Contact ID: 100
- Contact Name: John
- General Description: Barrel of Oil
- Amount: 500
What would be the right syntax? I've been trying all sorts of options but it appears the soap structure is quite flexible so there are very many ways of doing this. Couldn't figure it out from the manual either...
UPDATE 1: tried sample from MMK:
$client = new SoapClient("http://example.com/webservices?wsdl");
$params = array(
"id" => 100,
"name" => "John",
"description" => "Barrel of Oil",
"amount" => 500,
);
$response = $client->__soapCall("Function1", array($params));
But I get this response: Object has no 'Contact' property
. As you can see in the output of getTypes()
, there is a struct
called Contact
, so I guess I somehow need to make clear my parameters include the Contact data, but the question is: how?
UPDATE 2: I've also tried these structures, same error.
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500,
);
As well as:
$params = array(
"Contact" => array(
"id" => 100,
"name" => "John",
),
"description" => "Barrel of Oil",
"amount" => 500,
);
Error in both cases: Object has no 'Contact' property`
php soap
add a comment |
up vote
102
down vote
favorite
up vote
102
down vote
favorite
I'm used to writing PHP code, but do not often use Object-Oriented coding. I now need to interact with SOAP (as a client) and am not able to get the syntax right. I've got a WSDL file which allows me to properly set up a new connection using the SoapClient class. However, I'm unable to actually make the right call and get data returned. I need to send the following (simplified) data:
- Contact ID
- Contact Name
- General Description
- Amount
There are two functions defined in the WSDL document, but I only need one ("FirstFunction" below). Here is the script I run to get information on the available functions and types:
$client = new SoapClient("http://example.com/webservices?wsdl");
var_dump($client->__getFunctions());
var_dump($client->__getTypes());
And here is the output it generates:
array(
[0] => "FirstFunction Function1(FirstFunction $parameters)",
[1] => "SecondFunction Function2(SecondFunction $parameters)",
);
array(
[0] => struct Contact {
id id;
name name;
}
[1] => string "string description"
[2] => string "int amount"
}
Say I want to make a call to the FirstFunction with the data:
- Contact ID: 100
- Contact Name: John
- General Description: Barrel of Oil
- Amount: 500
What would be the right syntax? I've been trying all sorts of options but it appears the soap structure is quite flexible so there are very many ways of doing this. Couldn't figure it out from the manual either...
UPDATE 1: tried sample from MMK:
$client = new SoapClient("http://example.com/webservices?wsdl");
$params = array(
"id" => 100,
"name" => "John",
"description" => "Barrel of Oil",
"amount" => 500,
);
$response = $client->__soapCall("Function1", array($params));
But I get this response: Object has no 'Contact' property
. As you can see in the output of getTypes()
, there is a struct
called Contact
, so I guess I somehow need to make clear my parameters include the Contact data, but the question is: how?
UPDATE 2: I've also tried these structures, same error.
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500,
);
As well as:
$params = array(
"Contact" => array(
"id" => 100,
"name" => "John",
),
"description" => "Barrel of Oil",
"amount" => 500,
);
Error in both cases: Object has no 'Contact' property`
php soap
I'm used to writing PHP code, but do not often use Object-Oriented coding. I now need to interact with SOAP (as a client) and am not able to get the syntax right. I've got a WSDL file which allows me to properly set up a new connection using the SoapClient class. However, I'm unable to actually make the right call and get data returned. I need to send the following (simplified) data:
- Contact ID
- Contact Name
- General Description
- Amount
There are two functions defined in the WSDL document, but I only need one ("FirstFunction" below). Here is the script I run to get information on the available functions and types:
$client = new SoapClient("http://example.com/webservices?wsdl");
var_dump($client->__getFunctions());
var_dump($client->__getTypes());
And here is the output it generates:
array(
[0] => "FirstFunction Function1(FirstFunction $parameters)",
[1] => "SecondFunction Function2(SecondFunction $parameters)",
);
array(
[0] => struct Contact {
id id;
name name;
}
[1] => string "string description"
[2] => string "int amount"
}
Say I want to make a call to the FirstFunction with the data:
- Contact ID: 100
- Contact Name: John
- General Description: Barrel of Oil
- Amount: 500
What would be the right syntax? I've been trying all sorts of options but it appears the soap structure is quite flexible so there are very many ways of doing this. Couldn't figure it out from the manual either...
UPDATE 1: tried sample from MMK:
$client = new SoapClient("http://example.com/webservices?wsdl");
$params = array(
"id" => 100,
"name" => "John",
"description" => "Barrel of Oil",
"amount" => 500,
);
$response = $client->__soapCall("Function1", array($params));
But I get this response: Object has no 'Contact' property
. As you can see in the output of getTypes()
, there is a struct
called Contact
, so I guess I somehow need to make clear my parameters include the Contact data, but the question is: how?
UPDATE 2: I've also tried these structures, same error.
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500,
);
As well as:
$params = array(
"Contact" => array(
"id" => 100,
"name" => "John",
),
"description" => "Barrel of Oil",
"amount" => 500,
);
Error in both cases: Object has no 'Contact' property`
php soap
php soap
edited Jul 23 '12 at 20:13
asked Jul 21 '12 at 15:52
user1305445
add a comment |
add a comment |
11 Answers
11
active
oldest
votes
up vote
150
down vote
This is what you need to do.
Just to know, I tried to recreate your situation...
- For this example, I made a .NET sample webservice with a
WebMethod
calledFunction1
and these are the parameters:
Function1(Contact Contact, string description, int amount)
In which
Contact
is just a beanclass
that has getters and setters forid
andname
like in your case.You can download this .NET sample webservice at:
https://www.dropbox.com/s/6pz1w94a52o5xah/11593623.zip
The code.
This is what you need to do at PHP side:
(Tested and working)
<?php
/* Create a class for your webservice structure, in this case: Contact */
class Contact {
public function __construct($id, $name)
{
$this->id = $id;
$this->name = $name;
}
}
/* Initialize webservice with your WSDL */
$client = new SoapClient("http://localhost:10139/Service1.asmx?wsdl");
/* Fill your Contact Object */
$contact = new Contact(100, "John");
/* Set your parameters for the request */
$params = array(
"Contact" => $contact,
"description" => "Barrel of Oil",
"amount" => 500,
);
/* Invoke webservice method with your parameters, in this case: Function1 */
$response = $client->__soapCall("Function1", array($params));
/* Print webservice response */
var_dump($response);
?>
How I know this is working?
- If you do
print_r($params);
you will see this output, as your webservice expects:
Array ( [Contact] => Contact Object ( [id] => 100 [name] => John )
[description] => Barrel of Oil [amount] => 500 )
- When I debugged the sample .NET webservice I got this:
(As you can see, Contact
object is not null and also the other parameters, that means your request was successfully done from PHP side).
- The response from .NET sample webservice was the expected one and shown at PHP side:
object(stdClass)[3] public 'Function1Result' => string 'Detailed
information of your request! id: 100, name: John, description: Barrel
of Oil, amount: 500' (length=98)
Hope this helps :-)
2
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
4
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
1
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
4
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
add a comment |
up vote
63
down vote
You can use SOAP services this way too:
<?php
//Create the client object
$soapclient = new SoapClient('http://www.webservicex.net/globalweather.asmx?WSDL');
//Use the functions of the client, the params of the function are in
//the associative array
$params = array('CountryName' => 'Spain', 'CityName' => 'Alicante');
$response = $soapclient->getWeather($params);
var_dump($response);
// Get the Cities By Country
$param = array('CountryName' => 'Spain');
$response = $soapclient->getCitiesByCountry($param);
var_dump($response);
This is an example with a real service, and it works.
Hope this helps.
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
add a comment |
up vote
26
down vote
First initialize webservices:
$client = new SoapClient("http://example.com/webservices?wsdl");
Then set and pass the parameters:
$params = array (
"arg0" => $contactid,
"arg1" => $desc,
"arg2" => $contactname
);
$response = $client->__soapCall('methodname', array($params));
Note that the method name is available in WSDL as operation name, e.g.:
<operation name="methodname">
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
add a comment |
up vote
21
down vote
I don't know why my web service has the same structure with you but it doesn't need Class for parameter, just is array.
For example:
- My WSDL:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns="http://www.kiala.com/schemas/psws/1.0">
<soapenv:Header/>
<soapenv:Body>
<ns:createOrder reference="260778">
<identification>
<sender>5390a7006cee11e0ae3e0800200c9a66</sender>
<hash>831f8c1ad25e1dc89cf2d8f23d2af...fa85155f5c67627</hash>
<originator>VITS-STAELENS</originator>
</identification>
<delivery>
<from country="ES" node=””/>
<to country="ES" node="0299"/>
</delivery>
<parcel>
<description>Zoethout thee</description>
<weight>0.100</weight>
<orderNumber>10K24</orderNumber>
<orderDate>2012-12-31</orderDate>
</parcel>
<receiver>
<firstName>Gladys</firstName>
<surname>Roldan de Moras</surname>
<address>
<line1>Calle General Oraá 26</line1>
<line2>(4º izda)</line2>
<postalCode>28006</postalCode>
<city>Madrid</city>
<country>ES</country>
</address>
<email>gverbruggen@kiala.com</email>
<language>es</language>
</receiver>
</ns:createOrder>
</soapenv:Body>
</soapenv:Envelope>
I var_dump:
var_dump($client->getFunctions());
var_dump($client->getTypes());
Here is result:
array
0 => string 'OrderConfirmation createOrder(OrderRequest $createOrder)' (length=56)
array
0 => string 'struct OrderRequest {
Identification identification;
Delivery delivery;
Parcel parcel;
Receiver receiver;
string reference;
}' (length=130)
1 => string 'struct Identification {
string sender;
string hash;
string originator;
}' (length=75)
2 => string 'struct Delivery {
Node from;
Node to;
}' (length=41)
3 => string 'struct Node {
string country;
string node;
}' (length=46)
4 => string 'struct Parcel {
string description;
decimal weight;
string orderNumber;
date orderDate;
}' (length=93)
5 => string 'struct Receiver {
string firstName;
string surname;
Address address;
string email;
string language;
}' (length=106)
6 => string 'struct Address {
string line1;
string line2;
string postalCode;
string city;
string country;
}' (length=99)
7 => string 'struct OrderConfirmation {
string trackingNumber;
string reference;
}' (length=71)
8 => string 'struct OrderServiceException {
string code;
OrderServiceException faultInfo;
string message;
}' (length=97)
So in my code:
$client = new SoapClient('http://packandship-ws.kiala.com/psws/order?wsdl');
$params = array(
'reference' => $orderId,
'identification' => array(
'sender' => param('kiala', 'sender_id'),
'hash' => hash('sha512', $orderId . param('kiala', 'sender_id') . param('kiala', 'password')),
'originator' => null,
),
'delivery' => array(
'from' => array(
'country' => 'es',
'node' => '',
),
'to' => array(
'country' => 'es',
'node' => '0299'
),
),
'parcel' => array(
'description' => 'Description',
'weight' => 0.200,
'orderNumber' => $orderId,
'orderDate' => date('Y-m-d')
),
'receiver' => array(
'firstName' => 'Customer First Name',
'surname' => 'Customer Sur Name',
'address' => array(
'line1' => 'Line 1 Adress',
'line2' => 'Line 2 Adress',
'postalCode' => 28006,
'city' => 'Madrid',
'country' => 'es',
),
'email' => 'test.ceres@yahoo.com',
'language' => 'es'
)
);
$result = $client->createOrder($params);
var_dump($result);
but it successfully!
1
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
add a comment |
up vote
3
down vote
First, use SoapUI to create your soap project from the wsdl. Try to send a request to play with the wsdl's operations. Observe how the xml request composes your data fields.
And then, if you are having problem getting SoapClient acts as you want, here is how I debug it. Set the option trace so that the function __getLastRequest() is available for use.
$soapClient = new SoapClient('http://yourwdsdlurl.com?wsdl', ['trace' => true]);
$params = ['user' => 'Hey', 'account' => '12345'];
$response = $soapClient->__soapCall('<operation>', $params);
$xml = $soapClient->__getLastRequest();
Then the $xml variable contains the xml that SoapClient compose for your request. Compare this xml with the one generated in the SoapUI.
For me, SoapClient seems to ignore the keys of the associative array $params and interpret it as indexed array, causing wrong parameter data in the xml. That is, if I reorder the data in $params, the $response is completely different:
$params = ['account' => '12345', 'user' => 'Hey'];
$response = $soapClient->__soapCall('<operation>', $params);
add a comment |
up vote
2
down vote
If you create the object of SoapParam, This will resolve your problem. Create a class and map it with object type given by WebService, Initialize the values and send in the request. See the sample below.
struct Contact {
function Contact ($pid, $pname)
{
id = $pid;
name = $pname;
}
}
$struct = new Contact(100,"John");
$soapstruct = new SoapVar($struct, SOAP_ENC_OBJECT, "Contact","http://soapinterop.org/xsd");
$ContactParam = new SoapParam($soapstruct, "Contact")
$response = $client->Function1($ContactParam);
add a comment |
up vote
1
down vote
read this;-
http://php.net/manual/en/soapclient.call.php
Or
This is a good example, for the SOAP function "__call".
However it is deprecated.
<?php
$wsdl = "http://webservices.tekever.eu/ctt/?wsdl";
$int_zona = 5;
$int_peso = 1001;
$cliente = new SoapClient($wsdl);
print "<p>Envio Internacional: ";
$vem = $cliente->__call('CustoEMSInternacional',array($int_zona, $int_peso));
print $vem;
print "</p>";
?>
add a comment |
up vote
0
down vote
You need declare class Contract
class Contract {
public $id;
public $name;
}
$contract = new Contract();
$contract->id = 100;
$contract->name = "John";
$params = array(
"Contact" => $contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
or
$params = array(
$contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
Then
$response = $client->__soapCall("Function1", array("FirstFunction" => $params));
or
$response = $client->__soapCall("Function1", $params);
add a comment |
up vote
0
down vote
You need a multi-dimensional array, you can try the following:
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500
);
in PHP an array is a structure and is very flexible. Normally with soap calls I use an XML wrapper so unsure if it will work.
EDIT:
What you may want to try is creating a json query to send or using that to create a xml buy sort of following what is on this page: http://onwebdev.blogspot.com/2011/08/php-converting-rss-to-json.html
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
add a comment |
up vote
0
down vote
There is an option to generate php5 objects with WsdlInterpreter class. See more here: https://github.com/gkwelding/WSDLInterpreter
for example:
require_once 'WSDLInterpreter-v1.0.0/WSDLInterpreter.php';
$wsdlLocation = '<your wsdl url>?wsdl';
$wsdlInterpreter = new WSDLInterpreter($wsdlLocation);
$wsdlInterpreter->savePHP('.');
add a comment |
up vote
0
down vote
I had the same issue, but I just wrapped the arguments like this and it works now.
$args = array();
$args['Header'] = array(
'CustomerCode' => 'dsadsad',
'Language' => 'fdsfasdf'
);
$args['RequestObject'] = $whatever;
// this was the catch, double array with "Request"
$response = $this->client->__soapCall($name, array(array( 'Request' => $args )));
Using this function:
print_r($this->client->__getLastRequest());
You can see the Request XML whether it's changing or not depending on your arguments.
Use [ trace = 1, exceptions = 0 ] in SoapClient options.
add a comment |
11 Answers
11
active
oldest
votes
11 Answers
11
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
150
down vote
This is what you need to do.
Just to know, I tried to recreate your situation...
- For this example, I made a .NET sample webservice with a
WebMethod
calledFunction1
and these are the parameters:
Function1(Contact Contact, string description, int amount)
In which
Contact
is just a beanclass
that has getters and setters forid
andname
like in your case.You can download this .NET sample webservice at:
https://www.dropbox.com/s/6pz1w94a52o5xah/11593623.zip
The code.
This is what you need to do at PHP side:
(Tested and working)
<?php
/* Create a class for your webservice structure, in this case: Contact */
class Contact {
public function __construct($id, $name)
{
$this->id = $id;
$this->name = $name;
}
}
/* Initialize webservice with your WSDL */
$client = new SoapClient("http://localhost:10139/Service1.asmx?wsdl");
/* Fill your Contact Object */
$contact = new Contact(100, "John");
/* Set your parameters for the request */
$params = array(
"Contact" => $contact,
"description" => "Barrel of Oil",
"amount" => 500,
);
/* Invoke webservice method with your parameters, in this case: Function1 */
$response = $client->__soapCall("Function1", array($params));
/* Print webservice response */
var_dump($response);
?>
How I know this is working?
- If you do
print_r($params);
you will see this output, as your webservice expects:
Array ( [Contact] => Contact Object ( [id] => 100 [name] => John )
[description] => Barrel of Oil [amount] => 500 )
- When I debugged the sample .NET webservice I got this:
(As you can see, Contact
object is not null and also the other parameters, that means your request was successfully done from PHP side).
- The response from .NET sample webservice was the expected one and shown at PHP side:
object(stdClass)[3] public 'Function1Result' => string 'Detailed
information of your request! id: 100, name: John, description: Barrel
of Oil, amount: 500' (length=98)
Hope this helps :-)
2
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
4
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
1
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
4
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
add a comment |
up vote
150
down vote
This is what you need to do.
Just to know, I tried to recreate your situation...
- For this example, I made a .NET sample webservice with a
WebMethod
calledFunction1
and these are the parameters:
Function1(Contact Contact, string description, int amount)
In which
Contact
is just a beanclass
that has getters and setters forid
andname
like in your case.You can download this .NET sample webservice at:
https://www.dropbox.com/s/6pz1w94a52o5xah/11593623.zip
The code.
This is what you need to do at PHP side:
(Tested and working)
<?php
/* Create a class for your webservice structure, in this case: Contact */
class Contact {
public function __construct($id, $name)
{
$this->id = $id;
$this->name = $name;
}
}
/* Initialize webservice with your WSDL */
$client = new SoapClient("http://localhost:10139/Service1.asmx?wsdl");
/* Fill your Contact Object */
$contact = new Contact(100, "John");
/* Set your parameters for the request */
$params = array(
"Contact" => $contact,
"description" => "Barrel of Oil",
"amount" => 500,
);
/* Invoke webservice method with your parameters, in this case: Function1 */
$response = $client->__soapCall("Function1", array($params));
/* Print webservice response */
var_dump($response);
?>
How I know this is working?
- If you do
print_r($params);
you will see this output, as your webservice expects:
Array ( [Contact] => Contact Object ( [id] => 100 [name] => John )
[description] => Barrel of Oil [amount] => 500 )
- When I debugged the sample .NET webservice I got this:
(As you can see, Contact
object is not null and also the other parameters, that means your request was successfully done from PHP side).
- The response from .NET sample webservice was the expected one and shown at PHP side:
object(stdClass)[3] public 'Function1Result' => string 'Detailed
information of your request! id: 100, name: John, description: Barrel
of Oil, amount: 500' (length=98)
Hope this helps :-)
2
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
4
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
1
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
4
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
add a comment |
up vote
150
down vote
up vote
150
down vote
This is what you need to do.
Just to know, I tried to recreate your situation...
- For this example, I made a .NET sample webservice with a
WebMethod
calledFunction1
and these are the parameters:
Function1(Contact Contact, string description, int amount)
In which
Contact
is just a beanclass
that has getters and setters forid
andname
like in your case.You can download this .NET sample webservice at:
https://www.dropbox.com/s/6pz1w94a52o5xah/11593623.zip
The code.
This is what you need to do at PHP side:
(Tested and working)
<?php
/* Create a class for your webservice structure, in this case: Contact */
class Contact {
public function __construct($id, $name)
{
$this->id = $id;
$this->name = $name;
}
}
/* Initialize webservice with your WSDL */
$client = new SoapClient("http://localhost:10139/Service1.asmx?wsdl");
/* Fill your Contact Object */
$contact = new Contact(100, "John");
/* Set your parameters for the request */
$params = array(
"Contact" => $contact,
"description" => "Barrel of Oil",
"amount" => 500,
);
/* Invoke webservice method with your parameters, in this case: Function1 */
$response = $client->__soapCall("Function1", array($params));
/* Print webservice response */
var_dump($response);
?>
How I know this is working?
- If you do
print_r($params);
you will see this output, as your webservice expects:
Array ( [Contact] => Contact Object ( [id] => 100 [name] => John )
[description] => Barrel of Oil [amount] => 500 )
- When I debugged the sample .NET webservice I got this:
(As you can see, Contact
object is not null and also the other parameters, that means your request was successfully done from PHP side).
- The response from .NET sample webservice was the expected one and shown at PHP side:
object(stdClass)[3] public 'Function1Result' => string 'Detailed
information of your request! id: 100, name: John, description: Barrel
of Oil, amount: 500' (length=98)
Hope this helps :-)
This is what you need to do.
Just to know, I tried to recreate your situation...
- For this example, I made a .NET sample webservice with a
WebMethod
calledFunction1
and these are the parameters:
Function1(Contact Contact, string description, int amount)
In which
Contact
is just a beanclass
that has getters and setters forid
andname
like in your case.You can download this .NET sample webservice at:
https://www.dropbox.com/s/6pz1w94a52o5xah/11593623.zip
The code.
This is what you need to do at PHP side:
(Tested and working)
<?php
/* Create a class for your webservice structure, in this case: Contact */
class Contact {
public function __construct($id, $name)
{
$this->id = $id;
$this->name = $name;
}
}
/* Initialize webservice with your WSDL */
$client = new SoapClient("http://localhost:10139/Service1.asmx?wsdl");
/* Fill your Contact Object */
$contact = new Contact(100, "John");
/* Set your parameters for the request */
$params = array(
"Contact" => $contact,
"description" => "Barrel of Oil",
"amount" => 500,
);
/* Invoke webservice method with your parameters, in this case: Function1 */
$response = $client->__soapCall("Function1", array($params));
/* Print webservice response */
var_dump($response);
?>
How I know this is working?
- If you do
print_r($params);
you will see this output, as your webservice expects:
Array ( [Contact] => Contact Object ( [id] => 100 [name] => John )
[description] => Barrel of Oil [amount] => 500 )
- When I debugged the sample .NET webservice I got this:
(As you can see, Contact
object is not null and also the other parameters, that means your request was successfully done from PHP side).
- The response from .NET sample webservice was the expected one and shown at PHP side:
object(stdClass)[3] public 'Function1Result' => string 'Detailed
information of your request! id: 100, name: John, description: Barrel
of Oil, amount: 500' (length=98)
Hope this helps :-)
edited Nov 21 at 11:30


Sebastian Viereck
2,2782229
2,2782229
answered Jul 30 '12 at 1:37
Oscar Jara
10.8k104887
10.8k104887
2
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
4
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
1
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
4
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
add a comment |
2
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
4
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
1
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
4
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
2
2
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
Perfect! I acted like I knew a little bit more about SOAP services than I really did and this got me where I needed to be.
– chapman84
Mar 27 '13 at 13:52
4
4
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
@chapman84 you never accepted his answer.
– taco
Aug 26 '13 at 15:28
1
1
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
I didn't ask the question, otherwise I would have. The question and this answer got an upvote from me though.
– chapman84
Aug 26 '13 at 16:14
4
4
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
@user should accept it :) BTW, very nice answer, complete and very clear. +1
– Yann39
Aug 29 '13 at 13:37
add a comment |
up vote
63
down vote
You can use SOAP services this way too:
<?php
//Create the client object
$soapclient = new SoapClient('http://www.webservicex.net/globalweather.asmx?WSDL');
//Use the functions of the client, the params of the function are in
//the associative array
$params = array('CountryName' => 'Spain', 'CityName' => 'Alicante');
$response = $soapclient->getWeather($params);
var_dump($response);
// Get the Cities By Country
$param = array('CountryName' => 'Spain');
$response = $soapclient->getCitiesByCountry($param);
var_dump($response);
This is an example with a real service, and it works.
Hope this helps.
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
add a comment |
up vote
63
down vote
You can use SOAP services this way too:
<?php
//Create the client object
$soapclient = new SoapClient('http://www.webservicex.net/globalweather.asmx?WSDL');
//Use the functions of the client, the params of the function are in
//the associative array
$params = array('CountryName' => 'Spain', 'CityName' => 'Alicante');
$response = $soapclient->getWeather($params);
var_dump($response);
// Get the Cities By Country
$param = array('CountryName' => 'Spain');
$response = $soapclient->getCitiesByCountry($param);
var_dump($response);
This is an example with a real service, and it works.
Hope this helps.
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
add a comment |
up vote
63
down vote
up vote
63
down vote
You can use SOAP services this way too:
<?php
//Create the client object
$soapclient = new SoapClient('http://www.webservicex.net/globalweather.asmx?WSDL');
//Use the functions of the client, the params of the function are in
//the associative array
$params = array('CountryName' => 'Spain', 'CityName' => 'Alicante');
$response = $soapclient->getWeather($params);
var_dump($response);
// Get the Cities By Country
$param = array('CountryName' => 'Spain');
$response = $soapclient->getCitiesByCountry($param);
var_dump($response);
This is an example with a real service, and it works.
Hope this helps.
You can use SOAP services this way too:
<?php
//Create the client object
$soapclient = new SoapClient('http://www.webservicex.net/globalweather.asmx?WSDL');
//Use the functions of the client, the params of the function are in
//the associative array
$params = array('CountryName' => 'Spain', 'CityName' => 'Alicante');
$response = $soapclient->getWeather($params);
var_dump($response);
// Get the Cities By Country
$param = array('CountryName' => 'Spain');
$response = $soapclient->getCitiesByCountry($param);
var_dump($response);
This is an example with a real service, and it works.
Hope this helps.
edited Jun 14 '17 at 13:17
answered Jun 12 '13 at 14:00
Salvador P.
1,1441213
1,1441213
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
add a comment |
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
I get the following error : object(stdClass)#70 (1) { ["GetWeatherResult"]=> string(14) "Data Not Found" } Any idea?
– Ilker Baltaci
Jun 14 '17 at 12:57
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
Seems they have changed the strings of the cities. I've just updated the example with another call to another service they provide and it's working. I tried to use the strings they return as cities, but does not seem to work fine, anyways the getCitiesByCountry() function serves as example on how to make a call.
– Salvador P.
Jun 14 '17 at 13:18
add a comment |
up vote
26
down vote
First initialize webservices:
$client = new SoapClient("http://example.com/webservices?wsdl");
Then set and pass the parameters:
$params = array (
"arg0" => $contactid,
"arg1" => $desc,
"arg2" => $contactname
);
$response = $client->__soapCall('methodname', array($params));
Note that the method name is available in WSDL as operation name, e.g.:
<operation name="methodname">
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
add a comment |
up vote
26
down vote
First initialize webservices:
$client = new SoapClient("http://example.com/webservices?wsdl");
Then set and pass the parameters:
$params = array (
"arg0" => $contactid,
"arg1" => $desc,
"arg2" => $contactname
);
$response = $client->__soapCall('methodname', array($params));
Note that the method name is available in WSDL as operation name, e.g.:
<operation name="methodname">
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
add a comment |
up vote
26
down vote
up vote
26
down vote
First initialize webservices:
$client = new SoapClient("http://example.com/webservices?wsdl");
Then set and pass the parameters:
$params = array (
"arg0" => $contactid,
"arg1" => $desc,
"arg2" => $contactname
);
$response = $client->__soapCall('methodname', array($params));
Note that the method name is available in WSDL as operation name, e.g.:
<operation name="methodname">
First initialize webservices:
$client = new SoapClient("http://example.com/webservices?wsdl");
Then set and pass the parameters:
$params = array (
"arg0" => $contactid,
"arg1" => $desc,
"arg2" => $contactname
);
$response = $client->__soapCall('methodname', array($params));
Note that the method name is available in WSDL as operation name, e.g.:
<operation name="methodname">
edited Jun 21 '16 at 15:05
dotancohen
14.5k1896147
14.5k1896147
answered Jul 21 '12 at 16:18
MMK
969719
969719
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
add a comment |
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
Thanks! I've tried this but I get the error "Object has no 'Contact' property". Will update my question with full details. Any ideas?
– user1305445
Jul 21 '12 at 17:29
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
@user16441 Can you post the WSDL and schema of the service? I usually start by figuring out what XML the service expects, then using WireShark to figure out what my client is actually sending.
– davidfmatheson
Jul 30 '12 at 13:51
add a comment |
up vote
21
down vote
I don't know why my web service has the same structure with you but it doesn't need Class for parameter, just is array.
For example:
- My WSDL:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns="http://www.kiala.com/schemas/psws/1.0">
<soapenv:Header/>
<soapenv:Body>
<ns:createOrder reference="260778">
<identification>
<sender>5390a7006cee11e0ae3e0800200c9a66</sender>
<hash>831f8c1ad25e1dc89cf2d8f23d2af...fa85155f5c67627</hash>
<originator>VITS-STAELENS</originator>
</identification>
<delivery>
<from country="ES" node=””/>
<to country="ES" node="0299"/>
</delivery>
<parcel>
<description>Zoethout thee</description>
<weight>0.100</weight>
<orderNumber>10K24</orderNumber>
<orderDate>2012-12-31</orderDate>
</parcel>
<receiver>
<firstName>Gladys</firstName>
<surname>Roldan de Moras</surname>
<address>
<line1>Calle General Oraá 26</line1>
<line2>(4º izda)</line2>
<postalCode>28006</postalCode>
<city>Madrid</city>
<country>ES</country>
</address>
<email>gverbruggen@kiala.com</email>
<language>es</language>
</receiver>
</ns:createOrder>
</soapenv:Body>
</soapenv:Envelope>
I var_dump:
var_dump($client->getFunctions());
var_dump($client->getTypes());
Here is result:
array
0 => string 'OrderConfirmation createOrder(OrderRequest $createOrder)' (length=56)
array
0 => string 'struct OrderRequest {
Identification identification;
Delivery delivery;
Parcel parcel;
Receiver receiver;
string reference;
}' (length=130)
1 => string 'struct Identification {
string sender;
string hash;
string originator;
}' (length=75)
2 => string 'struct Delivery {
Node from;
Node to;
}' (length=41)
3 => string 'struct Node {
string country;
string node;
}' (length=46)
4 => string 'struct Parcel {
string description;
decimal weight;
string orderNumber;
date orderDate;
}' (length=93)
5 => string 'struct Receiver {
string firstName;
string surname;
Address address;
string email;
string language;
}' (length=106)
6 => string 'struct Address {
string line1;
string line2;
string postalCode;
string city;
string country;
}' (length=99)
7 => string 'struct OrderConfirmation {
string trackingNumber;
string reference;
}' (length=71)
8 => string 'struct OrderServiceException {
string code;
OrderServiceException faultInfo;
string message;
}' (length=97)
So in my code:
$client = new SoapClient('http://packandship-ws.kiala.com/psws/order?wsdl');
$params = array(
'reference' => $orderId,
'identification' => array(
'sender' => param('kiala', 'sender_id'),
'hash' => hash('sha512', $orderId . param('kiala', 'sender_id') . param('kiala', 'password')),
'originator' => null,
),
'delivery' => array(
'from' => array(
'country' => 'es',
'node' => '',
),
'to' => array(
'country' => 'es',
'node' => '0299'
),
),
'parcel' => array(
'description' => 'Description',
'weight' => 0.200,
'orderNumber' => $orderId,
'orderDate' => date('Y-m-d')
),
'receiver' => array(
'firstName' => 'Customer First Name',
'surname' => 'Customer Sur Name',
'address' => array(
'line1' => 'Line 1 Adress',
'line2' => 'Line 2 Adress',
'postalCode' => 28006,
'city' => 'Madrid',
'country' => 'es',
),
'email' => 'test.ceres@yahoo.com',
'language' => 'es'
)
);
$result = $client->createOrder($params);
var_dump($result);
but it successfully!
1
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
add a comment |
up vote
21
down vote
I don't know why my web service has the same structure with you but it doesn't need Class for parameter, just is array.
For example:
- My WSDL:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns="http://www.kiala.com/schemas/psws/1.0">
<soapenv:Header/>
<soapenv:Body>
<ns:createOrder reference="260778">
<identification>
<sender>5390a7006cee11e0ae3e0800200c9a66</sender>
<hash>831f8c1ad25e1dc89cf2d8f23d2af...fa85155f5c67627</hash>
<originator>VITS-STAELENS</originator>
</identification>
<delivery>
<from country="ES" node=””/>
<to country="ES" node="0299"/>
</delivery>
<parcel>
<description>Zoethout thee</description>
<weight>0.100</weight>
<orderNumber>10K24</orderNumber>
<orderDate>2012-12-31</orderDate>
</parcel>
<receiver>
<firstName>Gladys</firstName>
<surname>Roldan de Moras</surname>
<address>
<line1>Calle General Oraá 26</line1>
<line2>(4º izda)</line2>
<postalCode>28006</postalCode>
<city>Madrid</city>
<country>ES</country>
</address>
<email>gverbruggen@kiala.com</email>
<language>es</language>
</receiver>
</ns:createOrder>
</soapenv:Body>
</soapenv:Envelope>
I var_dump:
var_dump($client->getFunctions());
var_dump($client->getTypes());
Here is result:
array
0 => string 'OrderConfirmation createOrder(OrderRequest $createOrder)' (length=56)
array
0 => string 'struct OrderRequest {
Identification identification;
Delivery delivery;
Parcel parcel;
Receiver receiver;
string reference;
}' (length=130)
1 => string 'struct Identification {
string sender;
string hash;
string originator;
}' (length=75)
2 => string 'struct Delivery {
Node from;
Node to;
}' (length=41)
3 => string 'struct Node {
string country;
string node;
}' (length=46)
4 => string 'struct Parcel {
string description;
decimal weight;
string orderNumber;
date orderDate;
}' (length=93)
5 => string 'struct Receiver {
string firstName;
string surname;
Address address;
string email;
string language;
}' (length=106)
6 => string 'struct Address {
string line1;
string line2;
string postalCode;
string city;
string country;
}' (length=99)
7 => string 'struct OrderConfirmation {
string trackingNumber;
string reference;
}' (length=71)
8 => string 'struct OrderServiceException {
string code;
OrderServiceException faultInfo;
string message;
}' (length=97)
So in my code:
$client = new SoapClient('http://packandship-ws.kiala.com/psws/order?wsdl');
$params = array(
'reference' => $orderId,
'identification' => array(
'sender' => param('kiala', 'sender_id'),
'hash' => hash('sha512', $orderId . param('kiala', 'sender_id') . param('kiala', 'password')),
'originator' => null,
),
'delivery' => array(
'from' => array(
'country' => 'es',
'node' => '',
),
'to' => array(
'country' => 'es',
'node' => '0299'
),
),
'parcel' => array(
'description' => 'Description',
'weight' => 0.200,
'orderNumber' => $orderId,
'orderDate' => date('Y-m-d')
),
'receiver' => array(
'firstName' => 'Customer First Name',
'surname' => 'Customer Sur Name',
'address' => array(
'line1' => 'Line 1 Adress',
'line2' => 'Line 2 Adress',
'postalCode' => 28006,
'city' => 'Madrid',
'country' => 'es',
),
'email' => 'test.ceres@yahoo.com',
'language' => 'es'
)
);
$result = $client->createOrder($params);
var_dump($result);
but it successfully!
1
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
add a comment |
up vote
21
down vote
up vote
21
down vote
I don't know why my web service has the same structure with you but it doesn't need Class for parameter, just is array.
For example:
- My WSDL:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns="http://www.kiala.com/schemas/psws/1.0">
<soapenv:Header/>
<soapenv:Body>
<ns:createOrder reference="260778">
<identification>
<sender>5390a7006cee11e0ae3e0800200c9a66</sender>
<hash>831f8c1ad25e1dc89cf2d8f23d2af...fa85155f5c67627</hash>
<originator>VITS-STAELENS</originator>
</identification>
<delivery>
<from country="ES" node=””/>
<to country="ES" node="0299"/>
</delivery>
<parcel>
<description>Zoethout thee</description>
<weight>0.100</weight>
<orderNumber>10K24</orderNumber>
<orderDate>2012-12-31</orderDate>
</parcel>
<receiver>
<firstName>Gladys</firstName>
<surname>Roldan de Moras</surname>
<address>
<line1>Calle General Oraá 26</line1>
<line2>(4º izda)</line2>
<postalCode>28006</postalCode>
<city>Madrid</city>
<country>ES</country>
</address>
<email>gverbruggen@kiala.com</email>
<language>es</language>
</receiver>
</ns:createOrder>
</soapenv:Body>
</soapenv:Envelope>
I var_dump:
var_dump($client->getFunctions());
var_dump($client->getTypes());
Here is result:
array
0 => string 'OrderConfirmation createOrder(OrderRequest $createOrder)' (length=56)
array
0 => string 'struct OrderRequest {
Identification identification;
Delivery delivery;
Parcel parcel;
Receiver receiver;
string reference;
}' (length=130)
1 => string 'struct Identification {
string sender;
string hash;
string originator;
}' (length=75)
2 => string 'struct Delivery {
Node from;
Node to;
}' (length=41)
3 => string 'struct Node {
string country;
string node;
}' (length=46)
4 => string 'struct Parcel {
string description;
decimal weight;
string orderNumber;
date orderDate;
}' (length=93)
5 => string 'struct Receiver {
string firstName;
string surname;
Address address;
string email;
string language;
}' (length=106)
6 => string 'struct Address {
string line1;
string line2;
string postalCode;
string city;
string country;
}' (length=99)
7 => string 'struct OrderConfirmation {
string trackingNumber;
string reference;
}' (length=71)
8 => string 'struct OrderServiceException {
string code;
OrderServiceException faultInfo;
string message;
}' (length=97)
So in my code:
$client = new SoapClient('http://packandship-ws.kiala.com/psws/order?wsdl');
$params = array(
'reference' => $orderId,
'identification' => array(
'sender' => param('kiala', 'sender_id'),
'hash' => hash('sha512', $orderId . param('kiala', 'sender_id') . param('kiala', 'password')),
'originator' => null,
),
'delivery' => array(
'from' => array(
'country' => 'es',
'node' => '',
),
'to' => array(
'country' => 'es',
'node' => '0299'
),
),
'parcel' => array(
'description' => 'Description',
'weight' => 0.200,
'orderNumber' => $orderId,
'orderDate' => date('Y-m-d')
),
'receiver' => array(
'firstName' => 'Customer First Name',
'surname' => 'Customer Sur Name',
'address' => array(
'line1' => 'Line 1 Adress',
'line2' => 'Line 2 Adress',
'postalCode' => 28006,
'city' => 'Madrid',
'country' => 'es',
),
'email' => 'test.ceres@yahoo.com',
'language' => 'es'
)
);
$result = $client->createOrder($params);
var_dump($result);
but it successfully!
I don't know why my web service has the same structure with you but it doesn't need Class for parameter, just is array.
For example:
- My WSDL:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns="http://www.kiala.com/schemas/psws/1.0">
<soapenv:Header/>
<soapenv:Body>
<ns:createOrder reference="260778">
<identification>
<sender>5390a7006cee11e0ae3e0800200c9a66</sender>
<hash>831f8c1ad25e1dc89cf2d8f23d2af...fa85155f5c67627</hash>
<originator>VITS-STAELENS</originator>
</identification>
<delivery>
<from country="ES" node=””/>
<to country="ES" node="0299"/>
</delivery>
<parcel>
<description>Zoethout thee</description>
<weight>0.100</weight>
<orderNumber>10K24</orderNumber>
<orderDate>2012-12-31</orderDate>
</parcel>
<receiver>
<firstName>Gladys</firstName>
<surname>Roldan de Moras</surname>
<address>
<line1>Calle General Oraá 26</line1>
<line2>(4º izda)</line2>
<postalCode>28006</postalCode>
<city>Madrid</city>
<country>ES</country>
</address>
<email>gverbruggen@kiala.com</email>
<language>es</language>
</receiver>
</ns:createOrder>
</soapenv:Body>
</soapenv:Envelope>
I var_dump:
var_dump($client->getFunctions());
var_dump($client->getTypes());
Here is result:
array
0 => string 'OrderConfirmation createOrder(OrderRequest $createOrder)' (length=56)
array
0 => string 'struct OrderRequest {
Identification identification;
Delivery delivery;
Parcel parcel;
Receiver receiver;
string reference;
}' (length=130)
1 => string 'struct Identification {
string sender;
string hash;
string originator;
}' (length=75)
2 => string 'struct Delivery {
Node from;
Node to;
}' (length=41)
3 => string 'struct Node {
string country;
string node;
}' (length=46)
4 => string 'struct Parcel {
string description;
decimal weight;
string orderNumber;
date orderDate;
}' (length=93)
5 => string 'struct Receiver {
string firstName;
string surname;
Address address;
string email;
string language;
}' (length=106)
6 => string 'struct Address {
string line1;
string line2;
string postalCode;
string city;
string country;
}' (length=99)
7 => string 'struct OrderConfirmation {
string trackingNumber;
string reference;
}' (length=71)
8 => string 'struct OrderServiceException {
string code;
OrderServiceException faultInfo;
string message;
}' (length=97)
So in my code:
$client = new SoapClient('http://packandship-ws.kiala.com/psws/order?wsdl');
$params = array(
'reference' => $orderId,
'identification' => array(
'sender' => param('kiala', 'sender_id'),
'hash' => hash('sha512', $orderId . param('kiala', 'sender_id') . param('kiala', 'password')),
'originator' => null,
),
'delivery' => array(
'from' => array(
'country' => 'es',
'node' => '',
),
'to' => array(
'country' => 'es',
'node' => '0299'
),
),
'parcel' => array(
'description' => 'Description',
'weight' => 0.200,
'orderNumber' => $orderId,
'orderDate' => date('Y-m-d')
),
'receiver' => array(
'firstName' => 'Customer First Name',
'surname' => 'Customer Sur Name',
'address' => array(
'line1' => 'Line 1 Adress',
'line2' => 'Line 2 Adress',
'postalCode' => 28006,
'city' => 'Madrid',
'country' => 'es',
),
'email' => 'test.ceres@yahoo.com',
'language' => 'es'
)
);
$result = $client->createOrder($params);
var_dump($result);
but it successfully!
answered Jul 17 '13 at 3:17


Tín Phạm
436414
436414
1
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
add a comment |
1
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
1
1
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
Your example is more helpfull, cause it shows structure dependecies
– vladkras
Jun 10 '16 at 10:07
add a comment |
up vote
3
down vote
First, use SoapUI to create your soap project from the wsdl. Try to send a request to play with the wsdl's operations. Observe how the xml request composes your data fields.
And then, if you are having problem getting SoapClient acts as you want, here is how I debug it. Set the option trace so that the function __getLastRequest() is available for use.
$soapClient = new SoapClient('http://yourwdsdlurl.com?wsdl', ['trace' => true]);
$params = ['user' => 'Hey', 'account' => '12345'];
$response = $soapClient->__soapCall('<operation>', $params);
$xml = $soapClient->__getLastRequest();
Then the $xml variable contains the xml that SoapClient compose for your request. Compare this xml with the one generated in the SoapUI.
For me, SoapClient seems to ignore the keys of the associative array $params and interpret it as indexed array, causing wrong parameter data in the xml. That is, if I reorder the data in $params, the $response is completely different:
$params = ['account' => '12345', 'user' => 'Hey'];
$response = $soapClient->__soapCall('<operation>', $params);
add a comment |
up vote
3
down vote
First, use SoapUI to create your soap project from the wsdl. Try to send a request to play with the wsdl's operations. Observe how the xml request composes your data fields.
And then, if you are having problem getting SoapClient acts as you want, here is how I debug it. Set the option trace so that the function __getLastRequest() is available for use.
$soapClient = new SoapClient('http://yourwdsdlurl.com?wsdl', ['trace' => true]);
$params = ['user' => 'Hey', 'account' => '12345'];
$response = $soapClient->__soapCall('<operation>', $params);
$xml = $soapClient->__getLastRequest();
Then the $xml variable contains the xml that SoapClient compose for your request. Compare this xml with the one generated in the SoapUI.
For me, SoapClient seems to ignore the keys of the associative array $params and interpret it as indexed array, causing wrong parameter data in the xml. That is, if I reorder the data in $params, the $response is completely different:
$params = ['account' => '12345', 'user' => 'Hey'];
$response = $soapClient->__soapCall('<operation>', $params);
add a comment |
up vote
3
down vote
up vote
3
down vote
First, use SoapUI to create your soap project from the wsdl. Try to send a request to play with the wsdl's operations. Observe how the xml request composes your data fields.
And then, if you are having problem getting SoapClient acts as you want, here is how I debug it. Set the option trace so that the function __getLastRequest() is available for use.
$soapClient = new SoapClient('http://yourwdsdlurl.com?wsdl', ['trace' => true]);
$params = ['user' => 'Hey', 'account' => '12345'];
$response = $soapClient->__soapCall('<operation>', $params);
$xml = $soapClient->__getLastRequest();
Then the $xml variable contains the xml that SoapClient compose for your request. Compare this xml with the one generated in the SoapUI.
For me, SoapClient seems to ignore the keys of the associative array $params and interpret it as indexed array, causing wrong parameter data in the xml. That is, if I reorder the data in $params, the $response is completely different:
$params = ['account' => '12345', 'user' => 'Hey'];
$response = $soapClient->__soapCall('<operation>', $params);
First, use SoapUI to create your soap project from the wsdl. Try to send a request to play with the wsdl's operations. Observe how the xml request composes your data fields.
And then, if you are having problem getting SoapClient acts as you want, here is how I debug it. Set the option trace so that the function __getLastRequest() is available for use.
$soapClient = new SoapClient('http://yourwdsdlurl.com?wsdl', ['trace' => true]);
$params = ['user' => 'Hey', 'account' => '12345'];
$response = $soapClient->__soapCall('<operation>', $params);
$xml = $soapClient->__getLastRequest();
Then the $xml variable contains the xml that SoapClient compose for your request. Compare this xml with the one generated in the SoapUI.
For me, SoapClient seems to ignore the keys of the associative array $params and interpret it as indexed array, causing wrong parameter data in the xml. That is, if I reorder the data in $params, the $response is completely different:
$params = ['account' => '12345', 'user' => 'Hey'];
$response = $soapClient->__soapCall('<operation>', $params);
answered Jan 26 at 4:25
Sang Nguyen
412
412
add a comment |
add a comment |
up vote
2
down vote
If you create the object of SoapParam, This will resolve your problem. Create a class and map it with object type given by WebService, Initialize the values and send in the request. See the sample below.
struct Contact {
function Contact ($pid, $pname)
{
id = $pid;
name = $pname;
}
}
$struct = new Contact(100,"John");
$soapstruct = new SoapVar($struct, SOAP_ENC_OBJECT, "Contact","http://soapinterop.org/xsd");
$ContactParam = new SoapParam($soapstruct, "Contact")
$response = $client->Function1($ContactParam);
add a comment |
up vote
2
down vote
If you create the object of SoapParam, This will resolve your problem. Create a class and map it with object type given by WebService, Initialize the values and send in the request. See the sample below.
struct Contact {
function Contact ($pid, $pname)
{
id = $pid;
name = $pname;
}
}
$struct = new Contact(100,"John");
$soapstruct = new SoapVar($struct, SOAP_ENC_OBJECT, "Contact","http://soapinterop.org/xsd");
$ContactParam = new SoapParam($soapstruct, "Contact")
$response = $client->Function1($ContactParam);
add a comment |
up vote
2
down vote
up vote
2
down vote
If you create the object of SoapParam, This will resolve your problem. Create a class and map it with object type given by WebService, Initialize the values and send in the request. See the sample below.
struct Contact {
function Contact ($pid, $pname)
{
id = $pid;
name = $pname;
}
}
$struct = new Contact(100,"John");
$soapstruct = new SoapVar($struct, SOAP_ENC_OBJECT, "Contact","http://soapinterop.org/xsd");
$ContactParam = new SoapParam($soapstruct, "Contact")
$response = $client->Function1($ContactParam);
If you create the object of SoapParam, This will resolve your problem. Create a class and map it with object type given by WebService, Initialize the values and send in the request. See the sample below.
struct Contact {
function Contact ($pid, $pname)
{
id = $pid;
name = $pname;
}
}
$struct = new Contact(100,"John");
$soapstruct = new SoapVar($struct, SOAP_ENC_OBJECT, "Contact","http://soapinterop.org/xsd");
$ContactParam = new SoapParam($soapstruct, "Contact")
$response = $client->Function1($ContactParam);
edited Mar 3 at 0:20
abullock
13
13
answered Jul 25 '12 at 14:19
Umesh Chavan
566211
566211
add a comment |
add a comment |
up vote
1
down vote
read this;-
http://php.net/manual/en/soapclient.call.php
Or
This is a good example, for the SOAP function "__call".
However it is deprecated.
<?php
$wsdl = "http://webservices.tekever.eu/ctt/?wsdl";
$int_zona = 5;
$int_peso = 1001;
$cliente = new SoapClient($wsdl);
print "<p>Envio Internacional: ";
$vem = $cliente->__call('CustoEMSInternacional',array($int_zona, $int_peso));
print $vem;
print "</p>";
?>
add a comment |
up vote
1
down vote
read this;-
http://php.net/manual/en/soapclient.call.php
Or
This is a good example, for the SOAP function "__call".
However it is deprecated.
<?php
$wsdl = "http://webservices.tekever.eu/ctt/?wsdl";
$int_zona = 5;
$int_peso = 1001;
$cliente = new SoapClient($wsdl);
print "<p>Envio Internacional: ";
$vem = $cliente->__call('CustoEMSInternacional',array($int_zona, $int_peso));
print $vem;
print "</p>";
?>
add a comment |
up vote
1
down vote
up vote
1
down vote
read this;-
http://php.net/manual/en/soapclient.call.php
Or
This is a good example, for the SOAP function "__call".
However it is deprecated.
<?php
$wsdl = "http://webservices.tekever.eu/ctt/?wsdl";
$int_zona = 5;
$int_peso = 1001;
$cliente = new SoapClient($wsdl);
print "<p>Envio Internacional: ";
$vem = $cliente->__call('CustoEMSInternacional',array($int_zona, $int_peso));
print $vem;
print "</p>";
?>
read this;-
http://php.net/manual/en/soapclient.call.php
Or
This is a good example, for the SOAP function "__call".
However it is deprecated.
<?php
$wsdl = "http://webservices.tekever.eu/ctt/?wsdl";
$int_zona = 5;
$int_peso = 1001;
$cliente = new SoapClient($wsdl);
print "<p>Envio Internacional: ";
$vem = $cliente->__call('CustoEMSInternacional',array($int_zona, $int_peso));
print $vem;
print "</p>";
?>
answered Jul 29 '12 at 19:52
Abid Hussain
6,65822443
6,65822443
add a comment |
add a comment |
up vote
0
down vote
You need declare class Contract
class Contract {
public $id;
public $name;
}
$contract = new Contract();
$contract->id = 100;
$contract->name = "John";
$params = array(
"Contact" => $contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
or
$params = array(
$contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
Then
$response = $client->__soapCall("Function1", array("FirstFunction" => $params));
or
$response = $client->__soapCall("Function1", $params);
add a comment |
up vote
0
down vote
You need declare class Contract
class Contract {
public $id;
public $name;
}
$contract = new Contract();
$contract->id = 100;
$contract->name = "John";
$params = array(
"Contact" => $contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
or
$params = array(
$contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
Then
$response = $client->__soapCall("Function1", array("FirstFunction" => $params));
or
$response = $client->__soapCall("Function1", $params);
add a comment |
up vote
0
down vote
up vote
0
down vote
You need declare class Contract
class Contract {
public $id;
public $name;
}
$contract = new Contract();
$contract->id = 100;
$contract->name = "John";
$params = array(
"Contact" => $contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
or
$params = array(
$contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
Then
$response = $client->__soapCall("Function1", array("FirstFunction" => $params));
or
$response = $client->__soapCall("Function1", $params);
You need declare class Contract
class Contract {
public $id;
public $name;
}
$contract = new Contract();
$contract->id = 100;
$contract->name = "John";
$params = array(
"Contact" => $contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
or
$params = array(
$contract,
"description" => "Barrel of Oil",
"amount" => 500,
);
Then
$response = $client->__soapCall("Function1", array("FirstFunction" => $params));
or
$response = $client->__soapCall("Function1", $params);
answered Jul 29 '12 at 23:17
Ramil Amr
1,171824
1,171824
add a comment |
add a comment |
up vote
0
down vote
You need a multi-dimensional array, you can try the following:
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500
);
in PHP an array is a structure and is very flexible. Normally with soap calls I use an XML wrapper so unsure if it will work.
EDIT:
What you may want to try is creating a json query to send or using that to create a xml buy sort of following what is on this page: http://onwebdev.blogspot.com/2011/08/php-converting-rss-to-json.html
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
add a comment |
up vote
0
down vote
You need a multi-dimensional array, you can try the following:
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500
);
in PHP an array is a structure and is very flexible. Normally with soap calls I use an XML wrapper so unsure if it will work.
EDIT:
What you may want to try is creating a json query to send or using that to create a xml buy sort of following what is on this page: http://onwebdev.blogspot.com/2011/08/php-converting-rss-to-json.html
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
add a comment |
up vote
0
down vote
up vote
0
down vote
You need a multi-dimensional array, you can try the following:
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500
);
in PHP an array is a structure and is very flexible. Normally with soap calls I use an XML wrapper so unsure if it will work.
EDIT:
What you may want to try is creating a json query to send or using that to create a xml buy sort of following what is on this page: http://onwebdev.blogspot.com/2011/08/php-converting-rss-to-json.html
You need a multi-dimensional array, you can try the following:
$params = array(
array(
"id" => 100,
"name" => "John",
),
"Barrel of Oil",
500
);
in PHP an array is a structure and is very flexible. Normally with soap calls I use an XML wrapper so unsure if it will work.
EDIT:
What you may want to try is creating a json query to send or using that to create a xml buy sort of following what is on this page: http://onwebdev.blogspot.com/2011/08/php-converting-rss-to-json.html
edited Aug 8 '12 at 19:29
answered Jul 23 '12 at 19:29


James Williams
3,96111331
3,96111331
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
add a comment |
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
thanks, but it did not work either. How do you use XML wrappers exactly, perhaps that is easier to use than this...
– user1305445
Jul 23 '12 at 20:13
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
First you have to make sure that your WSDL can handle XML wrappers. But it is similar, you build the request in XML and in most cases use curl. I have use SOAP with XML to process transactions through banks. You can check out these as a starter point. forums.digitalpoint.com/showthread.php?t=424619#post4004636 w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
and this one w3schools.com/soap/soap_intro.asp
– James Williams
Jul 23 '12 at 20:44
add a comment |
up vote
0
down vote
There is an option to generate php5 objects with WsdlInterpreter class. See more here: https://github.com/gkwelding/WSDLInterpreter
for example:
require_once 'WSDLInterpreter-v1.0.0/WSDLInterpreter.php';
$wsdlLocation = '<your wsdl url>?wsdl';
$wsdlInterpreter = new WSDLInterpreter($wsdlLocation);
$wsdlInterpreter->savePHP('.');
add a comment |
up vote
0
down vote
There is an option to generate php5 objects with WsdlInterpreter class. See more here: https://github.com/gkwelding/WSDLInterpreter
for example:
require_once 'WSDLInterpreter-v1.0.0/WSDLInterpreter.php';
$wsdlLocation = '<your wsdl url>?wsdl';
$wsdlInterpreter = new WSDLInterpreter($wsdlLocation);
$wsdlInterpreter->savePHP('.');
add a comment |
up vote
0
down vote
up vote
0
down vote
There is an option to generate php5 objects with WsdlInterpreter class. See more here: https://github.com/gkwelding/WSDLInterpreter
for example:
require_once 'WSDLInterpreter-v1.0.0/WSDLInterpreter.php';
$wsdlLocation = '<your wsdl url>?wsdl';
$wsdlInterpreter = new WSDLInterpreter($wsdlLocation);
$wsdlInterpreter->savePHP('.');
There is an option to generate php5 objects with WsdlInterpreter class. See more here: https://github.com/gkwelding/WSDLInterpreter
for example:
require_once 'WSDLInterpreter-v1.0.0/WSDLInterpreter.php';
$wsdlLocation = '<your wsdl url>?wsdl';
$wsdlInterpreter = new WSDLInterpreter($wsdlLocation);
$wsdlInterpreter->savePHP('.');
edited Apr 23 at 14:47
answered Apr 23 at 14:41


István Döbrentei
41648
41648
add a comment |
add a comment |
up vote
0
down vote
I had the same issue, but I just wrapped the arguments like this and it works now.
$args = array();
$args['Header'] = array(
'CustomerCode' => 'dsadsad',
'Language' => 'fdsfasdf'
);
$args['RequestObject'] = $whatever;
// this was the catch, double array with "Request"
$response = $this->client->__soapCall($name, array(array( 'Request' => $args )));
Using this function:
print_r($this->client->__getLastRequest());
You can see the Request XML whether it's changing or not depending on your arguments.
Use [ trace = 1, exceptions = 0 ] in SoapClient options.
add a comment |
up vote
0
down vote
I had the same issue, but I just wrapped the arguments like this and it works now.
$args = array();
$args['Header'] = array(
'CustomerCode' => 'dsadsad',
'Language' => 'fdsfasdf'
);
$args['RequestObject'] = $whatever;
// this was the catch, double array with "Request"
$response = $this->client->__soapCall($name, array(array( 'Request' => $args )));
Using this function:
print_r($this->client->__getLastRequest());
You can see the Request XML whether it's changing or not depending on your arguments.
Use [ trace = 1, exceptions = 0 ] in SoapClient options.
add a comment |
up vote
0
down vote
up vote
0
down vote
I had the same issue, but I just wrapped the arguments like this and it works now.
$args = array();
$args['Header'] = array(
'CustomerCode' => 'dsadsad',
'Language' => 'fdsfasdf'
);
$args['RequestObject'] = $whatever;
// this was the catch, double array with "Request"
$response = $this->client->__soapCall($name, array(array( 'Request' => $args )));
Using this function:
print_r($this->client->__getLastRequest());
You can see the Request XML whether it's changing or not depending on your arguments.
Use [ trace = 1, exceptions = 0 ] in SoapClient options.
I had the same issue, but I just wrapped the arguments like this and it works now.
$args = array();
$args['Header'] = array(
'CustomerCode' => 'dsadsad',
'Language' => 'fdsfasdf'
);
$args['RequestObject'] = $whatever;
// this was the catch, double array with "Request"
$response = $this->client->__soapCall($name, array(array( 'Request' => $args )));
Using this function:
print_r($this->client->__getLastRequest());
You can see the Request XML whether it's changing or not depending on your arguments.
Use [ trace = 1, exceptions = 0 ] in SoapClient options.
answered Jun 21 at 14:54


Martin Zvarík
479412
479412
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f11593623%2fhow-to-make-a-php-soap-call-using-the-soapclient-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OjVAvUb,CN62sAE,P1wFKLLaLlqAVNgqNBURPcSF1zuCcg