How to Construct a POST Request Which Expects No Body
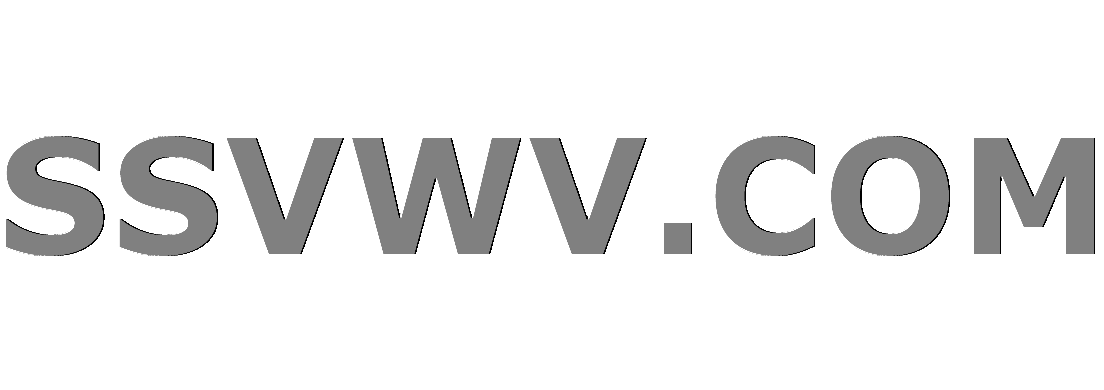
Multi tool use
I've an HTTP client sending many POST
requests to a server. The server responds to all requests with 201 Created
and a response body. For my purposes, the response header is enough, as I'm only interested in the Location
header. I'd like to avoid that the server produces a response body in order to significantly decrease network traffic.
According to RFC 7231, ...
[...] if one or more resources has been created on the origin server as a
result of successfully processing a POST request, the origin server
SHOULD send a 201 (Created) response containing a Location header [...]
..., thus, I assume, the server COULD also respond e.g. with 204 No Content
, omiting the body.
Therefore my question: Is it possible to construct a POST
request which makes the server respond with 204 No Content
or to omit the response body in another way?
Update 1: The server side is a Spring Data REST project and I'm free to configure it. I know that I could set RepositoryRestConfiguration#setReturnBodyOnCreate
to false
, but that would be overdone as it affects all incoming requests. Therefore, I'd prefer to make the decision on the client side.
http spring-boot post spring-data-rest
add a comment |
I've an HTTP client sending many POST
requests to a server. The server responds to all requests with 201 Created
and a response body. For my purposes, the response header is enough, as I'm only interested in the Location
header. I'd like to avoid that the server produces a response body in order to significantly decrease network traffic.
According to RFC 7231, ...
[...] if one or more resources has been created on the origin server as a
result of successfully processing a POST request, the origin server
SHOULD send a 201 (Created) response containing a Location header [...]
..., thus, I assume, the server COULD also respond e.g. with 204 No Content
, omiting the body.
Therefore my question: Is it possible to construct a POST
request which makes the server respond with 204 No Content
or to omit the response body in another way?
Update 1: The server side is a Spring Data REST project and I'm free to configure it. I know that I could set RepositoryRestConfiguration#setReturnBodyOnCreate
to false
, but that would be overdone as it affects all incoming requests. Therefore, I'd prefer to make the decision on the client side.
http spring-boot post spring-data-rest
add a comment |
I've an HTTP client sending many POST
requests to a server. The server responds to all requests with 201 Created
and a response body. For my purposes, the response header is enough, as I'm only interested in the Location
header. I'd like to avoid that the server produces a response body in order to significantly decrease network traffic.
According to RFC 7231, ...
[...] if one or more resources has been created on the origin server as a
result of successfully processing a POST request, the origin server
SHOULD send a 201 (Created) response containing a Location header [...]
..., thus, I assume, the server COULD also respond e.g. with 204 No Content
, omiting the body.
Therefore my question: Is it possible to construct a POST
request which makes the server respond with 204 No Content
or to omit the response body in another way?
Update 1: The server side is a Spring Data REST project and I'm free to configure it. I know that I could set RepositoryRestConfiguration#setReturnBodyOnCreate
to false
, but that would be overdone as it affects all incoming requests. Therefore, I'd prefer to make the decision on the client side.
http spring-boot post spring-data-rest
I've an HTTP client sending many POST
requests to a server. The server responds to all requests with 201 Created
and a response body. For my purposes, the response header is enough, as I'm only interested in the Location
header. I'd like to avoid that the server produces a response body in order to significantly decrease network traffic.
According to RFC 7231, ...
[...] if one or more resources has been created on the origin server as a
result of successfully processing a POST request, the origin server
SHOULD send a 201 (Created) response containing a Location header [...]
..., thus, I assume, the server COULD also respond e.g. with 204 No Content
, omiting the body.
Therefore my question: Is it possible to construct a POST
request which makes the server respond with 204 No Content
or to omit the response body in another way?
Update 1: The server side is a Spring Data REST project and I'm free to configure it. I know that I could set RepositoryRestConfiguration#setReturnBodyOnCreate
to false
, but that would be overdone as it affects all incoming requests. Therefore, I'd prefer to make the decision on the client side.
http spring-boot post spring-data-rest
http spring-boot post spring-data-rest
edited Dec 2 '18 at 9:22
aboger
asked Nov 20 '18 at 10:50


abogeraboger
44211229
44211229
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
There's no real lever you can pull from the client side to control if the server will respond with a body or not, unless the service you work with has a specific feature that allows this.
A header that a server might use is Prefer: return=minimal
but if the service doesn't explicitly document support for this, chances are low that this will work.
Really the only think you can do one the client is to:
- Kill the TCP connection as soon as you got the response headers
- Kill the HTTP/2 stream when you recieved the headers.
This is a pretty 'drastic' thing but clients do use this mechanism for some cases and it does work. However, if the POST response body was somewhat small there's a chance that it's not really making a ton of difference because the response might already have been sent.
ThePrefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.
– aboger
Nov 26 '18 at 10:21
add a comment |
There is no way to do it client side only as it is not natively implemented in Spring REST server.
Anyway, any client demand can be transformed as an extra custom header or a query parameter in the request.
A way could be to override default response handlers and detect custom header (implements Prefer: return=minimal
as suggested before for instance) and/or query param presence to trigger an empty response with a 204 status. This post may help you to figure it out.
add a comment |
Can you try changing your client such that you
- a) Query the server with HTTP HEAD requests instead of POST requests
- b) Analyze the response headers. There is no response body for HEAD requests as the purpose of HEAD requests is very similar to your requirement
- c) Perform the necessary POST requests only when required
I understand that you may have difficulties at the client end to apply these changes. But, in the longer run, I believe this would be worth it.
Thanks for the suggestion, butHEAD
requests areidempotent
andsafe
, therefore allPOST
requests are still necessary. I guess this would rather increase than decrease overhead.
– aboger
Nov 30 '18 at 11:33
add a comment |
Based on Evert's and Bertrand's answers plus a bit of googling, I finally implemented the following interceptor in the Spring Data REST server:
@Configuration
class RepositoryConfiguration {
@Bean
public MappedInterceptor preferReturnMinimalMappedInterceptor() {
return new MappedInterceptor(new String{"/**"}, new HandlerInterceptor() {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
if ("return=minimal".equals(request.getHeader("prefer"))) {
response.setContentLength(0);
response.addHeader("Preference-Applied", "return=minimal"");
}
return true;
}
});
}
}
It produces the following communication, which is good enough for my purposes:
> POST /versions HTTP/1.1
> Host: localhost:8080
> User-Agent: curl/7.59.0
> Accept: */*
> Content-Type: application/json
> Prefer: return=minimal
> Content-Length: 123
>
> [123 bytes data]
...
< HTTP/1.1 201
< Preference-Applied: return=minimal
< ETag: "0"
< Last-Modified: Fri, 30 Nov 2018 12:37:57 GMT
< Location: http://localhost:8080/versions/1
< Content-Type: application/hal+json;charset=UTF-8
< Content-Length: 0
< Date: Fri, 30 Nov 2018 12:37:57 GMT
I would like to share the bounty evenly, but this is not possible. It goes to Bertrand, as he came with an answer which guided me to the very implementation. Thanks for your help.
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
I tried that while implementing it,setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with201 Created
, as it's also a good piece of information.
– aboger
Nov 30 '18 at 14:54
I also guess that the response headerReturn: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.
– aboger
Nov 30 '18 at 14:57
1
Instead of theReturn: Minimal
you can useVary: Prefer
and/orPreference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391369%2fhow-to-construct-a-post-request-which-expects-no-body%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
There's no real lever you can pull from the client side to control if the server will respond with a body or not, unless the service you work with has a specific feature that allows this.
A header that a server might use is Prefer: return=minimal
but if the service doesn't explicitly document support for this, chances are low that this will work.
Really the only think you can do one the client is to:
- Kill the TCP connection as soon as you got the response headers
- Kill the HTTP/2 stream when you recieved the headers.
This is a pretty 'drastic' thing but clients do use this mechanism for some cases and it does work. However, if the POST response body was somewhat small there's a chance that it's not really making a ton of difference because the response might already have been sent.
ThePrefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.
– aboger
Nov 26 '18 at 10:21
add a comment |
There's no real lever you can pull from the client side to control if the server will respond with a body or not, unless the service you work with has a specific feature that allows this.
A header that a server might use is Prefer: return=minimal
but if the service doesn't explicitly document support for this, chances are low that this will work.
Really the only think you can do one the client is to:
- Kill the TCP connection as soon as you got the response headers
- Kill the HTTP/2 stream when you recieved the headers.
This is a pretty 'drastic' thing but clients do use this mechanism for some cases and it does work. However, if the POST response body was somewhat small there's a chance that it's not really making a ton of difference because the response might already have been sent.
ThePrefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.
– aboger
Nov 26 '18 at 10:21
add a comment |
There's no real lever you can pull from the client side to control if the server will respond with a body or not, unless the service you work with has a specific feature that allows this.
A header that a server might use is Prefer: return=minimal
but if the service doesn't explicitly document support for this, chances are low that this will work.
Really the only think you can do one the client is to:
- Kill the TCP connection as soon as you got the response headers
- Kill the HTTP/2 stream when you recieved the headers.
This is a pretty 'drastic' thing but clients do use this mechanism for some cases and it does work. However, if the POST response body was somewhat small there's a chance that it's not really making a ton of difference because the response might already have been sent.
There's no real lever you can pull from the client side to control if the server will respond with a body or not, unless the service you work with has a specific feature that allows this.
A header that a server might use is Prefer: return=minimal
but if the service doesn't explicitly document support for this, chances are low that this will work.
Really the only think you can do one the client is to:
- Kill the TCP connection as soon as you got the response headers
- Kill the HTTP/2 stream when you recieved the headers.
This is a pretty 'drastic' thing but clients do use this mechanism for some cases and it does work. However, if the POST response body was somewhat small there's a chance that it's not really making a ton of difference because the response might already have been sent.
answered Nov 23 '18 at 16:08
EvertEvert
40.5k1569123
40.5k1569123
ThePrefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.
– aboger
Nov 26 '18 at 10:21
add a comment |
ThePrefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.
– aboger
Nov 26 '18 at 10:21
The
Prefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.– aboger
Nov 26 '18 at 10:21
The
Prefer
header seems to be a perfect fit, but sadly didn't work for me. The server side is a Spring Data REST project, therefore I was able to try the header using an embedded Tomcat, Jetty and Undertow server. Killing the TCP connection or the HTTP/2 stream is not an option for me, but an interesting idea. Thanks for sharing.– aboger
Nov 26 '18 at 10:21
add a comment |
There is no way to do it client side only as it is not natively implemented in Spring REST server.
Anyway, any client demand can be transformed as an extra custom header or a query parameter in the request.
A way could be to override default response handlers and detect custom header (implements Prefer: return=minimal
as suggested before for instance) and/or query param presence to trigger an empty response with a 204 status. This post may help you to figure it out.
add a comment |
There is no way to do it client side only as it is not natively implemented in Spring REST server.
Anyway, any client demand can be transformed as an extra custom header or a query parameter in the request.
A way could be to override default response handlers and detect custom header (implements Prefer: return=minimal
as suggested before for instance) and/or query param presence to trigger an empty response with a 204 status. This post may help you to figure it out.
add a comment |
There is no way to do it client side only as it is not natively implemented in Spring REST server.
Anyway, any client demand can be transformed as an extra custom header or a query parameter in the request.
A way could be to override default response handlers and detect custom header (implements Prefer: return=minimal
as suggested before for instance) and/or query param presence to trigger an empty response with a 204 status. This post may help you to figure it out.
There is no way to do it client side only as it is not natively implemented in Spring REST server.
Anyway, any client demand can be transformed as an extra custom header or a query parameter in the request.
A way could be to override default response handlers and detect custom header (implements Prefer: return=minimal
as suggested before for instance) and/or query param presence to trigger an empty response with a 204 status. This post may help you to figure it out.
answered Nov 30 '18 at 8:33


BertrandBertrand
930415
930415
add a comment |
add a comment |
Can you try changing your client such that you
- a) Query the server with HTTP HEAD requests instead of POST requests
- b) Analyze the response headers. There is no response body for HEAD requests as the purpose of HEAD requests is very similar to your requirement
- c) Perform the necessary POST requests only when required
I understand that you may have difficulties at the client end to apply these changes. But, in the longer run, I believe this would be worth it.
Thanks for the suggestion, butHEAD
requests areidempotent
andsafe
, therefore allPOST
requests are still necessary. I guess this would rather increase than decrease overhead.
– aboger
Nov 30 '18 at 11:33
add a comment |
Can you try changing your client such that you
- a) Query the server with HTTP HEAD requests instead of POST requests
- b) Analyze the response headers. There is no response body for HEAD requests as the purpose of HEAD requests is very similar to your requirement
- c) Perform the necessary POST requests only when required
I understand that you may have difficulties at the client end to apply these changes. But, in the longer run, I believe this would be worth it.
Thanks for the suggestion, butHEAD
requests areidempotent
andsafe
, therefore allPOST
requests are still necessary. I guess this would rather increase than decrease overhead.
– aboger
Nov 30 '18 at 11:33
add a comment |
Can you try changing your client such that you
- a) Query the server with HTTP HEAD requests instead of POST requests
- b) Analyze the response headers. There is no response body for HEAD requests as the purpose of HEAD requests is very similar to your requirement
- c) Perform the necessary POST requests only when required
I understand that you may have difficulties at the client end to apply these changes. But, in the longer run, I believe this would be worth it.
Can you try changing your client such that you
- a) Query the server with HTTP HEAD requests instead of POST requests
- b) Analyze the response headers. There is no response body for HEAD requests as the purpose of HEAD requests is very similar to your requirement
- c) Perform the necessary POST requests only when required
I understand that you may have difficulties at the client end to apply these changes. But, in the longer run, I believe this would be worth it.
answered Nov 30 '18 at 9:46
Ruchira RandanaRuchira Randana
2,64811820
2,64811820
Thanks for the suggestion, butHEAD
requests areidempotent
andsafe
, therefore allPOST
requests are still necessary. I guess this would rather increase than decrease overhead.
– aboger
Nov 30 '18 at 11:33
add a comment |
Thanks for the suggestion, butHEAD
requests areidempotent
andsafe
, therefore allPOST
requests are still necessary. I guess this would rather increase than decrease overhead.
– aboger
Nov 30 '18 at 11:33
Thanks for the suggestion, but
HEAD
requests are idempotent
and safe
, therefore all POST
requests are still necessary. I guess this would rather increase than decrease overhead.– aboger
Nov 30 '18 at 11:33
Thanks for the suggestion, but
HEAD
requests are idempotent
and safe
, therefore all POST
requests are still necessary. I guess this would rather increase than decrease overhead.– aboger
Nov 30 '18 at 11:33
add a comment |
Based on Evert's and Bertrand's answers plus a bit of googling, I finally implemented the following interceptor in the Spring Data REST server:
@Configuration
class RepositoryConfiguration {
@Bean
public MappedInterceptor preferReturnMinimalMappedInterceptor() {
return new MappedInterceptor(new String{"/**"}, new HandlerInterceptor() {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
if ("return=minimal".equals(request.getHeader("prefer"))) {
response.setContentLength(0);
response.addHeader("Preference-Applied", "return=minimal"");
}
return true;
}
});
}
}
It produces the following communication, which is good enough for my purposes:
> POST /versions HTTP/1.1
> Host: localhost:8080
> User-Agent: curl/7.59.0
> Accept: */*
> Content-Type: application/json
> Prefer: return=minimal
> Content-Length: 123
>
> [123 bytes data]
...
< HTTP/1.1 201
< Preference-Applied: return=minimal
< ETag: "0"
< Last-Modified: Fri, 30 Nov 2018 12:37:57 GMT
< Location: http://localhost:8080/versions/1
< Content-Type: application/hal+json;charset=UTF-8
< Content-Length: 0
< Date: Fri, 30 Nov 2018 12:37:57 GMT
I would like to share the bounty evenly, but this is not possible. It goes to Bertrand, as he came with an answer which guided me to the very implementation. Thanks for your help.
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
I tried that while implementing it,setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with201 Created
, as it's also a good piece of information.
– aboger
Nov 30 '18 at 14:54
I also guess that the response headerReturn: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.
– aboger
Nov 30 '18 at 14:57
1
Instead of theReturn: Minimal
you can useVary: Prefer
and/orPreference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
|
show 2 more comments
Based on Evert's and Bertrand's answers plus a bit of googling, I finally implemented the following interceptor in the Spring Data REST server:
@Configuration
class RepositoryConfiguration {
@Bean
public MappedInterceptor preferReturnMinimalMappedInterceptor() {
return new MappedInterceptor(new String{"/**"}, new HandlerInterceptor() {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
if ("return=minimal".equals(request.getHeader("prefer"))) {
response.setContentLength(0);
response.addHeader("Preference-Applied", "return=minimal"");
}
return true;
}
});
}
}
It produces the following communication, which is good enough for my purposes:
> POST /versions HTTP/1.1
> Host: localhost:8080
> User-Agent: curl/7.59.0
> Accept: */*
> Content-Type: application/json
> Prefer: return=minimal
> Content-Length: 123
>
> [123 bytes data]
...
< HTTP/1.1 201
< Preference-Applied: return=minimal
< ETag: "0"
< Last-Modified: Fri, 30 Nov 2018 12:37:57 GMT
< Location: http://localhost:8080/versions/1
< Content-Type: application/hal+json;charset=UTF-8
< Content-Length: 0
< Date: Fri, 30 Nov 2018 12:37:57 GMT
I would like to share the bounty evenly, but this is not possible. It goes to Bertrand, as he came with an answer which guided me to the very implementation. Thanks for your help.
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
I tried that while implementing it,setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with201 Created
, as it's also a good piece of information.
– aboger
Nov 30 '18 at 14:54
I also guess that the response headerReturn: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.
– aboger
Nov 30 '18 at 14:57
1
Instead of theReturn: Minimal
you can useVary: Prefer
and/orPreference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
|
show 2 more comments
Based on Evert's and Bertrand's answers plus a bit of googling, I finally implemented the following interceptor in the Spring Data REST server:
@Configuration
class RepositoryConfiguration {
@Bean
public MappedInterceptor preferReturnMinimalMappedInterceptor() {
return new MappedInterceptor(new String{"/**"}, new HandlerInterceptor() {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
if ("return=minimal".equals(request.getHeader("prefer"))) {
response.setContentLength(0);
response.addHeader("Preference-Applied", "return=minimal"");
}
return true;
}
});
}
}
It produces the following communication, which is good enough for my purposes:
> POST /versions HTTP/1.1
> Host: localhost:8080
> User-Agent: curl/7.59.0
> Accept: */*
> Content-Type: application/json
> Prefer: return=minimal
> Content-Length: 123
>
> [123 bytes data]
...
< HTTP/1.1 201
< Preference-Applied: return=minimal
< ETag: "0"
< Last-Modified: Fri, 30 Nov 2018 12:37:57 GMT
< Location: http://localhost:8080/versions/1
< Content-Type: application/hal+json;charset=UTF-8
< Content-Length: 0
< Date: Fri, 30 Nov 2018 12:37:57 GMT
I would like to share the bounty evenly, but this is not possible. It goes to Bertrand, as he came with an answer which guided me to the very implementation. Thanks for your help.
Based on Evert's and Bertrand's answers plus a bit of googling, I finally implemented the following interceptor in the Spring Data REST server:
@Configuration
class RepositoryConfiguration {
@Bean
public MappedInterceptor preferReturnMinimalMappedInterceptor() {
return new MappedInterceptor(new String{"/**"}, new HandlerInterceptor() {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
if ("return=minimal".equals(request.getHeader("prefer"))) {
response.setContentLength(0);
response.addHeader("Preference-Applied", "return=minimal"");
}
return true;
}
});
}
}
It produces the following communication, which is good enough for my purposes:
> POST /versions HTTP/1.1
> Host: localhost:8080
> User-Agent: curl/7.59.0
> Accept: */*
> Content-Type: application/json
> Prefer: return=minimal
> Content-Length: 123
>
> [123 bytes data]
...
< HTTP/1.1 201
< Preference-Applied: return=minimal
< ETag: "0"
< Last-Modified: Fri, 30 Nov 2018 12:37:57 GMT
< Location: http://localhost:8080/versions/1
< Content-Type: application/hal+json;charset=UTF-8
< Content-Length: 0
< Date: Fri, 30 Nov 2018 12:37:57 GMT
I would like to share the bounty evenly, but this is not possible. It goes to Bertrand, as he came with an answer which guided me to the very implementation. Thanks for your help.
edited Nov 30 '18 at 17:52
answered Nov 30 '18 at 12:57


abogeraboger
44211229
44211229
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
I tried that while implementing it,setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with201 Created
, as it's also a good piece of information.
– aboger
Nov 30 '18 at 14:54
I also guess that the response headerReturn: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.
– aboger
Nov 30 '18 at 14:57
1
Instead of theReturn: Minimal
you can useVary: Prefer
and/orPreference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
|
show 2 more comments
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
I tried that while implementing it,setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with201 Created
, as it's also a good piece of information.
– aboger
Nov 30 '18 at 14:54
I also guess that the response headerReturn: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.
– aboger
Nov 30 '18 at 14:57
1
Instead of theReturn: Minimal
you can useVary: Prefer
and/orPreference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
Thank you and you're welcome
– Bertrand
Nov 30 '18 at 13:55
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
You can also add response.setStatus(204) to update HTTP response code
– Bertrand
Nov 30 '18 at 14:00
I tried that while implementing it,
setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with 201 Created
, as it's also a good piece of information.– aboger
Nov 30 '18 at 14:54
I tried that while implementing it,
setMethod()
and some other methods had no effect, so I also wasn't able to remove the content-type. I'm satisfied with 201 Created
, as it's also a good piece of information.– aboger
Nov 30 '18 at 14:54
I also guess that the response header
Return: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.– aboger
Nov 30 '18 at 14:57
I also guess that the response header
Return: minimal
is not compliant with HTTP standard, but rather a custom header. However, for me, that's not an issue.– aboger
Nov 30 '18 at 14:57
1
1
Instead of the
Return: Minimal
you can use Vary: Prefer
and/or Preference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
Instead of the
Return: Minimal
you can use Vary: Prefer
and/or Preference-Applied: return=minimal
– Evert
Nov 30 '18 at 17:00
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53391369%2fhow-to-construct-a-post-request-which-expects-no-body%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GA0ehQxWKuIP0BFP8053L 37uSZtAKGR