I2C slave module in Verilog does not acknowledge
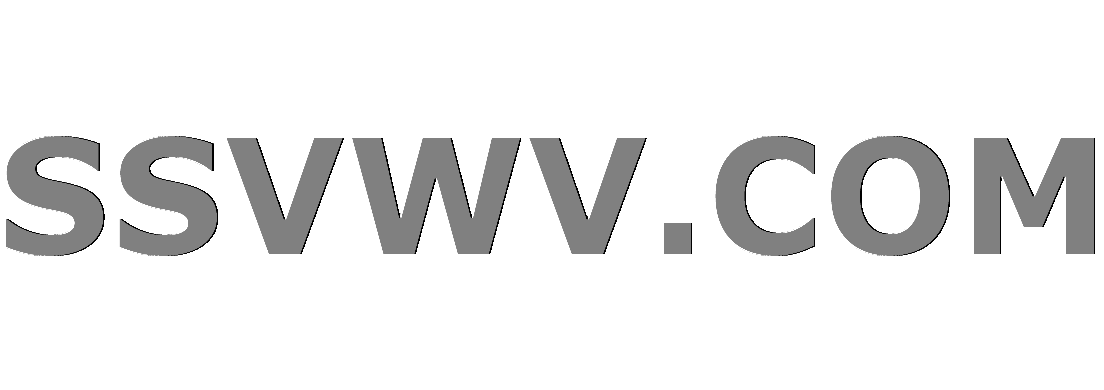
Multi tool use
I wrote this I2C slave module in Verilog:
module I2CSlave(
input iSCL,
input iI2C_CLK,
inout bSDA,
output reg [7:0] odata,
output reg oread,
output wire oactive
);
reg incycle = 1'b0;
reg pSDA;
reg pSCL;
always @(posedge iI2C_CLK) begin
if ((pSCL) && (iSCL) && (pSDA) && (~bSDA)) begin
incycle <= 1;
end
if ((pSCL) && (iSCL) && (~pSDA) && (bSDA)) begin
incycle <= 0;
end
pSDA <= bSDA;
pSCL <= iSCL;
end
assign oactive = incycle;
localparam STATE_IDLE = 0;
localparam STATE_ADDR = 1;
localparam STATE_RW = 2;
localparam STATE_ACK = 3;
localparam STATE_DATA = 4;
localparam STATE_ACK2 = 5;
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
reg rw;
reg lSDA;
always @(posedge iSCL) lSDA <= bSDA;
assign bSDA = ((state == STATE_ACK) || (state == STATE_ACK2)) ? 0 : 1'bz;
assign oread = (state == STATE_ACK2);
assign ostate = i;
always @(negedge iSCL or negedge incycle) begin
if (~incycle) begin
state <= STATE_IDLE;
addr_match <= 1;
end
else if (addr_match) begin
case (state)
STATE_IDLE: begin
state <= STATE_ADDR;
i <= 7;
end
STATE_ADDR: begin
if (addr[i-1] != lSDA) addr_match <= 0;
if (i == 1) begin
state <= STATE_RW;
i <= i - 1;
end
else i <= i - 1;
end
STATE_RW: begin
rw <= lSDA;
state <= STATE_ACK;
end
STATE_ACK: begin
state <= STATE_DATA;
i = 7;
end
STATE_DATA : begin
odata[i] <= lSDA;
if (i == 0) state <= STATE_ACK2;
else i <= i - 1;
end
STATE_ACK2: begin
state <= STATE_DATA;
i = 7;
end
endcase
end
end
endmodule
As of now it should just read the data sent by master. It seems to work well in simulation, but when I upload it into the FPGA, sometimes everything is OK, however sometimes it does not acknowledge the data sent by master and it just seems to ignore them. I am newbie in Verilog, so I hope, this is not a silly question.
verilog i2c
add a comment |
I wrote this I2C slave module in Verilog:
module I2CSlave(
input iSCL,
input iI2C_CLK,
inout bSDA,
output reg [7:0] odata,
output reg oread,
output wire oactive
);
reg incycle = 1'b0;
reg pSDA;
reg pSCL;
always @(posedge iI2C_CLK) begin
if ((pSCL) && (iSCL) && (pSDA) && (~bSDA)) begin
incycle <= 1;
end
if ((pSCL) && (iSCL) && (~pSDA) && (bSDA)) begin
incycle <= 0;
end
pSDA <= bSDA;
pSCL <= iSCL;
end
assign oactive = incycle;
localparam STATE_IDLE = 0;
localparam STATE_ADDR = 1;
localparam STATE_RW = 2;
localparam STATE_ACK = 3;
localparam STATE_DATA = 4;
localparam STATE_ACK2 = 5;
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
reg rw;
reg lSDA;
always @(posedge iSCL) lSDA <= bSDA;
assign bSDA = ((state == STATE_ACK) || (state == STATE_ACK2)) ? 0 : 1'bz;
assign oread = (state == STATE_ACK2);
assign ostate = i;
always @(negedge iSCL or negedge incycle) begin
if (~incycle) begin
state <= STATE_IDLE;
addr_match <= 1;
end
else if (addr_match) begin
case (state)
STATE_IDLE: begin
state <= STATE_ADDR;
i <= 7;
end
STATE_ADDR: begin
if (addr[i-1] != lSDA) addr_match <= 0;
if (i == 1) begin
state <= STATE_RW;
i <= i - 1;
end
else i <= i - 1;
end
STATE_RW: begin
rw <= lSDA;
state <= STATE_ACK;
end
STATE_ACK: begin
state <= STATE_DATA;
i = 7;
end
STATE_DATA : begin
odata[i] <= lSDA;
if (i == 0) state <= STATE_ACK2;
else i <= i - 1;
end
STATE_ACK2: begin
state <= STATE_DATA;
i = 7;
end
endcase
end
end
endmodule
As of now it should just read the data sent by master. It seems to work well in simulation, but when I upload it into the FPGA, sometimes everything is OK, however sometimes it does not acknowledge the data sent by master and it just seems to ignore them. I am newbie in Verilog, so I hope, this is not a silly question.
verilog i2c
How thoroughly does your simulation testbench check your design? Do you have 100% coverage?
– toolic
Nov 23 '18 at 19:27
The testbench only tries to initiate the communication by sending the address and then it sends 1 byte of data. Also the communication must be sped up in simulation, because there is only 100 us available in Quartus Lite.
– Martin Schmied
Nov 23 '18 at 19:51
add a comment |
I wrote this I2C slave module in Verilog:
module I2CSlave(
input iSCL,
input iI2C_CLK,
inout bSDA,
output reg [7:0] odata,
output reg oread,
output wire oactive
);
reg incycle = 1'b0;
reg pSDA;
reg pSCL;
always @(posedge iI2C_CLK) begin
if ((pSCL) && (iSCL) && (pSDA) && (~bSDA)) begin
incycle <= 1;
end
if ((pSCL) && (iSCL) && (~pSDA) && (bSDA)) begin
incycle <= 0;
end
pSDA <= bSDA;
pSCL <= iSCL;
end
assign oactive = incycle;
localparam STATE_IDLE = 0;
localparam STATE_ADDR = 1;
localparam STATE_RW = 2;
localparam STATE_ACK = 3;
localparam STATE_DATA = 4;
localparam STATE_ACK2 = 5;
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
reg rw;
reg lSDA;
always @(posedge iSCL) lSDA <= bSDA;
assign bSDA = ((state == STATE_ACK) || (state == STATE_ACK2)) ? 0 : 1'bz;
assign oread = (state == STATE_ACK2);
assign ostate = i;
always @(negedge iSCL or negedge incycle) begin
if (~incycle) begin
state <= STATE_IDLE;
addr_match <= 1;
end
else if (addr_match) begin
case (state)
STATE_IDLE: begin
state <= STATE_ADDR;
i <= 7;
end
STATE_ADDR: begin
if (addr[i-1] != lSDA) addr_match <= 0;
if (i == 1) begin
state <= STATE_RW;
i <= i - 1;
end
else i <= i - 1;
end
STATE_RW: begin
rw <= lSDA;
state <= STATE_ACK;
end
STATE_ACK: begin
state <= STATE_DATA;
i = 7;
end
STATE_DATA : begin
odata[i] <= lSDA;
if (i == 0) state <= STATE_ACK2;
else i <= i - 1;
end
STATE_ACK2: begin
state <= STATE_DATA;
i = 7;
end
endcase
end
end
endmodule
As of now it should just read the data sent by master. It seems to work well in simulation, but when I upload it into the FPGA, sometimes everything is OK, however sometimes it does not acknowledge the data sent by master and it just seems to ignore them. I am newbie in Verilog, so I hope, this is not a silly question.
verilog i2c
I wrote this I2C slave module in Verilog:
module I2CSlave(
input iSCL,
input iI2C_CLK,
inout bSDA,
output reg [7:0] odata,
output reg oread,
output wire oactive
);
reg incycle = 1'b0;
reg pSDA;
reg pSCL;
always @(posedge iI2C_CLK) begin
if ((pSCL) && (iSCL) && (pSDA) && (~bSDA)) begin
incycle <= 1;
end
if ((pSCL) && (iSCL) && (~pSDA) && (bSDA)) begin
incycle <= 0;
end
pSDA <= bSDA;
pSCL <= iSCL;
end
assign oactive = incycle;
localparam STATE_IDLE = 0;
localparam STATE_ADDR = 1;
localparam STATE_RW = 2;
localparam STATE_ACK = 3;
localparam STATE_DATA = 4;
localparam STATE_ACK2 = 5;
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
reg rw;
reg lSDA;
always @(posedge iSCL) lSDA <= bSDA;
assign bSDA = ((state == STATE_ACK) || (state == STATE_ACK2)) ? 0 : 1'bz;
assign oread = (state == STATE_ACK2);
assign ostate = i;
always @(negedge iSCL or negedge incycle) begin
if (~incycle) begin
state <= STATE_IDLE;
addr_match <= 1;
end
else if (addr_match) begin
case (state)
STATE_IDLE: begin
state <= STATE_ADDR;
i <= 7;
end
STATE_ADDR: begin
if (addr[i-1] != lSDA) addr_match <= 0;
if (i == 1) begin
state <= STATE_RW;
i <= i - 1;
end
else i <= i - 1;
end
STATE_RW: begin
rw <= lSDA;
state <= STATE_ACK;
end
STATE_ACK: begin
state <= STATE_DATA;
i = 7;
end
STATE_DATA : begin
odata[i] <= lSDA;
if (i == 0) state <= STATE_ACK2;
else i <= i - 1;
end
STATE_ACK2: begin
state <= STATE_DATA;
i = 7;
end
endcase
end
end
endmodule
As of now it should just read the data sent by master. It seems to work well in simulation, but when I upload it into the FPGA, sometimes everything is OK, however sometimes it does not acknowledge the data sent by master and it just seems to ignore them. I am newbie in Verilog, so I hope, this is not a silly question.
verilog i2c
verilog i2c
edited Nov 25 '18 at 12:36
Martin Schmied
asked Nov 23 '18 at 18:28
Martin SchmiedMartin Schmied
148
148
How thoroughly does your simulation testbench check your design? Do you have 100% coverage?
– toolic
Nov 23 '18 at 19:27
The testbench only tries to initiate the communication by sending the address and then it sends 1 byte of data. Also the communication must be sped up in simulation, because there is only 100 us available in Quartus Lite.
– Martin Schmied
Nov 23 '18 at 19:51
add a comment |
How thoroughly does your simulation testbench check your design? Do you have 100% coverage?
– toolic
Nov 23 '18 at 19:27
The testbench only tries to initiate the communication by sending the address and then it sends 1 byte of data. Also the communication must be sped up in simulation, because there is only 100 us available in Quartus Lite.
– Martin Schmied
Nov 23 '18 at 19:51
How thoroughly does your simulation testbench check your design? Do you have 100% coverage?
– toolic
Nov 23 '18 at 19:27
How thoroughly does your simulation testbench check your design? Do you have 100% coverage?
– toolic
Nov 23 '18 at 19:27
The testbench only tries to initiate the communication by sending the address and then it sends 1 byte of data. Also the communication must be sped up in simulation, because there is only 100 us available in Quartus Lite.
– Martin Schmied
Nov 23 '18 at 19:51
The testbench only tries to initiate the communication by sending the address and then it sends 1 byte of data. Also the communication must be sped up in simulation, because there is only 100 us available in Quartus Lite.
– Martin Schmied
Nov 23 '18 at 19:51
add a comment |
2 Answers
2
active
oldest
votes
One possible cause for random fails while running on real hardware is that you didn't synchronize inputs.
You are sampling slowly changing signals (i2c bus will have a long slopes) that are truly asynchronous to your design's clock. Depending on your luck, you will have random violations for setup/hold times of your fpga's d-flops, which results in metastability issues. The same value in the register might be treated differently in multiple parts of the chip. That will wreak havoc in your i2c slave's logic.
You must synchronize asynchronous inputs, in the simplest case passing it through a couple of registers before feeding it to the module's fsm.
add a comment |
You have several issues with your code which could cause mismatch in behavior in simulation and synthesis. For example, the following is not synthesizable and is ignored by synthesis tools. So, your initial state would be different. Check your logs for warnings. Do not use declaration assignments for regs
. (ok for wires).
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
The above means that you initialization does not work.
You messed up blocking and non-blocking assignments in the state machine. Make sure that you use nbas in all places where 'i = 7'. it should be
i <= 7;
And make sure that you test enough initialization and different conditions in simulation.
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451470%2fi2c-slave-module-in-verilog-does-not-acknowledge%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
One possible cause for random fails while running on real hardware is that you didn't synchronize inputs.
You are sampling slowly changing signals (i2c bus will have a long slopes) that are truly asynchronous to your design's clock. Depending on your luck, you will have random violations for setup/hold times of your fpga's d-flops, which results in metastability issues. The same value in the register might be treated differently in multiple parts of the chip. That will wreak havoc in your i2c slave's logic.
You must synchronize asynchronous inputs, in the simplest case passing it through a couple of registers before feeding it to the module's fsm.
add a comment |
One possible cause for random fails while running on real hardware is that you didn't synchronize inputs.
You are sampling slowly changing signals (i2c bus will have a long slopes) that are truly asynchronous to your design's clock. Depending on your luck, you will have random violations for setup/hold times of your fpga's d-flops, which results in metastability issues. The same value in the register might be treated differently in multiple parts of the chip. That will wreak havoc in your i2c slave's logic.
You must synchronize asynchronous inputs, in the simplest case passing it through a couple of registers before feeding it to the module's fsm.
add a comment |
One possible cause for random fails while running on real hardware is that you didn't synchronize inputs.
You are sampling slowly changing signals (i2c bus will have a long slopes) that are truly asynchronous to your design's clock. Depending on your luck, you will have random violations for setup/hold times of your fpga's d-flops, which results in metastability issues. The same value in the register might be treated differently in multiple parts of the chip. That will wreak havoc in your i2c slave's logic.
You must synchronize asynchronous inputs, in the simplest case passing it through a couple of registers before feeding it to the module's fsm.
One possible cause for random fails while running on real hardware is that you didn't synchronize inputs.
You are sampling slowly changing signals (i2c bus will have a long slopes) that are truly asynchronous to your design's clock. Depending on your luck, you will have random violations for setup/hold times of your fpga's d-flops, which results in metastability issues. The same value in the register might be treated differently in multiple parts of the chip. That will wreak havoc in your i2c slave's logic.
You must synchronize asynchronous inputs, in the simplest case passing it through a couple of registers before feeding it to the module's fsm.
answered Nov 24 '18 at 11:32
VladVlad
3,6921511
3,6921511
add a comment |
add a comment |
You have several issues with your code which could cause mismatch in behavior in simulation and synthesis. For example, the following is not synthesizable and is ignored by synthesis tools. So, your initial state would be different. Check your logs for warnings. Do not use declaration assignments for regs
. (ok for wires).
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
The above means that you initialization does not work.
You messed up blocking and non-blocking assignments in the state machine. Make sure that you use nbas in all places where 'i = 7'. it should be
i <= 7;
And make sure that you test enough initialization and different conditions in simulation.
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
|
show 1 more comment
You have several issues with your code which could cause mismatch in behavior in simulation and synthesis. For example, the following is not synthesizable and is ignored by synthesis tools. So, your initial state would be different. Check your logs for warnings. Do not use declaration assignments for regs
. (ok for wires).
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
The above means that you initialization does not work.
You messed up blocking and non-blocking assignments in the state machine. Make sure that you use nbas in all places where 'i = 7'. it should be
i <= 7;
And make sure that you test enough initialization and different conditions in simulation.
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
|
show 1 more comment
You have several issues with your code which could cause mismatch in behavior in simulation and synthesis. For example, the following is not synthesizable and is ignored by synthesis tools. So, your initial state would be different. Check your logs for warnings. Do not use declaration assignments for regs
. (ok for wires).
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
The above means that you initialization does not work.
You messed up blocking and non-blocking assignments in the state machine. Make sure that you use nbas in all places where 'i = 7'. it should be
i <= 7;
And make sure that you test enough initialization and different conditions in simulation.
You have several issues with your code which could cause mismatch in behavior in simulation and synthesis. For example, the following is not synthesizable and is ignored by synthesis tools. So, your initial state would be different. Check your logs for warnings. Do not use declaration assignments for regs
. (ok for wires).
reg [7:0] i = 0;
reg [7:0] state = STATE_IDLE;
reg [6:0] addr = 7'h03;
reg addr_match = 1;
The above means that you initialization does not work.
You messed up blocking and non-blocking assignments in the state machine. Make sure that you use nbas in all places where 'i = 7'. it should be
i <= 7;
And make sure that you test enough initialization and different conditions in simulation.
answered Nov 23 '18 at 22:04
SergeSerge
3,57921014
3,57921014
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
|
show 1 more comment
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Why not synthesizable? Yes, in ASIC you must reset registers explicitly, but sram-based fpga will definitely initialize registers to a specified values, and synthesizer will not complain. Or maybe it's only Altera/Xilinx allows this?
– Vlad
Nov 24 '18 at 8:09
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Ok, found a good answer to this: electronics.stackexchange.com/a/340338/127801
– Vlad
Nov 24 '18 at 8:24
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
Thanks for tip. I replaced the incorrect blocking assignments by the non-blocking ones. Then I removed the unnecessary initializations and I moved the needed ones into initial block. So the uninitialized registers should be equal to 0, right? The problem is, that the FPGA still behaves in the same way. What else exactly should I test in the simulation?
– Martin Schmied
Nov 24 '18 at 9:23
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@Vlad I am using Altera, so it probably should work even the way I had it in my original design, as you have written, but I have also tried to avoid it, as Serge has written, nevertheless it behaves the same way in both cases.
– Martin Schmied
Nov 24 '18 at 9:30
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
@MartinSchmied you have to remember that initial blocks are not synthesizable as well. You need to create a reset condition where you can initialize your variables.
– Serge
Nov 25 '18 at 13:48
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451470%2fi2c-slave-module-in-verilog-does-not-acknowledge%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HvX w qQHu
How thoroughly does your simulation testbench check your design? Do you have 100% coverage?
– toolic
Nov 23 '18 at 19:27
The testbench only tries to initiate the communication by sending the address and then it sends 1 byte of data. Also the communication must be sped up in simulation, because there is only 100 us available in Quartus Lite.
– Martin Schmied
Nov 23 '18 at 19:51