Laravel: Create a new autoconnected User with javascript
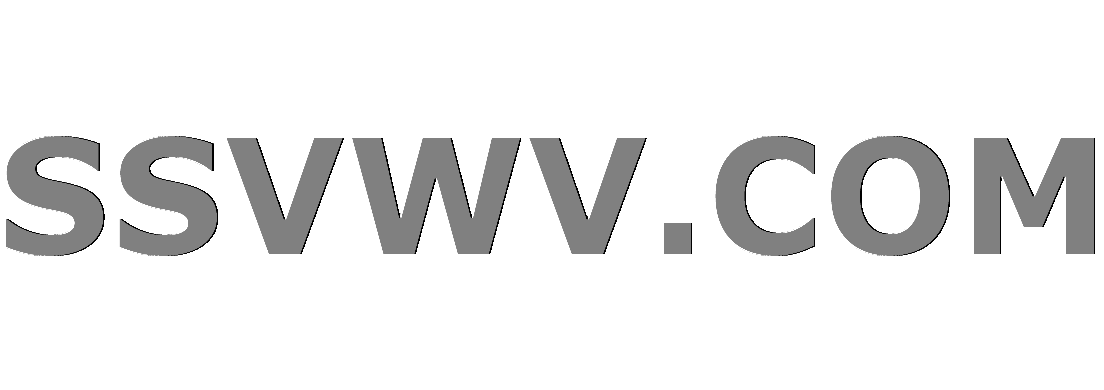
Multi tool use
I have a form where at some point, the user can enter his email. So, I use it to create on-the-fly his account. The creation is ok, I use the api route with a POST and behinf with a controller, I use User::create([...]) followed by Auth::login($user) ... Everything is fine from the point of view of the API script, the user is connected.
But from the form page point of view, if the user change or refresh the page, he won't be connected. I think it's about security reasons, but how can I do that ? I'm new in Laravel, I just want to found the proper "laravel" way.
Thank you.
edit:
In the routes file :
Route::post('/user', 'UserController@receiveData');
In the controller file :
class UserController extends Controller
{
public function receiveData (Request $request)
{
$response = new ServerResponse('User');
switch ( $action = $request->input('action') )
{
case 'create_user_from_email' :
/*if ( Auth::check() )
{
echo '(Connected, email:'.Auth::user()->email.')';
}
else
{
echo '(Not connected)';
}*/
if ( $email = $request->input('email') )
{
$errorToken = null;
if ( $user = User::createFromEmail($email, $errorToken) )
{
/* Données optionnelles */
$profileData = $request->input('profile') ? json_decode($request->input('profile'), true) : null;
$addressData = $request->input('address') ? json_decode($request->input('address'), true) : null;
/* Création du profile, de l'adresse et du compte en banque par défaut. */
$profile = $user->profile($profileData);
$address = $profile->primaryAddress($addressData);
$bankAccount = $user->primaryBankAccount();
/* Auto-connexion. */
Auth::login($user, true);
if ( Auth::check() )
{
$response->setStatus(0, 'New User created.');
$response->addData('user_id', $user->id);
$response->addData('profile_id', $profile->id);
$response->addData('address_id', $address->id);
$response->addData('bank_account_id', $bankAccount->id);
}
else
{
$response->setStatus(4, 'Unable to connect the new User !');
$response->addData('error', 'user_not_connected');
}
}
else
{
$response->setStatus(3, 'Unable to create a new User ! See "error" key.');
$response->addData('error', $errorToken);
}
}
else
{
$response->setStatus(2, 'There is email address !');
}
break;
default:
$response->setStatus(1, 'Action "'.$action.'" unhandled !');
break;
}
return $response->get();
}
}
javascript php laravel authentication
add a comment |
I have a form where at some point, the user can enter his email. So, I use it to create on-the-fly his account. The creation is ok, I use the api route with a POST and behinf with a controller, I use User::create([...]) followed by Auth::login($user) ... Everything is fine from the point of view of the API script, the user is connected.
But from the form page point of view, if the user change or refresh the page, he won't be connected. I think it's about security reasons, but how can I do that ? I'm new in Laravel, I just want to found the proper "laravel" way.
Thank you.
edit:
In the routes file :
Route::post('/user', 'UserController@receiveData');
In the controller file :
class UserController extends Controller
{
public function receiveData (Request $request)
{
$response = new ServerResponse('User');
switch ( $action = $request->input('action') )
{
case 'create_user_from_email' :
/*if ( Auth::check() )
{
echo '(Connected, email:'.Auth::user()->email.')';
}
else
{
echo '(Not connected)';
}*/
if ( $email = $request->input('email') )
{
$errorToken = null;
if ( $user = User::createFromEmail($email, $errorToken) )
{
/* Données optionnelles */
$profileData = $request->input('profile') ? json_decode($request->input('profile'), true) : null;
$addressData = $request->input('address') ? json_decode($request->input('address'), true) : null;
/* Création du profile, de l'adresse et du compte en banque par défaut. */
$profile = $user->profile($profileData);
$address = $profile->primaryAddress($addressData);
$bankAccount = $user->primaryBankAccount();
/* Auto-connexion. */
Auth::login($user, true);
if ( Auth::check() )
{
$response->setStatus(0, 'New User created.');
$response->addData('user_id', $user->id);
$response->addData('profile_id', $profile->id);
$response->addData('address_id', $address->id);
$response->addData('bank_account_id', $bankAccount->id);
}
else
{
$response->setStatus(4, 'Unable to connect the new User !');
$response->addData('error', 'user_not_connected');
}
}
else
{
$response->setStatus(3, 'Unable to create a new User ! See "error" key.');
$response->addData('error', $errorToken);
}
}
else
{
$response->setStatus(2, 'There is email address !');
}
break;
default:
$response->setStatus(1, 'Action "'.$action.'" unhandled !');
break;
}
return $response->get();
}
}
javascript php laravel authentication
Show some code you have done so far.
– Web Artisan
Nov 23 '18 at 15:06
Here, it's very basic... I don't know really what to show.
– Sébastien Bémelmans
Nov 23 '18 at 15:10
add a comment |
I have a form where at some point, the user can enter his email. So, I use it to create on-the-fly his account. The creation is ok, I use the api route with a POST and behinf with a controller, I use User::create([...]) followed by Auth::login($user) ... Everything is fine from the point of view of the API script, the user is connected.
But from the form page point of view, if the user change or refresh the page, he won't be connected. I think it's about security reasons, but how can I do that ? I'm new in Laravel, I just want to found the proper "laravel" way.
Thank you.
edit:
In the routes file :
Route::post('/user', 'UserController@receiveData');
In the controller file :
class UserController extends Controller
{
public function receiveData (Request $request)
{
$response = new ServerResponse('User');
switch ( $action = $request->input('action') )
{
case 'create_user_from_email' :
/*if ( Auth::check() )
{
echo '(Connected, email:'.Auth::user()->email.')';
}
else
{
echo '(Not connected)';
}*/
if ( $email = $request->input('email') )
{
$errorToken = null;
if ( $user = User::createFromEmail($email, $errorToken) )
{
/* Données optionnelles */
$profileData = $request->input('profile') ? json_decode($request->input('profile'), true) : null;
$addressData = $request->input('address') ? json_decode($request->input('address'), true) : null;
/* Création du profile, de l'adresse et du compte en banque par défaut. */
$profile = $user->profile($profileData);
$address = $profile->primaryAddress($addressData);
$bankAccount = $user->primaryBankAccount();
/* Auto-connexion. */
Auth::login($user, true);
if ( Auth::check() )
{
$response->setStatus(0, 'New User created.');
$response->addData('user_id', $user->id);
$response->addData('profile_id', $profile->id);
$response->addData('address_id', $address->id);
$response->addData('bank_account_id', $bankAccount->id);
}
else
{
$response->setStatus(4, 'Unable to connect the new User !');
$response->addData('error', 'user_not_connected');
}
}
else
{
$response->setStatus(3, 'Unable to create a new User ! See "error" key.');
$response->addData('error', $errorToken);
}
}
else
{
$response->setStatus(2, 'There is email address !');
}
break;
default:
$response->setStatus(1, 'Action "'.$action.'" unhandled !');
break;
}
return $response->get();
}
}
javascript php laravel authentication
I have a form where at some point, the user can enter his email. So, I use it to create on-the-fly his account. The creation is ok, I use the api route with a POST and behinf with a controller, I use User::create([...]) followed by Auth::login($user) ... Everything is fine from the point of view of the API script, the user is connected.
But from the form page point of view, if the user change or refresh the page, he won't be connected. I think it's about security reasons, but how can I do that ? I'm new in Laravel, I just want to found the proper "laravel" way.
Thank you.
edit:
In the routes file :
Route::post('/user', 'UserController@receiveData');
In the controller file :
class UserController extends Controller
{
public function receiveData (Request $request)
{
$response = new ServerResponse('User');
switch ( $action = $request->input('action') )
{
case 'create_user_from_email' :
/*if ( Auth::check() )
{
echo '(Connected, email:'.Auth::user()->email.')';
}
else
{
echo '(Not connected)';
}*/
if ( $email = $request->input('email') )
{
$errorToken = null;
if ( $user = User::createFromEmail($email, $errorToken) )
{
/* Données optionnelles */
$profileData = $request->input('profile') ? json_decode($request->input('profile'), true) : null;
$addressData = $request->input('address') ? json_decode($request->input('address'), true) : null;
/* Création du profile, de l'adresse et du compte en banque par défaut. */
$profile = $user->profile($profileData);
$address = $profile->primaryAddress($addressData);
$bankAccount = $user->primaryBankAccount();
/* Auto-connexion. */
Auth::login($user, true);
if ( Auth::check() )
{
$response->setStatus(0, 'New User created.');
$response->addData('user_id', $user->id);
$response->addData('profile_id', $profile->id);
$response->addData('address_id', $address->id);
$response->addData('bank_account_id', $bankAccount->id);
}
else
{
$response->setStatus(4, 'Unable to connect the new User !');
$response->addData('error', 'user_not_connected');
}
}
else
{
$response->setStatus(3, 'Unable to create a new User ! See "error" key.');
$response->addData('error', $errorToken);
}
}
else
{
$response->setStatus(2, 'There is email address !');
}
break;
default:
$response->setStatus(1, 'Action "'.$action.'" unhandled !');
break;
}
return $response->get();
}
}
javascript php laravel authentication
javascript php laravel authentication
edited Nov 23 '18 at 15:08
Sébastien Bémelmans
asked Nov 23 '18 at 15:05
Sébastien BémelmansSébastien Bémelmans
1281312
1281312
Show some code you have done so far.
– Web Artisan
Nov 23 '18 at 15:06
Here, it's very basic... I don't know really what to show.
– Sébastien Bémelmans
Nov 23 '18 at 15:10
add a comment |
Show some code you have done so far.
– Web Artisan
Nov 23 '18 at 15:06
Here, it's very basic... I don't know really what to show.
– Sébastien Bémelmans
Nov 23 '18 at 15:10
Show some code you have done so far.
– Web Artisan
Nov 23 '18 at 15:06
Show some code you have done so far.
– Web Artisan
Nov 23 '18 at 15:06
Here, it's very basic... I don't know really what to show.
– Sébastien Bémelmans
Nov 23 '18 at 15:10
Here, it's very basic... I don't know really what to show.
– Sébastien Bémelmans
Nov 23 '18 at 15:10
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449012%2flaravel-create-a-new-autoconnected-user-with-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449012%2flaravel-create-a-new-autoconnected-user-with-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
u vQT 0 LSgoL1c KjLK Tyl 70W5J2NE3 gU5,btC rDtmr,TYALaePe,uq0mE 6k,bQ56eAJT22Nb
Show some code you have done so far.
– Web Artisan
Nov 23 '18 at 15:06
Here, it's very basic... I don't know really what to show.
– Sébastien Bémelmans
Nov 23 '18 at 15:10