ScheduledThreadPoolExecutor doesn't await for TimerTask termination
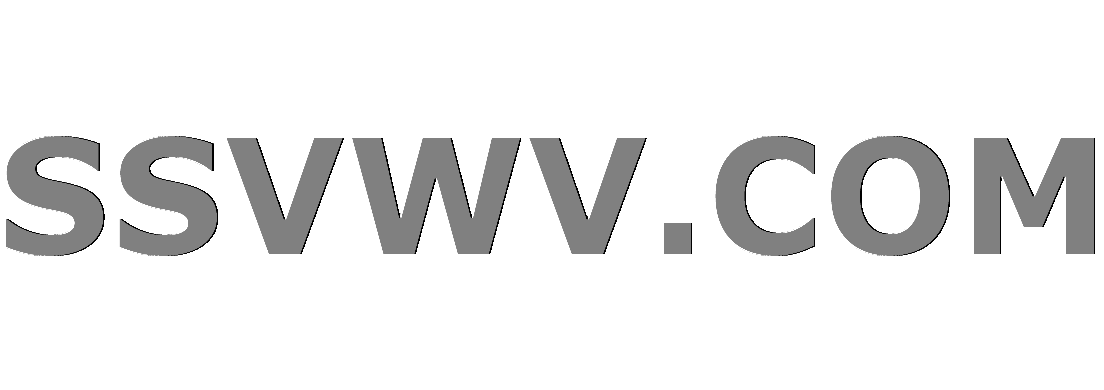
Multi tool use
I have to solve a java problem that I am not able to solve by myself.
I have a basic class and a test class :
public class Appender extends SMTPAppender{
private ScheduledThreadPoolExecutor scheduledThreadPoolExecutor = new ScheduledThreadPoolExecutor(1);
private final class MyTask extends TimerTask{
@Override
public void run(){
//some code
append(myEvent)
}
}
@Override
protected void append(ILoggingEvent event){
//some code
scheduledThreadPoolExecutor.schedule(task, cacheSize, TimeUnit.MILLISECONDS);
}
@Override
public synchronized void stop(){
super.stop()
scheduledThreadPoolExecutor.shutdown();
scheduledThreadPoolExecutor.awaitTermination(cacheSize, TimeUnit.MILLISECONDS);
}
}
public class AppenderTest {
@Test
public void myTest(){
Appender appender = new Appender();
methodWhichLogSomeLog(); // will call appender.append()
appender.stop();
//some test code
}
}
I am waiting for that my appender.stop() overrided has to wait for my ScheduledThreadPoolExecutor and its scheduled TimerTask.
Actually, my appender.stop() doesn't wait for this scheduled task and I don't know why. It seems to be the awaitTermination() role.
java timertask scheduledexecutorservice
|
show 3 more comments
I have to solve a java problem that I am not able to solve by myself.
I have a basic class and a test class :
public class Appender extends SMTPAppender{
private ScheduledThreadPoolExecutor scheduledThreadPoolExecutor = new ScheduledThreadPoolExecutor(1);
private final class MyTask extends TimerTask{
@Override
public void run(){
//some code
append(myEvent)
}
}
@Override
protected void append(ILoggingEvent event){
//some code
scheduledThreadPoolExecutor.schedule(task, cacheSize, TimeUnit.MILLISECONDS);
}
@Override
public synchronized void stop(){
super.stop()
scheduledThreadPoolExecutor.shutdown();
scheduledThreadPoolExecutor.awaitTermination(cacheSize, TimeUnit.MILLISECONDS);
}
}
public class AppenderTest {
@Test
public void myTest(){
Appender appender = new Appender();
methodWhichLogSomeLog(); // will call appender.append()
appender.stop();
//some test code
}
}
I am waiting for that my appender.stop() overrided has to wait for my ScheduledThreadPoolExecutor and its scheduled TimerTask.
Actually, my appender.stop() doesn't wait for this scheduled task and I don't know why. It seems to be the awaitTermination() role.
java timertask scheduledexecutorservice
How do you know that ??:>
– Antoniossss
Nov 23 '18 at 19:32
Because in debug, the main thread continu to process and doesn't wait for the new thread (ScheduledThreadPoolExecutor) which has to terminate after TimerTask schedule.
– Babi
Nov 26 '18 at 8:46
Are you sure? Since we are talking about concurrent execution, task could finish when you were stepping (if you are holding only 1 thread insteed of all). Also I am not sure how it should behave as awaitTerminatiion waits unitl currently queued tasks are finished. Its possible that scheduled tasks are not counted as "queued" due its undetermined delay.
– Antoniossss
Nov 26 '18 at 9:00
Thing is that my ScheduleThreadPoolExecutor (size 1), is : t - Initialize..... t1 - Scheduled --> schedule(myTask, 3000, milliseconds)..... t2 - Code reach scheduleThreadPool.shutdown() AND scheduleThreadPool.awaitForTermination() before myTask (TimerTask) start..... t3 - Error in my test because not enough data collected because of myTask didn't send event that it shall send.
– Babi
Nov 26 '18 at 14:02
If you shutdown the pool, scheduled tasks wont start. You will have to wait for it to start if you need that.
– Antoniossss
Nov 27 '18 at 7:35
|
show 3 more comments
I have to solve a java problem that I am not able to solve by myself.
I have a basic class and a test class :
public class Appender extends SMTPAppender{
private ScheduledThreadPoolExecutor scheduledThreadPoolExecutor = new ScheduledThreadPoolExecutor(1);
private final class MyTask extends TimerTask{
@Override
public void run(){
//some code
append(myEvent)
}
}
@Override
protected void append(ILoggingEvent event){
//some code
scheduledThreadPoolExecutor.schedule(task, cacheSize, TimeUnit.MILLISECONDS);
}
@Override
public synchronized void stop(){
super.stop()
scheduledThreadPoolExecutor.shutdown();
scheduledThreadPoolExecutor.awaitTermination(cacheSize, TimeUnit.MILLISECONDS);
}
}
public class AppenderTest {
@Test
public void myTest(){
Appender appender = new Appender();
methodWhichLogSomeLog(); // will call appender.append()
appender.stop();
//some test code
}
}
I am waiting for that my appender.stop() overrided has to wait for my ScheduledThreadPoolExecutor and its scheduled TimerTask.
Actually, my appender.stop() doesn't wait for this scheduled task and I don't know why. It seems to be the awaitTermination() role.
java timertask scheduledexecutorservice
I have to solve a java problem that I am not able to solve by myself.
I have a basic class and a test class :
public class Appender extends SMTPAppender{
private ScheduledThreadPoolExecutor scheduledThreadPoolExecutor = new ScheduledThreadPoolExecutor(1);
private final class MyTask extends TimerTask{
@Override
public void run(){
//some code
append(myEvent)
}
}
@Override
protected void append(ILoggingEvent event){
//some code
scheduledThreadPoolExecutor.schedule(task, cacheSize, TimeUnit.MILLISECONDS);
}
@Override
public synchronized void stop(){
super.stop()
scheduledThreadPoolExecutor.shutdown();
scheduledThreadPoolExecutor.awaitTermination(cacheSize, TimeUnit.MILLISECONDS);
}
}
public class AppenderTest {
@Test
public void myTest(){
Appender appender = new Appender();
methodWhichLogSomeLog(); // will call appender.append()
appender.stop();
//some test code
}
}
I am waiting for that my appender.stop() overrided has to wait for my ScheduledThreadPoolExecutor and its scheduled TimerTask.
Actually, my appender.stop() doesn't wait for this scheduled task and I don't know why. It seems to be the awaitTermination() role.
java timertask scheduledexecutorservice
java timertask scheduledexecutorservice
edited Nov 23 '18 at 15:43
Babi
asked Nov 23 '18 at 15:08


BabiBabi
66
66
How do you know that ??:>
– Antoniossss
Nov 23 '18 at 19:32
Because in debug, the main thread continu to process and doesn't wait for the new thread (ScheduledThreadPoolExecutor) which has to terminate after TimerTask schedule.
– Babi
Nov 26 '18 at 8:46
Are you sure? Since we are talking about concurrent execution, task could finish when you were stepping (if you are holding only 1 thread insteed of all). Also I am not sure how it should behave as awaitTerminatiion waits unitl currently queued tasks are finished. Its possible that scheduled tasks are not counted as "queued" due its undetermined delay.
– Antoniossss
Nov 26 '18 at 9:00
Thing is that my ScheduleThreadPoolExecutor (size 1), is : t - Initialize..... t1 - Scheduled --> schedule(myTask, 3000, milliseconds)..... t2 - Code reach scheduleThreadPool.shutdown() AND scheduleThreadPool.awaitForTermination() before myTask (TimerTask) start..... t3 - Error in my test because not enough data collected because of myTask didn't send event that it shall send.
– Babi
Nov 26 '18 at 14:02
If you shutdown the pool, scheduled tasks wont start. You will have to wait for it to start if you need that.
– Antoniossss
Nov 27 '18 at 7:35
|
show 3 more comments
How do you know that ??:>
– Antoniossss
Nov 23 '18 at 19:32
Because in debug, the main thread continu to process and doesn't wait for the new thread (ScheduledThreadPoolExecutor) which has to terminate after TimerTask schedule.
– Babi
Nov 26 '18 at 8:46
Are you sure? Since we are talking about concurrent execution, task could finish when you were stepping (if you are holding only 1 thread insteed of all). Also I am not sure how it should behave as awaitTerminatiion waits unitl currently queued tasks are finished. Its possible that scheduled tasks are not counted as "queued" due its undetermined delay.
– Antoniossss
Nov 26 '18 at 9:00
Thing is that my ScheduleThreadPoolExecutor (size 1), is : t - Initialize..... t1 - Scheduled --> schedule(myTask, 3000, milliseconds)..... t2 - Code reach scheduleThreadPool.shutdown() AND scheduleThreadPool.awaitForTermination() before myTask (TimerTask) start..... t3 - Error in my test because not enough data collected because of myTask didn't send event that it shall send.
– Babi
Nov 26 '18 at 14:02
If you shutdown the pool, scheduled tasks wont start. You will have to wait for it to start if you need that.
– Antoniossss
Nov 27 '18 at 7:35
How do you know that ??:>
– Antoniossss
Nov 23 '18 at 19:32
How do you know that ??:>
– Antoniossss
Nov 23 '18 at 19:32
Because in debug, the main thread continu to process and doesn't wait for the new thread (ScheduledThreadPoolExecutor) which has to terminate after TimerTask schedule.
– Babi
Nov 26 '18 at 8:46
Because in debug, the main thread continu to process and doesn't wait for the new thread (ScheduledThreadPoolExecutor) which has to terminate after TimerTask schedule.
– Babi
Nov 26 '18 at 8:46
Are you sure? Since we are talking about concurrent execution, task could finish when you were stepping (if you are holding only 1 thread insteed of all). Also I am not sure how it should behave as awaitTerminatiion waits unitl currently queued tasks are finished. Its possible that scheduled tasks are not counted as "queued" due its undetermined delay.
– Antoniossss
Nov 26 '18 at 9:00
Are you sure? Since we are talking about concurrent execution, task could finish when you were stepping (if you are holding only 1 thread insteed of all). Also I am not sure how it should behave as awaitTerminatiion waits unitl currently queued tasks are finished. Its possible that scheduled tasks are not counted as "queued" due its undetermined delay.
– Antoniossss
Nov 26 '18 at 9:00
Thing is that my ScheduleThreadPoolExecutor (size 1), is : t - Initialize..... t1 - Scheduled --> schedule(myTask, 3000, milliseconds)..... t2 - Code reach scheduleThreadPool.shutdown() AND scheduleThreadPool.awaitForTermination() before myTask (TimerTask) start..... t3 - Error in my test because not enough data collected because of myTask didn't send event that it shall send.
– Babi
Nov 26 '18 at 14:02
Thing is that my ScheduleThreadPoolExecutor (size 1), is : t - Initialize..... t1 - Scheduled --> schedule(myTask, 3000, milliseconds)..... t2 - Code reach scheduleThreadPool.shutdown() AND scheduleThreadPool.awaitForTermination() before myTask (TimerTask) start..... t3 - Error in my test because not enough data collected because of myTask didn't send event that it shall send.
– Babi
Nov 26 '18 at 14:02
If you shutdown the pool, scheduled tasks wont start. You will have to wait for it to start if you need that.
– Antoniossss
Nov 27 '18 at 7:35
If you shutdown the pool, scheduled tasks wont start. You will have to wait for it to start if you need that.
– Antoniossss
Nov 27 '18 at 7:35
|
show 3 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449041%2fscheduledthreadpoolexecutor-doesnt-await-for-timertask-termination%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53449041%2fscheduledthreadpoolexecutor-doesnt-await-for-timertask-termination%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AQYqCOWwUf J8po2eHDuV9j2B7glbdSpM,hge,pG8HwN6,6VtvzU5W1bg XxtJ
How do you know that ??:>
– Antoniossss
Nov 23 '18 at 19:32
Because in debug, the main thread continu to process and doesn't wait for the new thread (ScheduledThreadPoolExecutor) which has to terminate after TimerTask schedule.
– Babi
Nov 26 '18 at 8:46
Are you sure? Since we are talking about concurrent execution, task could finish when you were stepping (if you are holding only 1 thread insteed of all). Also I am not sure how it should behave as awaitTerminatiion waits unitl currently queued tasks are finished. Its possible that scheduled tasks are not counted as "queued" due its undetermined delay.
– Antoniossss
Nov 26 '18 at 9:00
Thing is that my ScheduleThreadPoolExecutor (size 1), is : t - Initialize..... t1 - Scheduled --> schedule(myTask, 3000, milliseconds)..... t2 - Code reach scheduleThreadPool.shutdown() AND scheduleThreadPool.awaitForTermination() before myTask (TimerTask) start..... t3 - Error in my test because not enough data collected because of myTask didn't send event that it shall send.
– Babi
Nov 26 '18 at 14:02
If you shutdown the pool, scheduled tasks wont start. You will have to wait for it to start if you need that.
– Antoniossss
Nov 27 '18 at 7:35