Populate and print array with random numbers using C
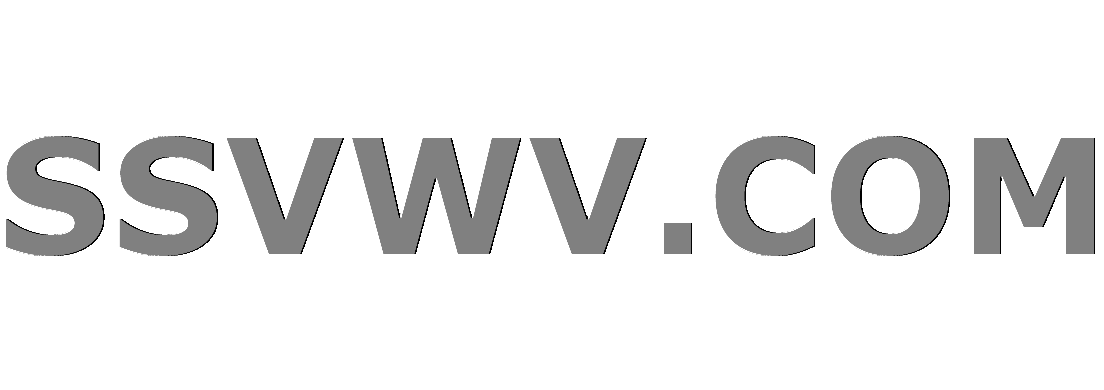
Multi tool use
I'm trying to write a program that will populate an array of 100 elements with numbers between 1 and 22, and then print the array in a 20 x 5 table. I was able to populate the array and print it, but can only get it to work with numbers 1-100, how can I change it to only do numbers 1-22?
#include <stdio.h>
#include <stdlib.h>
#define ARY_SIZE 100
void random (int randNos);
void printArray (int data, int size, int lineSize);
int main(void)
{
int randNos [ARY_SIZE];
random(randNos);
printArray(randNos, ARY_SIZE, 20);
return 0;
}
void random (int randNos)
{
int oneRandNo;
int haveRand[ARY_SIZE] = {0};
for (int i = 0; i < ARY_SIZE; i++)
{
do
{
oneRandNo = rand() % ARY_SIZE;
} while (haveRand[oneRandNo] == 1);
haveRand[oneRandNo] = 1;
randNos[i] = oneRandNo;
}
return;
}
void printArray (int data, int size, int lineSize)
{
int numPrinted = 0;
printf("n");
for (int i = 0; i < size; i++)
{
numPrinted++;
printf("%2d ", data[i]);
if (numPrinted >= lineSize)
{
printf("n");
numPrinted = 0;
}
}
printf("n");
return;
}
c arrays random
add a comment |
I'm trying to write a program that will populate an array of 100 elements with numbers between 1 and 22, and then print the array in a 20 x 5 table. I was able to populate the array and print it, but can only get it to work with numbers 1-100, how can I change it to only do numbers 1-22?
#include <stdio.h>
#include <stdlib.h>
#define ARY_SIZE 100
void random (int randNos);
void printArray (int data, int size, int lineSize);
int main(void)
{
int randNos [ARY_SIZE];
random(randNos);
printArray(randNos, ARY_SIZE, 20);
return 0;
}
void random (int randNos)
{
int oneRandNo;
int haveRand[ARY_SIZE] = {0};
for (int i = 0; i < ARY_SIZE; i++)
{
do
{
oneRandNo = rand() % ARY_SIZE;
} while (haveRand[oneRandNo] == 1);
haveRand[oneRandNo] = 1;
randNos[i] = oneRandNo;
}
return;
}
void printArray (int data, int size, int lineSize)
{
int numPrinted = 0;
printf("n");
for (int i = 0; i < size; i++)
{
numPrinted++;
printf("%2d ", data[i]);
if (numPrinted >= lineSize)
{
printf("n");
numPrinted = 0;
}
}
printf("n");
return;
}
c arrays random
rand() % ARY_SIZE
–>rand() % 22 + 1
– Swordfish
Nov 23 '18 at 1:25
You're using thehaveRand
array to avoid numbers that have already been used, but if you need to fill 100 array elements with random numbers from 1 to 22, then this is going to be impossible. Yourdo ... while
loop will run forever.
– squeamish ossifrage
Nov 23 '18 at 1:28
i would suggest populating an array with the sequential values 1-22 and then shuffling the array. it will be much faster.
– MFisherKDX
Nov 23 '18 at 1:29
@MFisherKDX you can fill an array of 100 elements with random numbers [1, 22] without having duplicates?
– Swordfish
Nov 23 '18 at 1:32
1
@Swordfish ... no. i guess i am confused on the problem statement. the OP should clarify what she is asking ... this could be impossible.
– MFisherKDX
Nov 23 '18 at 1:34
add a comment |
I'm trying to write a program that will populate an array of 100 elements with numbers between 1 and 22, and then print the array in a 20 x 5 table. I was able to populate the array and print it, but can only get it to work with numbers 1-100, how can I change it to only do numbers 1-22?
#include <stdio.h>
#include <stdlib.h>
#define ARY_SIZE 100
void random (int randNos);
void printArray (int data, int size, int lineSize);
int main(void)
{
int randNos [ARY_SIZE];
random(randNos);
printArray(randNos, ARY_SIZE, 20);
return 0;
}
void random (int randNos)
{
int oneRandNo;
int haveRand[ARY_SIZE] = {0};
for (int i = 0; i < ARY_SIZE; i++)
{
do
{
oneRandNo = rand() % ARY_SIZE;
} while (haveRand[oneRandNo] == 1);
haveRand[oneRandNo] = 1;
randNos[i] = oneRandNo;
}
return;
}
void printArray (int data, int size, int lineSize)
{
int numPrinted = 0;
printf("n");
for (int i = 0; i < size; i++)
{
numPrinted++;
printf("%2d ", data[i]);
if (numPrinted >= lineSize)
{
printf("n");
numPrinted = 0;
}
}
printf("n");
return;
}
c arrays random
I'm trying to write a program that will populate an array of 100 elements with numbers between 1 and 22, and then print the array in a 20 x 5 table. I was able to populate the array and print it, but can only get it to work with numbers 1-100, how can I change it to only do numbers 1-22?
#include <stdio.h>
#include <stdlib.h>
#define ARY_SIZE 100
void random (int randNos);
void printArray (int data, int size, int lineSize);
int main(void)
{
int randNos [ARY_SIZE];
random(randNos);
printArray(randNos, ARY_SIZE, 20);
return 0;
}
void random (int randNos)
{
int oneRandNo;
int haveRand[ARY_SIZE] = {0};
for (int i = 0; i < ARY_SIZE; i++)
{
do
{
oneRandNo = rand() % ARY_SIZE;
} while (haveRand[oneRandNo] == 1);
haveRand[oneRandNo] = 1;
randNos[i] = oneRandNo;
}
return;
}
void printArray (int data, int size, int lineSize)
{
int numPrinted = 0;
printf("n");
for (int i = 0; i < size; i++)
{
numPrinted++;
printf("%2d ", data[i]);
if (numPrinted >= lineSize)
{
printf("n");
numPrinted = 0;
}
}
printf("n");
return;
}
c arrays random
c arrays random
asked Nov 23 '18 at 1:21


Sarah
62
62
rand() % ARY_SIZE
–>rand() % 22 + 1
– Swordfish
Nov 23 '18 at 1:25
You're using thehaveRand
array to avoid numbers that have already been used, but if you need to fill 100 array elements with random numbers from 1 to 22, then this is going to be impossible. Yourdo ... while
loop will run forever.
– squeamish ossifrage
Nov 23 '18 at 1:28
i would suggest populating an array with the sequential values 1-22 and then shuffling the array. it will be much faster.
– MFisherKDX
Nov 23 '18 at 1:29
@MFisherKDX you can fill an array of 100 elements with random numbers [1, 22] without having duplicates?
– Swordfish
Nov 23 '18 at 1:32
1
@Swordfish ... no. i guess i am confused on the problem statement. the OP should clarify what she is asking ... this could be impossible.
– MFisherKDX
Nov 23 '18 at 1:34
add a comment |
rand() % ARY_SIZE
–>rand() % 22 + 1
– Swordfish
Nov 23 '18 at 1:25
You're using thehaveRand
array to avoid numbers that have already been used, but if you need to fill 100 array elements with random numbers from 1 to 22, then this is going to be impossible. Yourdo ... while
loop will run forever.
– squeamish ossifrage
Nov 23 '18 at 1:28
i would suggest populating an array with the sequential values 1-22 and then shuffling the array. it will be much faster.
– MFisherKDX
Nov 23 '18 at 1:29
@MFisherKDX you can fill an array of 100 elements with random numbers [1, 22] without having duplicates?
– Swordfish
Nov 23 '18 at 1:32
1
@Swordfish ... no. i guess i am confused on the problem statement. the OP should clarify what she is asking ... this could be impossible.
– MFisherKDX
Nov 23 '18 at 1:34
rand() % ARY_SIZE
–> rand() % 22 + 1
– Swordfish
Nov 23 '18 at 1:25
rand() % ARY_SIZE
–> rand() % 22 + 1
– Swordfish
Nov 23 '18 at 1:25
You're using the
haveRand
array to avoid numbers that have already been used, but if you need to fill 100 array elements with random numbers from 1 to 22, then this is going to be impossible. Your do ... while
loop will run forever.– squeamish ossifrage
Nov 23 '18 at 1:28
You're using the
haveRand
array to avoid numbers that have already been used, but if you need to fill 100 array elements with random numbers from 1 to 22, then this is going to be impossible. Your do ... while
loop will run forever.– squeamish ossifrage
Nov 23 '18 at 1:28
i would suggest populating an array with the sequential values 1-22 and then shuffling the array. it will be much faster.
– MFisherKDX
Nov 23 '18 at 1:29
i would suggest populating an array with the sequential values 1-22 and then shuffling the array. it will be much faster.
– MFisherKDX
Nov 23 '18 at 1:29
@MFisherKDX you can fill an array of 100 elements with random numbers [1, 22] without having duplicates?
– Swordfish
Nov 23 '18 at 1:32
@MFisherKDX you can fill an array of 100 elements with random numbers [1, 22] without having duplicates?
– Swordfish
Nov 23 '18 at 1:32
1
1
@Swordfish ... no. i guess i am confused on the problem statement. the OP should clarify what she is asking ... this could be impossible.
– MFisherKDX
Nov 23 '18 at 1:34
@Swordfish ... no. i guess i am confused on the problem statement. the OP should clarify what she is asking ... this could be impossible.
– MFisherKDX
Nov 23 '18 at 1:34
add a comment |
1 Answer
1
active
oldest
votes
@Sarah Simply include time.h
header file (from the standard library), then rewrite your random function as follow:
void Random(int RandNos)
{
/*
Since your random numbers are between 1 and 22, they correspond to the remainder of
unsigned integers divided by 22 (which lie between 0 and 21) plus 1, to have the
desired range of numbers.
*/
int oneRandNo;
// Here, we seed the random generator in order to make the random number truly "random".
srand((unsigned)time(NULL));
for(int i=0; i < ARY_SIZE; i++)
{
oneRandNo = ((unsigned )random() % 22 + 1);
randNos[i] = oneRandNo; // We record the generate random number
}
}
Note: You are asked to include time.h
in order to use the time()
function. If you are
working under Linux Or Mac OSX, you can find more information about this function by
type the command man 3 time
in the terminal to easily access the documentation.
Also, naming your function random
will conflict with that of the standard library. That is why I use Random
instead.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439645%2fpopulate-and-print-array-with-random-numbers-using-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
@Sarah Simply include time.h
header file (from the standard library), then rewrite your random function as follow:
void Random(int RandNos)
{
/*
Since your random numbers are between 1 and 22, they correspond to the remainder of
unsigned integers divided by 22 (which lie between 0 and 21) plus 1, to have the
desired range of numbers.
*/
int oneRandNo;
// Here, we seed the random generator in order to make the random number truly "random".
srand((unsigned)time(NULL));
for(int i=0; i < ARY_SIZE; i++)
{
oneRandNo = ((unsigned )random() % 22 + 1);
randNos[i] = oneRandNo; // We record the generate random number
}
}
Note: You are asked to include time.h
in order to use the time()
function. If you are
working under Linux Or Mac OSX, you can find more information about this function by
type the command man 3 time
in the terminal to easily access the documentation.
Also, naming your function random
will conflict with that of the standard library. That is why I use Random
instead.
add a comment |
@Sarah Simply include time.h
header file (from the standard library), then rewrite your random function as follow:
void Random(int RandNos)
{
/*
Since your random numbers are between 1 and 22, they correspond to the remainder of
unsigned integers divided by 22 (which lie between 0 and 21) plus 1, to have the
desired range of numbers.
*/
int oneRandNo;
// Here, we seed the random generator in order to make the random number truly "random".
srand((unsigned)time(NULL));
for(int i=0; i < ARY_SIZE; i++)
{
oneRandNo = ((unsigned )random() % 22 + 1);
randNos[i] = oneRandNo; // We record the generate random number
}
}
Note: You are asked to include time.h
in order to use the time()
function. If you are
working under Linux Or Mac OSX, you can find more information about this function by
type the command man 3 time
in the terminal to easily access the documentation.
Also, naming your function random
will conflict with that of the standard library. That is why I use Random
instead.
add a comment |
@Sarah Simply include time.h
header file (from the standard library), then rewrite your random function as follow:
void Random(int RandNos)
{
/*
Since your random numbers are between 1 and 22, they correspond to the remainder of
unsigned integers divided by 22 (which lie between 0 and 21) plus 1, to have the
desired range of numbers.
*/
int oneRandNo;
// Here, we seed the random generator in order to make the random number truly "random".
srand((unsigned)time(NULL));
for(int i=0; i < ARY_SIZE; i++)
{
oneRandNo = ((unsigned )random() % 22 + 1);
randNos[i] = oneRandNo; // We record the generate random number
}
}
Note: You are asked to include time.h
in order to use the time()
function. If you are
working under Linux Or Mac OSX, you can find more information about this function by
type the command man 3 time
in the terminal to easily access the documentation.
Also, naming your function random
will conflict with that of the standard library. That is why I use Random
instead.
@Sarah Simply include time.h
header file (from the standard library), then rewrite your random function as follow:
void Random(int RandNos)
{
/*
Since your random numbers are between 1 and 22, they correspond to the remainder of
unsigned integers divided by 22 (which lie between 0 and 21) plus 1, to have the
desired range of numbers.
*/
int oneRandNo;
// Here, we seed the random generator in order to make the random number truly "random".
srand((unsigned)time(NULL));
for(int i=0; i < ARY_SIZE; i++)
{
oneRandNo = ((unsigned )random() % 22 + 1);
randNos[i] = oneRandNo; // We record the generate random number
}
}
Note: You are asked to include time.h
in order to use the time()
function. If you are
working under Linux Or Mac OSX, you can find more information about this function by
type the command man 3 time
in the terminal to easily access the documentation.
Also, naming your function random
will conflict with that of the standard library. That is why I use Random
instead.
answered Nov 23 '18 at 2:01


eapetcho
42927
42927
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53439645%2fpopulate-and-print-array-with-random-numbers-using-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uNImJ5ieFSbZoOvztkqRP6oUyFlUYpnUh6HNS7q CSqbTAO,cs TC5R,nylEAz0GMWVO 2Z,xULtVCj3k6PqvKhx8yZI,0Z
rand() % ARY_SIZE
–>rand() % 22 + 1
– Swordfish
Nov 23 '18 at 1:25
You're using the
haveRand
array to avoid numbers that have already been used, but if you need to fill 100 array elements with random numbers from 1 to 22, then this is going to be impossible. Yourdo ... while
loop will run forever.– squeamish ossifrage
Nov 23 '18 at 1:28
i would suggest populating an array with the sequential values 1-22 and then shuffling the array. it will be much faster.
– MFisherKDX
Nov 23 '18 at 1:29
@MFisherKDX you can fill an array of 100 elements with random numbers [1, 22] without having duplicates?
– Swordfish
Nov 23 '18 at 1:32
1
@Swordfish ... no. i guess i am confused on the problem statement. the OP should clarify what she is asking ... this could be impossible.
– MFisherKDX
Nov 23 '18 at 1:34