Hidden Input Value not updating with Jquery
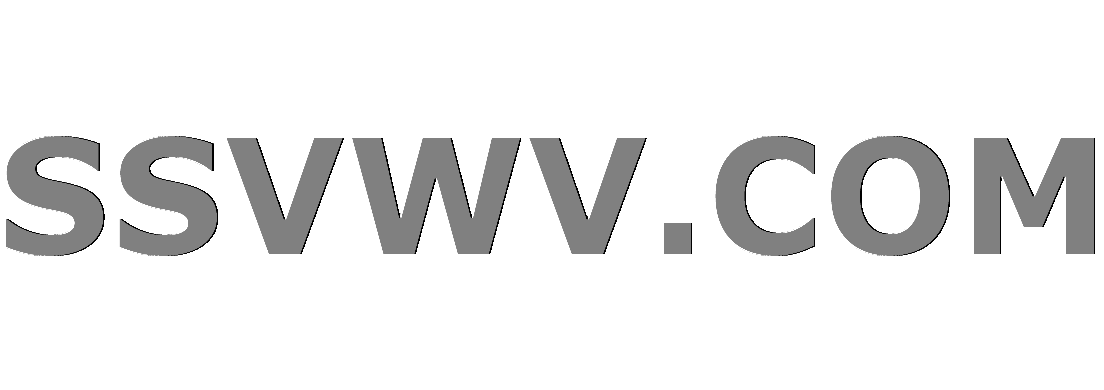
Multi tool use
up vote
-2
down vote
favorite
When a user clicks a checkbox, I need the jquery update_flavors function to run and update the hidden input the value attribute with all the flavors.
Currently, the update_flavors function does not seem to get called.
Updated JSFiddle: https://jsfiddle.net/ecqp9nwm/7/
HTML
<div class="row">
{% assign flavors = "apple, orange, cherry" | split: ","%}
{% for flavor in flavors %}
<div class="col-md-6">
<div class="form-check">
<label class="form-check-label" for="checkbox-{{flavor | handleize }}"><input name='contact[{{flavor| handleize }}]' type="checkbox" class="checkbox-flavor form-check-input" id="checkbox-{{flavor | handleize }}"> {{flavor}}</label>
</div>
</div>
{% endfor %}
</div>
<input type="hidden" id="checkbox-flavors" name="contact[flavor]" value="">
Jquery
<script>
function update_flavors() {
var allVals = ;
$('.checkbox-flavor :checked').each(function() {
allVals.push($(this).val());
});
$('#checkbox-flavors').val(allVals);
}
$(document).ready(function(){
$(".checkbox-flavor").click(function(){
update_flavors;
});
});
</script>
Solution:
I had a space in my selector! big oversight!
$('.checkbox-flavor :checked')
should have been $('.checkbox-flavor:checked')
and I needed to add a ()
to update_flavors();
working and improved: https://jsfiddle.net/ecqp9nwm/12
function update_flavors() {
var allVals = $('.checkbox-flavor:checked').map(function() {
return $(this).val()
}).get();
$('#checkbox-flavors').val(allVals.join(', '));
}
$(document).ready(function() {
$(".checkbox-flavor").change(update_flavors);
});
jquery
|
show 4 more comments
up vote
-2
down vote
favorite
When a user clicks a checkbox, I need the jquery update_flavors function to run and update the hidden input the value attribute with all the flavors.
Currently, the update_flavors function does not seem to get called.
Updated JSFiddle: https://jsfiddle.net/ecqp9nwm/7/
HTML
<div class="row">
{% assign flavors = "apple, orange, cherry" | split: ","%}
{% for flavor in flavors %}
<div class="col-md-6">
<div class="form-check">
<label class="form-check-label" for="checkbox-{{flavor | handleize }}"><input name='contact[{{flavor| handleize }}]' type="checkbox" class="checkbox-flavor form-check-input" id="checkbox-{{flavor | handleize }}"> {{flavor}}</label>
</div>
</div>
{% endfor %}
</div>
<input type="hidden" id="checkbox-flavors" name="contact[flavor]" value="">
Jquery
<script>
function update_flavors() {
var allVals = ;
$('.checkbox-flavor :checked').each(function() {
allVals.push($(this).val());
});
$('#checkbox-flavors').val(allVals);
}
$(document).ready(function(){
$(".checkbox-flavor").click(function(){
update_flavors;
});
});
</script>
Solution:
I had a space in my selector! big oversight!
$('.checkbox-flavor :checked')
should have been $('.checkbox-flavor:checked')
and I needed to add a ()
to update_flavors();
working and improved: https://jsfiddle.net/ecqp9nwm/12
function update_flavors() {
var allVals = $('.checkbox-flavor:checked').map(function() {
return $(this).val()
}).get();
$('#checkbox-flavors').val(allVals.join(', '));
}
$(document).ready(function() {
$(".checkbox-flavor").change(update_flavors);
});
jquery
1
stackoverflow.com/questions/9454645/… Don't repeat ids. Use classes instead.
– Taplar
2 days ago
Where do you have an element with the IDcheckbox-flavor
to be matched with$("#checkbox-flavor")
?
– j08691
2 days ago
Oh, good point. flavor would beapple
,orange
orcherry
(i guess?), so it's not even a dup id anyway. But still, you should use a class to avoid that issue.
– Taplar
2 days ago
@j08691 typo, corrected, but still does not run. See js fiddle
– user2012677
2 days ago
1
update_flavors();
You're missing your()
, or get rid of the outer anonymous function
– Taplar
2 days ago
|
show 4 more comments
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
When a user clicks a checkbox, I need the jquery update_flavors function to run and update the hidden input the value attribute with all the flavors.
Currently, the update_flavors function does not seem to get called.
Updated JSFiddle: https://jsfiddle.net/ecqp9nwm/7/
HTML
<div class="row">
{% assign flavors = "apple, orange, cherry" | split: ","%}
{% for flavor in flavors %}
<div class="col-md-6">
<div class="form-check">
<label class="form-check-label" for="checkbox-{{flavor | handleize }}"><input name='contact[{{flavor| handleize }}]' type="checkbox" class="checkbox-flavor form-check-input" id="checkbox-{{flavor | handleize }}"> {{flavor}}</label>
</div>
</div>
{% endfor %}
</div>
<input type="hidden" id="checkbox-flavors" name="contact[flavor]" value="">
Jquery
<script>
function update_flavors() {
var allVals = ;
$('.checkbox-flavor :checked').each(function() {
allVals.push($(this).val());
});
$('#checkbox-flavors').val(allVals);
}
$(document).ready(function(){
$(".checkbox-flavor").click(function(){
update_flavors;
});
});
</script>
Solution:
I had a space in my selector! big oversight!
$('.checkbox-flavor :checked')
should have been $('.checkbox-flavor:checked')
and I needed to add a ()
to update_flavors();
working and improved: https://jsfiddle.net/ecqp9nwm/12
function update_flavors() {
var allVals = $('.checkbox-flavor:checked').map(function() {
return $(this).val()
}).get();
$('#checkbox-flavors').val(allVals.join(', '));
}
$(document).ready(function() {
$(".checkbox-flavor").change(update_flavors);
});
jquery
When a user clicks a checkbox, I need the jquery update_flavors function to run and update the hidden input the value attribute with all the flavors.
Currently, the update_flavors function does not seem to get called.
Updated JSFiddle: https://jsfiddle.net/ecqp9nwm/7/
HTML
<div class="row">
{% assign flavors = "apple, orange, cherry" | split: ","%}
{% for flavor in flavors %}
<div class="col-md-6">
<div class="form-check">
<label class="form-check-label" for="checkbox-{{flavor | handleize }}"><input name='contact[{{flavor| handleize }}]' type="checkbox" class="checkbox-flavor form-check-input" id="checkbox-{{flavor | handleize }}"> {{flavor}}</label>
</div>
</div>
{% endfor %}
</div>
<input type="hidden" id="checkbox-flavors" name="contact[flavor]" value="">
Jquery
<script>
function update_flavors() {
var allVals = ;
$('.checkbox-flavor :checked').each(function() {
allVals.push($(this).val());
});
$('#checkbox-flavors').val(allVals);
}
$(document).ready(function(){
$(".checkbox-flavor").click(function(){
update_flavors;
});
});
</script>
Solution:
I had a space in my selector! big oversight!
$('.checkbox-flavor :checked')
should have been $('.checkbox-flavor:checked')
and I needed to add a ()
to update_flavors();
working and improved: https://jsfiddle.net/ecqp9nwm/12
function update_flavors() {
var allVals = $('.checkbox-flavor:checked').map(function() {
return $(this).val()
}).get();
$('#checkbox-flavors').val(allVals.join(', '));
}
$(document).ready(function() {
$(".checkbox-flavor").change(update_flavors);
});
jquery
jquery
edited 2 days ago
asked 2 days ago
user2012677
1,37341435
1,37341435
1
stackoverflow.com/questions/9454645/… Don't repeat ids. Use classes instead.
– Taplar
2 days ago
Where do you have an element with the IDcheckbox-flavor
to be matched with$("#checkbox-flavor")
?
– j08691
2 days ago
Oh, good point. flavor would beapple
,orange
orcherry
(i guess?), so it's not even a dup id anyway. But still, you should use a class to avoid that issue.
– Taplar
2 days ago
@j08691 typo, corrected, but still does not run. See js fiddle
– user2012677
2 days ago
1
update_flavors();
You're missing your()
, or get rid of the outer anonymous function
– Taplar
2 days ago
|
show 4 more comments
1
stackoverflow.com/questions/9454645/… Don't repeat ids. Use classes instead.
– Taplar
2 days ago
Where do you have an element with the IDcheckbox-flavor
to be matched with$("#checkbox-flavor")
?
– j08691
2 days ago
Oh, good point. flavor would beapple
,orange
orcherry
(i guess?), so it's not even a dup id anyway. But still, you should use a class to avoid that issue.
– Taplar
2 days ago
@j08691 typo, corrected, but still does not run. See js fiddle
– user2012677
2 days ago
1
update_flavors();
You're missing your()
, or get rid of the outer anonymous function
– Taplar
2 days ago
1
1
stackoverflow.com/questions/9454645/… Don't repeat ids. Use classes instead.
– Taplar
2 days ago
stackoverflow.com/questions/9454645/… Don't repeat ids. Use classes instead.
– Taplar
2 days ago
Where do you have an element with the ID
checkbox-flavor
to be matched with $("#checkbox-flavor")
?– j08691
2 days ago
Where do you have an element with the ID
checkbox-flavor
to be matched with $("#checkbox-flavor")
?– j08691
2 days ago
Oh, good point. flavor would be
apple
, orange
or cherry
(i guess?), so it's not even a dup id anyway. But still, you should use a class to avoid that issue.– Taplar
2 days ago
Oh, good point. flavor would be
apple
, orange
or cherry
(i guess?), so it's not even a dup id anyway. But still, you should use a class to avoid that issue.– Taplar
2 days ago
@j08691 typo, corrected, but still does not run. See js fiddle
– user2012677
2 days ago
@j08691 typo, corrected, but still does not run. See js fiddle
– user2012677
2 days ago
1
1
update_flavors();
You're missing your ()
, or get rid of the outer anonymous function– Taplar
2 days ago
update_flavors();
You're missing your ()
, or get rid of the outer anonymous function– Taplar
2 days ago
|
show 4 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
Currently your checkboxes have no values to be added. They just have labels.
This site is such a pain in the hind quarters to post any sort of solution to, but try something like this:
function updateFlavors(e) {
console.log("update");
var checks = $(".checkbox-flavor:checked");
var vals = $.map(checks, function(elem, i) {
var inp = $(elem);
return inp.val();
});
$('#checkbox-flavors').val(vals.join(", "));}
$(document).ready(function() {
$(".checkbox-flavor").each(function() {
var inp = $(this);
inp.val(inp.parent().find("label").text());
})
$(".check_holder").on("change", ".checkbox-flavor", updateFlavors);
});
By using the change handler, you update when an item is checked or unchecked.
Also, by assigning the handler to a parent element, you only have one handler running instead of one for every input element.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Currently your checkboxes have no values to be added. They just have labels.
This site is such a pain in the hind quarters to post any sort of solution to, but try something like this:
function updateFlavors(e) {
console.log("update");
var checks = $(".checkbox-flavor:checked");
var vals = $.map(checks, function(elem, i) {
var inp = $(elem);
return inp.val();
});
$('#checkbox-flavors').val(vals.join(", "));}
$(document).ready(function() {
$(".checkbox-flavor").each(function() {
var inp = $(this);
inp.val(inp.parent().find("label").text());
})
$(".check_holder").on("change", ".checkbox-flavor", updateFlavors);
});
By using the change handler, you update when an item is checked or unchecked.
Also, by assigning the handler to a parent element, you only have one handler running instead of one for every input element.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
Currently your checkboxes have no values to be added. They just have labels.
This site is such a pain in the hind quarters to post any sort of solution to, but try something like this:
function updateFlavors(e) {
console.log("update");
var checks = $(".checkbox-flavor:checked");
var vals = $.map(checks, function(elem, i) {
var inp = $(elem);
return inp.val();
});
$('#checkbox-flavors').val(vals.join(", "));}
$(document).ready(function() {
$(".checkbox-flavor").each(function() {
var inp = $(this);
inp.val(inp.parent().find("label").text());
})
$(".check_holder").on("change", ".checkbox-flavor", updateFlavors);
});
By using the change handler, you update when an item is checked or unchecked.
Also, by assigning the handler to a parent element, you only have one handler running instead of one for every input element.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
Currently your checkboxes have no values to be added. They just have labels.
This site is such a pain in the hind quarters to post any sort of solution to, but try something like this:
function updateFlavors(e) {
console.log("update");
var checks = $(".checkbox-flavor:checked");
var vals = $.map(checks, function(elem, i) {
var inp = $(elem);
return inp.val();
});
$('#checkbox-flavors').val(vals.join(", "));}
$(document).ready(function() {
$(".checkbox-flavor").each(function() {
var inp = $(this);
inp.val(inp.parent().find("label").text());
})
$(".check_holder").on("change", ".checkbox-flavor", updateFlavors);
});
By using the change handler, you update when an item is checked or unchecked.
Also, by assigning the handler to a parent element, you only have one handler running instead of one for every input element.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Currently your checkboxes have no values to be added. They just have labels.
This site is such a pain in the hind quarters to post any sort of solution to, but try something like this:
function updateFlavors(e) {
console.log("update");
var checks = $(".checkbox-flavor:checked");
var vals = $.map(checks, function(elem, i) {
var inp = $(elem);
return inp.val();
});
$('#checkbox-flavors').val(vals.join(", "));}
$(document).ready(function() {
$(".checkbox-flavor").each(function() {
var inp = $(this);
inp.val(inp.parent().find("label").text());
})
$(".check_holder").on("change", ".checkbox-flavor", updateFlavors);
});
By using the change handler, you update when an item is checked or unchecked.
Also, by assigning the handler to a parent element, you only have one handler running instead of one for every input element.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 2 days ago
Devi8
1
1
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Devi8 is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402703%2fhidden-input-value-not-updating-with-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KAwGThzwcSz mlplXMQS8y o9X,tMjROUYboivzUcVzQ04dJ SKLLED6e ZfBoxoBEdyOZLOy6KJghK4 aS9aNb09J
1
stackoverflow.com/questions/9454645/… Don't repeat ids. Use classes instead.
– Taplar
2 days ago
Where do you have an element with the ID
checkbox-flavor
to be matched with$("#checkbox-flavor")
?– j08691
2 days ago
Oh, good point. flavor would be
apple
,orange
orcherry
(i guess?), so it's not even a dup id anyway. But still, you should use a class to avoid that issue.– Taplar
2 days ago
@j08691 typo, corrected, but still does not run. See js fiddle
– user2012677
2 days ago
1
update_flavors();
You're missing your()
, or get rid of the outer anonymous function– Taplar
2 days ago