Moore fsm VHDL Testbench
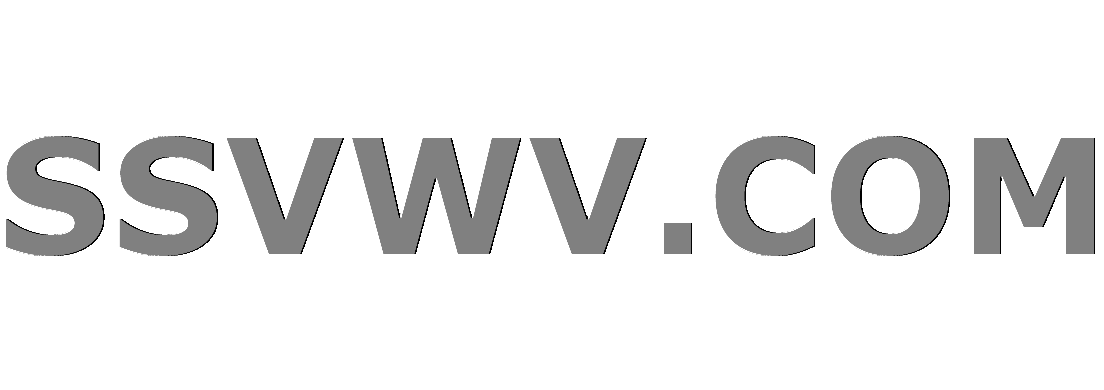
Multi tool use
up vote
0
down vote
favorite
Hello i got this FSM.
I wrote the VHDL code :
library ieee;
use ieee.std_logic_1164.all;
entity fsm is
port(
clk, reset : in std_logic;
level : in std_logic;
Moore_tick: out std_logic
);
end fsm;
architecture rtl of fsm is
type stateFsm_type is (s1, s2, s3); -- 3 states are required
signal stateFsm_reg, stateFsm_next : stateFsm_type;
begin
process(clk, reset)
begin
if (reset = '1') then -- go to state zero if reset
stateFsm_reg <= s1;
elsif (clk'event and clk = '1') then -- otherwise update the states
stateFsm_reg <= stateFsm_next;
else
null;
end if;
end process;
process(stateFsm_reg, level)
begin
-- store current state as next
stateFsm_next <= stateFsm_reg; -- required: when no case statement is satisfied
Moore_tick <= '0'; -- set tick to zero (so that 'tick = 1' is available for 1 cycle only)
case stateFsm_reg is
when s1 => -- if state is zero,
if level = '1' then -- and level is 1
stateFsm_next <= s2; -- then go to state edge.
end if;
when s2 =>
Moore_tick <= '1'; -- set the tick to 1.
if level = '1' then -- if level is 1,
stateFsm_next <= s3; --go to state one,
else
stateFsm_next <= s1; -- else go to state zero.
end if;
when s3 =>
if level = '0' then -- if level is 0,
stateFsm_next <= s1; -- then go to state zero.
end if;
end case;
end process;
end rtl;
So i compiled the code without errors. Currently i am trying to create a testbench so to be able to get the visuals so to be sure that it is workign correctly.
This is the code that i wrote for the testbench.
[

Sai Varun
464
464
Hello i made the changes that you sugggested. and i get these waveforms. Why i dont get output values for thestateFsm_reg
and thestateFsm_next
thought?
– Ioan Kats
yesterday
In yout testbench, you have declared the signalsstateFsm_reg
andstateFsm_next
as std_logic, instead they should be of your declared type stateFsm_type (as in your rtl code)
– Sai Varun
18 hours ago
Hello again i changed the signals i addedtype stateFsm_type is (s1, s2 , s3 )
signal stateFsm_reg, stateFsm_next : stateFsm_type ;
i get outputs1
but it does not change. All the time iss1
– Ioan Kats
11 hours ago
changed code and/or relevant figures might be more helpful to provide answers
– Sai Varun
11 hours ago
i updated the testbench and the waveforms!
– Ioan Kats
11 hours ago
|
show 3 more comments
Hello i made the changes that you sugggested. and i get these waveforms. Why i dont get output values for thestateFsm_reg
and thestateFsm_next
thought?
– Ioan Kats
yesterday
In yout testbench, you have declared the signalsstateFsm_reg
andstateFsm_next
as std_logic, instead they should be of your declared type stateFsm_type (as in your rtl code)
– Sai Varun
18 hours ago
Hello again i changed the signals i addedtype stateFsm_type is (s1, s2 , s3 )
signal stateFsm_reg, stateFsm_next : stateFsm_type ;
i get outputs1
but it does not change. All the time iss1
– Ioan Kats
11 hours ago
changed code and/or relevant figures might be more helpful to provide answers
– Sai Varun
11 hours ago
i updated the testbench and the waveforms!
– Ioan Kats
11 hours ago
Hello i made the changes that you sugggested. and i get these waveforms. Why i dont get output values for the
stateFsm_reg
and the stateFsm_next
thought?– Ioan Kats
yesterday
Hello i made the changes that you sugggested. and i get these waveforms. Why i dont get output values for the
stateFsm_reg
and the stateFsm_next
thought?– Ioan Kats
yesterday
In yout testbench, you have declared the signals
stateFsm_reg
and stateFsm_next
as std_logic, instead they should be of your declared type stateFsm_type (as in your rtl code)– Sai Varun
18 hours ago
In yout testbench, you have declared the signals
stateFsm_reg
and stateFsm_next
as std_logic, instead they should be of your declared type stateFsm_type (as in your rtl code)– Sai Varun
18 hours ago
Hello again i changed the signals i added
type stateFsm_type is (s1, s2 , s3 )
signal stateFsm_reg, stateFsm_next : stateFsm_type ;
i get output s1
but it does not change. All the time is s1
– Ioan Kats
11 hours ago
Hello again i changed the signals i added
type stateFsm_type is (s1, s2 , s3 )
signal stateFsm_reg, stateFsm_next : stateFsm_type ;
i get output s1
but it does not change. All the time is s1
– Ioan Kats
11 hours ago
changed code and/or relevant figures might be more helpful to provide answers
– Sai Varun
11 hours ago
changed code and/or relevant figures might be more helpful to provide answers
– Sai Varun
11 hours ago
i updated the testbench and the waveforms!
– Ioan Kats
11 hours ago
i updated the testbench and the waveforms!
– Ioan Kats
11 hours ago
|
show 3 more comments
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402732%2fmoore-fsm-vhdl-testbench%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ULij2E,ncIcvA Pw4bkfrZcQTZa
So far you don't appear to be asking a question and are simply stating you are stuck. What's got you stuck? Note that Moore_tick is an output from fsm and assigning it in the testbench creates a second driver where the value of the signal will the resolution of the two drivers.
– user1155120
2 days ago
Testing all the branches in your state machine can be done by manipulating reset and level and not driving Moore_tick.
– user1155120
2 days ago