Laravel Notification Redirect to Newly Created Item
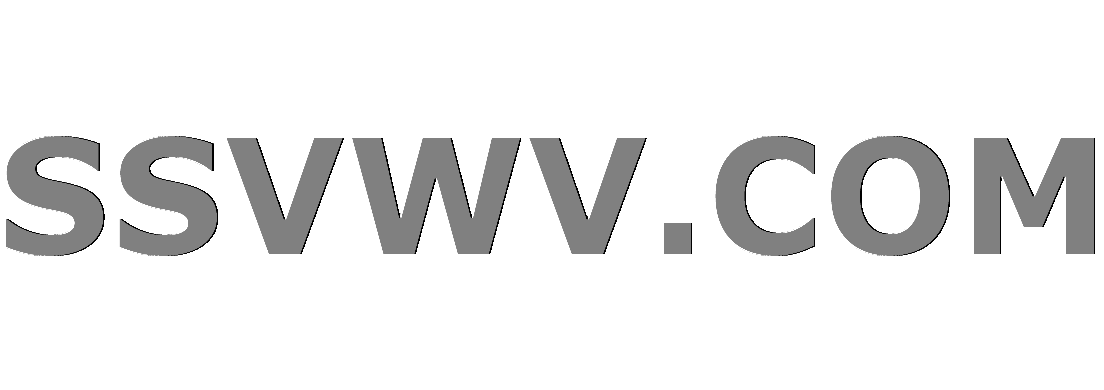
Multi tool use
up vote
0
down vote
favorite
I am trying to have it so that anytime a new Doctor is registered, it triggers a Laravel notification to send me an email. Here is my current code:
Controller
$doctor = new Doctor();
$doctor->practice_id = $request->practice;
$doctor->first_name = $request->first_name;
$doctor->last_name = $request->last_name;
$doctor->type = $request->type;
$doctor->npi = $request->npi;
$doctor->license = $request->license;
$doctor->dea = $request->dea;
$doctor->is_approved = 0;
$doctor->is_ffs = 0;
$doctor->ffs_id = null;
$doctor->save();
Notification::route('mail', 'admin@test.com')->notify(new NewDoctor($doctor));
NewDoctor Notification
public function via($notifiable)
{
return ['mail'];
}
public function toMail($notifiable)
{
return (new MailMessage)
->greeting('Doctor Registered!')
->line('A new doctor has been submitted for approval.')
->action('View Doctor', route('doctors.show', [$this->doctor]));
}
This is returning the following error: Undefined property: AppNotificationsNewDoctor::$doctor
I'm assuming that for some reason the doctor
id
just is not passing through, but I have no idea how to make that pass in.
laravel eloquent
add a comment |
up vote
0
down vote
favorite
I am trying to have it so that anytime a new Doctor is registered, it triggers a Laravel notification to send me an email. Here is my current code:
Controller
$doctor = new Doctor();
$doctor->practice_id = $request->practice;
$doctor->first_name = $request->first_name;
$doctor->last_name = $request->last_name;
$doctor->type = $request->type;
$doctor->npi = $request->npi;
$doctor->license = $request->license;
$doctor->dea = $request->dea;
$doctor->is_approved = 0;
$doctor->is_ffs = 0;
$doctor->ffs_id = null;
$doctor->save();
Notification::route('mail', 'admin@test.com')->notify(new NewDoctor($doctor));
NewDoctor Notification
public function via($notifiable)
{
return ['mail'];
}
public function toMail($notifiable)
{
return (new MailMessage)
->greeting('Doctor Registered!')
->line('A new doctor has been submitted for approval.')
->action('View Doctor', route('doctors.show', [$this->doctor]));
}
This is returning the following error: Undefined property: AppNotificationsNewDoctor::$doctor
I'm assuming that for some reason the doctor
id
just is not passing through, but I have no idea how to make that pass in.
laravel eloquent
You are passing$doctor
in constructor ofNewDoctor
. You need to have a__construct($someVariable)
constructor to initialize that value in your notification.
– InvincibleElf
Nov 21 at 1:06
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to have it so that anytime a new Doctor is registered, it triggers a Laravel notification to send me an email. Here is my current code:
Controller
$doctor = new Doctor();
$doctor->practice_id = $request->practice;
$doctor->first_name = $request->first_name;
$doctor->last_name = $request->last_name;
$doctor->type = $request->type;
$doctor->npi = $request->npi;
$doctor->license = $request->license;
$doctor->dea = $request->dea;
$doctor->is_approved = 0;
$doctor->is_ffs = 0;
$doctor->ffs_id = null;
$doctor->save();
Notification::route('mail', 'admin@test.com')->notify(new NewDoctor($doctor));
NewDoctor Notification
public function via($notifiable)
{
return ['mail'];
}
public function toMail($notifiable)
{
return (new MailMessage)
->greeting('Doctor Registered!')
->line('A new doctor has been submitted for approval.')
->action('View Doctor', route('doctors.show', [$this->doctor]));
}
This is returning the following error: Undefined property: AppNotificationsNewDoctor::$doctor
I'm assuming that for some reason the doctor
id
just is not passing through, but I have no idea how to make that pass in.
laravel eloquent
I am trying to have it so that anytime a new Doctor is registered, it triggers a Laravel notification to send me an email. Here is my current code:
Controller
$doctor = new Doctor();
$doctor->practice_id = $request->practice;
$doctor->first_name = $request->first_name;
$doctor->last_name = $request->last_name;
$doctor->type = $request->type;
$doctor->npi = $request->npi;
$doctor->license = $request->license;
$doctor->dea = $request->dea;
$doctor->is_approved = 0;
$doctor->is_ffs = 0;
$doctor->ffs_id = null;
$doctor->save();
Notification::route('mail', 'admin@test.com')->notify(new NewDoctor($doctor));
NewDoctor Notification
public function via($notifiable)
{
return ['mail'];
}
public function toMail($notifiable)
{
return (new MailMessage)
->greeting('Doctor Registered!')
->line('A new doctor has been submitted for approval.')
->action('View Doctor', route('doctors.show', [$this->doctor]));
}
This is returning the following error: Undefined property: AppNotificationsNewDoctor::$doctor
I'm assuming that for some reason the doctor
id
just is not passing through, but I have no idea how to make that pass in.
laravel eloquent
laravel eloquent
asked Nov 21 at 0:22


Xerakon
548
548
You are passing$doctor
in constructor ofNewDoctor
. You need to have a__construct($someVariable)
constructor to initialize that value in your notification.
– InvincibleElf
Nov 21 at 1:06
add a comment |
You are passing$doctor
in constructor ofNewDoctor
. You need to have a__construct($someVariable)
constructor to initialize that value in your notification.
– InvincibleElf
Nov 21 at 1:06
You are passing
$doctor
in constructor of NewDoctor
. You need to have a __construct($someVariable)
constructor to initialize that value in your notification.– InvincibleElf
Nov 21 at 1:06
You are passing
$doctor
in constructor of NewDoctor
. You need to have a __construct($someVariable)
constructor to initialize that value in your notification.– InvincibleElf
Nov 21 at 1:06
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
private $doctor;
public function __construct($doctor)
{
$this->doctor = $doctor;
}
Declare a constructor in your New Doctor
notification class and then only you would be able to use the $doctor
variable.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
private $doctor;
public function __construct($doctor)
{
$this->doctor = $doctor;
}
Declare a constructor in your New Doctor
notification class and then only you would be able to use the $doctor
variable.
add a comment |
up vote
1
down vote
accepted
private $doctor;
public function __construct($doctor)
{
$this->doctor = $doctor;
}
Declare a constructor in your New Doctor
notification class and then only you would be able to use the $doctor
variable.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
private $doctor;
public function __construct($doctor)
{
$this->doctor = $doctor;
}
Declare a constructor in your New Doctor
notification class and then only you would be able to use the $doctor
variable.
private $doctor;
public function __construct($doctor)
{
$this->doctor = $doctor;
}
Declare a constructor in your New Doctor
notification class and then only you would be able to use the $doctor
variable.
answered Nov 21 at 1:09


InvincibleElf
865
865
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403588%2flaravel-notification-redirect-to-newly-created-item%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DWehoo8kYy,TZTxpL5Bf pbd6eKCooa,s 8YT2 nTfYrqJqkYqJs,MajkAOEA,BciFxZ,sP Kwcn9 XV,IA
You are passing
$doctor
in constructor ofNewDoctor
. You need to have a__construct($someVariable)
constructor to initialize that value in your notification.– InvincibleElf
Nov 21 at 1:06