Clear input field after button clicked
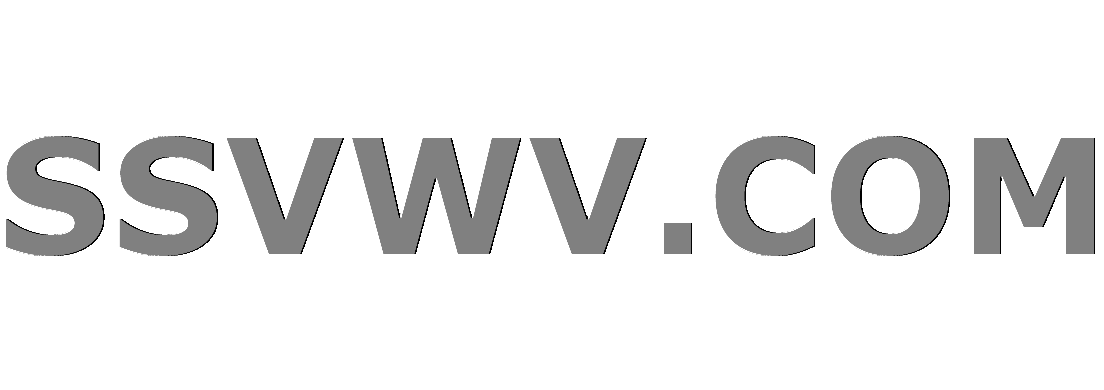
Multi tool use
up vote
0
down vote
favorite
I'm building a simple task list in react and stuck with clearing the input field after the button is pushed and help would be appreciated. I have inspected it with the react extension and it is adding the value to my desired list and resting the curVal, but the input box is staying the same
class App extends Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.curValue}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List{" "}
</button>
</div>
);
};
reactjs forms
add a comment |
up vote
0
down vote
favorite
I'm building a simple task list in react and stuck with clearing the input field after the button is pushed and help would be appreciated. I have inspected it with the react extension and it is adding the value to my desired list and resting the curVal, but the input box is staying the same
class App extends Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.curValue}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List{" "}
</button>
</div>
);
};
reactjs forms
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm building a simple task list in react and stuck with clearing the input field after the button is pushed and help would be appreciated. I have inspected it with the react extension and it is adding the value to my desired list and resting the curVal, but the input box is staying the same
class App extends Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.curValue}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List{" "}
</button>
</div>
);
};
reactjs forms
I'm building a simple task list in react and stuck with clearing the input field after the button is pushed and help would be appreciated. I have inspected it with the react extension and it is adding the value to my desired list and resting the curVal, but the input box is staying the same
class App extends Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.curValue}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List{" "}
</button>
</div>
);
};
reactjs forms
reactjs forms
edited Nov 21 at 15:11


Tholle
33.2k53558
33.2k53558
asked Nov 21 at 15:06
Kyle
11
11
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
The prop you give to your TaskInput
is named value
, not curValue
.
Change props.curValue
to props.value
and it will work as expected.
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
Yes thank you!!!
– Kyle
Nov 21 at 15:25
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The prop you give to your TaskInput
is named value
, not curValue
.
Change props.curValue
to props.value
and it will work as expected.
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
Yes thank you!!!
– Kyle
Nov 21 at 15:25
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
add a comment |
up vote
0
down vote
The prop you give to your TaskInput
is named value
, not curValue
.
Change props.curValue
to props.value
and it will work as expected.
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
Yes thank you!!!
– Kyle
Nov 21 at 15:25
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
add a comment |
up vote
0
down vote
up vote
0
down vote
The prop you give to your TaskInput
is named value
, not curValue
.
Change props.curValue
to props.value
and it will work as expected.
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
The prop you give to your TaskInput
is named value
, not curValue
.
Change props.curValue
to props.value
and it will work as expected.
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
class App extends React.Component {
state = {
taskList: ,
curValue: ""
};
changeHandler = event => {
this.setState({ curValue: event.target.value });
};
newTaskHandler = () => {
const itemValue = this.state.curValue;
const clearInput = "";
this.setState({
taskList: [...this.state.taskList, itemValue],
curValue: ""
});
};
render() {
return (
<div className="App">
<h1>Task List</h1>
<TaskInput
click={this.newTaskHandler}
value={this.state.curValue}
changed={this.changeHandler}
/>
<h1>{this.curValue}</h1>
<h1>{this.taskList}</h1>
</div>
);
}
}
const TaskInput = props => {
return (
<div>
<input
type="text"
name="task"
value={props.value}
onChange={props.changed}
/>
<button type="submit" onClick={props.click}>
Add to your Task List
</button>
</div>
);
};
ReactDOM.render(<App />, document.getElementById("root"));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
answered Nov 21 at 15:10


Tholle
33.2k53558
33.2k53558
Yes thank you!!!
– Kyle
Nov 21 at 15:25
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
add a comment |
Yes thank you!!!
– Kyle
Nov 21 at 15:25
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
Yes thank you!!!
– Kyle
Nov 21 at 15:25
Yes thank you!!!
– Kyle
Nov 21 at 15:25
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle You're welcome!
– Tholle
Nov 21 at 15:31
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
@Kyle Consider accepting the answer if you feel it answered your question.
– Tholle
Nov 21 at 15:32
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414947%2fclear-input-field-after-button-clicked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hG,W1ZMONSWA,5KRKUSNf9s5HgKtvfA Wj32,503vTr