WPF show MouseMove position
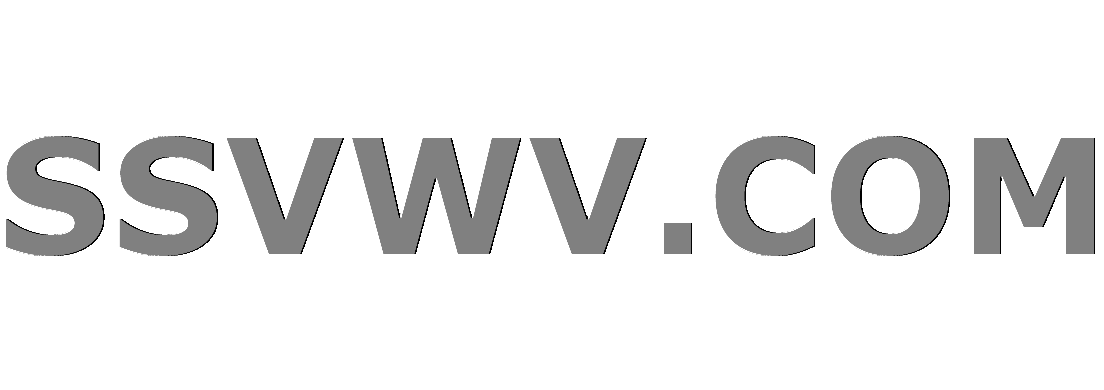
Multi tool use
up vote
0
down vote
favorite
I have XAML:
<Grid MouseMove="onMouseMove" >
<ItemsControl Name="btnTableImageList">
<ItemsControl.ItemTemplate>
<DataTemplate>
<Button Content="{Binding Content}"
Height="{Binding Height}"
Width="{Binding Width}"
Tag="{Binding Tag}"
Margin="{Binding Margin}"
Background="{Binding Background}"
HorizontalAlignment="Center"
MouseDown="tblButton_MouseDown"
MouseUp="tblButton_MouseUp"
Click="ClickHandlerTableBtn"
TextBlock.TextAlignment="Center" />
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Grid>
And code behind:
private void onMouseMove(object sender, MouseEventArgs e)
{
lblCoord.Content = Mouse.GetPosition(Application.Current.MainWindow);
}
On the form there is Label named lblCoord, and there are two buttons that are created after form is loaded.
I want to display mouse coordinate in lblCoord in relation to the Grid, but coords are displayed only when i move mouse cursor over any of the buttons that are placed inside that grid.
My guess is that I am placing MouseMove="onMouseMove" in wrong place.
Thanks for your help.
c# wpf
|
show 6 more comments
up vote
0
down vote
favorite
I have XAML:
<Grid MouseMove="onMouseMove" >
<ItemsControl Name="btnTableImageList">
<ItemsControl.ItemTemplate>
<DataTemplate>
<Button Content="{Binding Content}"
Height="{Binding Height}"
Width="{Binding Width}"
Tag="{Binding Tag}"
Margin="{Binding Margin}"
Background="{Binding Background}"
HorizontalAlignment="Center"
MouseDown="tblButton_MouseDown"
MouseUp="tblButton_MouseUp"
Click="ClickHandlerTableBtn"
TextBlock.TextAlignment="Center" />
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Grid>
And code behind:
private void onMouseMove(object sender, MouseEventArgs e)
{
lblCoord.Content = Mouse.GetPosition(Application.Current.MainWindow);
}
On the form there is Label named lblCoord, and there are two buttons that are created after form is loaded.
I want to display mouse coordinate in lblCoord in relation to the Grid, but coords are displayed only when i move mouse cursor over any of the buttons that are placed inside that grid.
My guess is that I am placing MouseMove="onMouseMove" in wrong place.
Thanks for your help.
c# wpf
2
Without testing this I know that Button in WPF captures the mouse click, it may also be capturing the MouseMove. I'd have to test and see. If so, in WPF you can use PreviewMouseMove which is captured outmost first. So try using PreviewMouseMove and see if that works. Events are tunneled down from outer most content to inner and raised usually with events prefixed with Preview. Other events are bubbled from inner most to out and do not include the Preview prefix.
– Michael Puckett II
Nov 21 at 14:57
You might need to calculate the possition relative to the screen.
– ikerbera
Nov 21 at 14:59
Same thing happens with PreviewMouseMove. position is shown only when mouse cursor goes over buttons and completly ignores grid, or whatever else is behind cursor except if it's a button.
– Bodul
Nov 21 at 15:00
1
I'll have to play with this in code. What is the content in the button? Verify that content isn't capturing the events. Just scanning it that's my assumption but I'm not sure. It may be that the Grid doesn't fire the MouseMove event if it's transparent bc I know you can click through transparency in WPF. If it's a transparency issue you can make the Background a color that's so transparent (but not completely) you can't see it but it still registers click. Possibly the same with MouseMove...
– Michael Puckett II
Nov 21 at 15:05
1
Buttons are dynamicly created during runtime, so a lot of stuff is loaded from database. But for test you can change XAML button code to <Button Content ="Test" /> it will behave exactly the same.
– Bodul
Nov 21 at 15:08
|
show 6 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have XAML:
<Grid MouseMove="onMouseMove" >
<ItemsControl Name="btnTableImageList">
<ItemsControl.ItemTemplate>
<DataTemplate>
<Button Content="{Binding Content}"
Height="{Binding Height}"
Width="{Binding Width}"
Tag="{Binding Tag}"
Margin="{Binding Margin}"
Background="{Binding Background}"
HorizontalAlignment="Center"
MouseDown="tblButton_MouseDown"
MouseUp="tblButton_MouseUp"
Click="ClickHandlerTableBtn"
TextBlock.TextAlignment="Center" />
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Grid>
And code behind:
private void onMouseMove(object sender, MouseEventArgs e)
{
lblCoord.Content = Mouse.GetPosition(Application.Current.MainWindow);
}
On the form there is Label named lblCoord, and there are two buttons that are created after form is loaded.
I want to display mouse coordinate in lblCoord in relation to the Grid, but coords are displayed only when i move mouse cursor over any of the buttons that are placed inside that grid.
My guess is that I am placing MouseMove="onMouseMove" in wrong place.
Thanks for your help.
c# wpf
I have XAML:
<Grid MouseMove="onMouseMove" >
<ItemsControl Name="btnTableImageList">
<ItemsControl.ItemTemplate>
<DataTemplate>
<Button Content="{Binding Content}"
Height="{Binding Height}"
Width="{Binding Width}"
Tag="{Binding Tag}"
Margin="{Binding Margin}"
Background="{Binding Background}"
HorizontalAlignment="Center"
MouseDown="tblButton_MouseDown"
MouseUp="tblButton_MouseUp"
Click="ClickHandlerTableBtn"
TextBlock.TextAlignment="Center" />
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
</Grid>
And code behind:
private void onMouseMove(object sender, MouseEventArgs e)
{
lblCoord.Content = Mouse.GetPosition(Application.Current.MainWindow);
}
On the form there is Label named lblCoord, and there are two buttons that are created after form is loaded.
I want to display mouse coordinate in lblCoord in relation to the Grid, but coords are displayed only when i move mouse cursor over any of the buttons that are placed inside that grid.
My guess is that I am placing MouseMove="onMouseMove" in wrong place.
Thanks for your help.
c# wpf
c# wpf
edited Nov 21 at 15:00
Lews Therin
2,27811436
2,27811436
asked Nov 21 at 14:53
Bodul
898
898
2
Without testing this I know that Button in WPF captures the mouse click, it may also be capturing the MouseMove. I'd have to test and see. If so, in WPF you can use PreviewMouseMove which is captured outmost first. So try using PreviewMouseMove and see if that works. Events are tunneled down from outer most content to inner and raised usually with events prefixed with Preview. Other events are bubbled from inner most to out and do not include the Preview prefix.
– Michael Puckett II
Nov 21 at 14:57
You might need to calculate the possition relative to the screen.
– ikerbera
Nov 21 at 14:59
Same thing happens with PreviewMouseMove. position is shown only when mouse cursor goes over buttons and completly ignores grid, or whatever else is behind cursor except if it's a button.
– Bodul
Nov 21 at 15:00
1
I'll have to play with this in code. What is the content in the button? Verify that content isn't capturing the events. Just scanning it that's my assumption but I'm not sure. It may be that the Grid doesn't fire the MouseMove event if it's transparent bc I know you can click through transparency in WPF. If it's a transparency issue you can make the Background a color that's so transparent (but not completely) you can't see it but it still registers click. Possibly the same with MouseMove...
– Michael Puckett II
Nov 21 at 15:05
1
Buttons are dynamicly created during runtime, so a lot of stuff is loaded from database. But for test you can change XAML button code to <Button Content ="Test" /> it will behave exactly the same.
– Bodul
Nov 21 at 15:08
|
show 6 more comments
2
Without testing this I know that Button in WPF captures the mouse click, it may also be capturing the MouseMove. I'd have to test and see. If so, in WPF you can use PreviewMouseMove which is captured outmost first. So try using PreviewMouseMove and see if that works. Events are tunneled down from outer most content to inner and raised usually with events prefixed with Preview. Other events are bubbled from inner most to out and do not include the Preview prefix.
– Michael Puckett II
Nov 21 at 14:57
You might need to calculate the possition relative to the screen.
– ikerbera
Nov 21 at 14:59
Same thing happens with PreviewMouseMove. position is shown only when mouse cursor goes over buttons and completly ignores grid, or whatever else is behind cursor except if it's a button.
– Bodul
Nov 21 at 15:00
1
I'll have to play with this in code. What is the content in the button? Verify that content isn't capturing the events. Just scanning it that's my assumption but I'm not sure. It may be that the Grid doesn't fire the MouseMove event if it's transparent bc I know you can click through transparency in WPF. If it's a transparency issue you can make the Background a color that's so transparent (but not completely) you can't see it but it still registers click. Possibly the same with MouseMove...
– Michael Puckett II
Nov 21 at 15:05
1
Buttons are dynamicly created during runtime, so a lot of stuff is loaded from database. But for test you can change XAML button code to <Button Content ="Test" /> it will behave exactly the same.
– Bodul
Nov 21 at 15:08
2
2
Without testing this I know that Button in WPF captures the mouse click, it may also be capturing the MouseMove. I'd have to test and see. If so, in WPF you can use PreviewMouseMove which is captured outmost first. So try using PreviewMouseMove and see if that works. Events are tunneled down from outer most content to inner and raised usually with events prefixed with Preview. Other events are bubbled from inner most to out and do not include the Preview prefix.
– Michael Puckett II
Nov 21 at 14:57
Without testing this I know that Button in WPF captures the mouse click, it may also be capturing the MouseMove. I'd have to test and see. If so, in WPF you can use PreviewMouseMove which is captured outmost first. So try using PreviewMouseMove and see if that works. Events are tunneled down from outer most content to inner and raised usually with events prefixed with Preview. Other events are bubbled from inner most to out and do not include the Preview prefix.
– Michael Puckett II
Nov 21 at 14:57
You might need to calculate the possition relative to the screen.
– ikerbera
Nov 21 at 14:59
You might need to calculate the possition relative to the screen.
– ikerbera
Nov 21 at 14:59
Same thing happens with PreviewMouseMove. position is shown only when mouse cursor goes over buttons and completly ignores grid, or whatever else is behind cursor except if it's a button.
– Bodul
Nov 21 at 15:00
Same thing happens with PreviewMouseMove. position is shown only when mouse cursor goes over buttons and completly ignores grid, or whatever else is behind cursor except if it's a button.
– Bodul
Nov 21 at 15:00
1
1
I'll have to play with this in code. What is the content in the button? Verify that content isn't capturing the events. Just scanning it that's my assumption but I'm not sure. It may be that the Grid doesn't fire the MouseMove event if it's transparent bc I know you can click through transparency in WPF. If it's a transparency issue you can make the Background a color that's so transparent (but not completely) you can't see it but it still registers click. Possibly the same with MouseMove...
– Michael Puckett II
Nov 21 at 15:05
I'll have to play with this in code. What is the content in the button? Verify that content isn't capturing the events. Just scanning it that's my assumption but I'm not sure. It may be that the Grid doesn't fire the MouseMove event if it's transparent bc I know you can click through transparency in WPF. If it's a transparency issue you can make the Background a color that's so transparent (but not completely) you can't see it but it still registers click. Possibly the same with MouseMove...
– Michael Puckett II
Nov 21 at 15:05
1
1
Buttons are dynamicly created during runtime, so a lot of stuff is loaded from database. But for test you can change XAML button code to <Button Content ="Test" /> it will behave exactly the same.
– Bodul
Nov 21 at 15:08
Buttons are dynamicly created during runtime, so a lot of stuff is loaded from database. But for test you can change XAML button code to <Button Content ="Test" /> it will behave exactly the same.
– Bodul
Nov 21 at 15:08
|
show 6 more comments
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
It will work when you set Background
of Grid to anything but Transparent
As default, Grid's background is transparent. When it is transparent, mouse events work when you set Background="Transparent"
too.
Mouse events handled nearest parent element with background IMHO
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
It will work when you set Background
of Grid to anything but Transparent
As default, Grid's background is transparent. When it is transparent, mouse events work when you set Background="Transparent"
too.
Mouse events handled nearest parent element with background IMHO
add a comment |
up vote
2
down vote
accepted
It will work when you set Background
of Grid to anything but Transparent
As default, Grid's background is transparent. When it is transparent, mouse events work when you set Background="Transparent"
too.
Mouse events handled nearest parent element with background IMHO
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
It will work when you set Background
of Grid to anything but Transparent
As default, Grid's background is transparent. When it is transparent, mouse events work when you set Background="Transparent"
too.
Mouse events handled nearest parent element with background IMHO
It will work when you set Background
of Grid to anything but Transparent
As default, Grid's background is transparent. When it is transparent, mouse events work when you set Background="Transparent"
too.
Mouse events handled nearest parent element with background IMHO
edited Nov 21 at 15:22
answered Nov 21 at 15:11


tetralobita
384513
384513
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414719%2fwpf-show-mousemove-position%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
T,iPI9yH5rdSIdbjleX gKOhGCDXfsywvR1AaA0
2
Without testing this I know that Button in WPF captures the mouse click, it may also be capturing the MouseMove. I'd have to test and see. If so, in WPF you can use PreviewMouseMove which is captured outmost first. So try using PreviewMouseMove and see if that works. Events are tunneled down from outer most content to inner and raised usually with events prefixed with Preview. Other events are bubbled from inner most to out and do not include the Preview prefix.
– Michael Puckett II
Nov 21 at 14:57
You might need to calculate the possition relative to the screen.
– ikerbera
Nov 21 at 14:59
Same thing happens with PreviewMouseMove. position is shown only when mouse cursor goes over buttons and completly ignores grid, or whatever else is behind cursor except if it's a button.
– Bodul
Nov 21 at 15:00
1
I'll have to play with this in code. What is the content in the button? Verify that content isn't capturing the events. Just scanning it that's my assumption but I'm not sure. It may be that the Grid doesn't fire the MouseMove event if it's transparent bc I know you can click through transparency in WPF. If it's a transparency issue you can make the Background a color that's so transparent (but not completely) you can't see it but it still registers click. Possibly the same with MouseMove...
– Michael Puckett II
Nov 21 at 15:05
1
Buttons are dynamicly created during runtime, so a lot of stuff is loaded from database. But for test you can change XAML button code to <Button Content ="Test" /> it will behave exactly the same.
– Bodul
Nov 21 at 15:08