unable to test a method which uses Observable in Jasmine
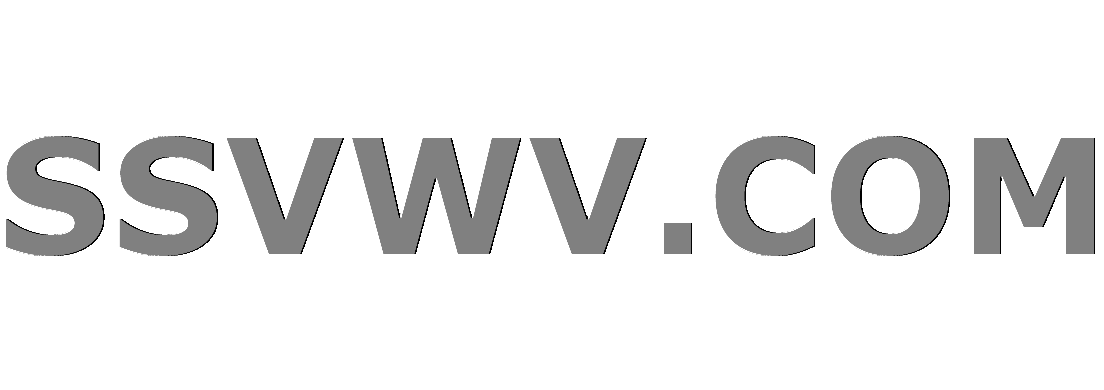
Multi tool use
up vote
0
down vote
favorite
My Angular
application has a service QuestionManagementService
which response on a BackendService
to send REST
messages. The BackendService
in turn uses HttpClient
. I am trying to test the QuestionManagementService
in isolation.
The method I am testing is addQuestion
addQuestion(question:PracticeQuestion):any{
console.log("In QuestionManagementService: addQuestion: ",question);
this.bs.createQuestion(question).subscribe((res)=>{
console.log("add practice question - response is ",res);//I EXPECT THESE PRINTS TO SHOW BUT THEY DON'T
let ev = <HttpEvent<any>>(res);
if(ev.type === HttpEventType.Response) {
console.log('response from server: returning body '+ev.body);
let isResponseStructureOK: boolean = this.helper.validateServerResponseStructure(ev.body);
if (isResponseStructureOK) {
let response:ServerResponseAPI = ev.body;
console.log("received response from server: " + response.result);
this.addQuestionSubject.next(new Result(response.result,response['additional-info']));
} else {
console.log("received incorrect response structure from server: ", ev.body);
this.addQuestionSubject.next(new Result('error','Invalid response structure from server'));
}
}
else {
console.log("not response. ignoring");
}
},
(error:ServerResponseAPI)=>{
console.log("got error from the Observable: ",error);
let errorMessage:string = this.helper.userFriendlyErrorMessage(error);
this.addQuestionSubject.next(new Result('error',errorMessage));//TODOM - need to standardise errors
},
()=>{ //observable complete
console.log("observable completed")
});
}
As I am doing unit testing of addQuestion
, I thought that I can mock the createQuestion
method of the BackendService
. The spec
I have written so far is the following but I don't think it is correct as I don't see any prints on the console when the mocked response of createQuestion
is received.
fit('should add a question',()=>{
let backendService = TestBed.get(WebToBackendInterfaceService);
let questionService = TestBed.get(QuestionManagementService);
let question = new PracticeQuestion(...);
const responseData = { result: 'success', ['additional-info']: 'question added successfully' };
let httpResponseEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
//mock response of WebToBackendInterfaceService
spyOn(backendService,'createQuestion').and.returnValue(new Observable(()=>{
httpResponseEvent;
}));
questionService.addQuestion$.subscribe((res:Result)=>{
console.log('received response from Question Services',res);
expect(res).toBeTruthy();
let validResponse:boolean = ((res.result === 'success') || (res.result === 'initial')) ;
expect(validResponse).toEqual(true);
});
questionService.addQuestion(question);
expect(backendService.createQuestion).toHaveBeenCalled();
});
angular6 angular-testing
add a comment |
up vote
0
down vote
favorite
My Angular
application has a service QuestionManagementService
which response on a BackendService
to send REST
messages. The BackendService
in turn uses HttpClient
. I am trying to test the QuestionManagementService
in isolation.
The method I am testing is addQuestion
addQuestion(question:PracticeQuestion):any{
console.log("In QuestionManagementService: addQuestion: ",question);
this.bs.createQuestion(question).subscribe((res)=>{
console.log("add practice question - response is ",res);//I EXPECT THESE PRINTS TO SHOW BUT THEY DON'T
let ev = <HttpEvent<any>>(res);
if(ev.type === HttpEventType.Response) {
console.log('response from server: returning body '+ev.body);
let isResponseStructureOK: boolean = this.helper.validateServerResponseStructure(ev.body);
if (isResponseStructureOK) {
let response:ServerResponseAPI = ev.body;
console.log("received response from server: " + response.result);
this.addQuestionSubject.next(new Result(response.result,response['additional-info']));
} else {
console.log("received incorrect response structure from server: ", ev.body);
this.addQuestionSubject.next(new Result('error','Invalid response structure from server'));
}
}
else {
console.log("not response. ignoring");
}
},
(error:ServerResponseAPI)=>{
console.log("got error from the Observable: ",error);
let errorMessage:string = this.helper.userFriendlyErrorMessage(error);
this.addQuestionSubject.next(new Result('error',errorMessage));//TODOM - need to standardise errors
},
()=>{ //observable complete
console.log("observable completed")
});
}
As I am doing unit testing of addQuestion
, I thought that I can mock the createQuestion
method of the BackendService
. The spec
I have written so far is the following but I don't think it is correct as I don't see any prints on the console when the mocked response of createQuestion
is received.
fit('should add a question',()=>{
let backendService = TestBed.get(WebToBackendInterfaceService);
let questionService = TestBed.get(QuestionManagementService);
let question = new PracticeQuestion(...);
const responseData = { result: 'success', ['additional-info']: 'question added successfully' };
let httpResponseEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
//mock response of WebToBackendInterfaceService
spyOn(backendService,'createQuestion').and.returnValue(new Observable(()=>{
httpResponseEvent;
}));
questionService.addQuestion$.subscribe((res:Result)=>{
console.log('received response from Question Services',res);
expect(res).toBeTruthy();
let validResponse:boolean = ((res.result === 'success') || (res.result === 'initial')) ;
expect(validResponse).toEqual(true);
});
questionService.addQuestion(question);
expect(backendService.createQuestion).toHaveBeenCalled();
});
angular6 angular-testing
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
My Angular
application has a service QuestionManagementService
which response on a BackendService
to send REST
messages. The BackendService
in turn uses HttpClient
. I am trying to test the QuestionManagementService
in isolation.
The method I am testing is addQuestion
addQuestion(question:PracticeQuestion):any{
console.log("In QuestionManagementService: addQuestion: ",question);
this.bs.createQuestion(question).subscribe((res)=>{
console.log("add practice question - response is ",res);//I EXPECT THESE PRINTS TO SHOW BUT THEY DON'T
let ev = <HttpEvent<any>>(res);
if(ev.type === HttpEventType.Response) {
console.log('response from server: returning body '+ev.body);
let isResponseStructureOK: boolean = this.helper.validateServerResponseStructure(ev.body);
if (isResponseStructureOK) {
let response:ServerResponseAPI = ev.body;
console.log("received response from server: " + response.result);
this.addQuestionSubject.next(new Result(response.result,response['additional-info']));
} else {
console.log("received incorrect response structure from server: ", ev.body);
this.addQuestionSubject.next(new Result('error','Invalid response structure from server'));
}
}
else {
console.log("not response. ignoring");
}
},
(error:ServerResponseAPI)=>{
console.log("got error from the Observable: ",error);
let errorMessage:string = this.helper.userFriendlyErrorMessage(error);
this.addQuestionSubject.next(new Result('error',errorMessage));//TODOM - need to standardise errors
},
()=>{ //observable complete
console.log("observable completed")
});
}
As I am doing unit testing of addQuestion
, I thought that I can mock the createQuestion
method of the BackendService
. The spec
I have written so far is the following but I don't think it is correct as I don't see any prints on the console when the mocked response of createQuestion
is received.
fit('should add a question',()=>{
let backendService = TestBed.get(WebToBackendInterfaceService);
let questionService = TestBed.get(QuestionManagementService);
let question = new PracticeQuestion(...);
const responseData = { result: 'success', ['additional-info']: 'question added successfully' };
let httpResponseEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
//mock response of WebToBackendInterfaceService
spyOn(backendService,'createQuestion').and.returnValue(new Observable(()=>{
httpResponseEvent;
}));
questionService.addQuestion$.subscribe((res:Result)=>{
console.log('received response from Question Services',res);
expect(res).toBeTruthy();
let validResponse:boolean = ((res.result === 'success') || (res.result === 'initial')) ;
expect(validResponse).toEqual(true);
});
questionService.addQuestion(question);
expect(backendService.createQuestion).toHaveBeenCalled();
});
angular6 angular-testing
My Angular
application has a service QuestionManagementService
which response on a BackendService
to send REST
messages. The BackendService
in turn uses HttpClient
. I am trying to test the QuestionManagementService
in isolation.
The method I am testing is addQuestion
addQuestion(question:PracticeQuestion):any{
console.log("In QuestionManagementService: addQuestion: ",question);
this.bs.createQuestion(question).subscribe((res)=>{
console.log("add practice question - response is ",res);//I EXPECT THESE PRINTS TO SHOW BUT THEY DON'T
let ev = <HttpEvent<any>>(res);
if(ev.type === HttpEventType.Response) {
console.log('response from server: returning body '+ev.body);
let isResponseStructureOK: boolean = this.helper.validateServerResponseStructure(ev.body);
if (isResponseStructureOK) {
let response:ServerResponseAPI = ev.body;
console.log("received response from server: " + response.result);
this.addQuestionSubject.next(new Result(response.result,response['additional-info']));
} else {
console.log("received incorrect response structure from server: ", ev.body);
this.addQuestionSubject.next(new Result('error','Invalid response structure from server'));
}
}
else {
console.log("not response. ignoring");
}
},
(error:ServerResponseAPI)=>{
console.log("got error from the Observable: ",error);
let errorMessage:string = this.helper.userFriendlyErrorMessage(error);
this.addQuestionSubject.next(new Result('error',errorMessage));//TODOM - need to standardise errors
},
()=>{ //observable complete
console.log("observable completed")
});
}
As I am doing unit testing of addQuestion
, I thought that I can mock the createQuestion
method of the BackendService
. The spec
I have written so far is the following but I don't think it is correct as I don't see any prints on the console when the mocked response of createQuestion
is received.
fit('should add a question',()=>{
let backendService = TestBed.get(WebToBackendInterfaceService);
let questionService = TestBed.get(QuestionManagementService);
let question = new PracticeQuestion(...);
const responseData = { result: 'success', ['additional-info']: 'question added successfully' };
let httpResponseEvent:HttpResponse<any> = new HttpResponse<any>({body:responseData});
//mock response of WebToBackendInterfaceService
spyOn(backendService,'createQuestion').and.returnValue(new Observable(()=>{
httpResponseEvent;
}));
questionService.addQuestion$.subscribe((res:Result)=>{
console.log('received response from Question Services',res);
expect(res).toBeTruthy();
let validResponse:boolean = ((res.result === 'success') || (res.result === 'initial')) ;
expect(validResponse).toEqual(true);
});
questionService.addQuestion(question);
expect(backendService.createQuestion).toHaveBeenCalled();
});
angular6 angular-testing
angular6 angular-testing
asked Nov 21 at 22:26
Manu Chadha
2,70121336
2,70121336
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
The issue was that I was creating an Observable
but I didn't push the value to an Observer
using next
. The correct implementation is (code snippet)
spyOn(backendService,'createQuestion').and.returnValue(new Observable((subscriber)=>{ //subscriber or observer
subscriber.next(httpResponseEvent)}
));
When creating an Observable
, the Observable's constructor in Rxjs takes a subscribe
function as argument. The definition of subscribe
function is
(observer)=>{
/*logic of calculating values which Observer should produce and emit then using observer.next(value)*/
}
reference - http://reactivex.io/rxjs/class/es6/Observable.js~Observable.html
With the above code, the this.bs.createQuestion(question)
returns a mock Observable
whose subscribe
function is
(subscriber)=>{ //subscribe function
subscriber.next(httpResponseEvent)
}
The above subscribe
function will be called whenever any Observer
subscribes to the Observable
. So in code .subscribe((res)=>{...}
, I subscribe to the mock Observable
and now the subscribe
function will emit the dummy value, httpResponseEvent
to my code. For those new to Observables
, the subscribe
function takes an Observer
as argument. An Observer
is an object which has 3 methods next
, error
and complete
. Note that the subscribe
function takes such an object
(res)=>{... },
(error:ServerResponseAPI)=>{
...
},
()=>{ //observable complete
...
})
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421302%2funable-to-test-a-method-which-uses-observable-in-jasmine%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The issue was that I was creating an Observable
but I didn't push the value to an Observer
using next
. The correct implementation is (code snippet)
spyOn(backendService,'createQuestion').and.returnValue(new Observable((subscriber)=>{ //subscriber or observer
subscriber.next(httpResponseEvent)}
));
When creating an Observable
, the Observable's constructor in Rxjs takes a subscribe
function as argument. The definition of subscribe
function is
(observer)=>{
/*logic of calculating values which Observer should produce and emit then using observer.next(value)*/
}
reference - http://reactivex.io/rxjs/class/es6/Observable.js~Observable.html
With the above code, the this.bs.createQuestion(question)
returns a mock Observable
whose subscribe
function is
(subscriber)=>{ //subscribe function
subscriber.next(httpResponseEvent)
}
The above subscribe
function will be called whenever any Observer
subscribes to the Observable
. So in code .subscribe((res)=>{...}
, I subscribe to the mock Observable
and now the subscribe
function will emit the dummy value, httpResponseEvent
to my code. For those new to Observables
, the subscribe
function takes an Observer
as argument. An Observer
is an object which has 3 methods next
, error
and complete
. Note that the subscribe
function takes such an object
(res)=>{... },
(error:ServerResponseAPI)=>{
...
},
()=>{ //observable complete
...
})
add a comment |
up vote
0
down vote
The issue was that I was creating an Observable
but I didn't push the value to an Observer
using next
. The correct implementation is (code snippet)
spyOn(backendService,'createQuestion').and.returnValue(new Observable((subscriber)=>{ //subscriber or observer
subscriber.next(httpResponseEvent)}
));
When creating an Observable
, the Observable's constructor in Rxjs takes a subscribe
function as argument. The definition of subscribe
function is
(observer)=>{
/*logic of calculating values which Observer should produce and emit then using observer.next(value)*/
}
reference - http://reactivex.io/rxjs/class/es6/Observable.js~Observable.html
With the above code, the this.bs.createQuestion(question)
returns a mock Observable
whose subscribe
function is
(subscriber)=>{ //subscribe function
subscriber.next(httpResponseEvent)
}
The above subscribe
function will be called whenever any Observer
subscribes to the Observable
. So in code .subscribe((res)=>{...}
, I subscribe to the mock Observable
and now the subscribe
function will emit the dummy value, httpResponseEvent
to my code. For those new to Observables
, the subscribe
function takes an Observer
as argument. An Observer
is an object which has 3 methods next
, error
and complete
. Note that the subscribe
function takes such an object
(res)=>{... },
(error:ServerResponseAPI)=>{
...
},
()=>{ //observable complete
...
})
add a comment |
up vote
0
down vote
up vote
0
down vote
The issue was that I was creating an Observable
but I didn't push the value to an Observer
using next
. The correct implementation is (code snippet)
spyOn(backendService,'createQuestion').and.returnValue(new Observable((subscriber)=>{ //subscriber or observer
subscriber.next(httpResponseEvent)}
));
When creating an Observable
, the Observable's constructor in Rxjs takes a subscribe
function as argument. The definition of subscribe
function is
(observer)=>{
/*logic of calculating values which Observer should produce and emit then using observer.next(value)*/
}
reference - http://reactivex.io/rxjs/class/es6/Observable.js~Observable.html
With the above code, the this.bs.createQuestion(question)
returns a mock Observable
whose subscribe
function is
(subscriber)=>{ //subscribe function
subscriber.next(httpResponseEvent)
}
The above subscribe
function will be called whenever any Observer
subscribes to the Observable
. So in code .subscribe((res)=>{...}
, I subscribe to the mock Observable
and now the subscribe
function will emit the dummy value, httpResponseEvent
to my code. For those new to Observables
, the subscribe
function takes an Observer
as argument. An Observer
is an object which has 3 methods next
, error
and complete
. Note that the subscribe
function takes such an object
(res)=>{... },
(error:ServerResponseAPI)=>{
...
},
()=>{ //observable complete
...
})
The issue was that I was creating an Observable
but I didn't push the value to an Observer
using next
. The correct implementation is (code snippet)
spyOn(backendService,'createQuestion').and.returnValue(new Observable((subscriber)=>{ //subscriber or observer
subscriber.next(httpResponseEvent)}
));
When creating an Observable
, the Observable's constructor in Rxjs takes a subscribe
function as argument. The definition of subscribe
function is
(observer)=>{
/*logic of calculating values which Observer should produce and emit then using observer.next(value)*/
}
reference - http://reactivex.io/rxjs/class/es6/Observable.js~Observable.html
With the above code, the this.bs.createQuestion(question)
returns a mock Observable
whose subscribe
function is
(subscriber)=>{ //subscribe function
subscriber.next(httpResponseEvent)
}
The above subscribe
function will be called whenever any Observer
subscribes to the Observable
. So in code .subscribe((res)=>{...}
, I subscribe to the mock Observable
and now the subscribe
function will emit the dummy value, httpResponseEvent
to my code. For those new to Observables
, the subscribe
function takes an Observer
as argument. An Observer
is an object which has 3 methods next
, error
and complete
. Note that the subscribe
function takes such an object
(res)=>{... },
(error:ServerResponseAPI)=>{
...
},
()=>{ //observable complete
...
})
answered Nov 22 at 17:18
Manu Chadha
2,70121336
2,70121336
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421302%2funable-to-test-a-method-which-uses-observable-in-jasmine%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ut916QS Xi,oS,YZw,SBkOIB vjXDFWLalix xfLzjywjVYO5,deJC4d