Modify field names in serializer in Django Rest Framework
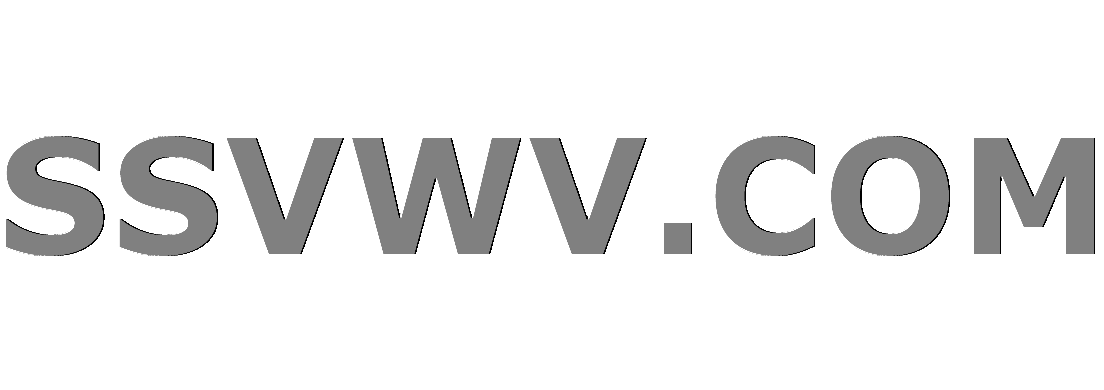
Multi tool use
I have an object I'd like to serialize using DRF's serializers, but I'd like to normalize some field names. I thought I might be able to use the source
attribute to achieve this:
user = { 'FirstName': 'John', 'LastName': 'Doe' }
serialized = UserSerializer(data=user)
class UserSerializer(serializers.Serializer):
first_name = serializers.CharField(source="FirstName")
last_name = serializers.CharField(source="LastName")
However, I'm not sure how to access the data object passed in - is there a way without creating a new method or some kind of complex super()
call?
edit:
Use case: I'm hitting an API which returns values in CamelCase ('FirstName', 'LastName' etc) which I need to validate and modify key names to snake case. I was hoping I could use a standalone serializer, and transform the names in the serializer. I don't have a model for the data that must be transformed.
python django-rest-framework serializer
add a comment |
I have an object I'd like to serialize using DRF's serializers, but I'd like to normalize some field names. I thought I might be able to use the source
attribute to achieve this:
user = { 'FirstName': 'John', 'LastName': 'Doe' }
serialized = UserSerializer(data=user)
class UserSerializer(serializers.Serializer):
first_name = serializers.CharField(source="FirstName")
last_name = serializers.CharField(source="LastName")
However, I'm not sure how to access the data object passed in - is there a way without creating a new method or some kind of complex super()
call?
edit:
Use case: I'm hitting an API which returns values in CamelCase ('FirstName', 'LastName' etc) which I need to validate and modify key names to snake case. I was hoping I could use a standalone serializer, and transform the names in the serializer. I don't have a model for the data that must be transformed.
python django-rest-framework serializer
add a comment |
I have an object I'd like to serialize using DRF's serializers, but I'd like to normalize some field names. I thought I might be able to use the source
attribute to achieve this:
user = { 'FirstName': 'John', 'LastName': 'Doe' }
serialized = UserSerializer(data=user)
class UserSerializer(serializers.Serializer):
first_name = serializers.CharField(source="FirstName")
last_name = serializers.CharField(source="LastName")
However, I'm not sure how to access the data object passed in - is there a way without creating a new method or some kind of complex super()
call?
edit:
Use case: I'm hitting an API which returns values in CamelCase ('FirstName', 'LastName' etc) which I need to validate and modify key names to snake case. I was hoping I could use a standalone serializer, and transform the names in the serializer. I don't have a model for the data that must be transformed.
python django-rest-framework serializer
I have an object I'd like to serialize using DRF's serializers, but I'd like to normalize some field names. I thought I might be able to use the source
attribute to achieve this:
user = { 'FirstName': 'John', 'LastName': 'Doe' }
serialized = UserSerializer(data=user)
class UserSerializer(serializers.Serializer):
first_name = serializers.CharField(source="FirstName")
last_name = serializers.CharField(source="LastName")
However, I'm not sure how to access the data object passed in - is there a way without creating a new method or some kind of complex super()
call?
edit:
Use case: I'm hitting an API which returns values in CamelCase ('FirstName', 'LastName' etc) which I need to validate and modify key names to snake case. I was hoping I could use a standalone serializer, and transform the names in the serializer. I don't have a model for the data that must be transformed.
python django-rest-framework serializer
python django-rest-framework serializer
edited Nov 23 '18 at 21:14
Toby
asked Nov 23 '18 at 19:36
TobyToby
5,23442248
5,23442248
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can achieve this with properties on your Django model:
class Foo(models.model):
bar = models.CharField(max_length=40)
@property
def sanitized_bar(self):
print("Getting value")
return self.bar.lower()
@sanitized_bar.setter
def sanitized_bar(self, value):
self.bar = value.lower()
class FooSerializer(serializers.Serializer):
bar = CharField(source='sanitized_bar')
Doing it this way gives you sanitized control on your DB if you need to use those models in management commands or elsewhere, in addition to your DRF interface.
You can also do a pre-save hook:
class Foo(models.model):
def save(self, *args, **kwargs):
if self.bar:
self.bar = self.bar.lower()
super().save(*args, **kwargs)
That makes sense - but what is this is not a model serializer? I assume thesource
attribute is not the way to achieve this..
– Toby
Nov 23 '18 at 20:16
Yeah, you'd have to do:f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also makebar
be the property and shadow it withbar_private
so the names are less confusing.
– Ross Rogers
Nov 23 '18 at 20:23
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
add a comment |
Try this:
class UserSerializer(serializers.Serializer):
FirstName = serializers.CharField(source="first_name")
LastName = serializers.CharField(source="last_name")
usage(serializing):
class Person:
first_name = "first name"
last_name = "last name"
person1 = Person()
serialized_data = UserSerializer(person1).data # = {"FirstName": "first name", "LastName": "last name"}
usage(deserializing):
data = { 'FirstName': 'John', 'LastName': 'Doe' }
serializer = UserSerializer(data=data)
serializer.is_valid()
valid_deserialized_data = serializer.validated_data # = { 'first_name': 'John', 'last_name': 'Doe' }
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452150%2fmodify-field-names-in-serializer-in-django-rest-framework%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can achieve this with properties on your Django model:
class Foo(models.model):
bar = models.CharField(max_length=40)
@property
def sanitized_bar(self):
print("Getting value")
return self.bar.lower()
@sanitized_bar.setter
def sanitized_bar(self, value):
self.bar = value.lower()
class FooSerializer(serializers.Serializer):
bar = CharField(source='sanitized_bar')
Doing it this way gives you sanitized control on your DB if you need to use those models in management commands or elsewhere, in addition to your DRF interface.
You can also do a pre-save hook:
class Foo(models.model):
def save(self, *args, **kwargs):
if self.bar:
self.bar = self.bar.lower()
super().save(*args, **kwargs)
That makes sense - but what is this is not a model serializer? I assume thesource
attribute is not the way to achieve this..
– Toby
Nov 23 '18 at 20:16
Yeah, you'd have to do:f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also makebar
be the property and shadow it withbar_private
so the names are less confusing.
– Ross Rogers
Nov 23 '18 at 20:23
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
add a comment |
You can achieve this with properties on your Django model:
class Foo(models.model):
bar = models.CharField(max_length=40)
@property
def sanitized_bar(self):
print("Getting value")
return self.bar.lower()
@sanitized_bar.setter
def sanitized_bar(self, value):
self.bar = value.lower()
class FooSerializer(serializers.Serializer):
bar = CharField(source='sanitized_bar')
Doing it this way gives you sanitized control on your DB if you need to use those models in management commands or elsewhere, in addition to your DRF interface.
You can also do a pre-save hook:
class Foo(models.model):
def save(self, *args, **kwargs):
if self.bar:
self.bar = self.bar.lower()
super().save(*args, **kwargs)
That makes sense - but what is this is not a model serializer? I assume thesource
attribute is not the way to achieve this..
– Toby
Nov 23 '18 at 20:16
Yeah, you'd have to do:f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also makebar
be the property and shadow it withbar_private
so the names are less confusing.
– Ross Rogers
Nov 23 '18 at 20:23
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
add a comment |
You can achieve this with properties on your Django model:
class Foo(models.model):
bar = models.CharField(max_length=40)
@property
def sanitized_bar(self):
print("Getting value")
return self.bar.lower()
@sanitized_bar.setter
def sanitized_bar(self, value):
self.bar = value.lower()
class FooSerializer(serializers.Serializer):
bar = CharField(source='sanitized_bar')
Doing it this way gives you sanitized control on your DB if you need to use those models in management commands or elsewhere, in addition to your DRF interface.
You can also do a pre-save hook:
class Foo(models.model):
def save(self, *args, **kwargs):
if self.bar:
self.bar = self.bar.lower()
super().save(*args, **kwargs)
You can achieve this with properties on your Django model:
class Foo(models.model):
bar = models.CharField(max_length=40)
@property
def sanitized_bar(self):
print("Getting value")
return self.bar.lower()
@sanitized_bar.setter
def sanitized_bar(self, value):
self.bar = value.lower()
class FooSerializer(serializers.Serializer):
bar = CharField(source='sanitized_bar')
Doing it this way gives you sanitized control on your DB if you need to use those models in management commands or elsewhere, in addition to your DRF interface.
You can also do a pre-save hook:
class Foo(models.model):
def save(self, *args, **kwargs):
if self.bar:
self.bar = self.bar.lower()
super().save(*args, **kwargs)
edited Nov 23 '18 at 20:24
answered Nov 23 '18 at 20:05


Ross RogersRoss Rogers
11.7k1682138
11.7k1682138
That makes sense - but what is this is not a model serializer? I assume thesource
attribute is not the way to achieve this..
– Toby
Nov 23 '18 at 20:16
Yeah, you'd have to do:f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also makebar
be the property and shadow it withbar_private
so the names are less confusing.
– Ross Rogers
Nov 23 '18 at 20:23
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
add a comment |
That makes sense - but what is this is not a model serializer? I assume thesource
attribute is not the way to achieve this..
– Toby
Nov 23 '18 at 20:16
Yeah, you'd have to do:f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also makebar
be the property and shadow it withbar_private
so the names are less confusing.
– Ross Rogers
Nov 23 '18 at 20:23
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
That makes sense - but what is this is not a model serializer? I assume the
source
attribute is not the way to achieve this..– Toby
Nov 23 '18 at 20:16
That makes sense - but what is this is not a model serializer? I assume the
source
attribute is not the way to achieve this..– Toby
Nov 23 '18 at 20:16
Yeah, you'd have to do:
f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also make bar
be the property and shadow it with bar_private
so the names are less confusing.– Ross Rogers
Nov 23 '18 at 20:23
Yeah, you'd have to do:
f = Foo(); f.sanitized_bar = 'baz'
. The alternative is to implement a pre-save hook, but you would then have to save the model instance before it could be used. You could also make bar
be the property and shadow it with bar_private
so the names are less confusing.– Ross Rogers
Nov 23 '18 at 20:23
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
Okay.. I was hoping there was a simple solution I was missing - I'll look into switching things around, thanks.
– Toby
Nov 23 '18 at 20:30
add a comment |
Try this:
class UserSerializer(serializers.Serializer):
FirstName = serializers.CharField(source="first_name")
LastName = serializers.CharField(source="last_name")
usage(serializing):
class Person:
first_name = "first name"
last_name = "last name"
person1 = Person()
serialized_data = UserSerializer(person1).data # = {"FirstName": "first name", "LastName": "last name"}
usage(deserializing):
data = { 'FirstName': 'John', 'LastName': 'Doe' }
serializer = UserSerializer(data=data)
serializer.is_valid()
valid_deserialized_data = serializer.validated_data # = { 'first_name': 'John', 'last_name': 'Doe' }
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
add a comment |
Try this:
class UserSerializer(serializers.Serializer):
FirstName = serializers.CharField(source="first_name")
LastName = serializers.CharField(source="last_name")
usage(serializing):
class Person:
first_name = "first name"
last_name = "last name"
person1 = Person()
serialized_data = UserSerializer(person1).data # = {"FirstName": "first name", "LastName": "last name"}
usage(deserializing):
data = { 'FirstName': 'John', 'LastName': 'Doe' }
serializer = UserSerializer(data=data)
serializer.is_valid()
valid_deserialized_data = serializer.validated_data # = { 'first_name': 'John', 'last_name': 'Doe' }
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
add a comment |
Try this:
class UserSerializer(serializers.Serializer):
FirstName = serializers.CharField(source="first_name")
LastName = serializers.CharField(source="last_name")
usage(serializing):
class Person:
first_name = "first name"
last_name = "last name"
person1 = Person()
serialized_data = UserSerializer(person1).data # = {"FirstName": "first name", "LastName": "last name"}
usage(deserializing):
data = { 'FirstName': 'John', 'LastName': 'Doe' }
serializer = UserSerializer(data=data)
serializer.is_valid()
valid_deserialized_data = serializer.validated_data # = { 'first_name': 'John', 'last_name': 'Doe' }
Try this:
class UserSerializer(serializers.Serializer):
FirstName = serializers.CharField(source="first_name")
LastName = serializers.CharField(source="last_name")
usage(serializing):
class Person:
first_name = "first name"
last_name = "last name"
person1 = Person()
serialized_data = UserSerializer(person1).data # = {"FirstName": "first name", "LastName": "last name"}
usage(deserializing):
data = { 'FirstName': 'John', 'LastName': 'Doe' }
serializer = UserSerializer(data=data)
serializer.is_valid()
valid_deserialized_data = serializer.validated_data # = { 'first_name': 'John', 'last_name': 'Doe' }
edited Nov 23 '18 at 20:55
answered Nov 23 '18 at 20:41


Ehsan NouriEhsan Nouri
1,15649
1,15649
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
add a comment |
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
This seems useful - but I was under the impression that the fields in the serializer should be my desired field names - not the other way around. I've added a use case to the question that hopefully clarifies. Can I still declare my serializer fields in snake case with your answer?
– Toby
Nov 23 '18 at 21:16
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
drf tries to get the value for each field using the declared name in the serializer, so you have to name the fields based on the names in the returned data of that API.
– Ehsan Nouri
Nov 23 '18 at 21:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452150%2fmodify-field-names-in-serializer-in-django-rest-framework%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6ujZdOVt7zMkjKZUxnaXn