Echoing Integers Using an Array with Java
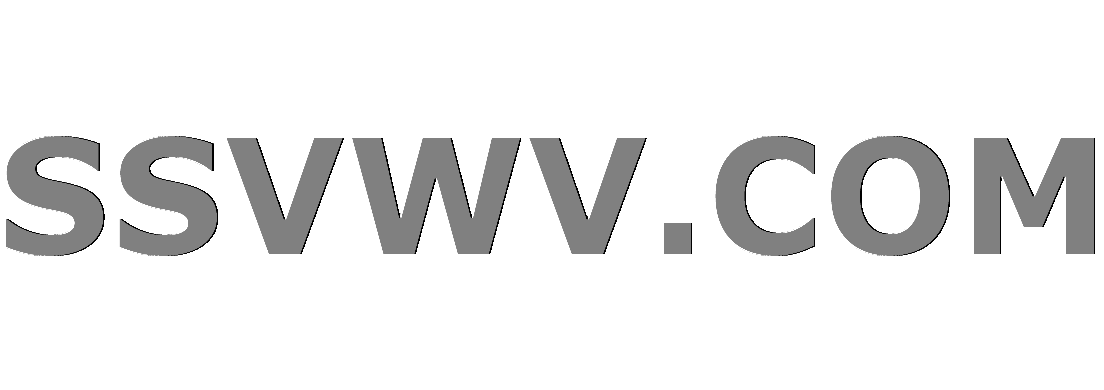
Multi tool use
up vote
0
down vote
favorite
I'm working to create a program that takes in 5 integers
via user input, separated by spaces on the same line. From there I need to assign these integers to an array
and then echo the array
back.
Should be pretty straightforward, but I am having a tough time where my echo back is not inserting spaces between the integers i.e, the users input is {1 2 3 4 5}
and the result is 12345
, which should ideally be {1 2 3 4 5}
.
This is my first opportunity to play with arrays
, so any assistance would be helpful!
Below is the code I have written thus far:
package echo5ints;
import java.util.Scanner;
/**
*
* @author laure
*/
public class U7D1_Echo5Ints {
/**
* @param args the command line arguments
*/
public static void main(String args) {
int i =0;
int arr=new int[5];
Scanner input = new Scanner(System.in);
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
Scanner numbers = new Scanner(line);
for(i=0;i<5;i++)
arr[i]=numbers.nextInt();
System.out.println("These were the 5 numbers entered: " + arr[0] + arr[1] + arr[2] + arr[3] + arr[4] );
}
}
java arrays integer echo
add a comment |
up vote
0
down vote
favorite
I'm working to create a program that takes in 5 integers
via user input, separated by spaces on the same line. From there I need to assign these integers to an array
and then echo the array
back.
Should be pretty straightforward, but I am having a tough time where my echo back is not inserting spaces between the integers i.e, the users input is {1 2 3 4 5}
and the result is 12345
, which should ideally be {1 2 3 4 5}
.
This is my first opportunity to play with arrays
, so any assistance would be helpful!
Below is the code I have written thus far:
package echo5ints;
import java.util.Scanner;
/**
*
* @author laure
*/
public class U7D1_Echo5Ints {
/**
* @param args the command line arguments
*/
public static void main(String args) {
int i =0;
int arr=new int[5];
Scanner input = new Scanner(System.in);
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
Scanner numbers = new Scanner(line);
for(i=0;i<5;i++)
arr[i]=numbers.nextInt();
System.out.println("These were the 5 numbers entered: " + arr[0] + arr[1] + arr[2] + arr[3] + arr[4] );
}
}
java arrays integer echo
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm working to create a program that takes in 5 integers
via user input, separated by spaces on the same line. From there I need to assign these integers to an array
and then echo the array
back.
Should be pretty straightforward, but I am having a tough time where my echo back is not inserting spaces between the integers i.e, the users input is {1 2 3 4 5}
and the result is 12345
, which should ideally be {1 2 3 4 5}
.
This is my first opportunity to play with arrays
, so any assistance would be helpful!
Below is the code I have written thus far:
package echo5ints;
import java.util.Scanner;
/**
*
* @author laure
*/
public class U7D1_Echo5Ints {
/**
* @param args the command line arguments
*/
public static void main(String args) {
int i =0;
int arr=new int[5];
Scanner input = new Scanner(System.in);
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
Scanner numbers = new Scanner(line);
for(i=0;i<5;i++)
arr[i]=numbers.nextInt();
System.out.println("These were the 5 numbers entered: " + arr[0] + arr[1] + arr[2] + arr[3] + arr[4] );
}
}
java arrays integer echo
I'm working to create a program that takes in 5 integers
via user input, separated by spaces on the same line. From there I need to assign these integers to an array
and then echo the array
back.
Should be pretty straightforward, but I am having a tough time where my echo back is not inserting spaces between the integers i.e, the users input is {1 2 3 4 5}
and the result is 12345
, which should ideally be {1 2 3 4 5}
.
This is my first opportunity to play with arrays
, so any assistance would be helpful!
Below is the code I have written thus far:
package echo5ints;
import java.util.Scanner;
/**
*
* @author laure
*/
public class U7D1_Echo5Ints {
/**
* @param args the command line arguments
*/
public static void main(String args) {
int i =0;
int arr=new int[5];
Scanner input = new Scanner(System.in);
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
Scanner numbers = new Scanner(line);
for(i=0;i<5;i++)
arr[i]=numbers.nextInt();
System.out.println("These were the 5 numbers entered: " + arr[0] + arr[1] + arr[2] + arr[3] + arr[4] );
}
}
java arrays integer echo
java arrays integer echo
edited Nov 22 at 6:54


Quick learner
2,3571824
2,3571824
asked Nov 22 at 6:32
Lauren Catherine Ayala
1
1
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
try using split
instead and printf
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
String arr = line.split (" ");
System.out.printf("These were the 5 numbers entered: %s %s %s %s %s",
arr[0], arr[1], arr[2], arr[3], arr[4] );
add a comment |
up vote
0
down vote
The spaces you seems to have added is for code and not for print statements.
Printf only print what appears inside quotes or the values held by variables.
SO your print line should be
System.out.println("These were the 5 numbers entered: " + arr[0] +" "+ arr[1] +" " + arr[2] +" " + arr[3] +" " + arr[4] );
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425089%2fechoing-integers-using-an-array-with-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
try using split
instead and printf
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
String arr = line.split (" ");
System.out.printf("These were the 5 numbers entered: %s %s %s %s %s",
arr[0], arr[1], arr[2], arr[3], arr[4] );
add a comment |
up vote
0
down vote
try using split
instead and printf
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
String arr = line.split (" ");
System.out.printf("These were the 5 numbers entered: %s %s %s %s %s",
arr[0], arr[1], arr[2], arr[3], arr[4] );
add a comment |
up vote
0
down vote
up vote
0
down vote
try using split
instead and printf
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
String arr = line.split (" ");
System.out.printf("These were the 5 numbers entered: %s %s %s %s %s",
arr[0], arr[1], arr[2], arr[3], arr[4] );
try using split
instead and printf
System.out.print("Please enter 5 numbers: ");
String line = input.nextLine();
String arr = line.split (" ");
System.out.printf("These were the 5 numbers entered: %s %s %s %s %s",
arr[0], arr[1], arr[2], arr[3], arr[4] );
answered Nov 22 at 6:34


Scary Wombat
34.9k32252
34.9k32252
add a comment |
add a comment |
up vote
0
down vote
The spaces you seems to have added is for code and not for print statements.
Printf only print what appears inside quotes or the values held by variables.
SO your print line should be
System.out.println("These were the 5 numbers entered: " + arr[0] +" "+ arr[1] +" " + arr[2] +" " + arr[3] +" " + arr[4] );
add a comment |
up vote
0
down vote
The spaces you seems to have added is for code and not for print statements.
Printf only print what appears inside quotes or the values held by variables.
SO your print line should be
System.out.println("These were the 5 numbers entered: " + arr[0] +" "+ arr[1] +" " + arr[2] +" " + arr[3] +" " + arr[4] );
add a comment |
up vote
0
down vote
up vote
0
down vote
The spaces you seems to have added is for code and not for print statements.
Printf only print what appears inside quotes or the values held by variables.
SO your print line should be
System.out.println("These were the 5 numbers entered: " + arr[0] +" "+ arr[1] +" " + arr[2] +" " + arr[3] +" " + arr[4] );
The spaces you seems to have added is for code and not for print statements.
Printf only print what appears inside quotes or the values held by variables.
SO your print line should be
System.out.println("These were the 5 numbers entered: " + arr[0] +" "+ arr[1] +" " + arr[2] +" " + arr[3] +" " + arr[4] );
answered Nov 22 at 6:37
CS_noob
4031311
4031311
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425089%2fechoing-integers-using-an-array-with-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q2ufZrc2ckEZeCGk,uqjltEoN D4 l,TQzEnalF8