C3js piechart label positioning and label backgound
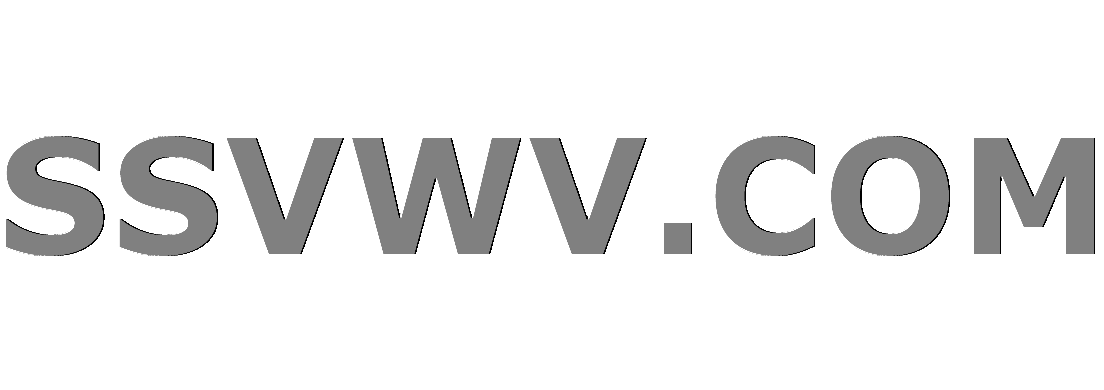
Multi tool use
I am trying to customize c3js piechart labels.
I want them to be placed outside the pie and have linkes coloured backgrounds.
This is how far I got:
var columns = ['data1', 'data2', 'data3', 'data4'];
var data = [150, 250, 300, 50];
var colors = ['#0065A3', '#767670', '#D73648', '#7FB2CE', '#00345B'];
var chart = c3.generate({
bindto: d3.select('#chart'),
data: {
columns: [[columns[0]].concat(data[0])],
type: 'pie',
},
legend: {
position: 'right',
show: true
},
pie: {
label: {
threshold: 0.001,
format: function(value, ratio, id) {
return [id, d3.format(",.0f")(value), "[" + d3.format(",.1%")(ratio) + "]"].join(';');
}
}
},
color: {
pattern: colors
},
onrendered: redrawLabelBackgrounds
});
function addLabelBackground(index) {
//get label text element
var textLabel = d3.select(".c3-target-" + columns[index] + " > text");
//add rect to parent
var labelNode = textLabel.node();
if (labelNode /*&& labelNode.innerHTML.length > 0*/ ) {
var p = d3.select(labelNode.parentNode).insert("rect", "text")
.style("fill", colors[index]);
}
}
addLabelBackground(0);
for (i = 1; i < columns.length; i++) {
setTimeout(function(column) {
chart.load({
columns: [
[columns[column]].concat(data[column]),
]
});
addLabelBackground(column);
}, (i * 5000 / columns.length), i);
}
function redrawLabelBackgrounds() {
//for all label texts drawn yet
d3.selectAll(".c3-chart-arc > text").each(function(v) {
// get d3 node
var labelNode = d3.select(this).node();
//check if label is drawn
if (labelNode && labelNode.innerHTML.length > 0) {
//build data
var data = labelNode.innerHTML.split(';');
d3.select(this).text("")
data.forEach(function(i, n) {
d3.select(this).append("tspan")
.text(i)
.attr("dy", (n === 0) ? 0 : "1.2em")
.attr("x", 0)
.attr("text-anchor", "middle");
}, this);
//get pos os the label text
var pos = d3.select(this).attr("transform").match(/-?d+(.d+)?/g);
//get surrounding box of the label
var bbox = d3.select(this).node().getBBox();
// TODO: mofify the pos of the text
//pos[0]=
//pos[1]=
//d3.select(this).attr("transform", "translate(" + pos[0] + "," + pos[1] + ")");
//now draw and move the rects
var padding = 5;
// TODO fix positioning bug
d3.select(labelNode.parentNode).select("rect")
.attr("transform", "translate(" + (pos[0] - (bbox.width + padding) / 2) + "," + (pos[1] - (bbox.height + padding) / 2) + ")")
.attr("width", function(d) {
return bbox.width + padding;
})
.attr("height", function(d) {
return bbox.height + padding;
});
}
});
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/d3/5.7.0/d3.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.js"></script>
<div id="chart">
</div>
So I am already familiar with
Label outside arc (Pie chart) c3.js
and therefor with https://github.com/c3js/c3/issues/1982 and Label outside arc (Pie chart) d3.js
I want to solve this without using D3 to draw the pie so I can't use d3.svg.arc()
to get the proper position/radius of the pie/arc.
(so I can use h = Math.sqrt(x*x + y*y);
and then return "translate(" + (x/h * labelr) + ',' +(y/h * labelr) + ")";
)
Also I do not know why the box is not in the right position. If i use labels which only have one line and no tspans the boxes are correct positioned...
edit: jsfiddle: http://jsfiddle.net/gothmogg/ckvo7ns4/12/
edit2: updated jsfiddle http://jsfiddle.net/ckvo7ns4/16/
positioning of label background does work now:
.attr("transform", "translate(" + (pos[0] - bbox.width/2 - padding/2) + "," + (pos[1] - bbox.height/data.length) + ")")
javascript d3.js svg pie-chart c3.js
add a comment |
I am trying to customize c3js piechart labels.
I want them to be placed outside the pie and have linkes coloured backgrounds.
This is how far I got:
var columns = ['data1', 'data2', 'data3', 'data4'];
var data = [150, 250, 300, 50];
var colors = ['#0065A3', '#767670', '#D73648', '#7FB2CE', '#00345B'];
var chart = c3.generate({
bindto: d3.select('#chart'),
data: {
columns: [[columns[0]].concat(data[0])],
type: 'pie',
},
legend: {
position: 'right',
show: true
},
pie: {
label: {
threshold: 0.001,
format: function(value, ratio, id) {
return [id, d3.format(",.0f")(value), "[" + d3.format(",.1%")(ratio) + "]"].join(';');
}
}
},
color: {
pattern: colors
},
onrendered: redrawLabelBackgrounds
});
function addLabelBackground(index) {
//get label text element
var textLabel = d3.select(".c3-target-" + columns[index] + " > text");
//add rect to parent
var labelNode = textLabel.node();
if (labelNode /*&& labelNode.innerHTML.length > 0*/ ) {
var p = d3.select(labelNode.parentNode).insert("rect", "text")
.style("fill", colors[index]);
}
}
addLabelBackground(0);
for (i = 1; i < columns.length; i++) {
setTimeout(function(column) {
chart.load({
columns: [
[columns[column]].concat(data[column]),
]
});
addLabelBackground(column);
}, (i * 5000 / columns.length), i);
}
function redrawLabelBackgrounds() {
//for all label texts drawn yet
d3.selectAll(".c3-chart-arc > text").each(function(v) {
// get d3 node
var labelNode = d3.select(this).node();
//check if label is drawn
if (labelNode && labelNode.innerHTML.length > 0) {
//build data
var data = labelNode.innerHTML.split(';');
d3.select(this).text("")
data.forEach(function(i, n) {
d3.select(this).append("tspan")
.text(i)
.attr("dy", (n === 0) ? 0 : "1.2em")
.attr("x", 0)
.attr("text-anchor", "middle");
}, this);
//get pos os the label text
var pos = d3.select(this).attr("transform").match(/-?d+(.d+)?/g);
//get surrounding box of the label
var bbox = d3.select(this).node().getBBox();
// TODO: mofify the pos of the text
//pos[0]=
//pos[1]=
//d3.select(this).attr("transform", "translate(" + pos[0] + "," + pos[1] + ")");
//now draw and move the rects
var padding = 5;
// TODO fix positioning bug
d3.select(labelNode.parentNode).select("rect")
.attr("transform", "translate(" + (pos[0] - (bbox.width + padding) / 2) + "," + (pos[1] - (bbox.height + padding) / 2) + ")")
.attr("width", function(d) {
return bbox.width + padding;
})
.attr("height", function(d) {
return bbox.height + padding;
});
}
});
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/d3/5.7.0/d3.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.js"></script>
<div id="chart">
</div>
So I am already familiar with
Label outside arc (Pie chart) c3.js
and therefor with https://github.com/c3js/c3/issues/1982 and Label outside arc (Pie chart) d3.js
I want to solve this without using D3 to draw the pie so I can't use d3.svg.arc()
to get the proper position/radius of the pie/arc.
(so I can use h = Math.sqrt(x*x + y*y);
and then return "translate(" + (x/h * labelr) + ',' +(y/h * labelr) + ")";
)
Also I do not know why the box is not in the right position. If i use labels which only have one line and no tspans the boxes are correct positioned...
edit: jsfiddle: http://jsfiddle.net/gothmogg/ckvo7ns4/12/
edit2: updated jsfiddle http://jsfiddle.net/ckvo7ns4/16/
positioning of label background does work now:
.attr("transform", "translate(" + (pos[0] - bbox.width/2 - padding/2) + "," + (pos[1] - bbox.height/data.length) + ")")
javascript d3.js svg pie-chart c3.js
add a comment |
I am trying to customize c3js piechart labels.
I want them to be placed outside the pie and have linkes coloured backgrounds.
This is how far I got:
var columns = ['data1', 'data2', 'data3', 'data4'];
var data = [150, 250, 300, 50];
var colors = ['#0065A3', '#767670', '#D73648', '#7FB2CE', '#00345B'];
var chart = c3.generate({
bindto: d3.select('#chart'),
data: {
columns: [[columns[0]].concat(data[0])],
type: 'pie',
},
legend: {
position: 'right',
show: true
},
pie: {
label: {
threshold: 0.001,
format: function(value, ratio, id) {
return [id, d3.format(",.0f")(value), "[" + d3.format(",.1%")(ratio) + "]"].join(';');
}
}
},
color: {
pattern: colors
},
onrendered: redrawLabelBackgrounds
});
function addLabelBackground(index) {
//get label text element
var textLabel = d3.select(".c3-target-" + columns[index] + " > text");
//add rect to parent
var labelNode = textLabel.node();
if (labelNode /*&& labelNode.innerHTML.length > 0*/ ) {
var p = d3.select(labelNode.parentNode).insert("rect", "text")
.style("fill", colors[index]);
}
}
addLabelBackground(0);
for (i = 1; i < columns.length; i++) {
setTimeout(function(column) {
chart.load({
columns: [
[columns[column]].concat(data[column]),
]
});
addLabelBackground(column);
}, (i * 5000 / columns.length), i);
}
function redrawLabelBackgrounds() {
//for all label texts drawn yet
d3.selectAll(".c3-chart-arc > text").each(function(v) {
// get d3 node
var labelNode = d3.select(this).node();
//check if label is drawn
if (labelNode && labelNode.innerHTML.length > 0) {
//build data
var data = labelNode.innerHTML.split(';');
d3.select(this).text("")
data.forEach(function(i, n) {
d3.select(this).append("tspan")
.text(i)
.attr("dy", (n === 0) ? 0 : "1.2em")
.attr("x", 0)
.attr("text-anchor", "middle");
}, this);
//get pos os the label text
var pos = d3.select(this).attr("transform").match(/-?d+(.d+)?/g);
//get surrounding box of the label
var bbox = d3.select(this).node().getBBox();
// TODO: mofify the pos of the text
//pos[0]=
//pos[1]=
//d3.select(this).attr("transform", "translate(" + pos[0] + "," + pos[1] + ")");
//now draw and move the rects
var padding = 5;
// TODO fix positioning bug
d3.select(labelNode.parentNode).select("rect")
.attr("transform", "translate(" + (pos[0] - (bbox.width + padding) / 2) + "," + (pos[1] - (bbox.height + padding) / 2) + ")")
.attr("width", function(d) {
return bbox.width + padding;
})
.attr("height", function(d) {
return bbox.height + padding;
});
}
});
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/d3/5.7.0/d3.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.js"></script>
<div id="chart">
</div>
So I am already familiar with
Label outside arc (Pie chart) c3.js
and therefor with https://github.com/c3js/c3/issues/1982 and Label outside arc (Pie chart) d3.js
I want to solve this without using D3 to draw the pie so I can't use d3.svg.arc()
to get the proper position/radius of the pie/arc.
(so I can use h = Math.sqrt(x*x + y*y);
and then return "translate(" + (x/h * labelr) + ',' +(y/h * labelr) + ")";
)
Also I do not know why the box is not in the right position. If i use labels which only have one line and no tspans the boxes are correct positioned...
edit: jsfiddle: http://jsfiddle.net/gothmogg/ckvo7ns4/12/
edit2: updated jsfiddle http://jsfiddle.net/ckvo7ns4/16/
positioning of label background does work now:
.attr("transform", "translate(" + (pos[0] - bbox.width/2 - padding/2) + "," + (pos[1] - bbox.height/data.length) + ")")
javascript d3.js svg pie-chart c3.js
I am trying to customize c3js piechart labels.
I want them to be placed outside the pie and have linkes coloured backgrounds.
This is how far I got:
var columns = ['data1', 'data2', 'data3', 'data4'];
var data = [150, 250, 300, 50];
var colors = ['#0065A3', '#767670', '#D73648', '#7FB2CE', '#00345B'];
var chart = c3.generate({
bindto: d3.select('#chart'),
data: {
columns: [[columns[0]].concat(data[0])],
type: 'pie',
},
legend: {
position: 'right',
show: true
},
pie: {
label: {
threshold: 0.001,
format: function(value, ratio, id) {
return [id, d3.format(",.0f")(value), "[" + d3.format(",.1%")(ratio) + "]"].join(';');
}
}
},
color: {
pattern: colors
},
onrendered: redrawLabelBackgrounds
});
function addLabelBackground(index) {
//get label text element
var textLabel = d3.select(".c3-target-" + columns[index] + " > text");
//add rect to parent
var labelNode = textLabel.node();
if (labelNode /*&& labelNode.innerHTML.length > 0*/ ) {
var p = d3.select(labelNode.parentNode).insert("rect", "text")
.style("fill", colors[index]);
}
}
addLabelBackground(0);
for (i = 1; i < columns.length; i++) {
setTimeout(function(column) {
chart.load({
columns: [
[columns[column]].concat(data[column]),
]
});
addLabelBackground(column);
}, (i * 5000 / columns.length), i);
}
function redrawLabelBackgrounds() {
//for all label texts drawn yet
d3.selectAll(".c3-chart-arc > text").each(function(v) {
// get d3 node
var labelNode = d3.select(this).node();
//check if label is drawn
if (labelNode && labelNode.innerHTML.length > 0) {
//build data
var data = labelNode.innerHTML.split(';');
d3.select(this).text("")
data.forEach(function(i, n) {
d3.select(this).append("tspan")
.text(i)
.attr("dy", (n === 0) ? 0 : "1.2em")
.attr("x", 0)
.attr("text-anchor", "middle");
}, this);
//get pos os the label text
var pos = d3.select(this).attr("transform").match(/-?d+(.d+)?/g);
//get surrounding box of the label
var bbox = d3.select(this).node().getBBox();
// TODO: mofify the pos of the text
//pos[0]=
//pos[1]=
//d3.select(this).attr("transform", "translate(" + pos[0] + "," + pos[1] + ")");
//now draw and move the rects
var padding = 5;
// TODO fix positioning bug
d3.select(labelNode.parentNode).select("rect")
.attr("transform", "translate(" + (pos[0] - (bbox.width + padding) / 2) + "," + (pos[1] - (bbox.height + padding) / 2) + ")")
.attr("width", function(d) {
return bbox.width + padding;
})
.attr("height", function(d) {
return bbox.height + padding;
});
}
});
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/d3/5.7.0/d3.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.js"></script>
<div id="chart">
</div>
So I am already familiar with
Label outside arc (Pie chart) c3.js
and therefor with https://github.com/c3js/c3/issues/1982 and Label outside arc (Pie chart) d3.js
I want to solve this without using D3 to draw the pie so I can't use d3.svg.arc()
to get the proper position/radius of the pie/arc.
(so I can use h = Math.sqrt(x*x + y*y);
and then return "translate(" + (x/h * labelr) + ',' +(y/h * labelr) + ")";
)
Also I do not know why the box is not in the right position. If i use labels which only have one line and no tspans the boxes are correct positioned...
edit: jsfiddle: http://jsfiddle.net/gothmogg/ckvo7ns4/12/
edit2: updated jsfiddle http://jsfiddle.net/ckvo7ns4/16/
positioning of label background does work now:
.attr("transform", "translate(" + (pos[0] - bbox.width/2 - padding/2) + "," + (pos[1] - bbox.height/data.length) + ")")
var columns = ['data1', 'data2', 'data3', 'data4'];
var data = [150, 250, 300, 50];
var colors = ['#0065A3', '#767670', '#D73648', '#7FB2CE', '#00345B'];
var chart = c3.generate({
bindto: d3.select('#chart'),
data: {
columns: [[columns[0]].concat(data[0])],
type: 'pie',
},
legend: {
position: 'right',
show: true
},
pie: {
label: {
threshold: 0.001,
format: function(value, ratio, id) {
return [id, d3.format(",.0f")(value), "[" + d3.format(",.1%")(ratio) + "]"].join(';');
}
}
},
color: {
pattern: colors
},
onrendered: redrawLabelBackgrounds
});
function addLabelBackground(index) {
//get label text element
var textLabel = d3.select(".c3-target-" + columns[index] + " > text");
//add rect to parent
var labelNode = textLabel.node();
if (labelNode /*&& labelNode.innerHTML.length > 0*/ ) {
var p = d3.select(labelNode.parentNode).insert("rect", "text")
.style("fill", colors[index]);
}
}
addLabelBackground(0);
for (i = 1; i < columns.length; i++) {
setTimeout(function(column) {
chart.load({
columns: [
[columns[column]].concat(data[column]),
]
});
addLabelBackground(column);
}, (i * 5000 / columns.length), i);
}
function redrawLabelBackgrounds() {
//for all label texts drawn yet
d3.selectAll(".c3-chart-arc > text").each(function(v) {
// get d3 node
var labelNode = d3.select(this).node();
//check if label is drawn
if (labelNode && labelNode.innerHTML.length > 0) {
//build data
var data = labelNode.innerHTML.split(';');
d3.select(this).text("")
data.forEach(function(i, n) {
d3.select(this).append("tspan")
.text(i)
.attr("dy", (n === 0) ? 0 : "1.2em")
.attr("x", 0)
.attr("text-anchor", "middle");
}, this);
//get pos os the label text
var pos = d3.select(this).attr("transform").match(/-?d+(.d+)?/g);
//get surrounding box of the label
var bbox = d3.select(this).node().getBBox();
// TODO: mofify the pos of the text
//pos[0]=
//pos[1]=
//d3.select(this).attr("transform", "translate(" + pos[0] + "," + pos[1] + ")");
//now draw and move the rects
var padding = 5;
// TODO fix positioning bug
d3.select(labelNode.parentNode).select("rect")
.attr("transform", "translate(" + (pos[0] - (bbox.width + padding) / 2) + "," + (pos[1] - (bbox.height + padding) / 2) + ")")
.attr("width", function(d) {
return bbox.width + padding;
})
.attr("height", function(d) {
return bbox.height + padding;
});
}
});
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/d3/5.7.0/d3.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.js"></script>
<div id="chart">
</div>
var columns = ['data1', 'data2', 'data3', 'data4'];
var data = [150, 250, 300, 50];
var colors = ['#0065A3', '#767670', '#D73648', '#7FB2CE', '#00345B'];
var chart = c3.generate({
bindto: d3.select('#chart'),
data: {
columns: [[columns[0]].concat(data[0])],
type: 'pie',
},
legend: {
position: 'right',
show: true
},
pie: {
label: {
threshold: 0.001,
format: function(value, ratio, id) {
return [id, d3.format(",.0f")(value), "[" + d3.format(",.1%")(ratio) + "]"].join(';');
}
}
},
color: {
pattern: colors
},
onrendered: redrawLabelBackgrounds
});
function addLabelBackground(index) {
//get label text element
var textLabel = d3.select(".c3-target-" + columns[index] + " > text");
//add rect to parent
var labelNode = textLabel.node();
if (labelNode /*&& labelNode.innerHTML.length > 0*/ ) {
var p = d3.select(labelNode.parentNode).insert("rect", "text")
.style("fill", colors[index]);
}
}
addLabelBackground(0);
for (i = 1; i < columns.length; i++) {
setTimeout(function(column) {
chart.load({
columns: [
[columns[column]].concat(data[column]),
]
});
addLabelBackground(column);
}, (i * 5000 / columns.length), i);
}
function redrawLabelBackgrounds() {
//for all label texts drawn yet
d3.selectAll(".c3-chart-arc > text").each(function(v) {
// get d3 node
var labelNode = d3.select(this).node();
//check if label is drawn
if (labelNode && labelNode.innerHTML.length > 0) {
//build data
var data = labelNode.innerHTML.split(';');
d3.select(this).text("")
data.forEach(function(i, n) {
d3.select(this).append("tspan")
.text(i)
.attr("dy", (n === 0) ? 0 : "1.2em")
.attr("x", 0)
.attr("text-anchor", "middle");
}, this);
//get pos os the label text
var pos = d3.select(this).attr("transform").match(/-?d+(.d+)?/g);
//get surrounding box of the label
var bbox = d3.select(this).node().getBBox();
// TODO: mofify the pos of the text
//pos[0]=
//pos[1]=
//d3.select(this).attr("transform", "translate(" + pos[0] + "," + pos[1] + ")");
//now draw and move the rects
var padding = 5;
// TODO fix positioning bug
d3.select(labelNode.parentNode).select("rect")
.attr("transform", "translate(" + (pos[0] - (bbox.width + padding) / 2) + "," + (pos[1] - (bbox.height + padding) / 2) + ")")
.attr("width", function(d) {
return bbox.width + padding;
})
.attr("height", function(d) {
return bbox.height + padding;
});
}
});
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/d3/5.7.0/d3.min.js"></script>
<link href="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/c3/0.6.8/c3.min.js"></script>
<div id="chart">
</div>
javascript d3.js svg pie-chart c3.js
javascript d3.js svg pie-chart c3.js
edited Nov 27 at 15:05
asked Nov 22 at 14:20


gothmogg
34
34
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432955%2fc3js-piechart-label-positioning-and-label-backgound%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432955%2fc3js-piechart-label-positioning-and-label-backgound%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LOeMb20yYoEYAk6vCrtTqY8oXDLt6LB5RObvnFCw9LsPyAq93N,U0vnGKPtq6 nF2sMf9mN,BSYU,ZH4Kwlc