Extract dataURL from Javascript function
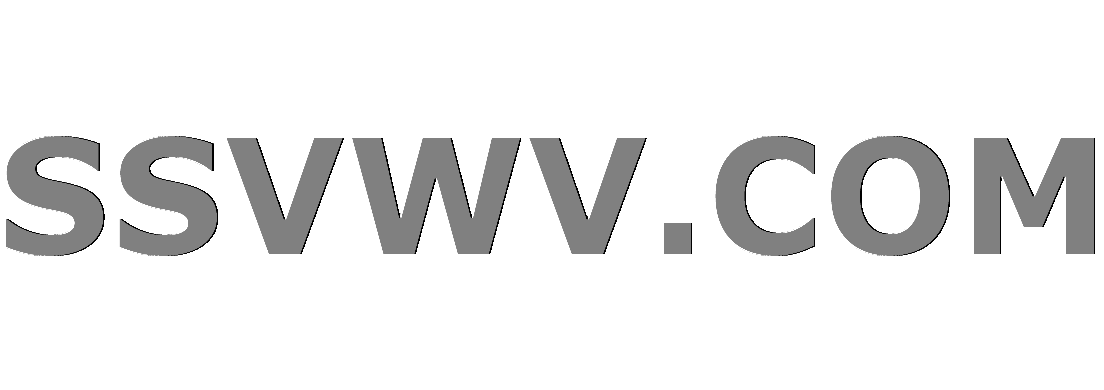
Multi tool use
up vote
0
down vote
favorite
I have a function that aims to create a dataURL of a file using the FileReader. Considering this example.
toDataURL(url, callback){
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
}
I want to extract the variable this.result
in the inline function as a return variable. It has not acces from other function. How can I do it?
javascript
add a comment |
up vote
0
down vote
favorite
I have a function that aims to create a dataURL of a file using the FileReader. Considering this example.
toDataURL(url, callback){
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
}
I want to extract the variable this.result
in the inline function as a return variable. It has not acces from other function. How can I do it?
javascript
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a function that aims to create a dataURL of a file using the FileReader. Considering this example.
toDataURL(url, callback){
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
}
I want to extract the variable this.result
in the inline function as a return variable. It has not acces from other function. How can I do it?
javascript
I have a function that aims to create a dataURL of a file using the FileReader. Considering this example.
toDataURL(url, callback){
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
}
I want to extract the variable this.result
in the inline function as a return variable. It has not acces from other function. How can I do it?
javascript
javascript
asked Nov 21 at 15:33
Kuler Can
61
61
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Basicaly, what you want to do is return a value from an async function, you can do this with Promises, and optionally async/await.
Check this out:
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
add a comment |
up vote
0
down vote
You could try to use Promise
, like this:
toDataURL(url){
var promise = new Promise(function (extract) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
extract(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
});
return promise;
}
(async function () {
var result = await toDataURL('/someurl');
console.log(result);
}());
Inside the toDataURL
function, we create a promise. When returning the result, we just need to put it inside extract
method.
Then, we create an asynchronous function to use await
keyword to get the result directly.
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Basicaly, what you want to do is return a value from an async function, you can do this with Promises, and optionally async/await.
Check this out:
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
add a comment |
up vote
0
down vote
Basicaly, what you want to do is return a value from an async function, you can do this with Promises, and optionally async/await.
Check this out:
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
add a comment |
up vote
0
down vote
up vote
0
down vote
Basicaly, what you want to do is return a value from an async function, you can do this with Promises, and optionally async/await.
Check this out:
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
Basicaly, what you want to do is return a value from an async function, you can do this with Promises, and optionally async/await.
Check this out:
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
function toDataURL(url, callback) {
return new Promise( function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function() {
var fr = new FileReader();
var test = fr.onload = function() {
resolve(this.result)
callback(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.onerror = function() {
reject('Error);
}
xhr.send();
});
}
const result = await toDataURL('some url');
// or
toDataURL('some url').then((result) => {
// do something with the result
});
answered Nov 21 at 15:39


rubentd
986821
986821
add a comment |
add a comment |
up vote
0
down vote
You could try to use Promise
, like this:
toDataURL(url){
var promise = new Promise(function (extract) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
extract(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
});
return promise;
}
(async function () {
var result = await toDataURL('/someurl');
console.log(result);
}());
Inside the toDataURL
function, we create a promise. When returning the result, we just need to put it inside extract
method.
Then, we create an asynchronous function to use await
keyword to get the result directly.
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
add a comment |
up vote
0
down vote
You could try to use Promise
, like this:
toDataURL(url){
var promise = new Promise(function (extract) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
extract(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
});
return promise;
}
(async function () {
var result = await toDataURL('/someurl');
console.log(result);
}());
Inside the toDataURL
function, we create a promise. When returning the result, we just need to put it inside extract
method.
Then, we create an asynchronous function to use await
keyword to get the result directly.
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
add a comment |
up vote
0
down vote
up vote
0
down vote
You could try to use Promise
, like this:
toDataURL(url){
var promise = new Promise(function (extract) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
extract(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
});
return promise;
}
(async function () {
var result = await toDataURL('/someurl');
console.log(result);
}());
Inside the toDataURL
function, we create a promise. When returning the result, we just need to put it inside extract
method.
Then, we create an asynchronous function to use await
keyword to get the result directly.
You could try to use Promise
, like this:
toDataURL(url){
var promise = new Promise(function (extract) {
var xhr = new XMLHttpRequest();
xhr.open('get', url);
xhr.responseType = 'blob';
xhr.onload = function(){
var fr = new FileReader();
var test = fr.onload = function(){
extract(this.result);
};
fr.readAsDataURL(xhr.response); // async call
};
xhr.send();
});
return promise;
}
(async function () {
var result = await toDataURL('/someurl');
console.log(result);
}());
Inside the toDataURL
function, we create a promise. When returning the result, we just need to put it inside extract
method.
Then, we create an asynchronous function to use await
keyword to get the result directly.
answered Nov 21 at 15:40


Foo
1
1
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
add a comment |
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
I have an other questio. For Typescrippt, how can I change the global variable in the promise function?
– Kuler Can
Nov 27 at 9:20
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53415469%2fextract-dataurl-from-javascript-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W 3QrBBQfDot,1bJOp,mhFKPGJtE0I,BV8 TYXlIKN VaNwY