Getting “out-of-bounds” and “variable uninitialized” warnings with mapped shared memory
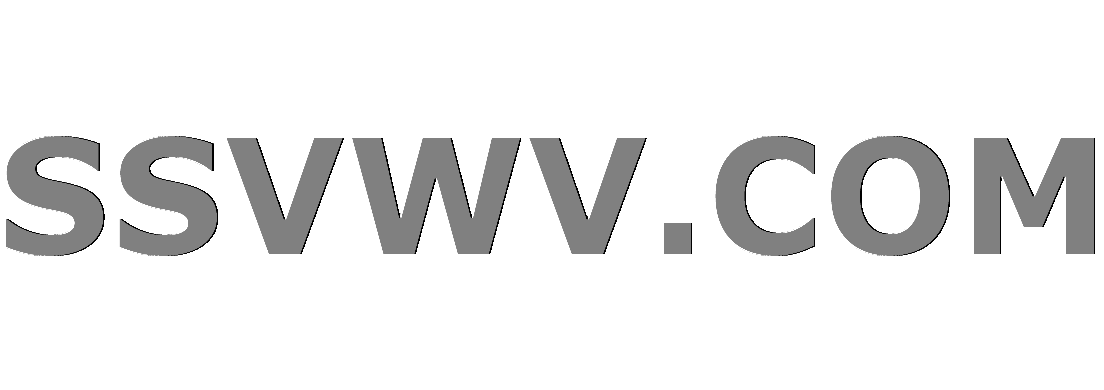
Multi tool use
up vote
0
down vote
favorite
As part of my C application for Linux, I wrote a small library to allow different processes to communicate through shared memory areas.
I am having lots of alerts for potential out-of-bounds pointers and potentially uninitialized variables contained in the memory areas I create this way.
Consider the function I wrote to create a shared memory area:
int LayOutShm (const char *shm_key, size_t memory_size, void ** new_memory)
{
int fd_value = 0;
void * pshm = NULL;
int return_value = MEM_MGMT_SHM_ERROR;
/* Force new_buffer pointer to NULL just like we initialize return_value to MEM_MGMT_SHM_ERROR */
*new_memory = NULL;
/* Shared object is created with O_EXCL to prevent two namesake areas from being created*/
fd_value = shm_open(shm_key, O_CREAT | O_RDWR | O_EXCL , S_IRWXU);
if(fd_value > 0){
if(ftruncate(fd_value, memory_size) == 0){
/* Here is where we get the pointer to the created memory area */
pshm = mmap(NULL, memory_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd_value, 0);
if(pshm != MAP_FAILED){
return_value = MEM_MGMT_SHM_OK;
memset(pshm,0,memory_size); /* Initialize the memory area */
*new_memory = pshm; /* Pass the pointer back to the caller */
}
}
}
return return_value;
}/*LayOutShm*/
Now, this is some piece of code were I get warnings:
#define SHM_KEY "my_shm_key"
typedef struct{
pthread_mutex_t shm_mutex;
int shm_var1;
char shm_var2;
union{
int shm_union_var1;
char shm_union_var2;
}shm_union
}t_shm_area;
static t_shm_area * shm_area = NULL;
static int ShmInitialization(void)
{
int return_value = -1;
(void)LayOutShm(SHM_KEY, sizeof(t_shm_area), (void**)&shm_area);
/*Check for errors in shared memory creation*/
if (shm_area == NULL){
syslog(LOG_ERR | LOG_USER, "Error laying out shared memory segmentn");
}
else{
shm_area->var1 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
shm_area->shm_union.shm_union_var2 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
/*Create empty attributes structure*/
pthread_mutexattr_t mutex_attributes;
/*Initialize attributes structures with default values*/
(void)pthread_mutexattr_init(&mutex_attributes);
/*Set attributes structure with shared memory value*/
(void)pthread_mutexattr_setpshared(&mutex_attributes, PTHREAD_PROCESS_SHARED);
/*settype mutex PTHREAD_MUTEX_ERRORCHECK*/
(void)pthread_mutexattr_settype(&mutex_attributes, PTHREAD_MUTEX_ERRORCHECK);
/*Initialize the mutex with all the previous attributes*/
(void)pthread_mutex_init(&shm_area->shm_mutex, &mutex_attributes);
return_value = 0;
}
return return_value;
}/*ShmInitialization*/
This is the first time I try my hand at double pointers, so I wouldn't be surprised if I were screwing things up with either that void ** in the declaration or the way I cast the input double pointer to void.
A previous implementation of the function returned the pointer directly and didn't generate these problems, but I was asked to change it so that we can return a status code (even if we don't use them for now).
After that area is created, any locally declared variable I pass to the library to get values from the shared memory gets "tainted" with warnings from potential "out-of-bounds" runtime errors or "variable might not be initialized" warnings. The library has already been tested with several different types of data structures (some of them as big as 15kB) and data integrity and performance are satisfying.
Any idea why am I getting these warnings?
Thank you very much, best regards!
c pointers shared-memory void-pointers
add a comment |
up vote
0
down vote
favorite
As part of my C application for Linux, I wrote a small library to allow different processes to communicate through shared memory areas.
I am having lots of alerts for potential out-of-bounds pointers and potentially uninitialized variables contained in the memory areas I create this way.
Consider the function I wrote to create a shared memory area:
int LayOutShm (const char *shm_key, size_t memory_size, void ** new_memory)
{
int fd_value = 0;
void * pshm = NULL;
int return_value = MEM_MGMT_SHM_ERROR;
/* Force new_buffer pointer to NULL just like we initialize return_value to MEM_MGMT_SHM_ERROR */
*new_memory = NULL;
/* Shared object is created with O_EXCL to prevent two namesake areas from being created*/
fd_value = shm_open(shm_key, O_CREAT | O_RDWR | O_EXCL , S_IRWXU);
if(fd_value > 0){
if(ftruncate(fd_value, memory_size) == 0){
/* Here is where we get the pointer to the created memory area */
pshm = mmap(NULL, memory_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd_value, 0);
if(pshm != MAP_FAILED){
return_value = MEM_MGMT_SHM_OK;
memset(pshm,0,memory_size); /* Initialize the memory area */
*new_memory = pshm; /* Pass the pointer back to the caller */
}
}
}
return return_value;
}/*LayOutShm*/
Now, this is some piece of code were I get warnings:
#define SHM_KEY "my_shm_key"
typedef struct{
pthread_mutex_t shm_mutex;
int shm_var1;
char shm_var2;
union{
int shm_union_var1;
char shm_union_var2;
}shm_union
}t_shm_area;
static t_shm_area * shm_area = NULL;
static int ShmInitialization(void)
{
int return_value = -1;
(void)LayOutShm(SHM_KEY, sizeof(t_shm_area), (void**)&shm_area);
/*Check for errors in shared memory creation*/
if (shm_area == NULL){
syslog(LOG_ERR | LOG_USER, "Error laying out shared memory segmentn");
}
else{
shm_area->var1 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
shm_area->shm_union.shm_union_var2 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
/*Create empty attributes structure*/
pthread_mutexattr_t mutex_attributes;
/*Initialize attributes structures with default values*/
(void)pthread_mutexattr_init(&mutex_attributes);
/*Set attributes structure with shared memory value*/
(void)pthread_mutexattr_setpshared(&mutex_attributes, PTHREAD_PROCESS_SHARED);
/*settype mutex PTHREAD_MUTEX_ERRORCHECK*/
(void)pthread_mutexattr_settype(&mutex_attributes, PTHREAD_MUTEX_ERRORCHECK);
/*Initialize the mutex with all the previous attributes*/
(void)pthread_mutex_init(&shm_area->shm_mutex, &mutex_attributes);
return_value = 0;
}
return return_value;
}/*ShmInitialization*/
This is the first time I try my hand at double pointers, so I wouldn't be surprised if I were screwing things up with either that void ** in the declaration or the way I cast the input double pointer to void.
A previous implementation of the function returned the pointer directly and didn't generate these problems, but I was asked to change it so that we can return a status code (even if we don't use them for now).
After that area is created, any locally declared variable I pass to the library to get values from the shared memory gets "tainted" with warnings from potential "out-of-bounds" runtime errors or "variable might not be initialized" warnings. The library has already been tested with several different types of data structures (some of them as big as 15kB) and data integrity and performance are satisfying.
Any idea why am I getting these warnings?
Thank you very much, best regards!
c pointers shared-memory void-pointers
1
Probably because there is no such thing as a generic pointer-to-pointer.void**
is not compatible witht_shm_area **
. The only generic pointer type isvoid*
. So you need a step in between:void* v = shm_area;
.LayOutShm(...&v); shm_area = v;
– Lundin
Nov 21 at 10:34
That makes a lot of sense! Should I cast "v" when assigning it back to shm_area? As inshm_area = (t_shm_area *)v;
– Jorge Juan Torres Quiroga
Nov 21 at 11:21
1
No, that's not needed when converting to/from void pointers.
– Lundin
Nov 21 at 11:27
I'll change the code accordingly and check the functionality plus the static analysis reports. I'll be back with them as soon as they are available. Thank you very much!
– Jorge Juan Torres Quiroga
Nov 21 at 11:32
I am glad to report that @Lundin solution worked like a charm! Please, post your comment as an answer so I can give it the green tick ^^ Thank you!
– Jorge Juan Torres Quiroga
Nov 21 at 13:19
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
As part of my C application for Linux, I wrote a small library to allow different processes to communicate through shared memory areas.
I am having lots of alerts for potential out-of-bounds pointers and potentially uninitialized variables contained in the memory areas I create this way.
Consider the function I wrote to create a shared memory area:
int LayOutShm (const char *shm_key, size_t memory_size, void ** new_memory)
{
int fd_value = 0;
void * pshm = NULL;
int return_value = MEM_MGMT_SHM_ERROR;
/* Force new_buffer pointer to NULL just like we initialize return_value to MEM_MGMT_SHM_ERROR */
*new_memory = NULL;
/* Shared object is created with O_EXCL to prevent two namesake areas from being created*/
fd_value = shm_open(shm_key, O_CREAT | O_RDWR | O_EXCL , S_IRWXU);
if(fd_value > 0){
if(ftruncate(fd_value, memory_size) == 0){
/* Here is where we get the pointer to the created memory area */
pshm = mmap(NULL, memory_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd_value, 0);
if(pshm != MAP_FAILED){
return_value = MEM_MGMT_SHM_OK;
memset(pshm,0,memory_size); /* Initialize the memory area */
*new_memory = pshm; /* Pass the pointer back to the caller */
}
}
}
return return_value;
}/*LayOutShm*/
Now, this is some piece of code were I get warnings:
#define SHM_KEY "my_shm_key"
typedef struct{
pthread_mutex_t shm_mutex;
int shm_var1;
char shm_var2;
union{
int shm_union_var1;
char shm_union_var2;
}shm_union
}t_shm_area;
static t_shm_area * shm_area = NULL;
static int ShmInitialization(void)
{
int return_value = -1;
(void)LayOutShm(SHM_KEY, sizeof(t_shm_area), (void**)&shm_area);
/*Check for errors in shared memory creation*/
if (shm_area == NULL){
syslog(LOG_ERR | LOG_USER, "Error laying out shared memory segmentn");
}
else{
shm_area->var1 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
shm_area->shm_union.shm_union_var2 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
/*Create empty attributes structure*/
pthread_mutexattr_t mutex_attributes;
/*Initialize attributes structures with default values*/
(void)pthread_mutexattr_init(&mutex_attributes);
/*Set attributes structure with shared memory value*/
(void)pthread_mutexattr_setpshared(&mutex_attributes, PTHREAD_PROCESS_SHARED);
/*settype mutex PTHREAD_MUTEX_ERRORCHECK*/
(void)pthread_mutexattr_settype(&mutex_attributes, PTHREAD_MUTEX_ERRORCHECK);
/*Initialize the mutex with all the previous attributes*/
(void)pthread_mutex_init(&shm_area->shm_mutex, &mutex_attributes);
return_value = 0;
}
return return_value;
}/*ShmInitialization*/
This is the first time I try my hand at double pointers, so I wouldn't be surprised if I were screwing things up with either that void ** in the declaration or the way I cast the input double pointer to void.
A previous implementation of the function returned the pointer directly and didn't generate these problems, but I was asked to change it so that we can return a status code (even if we don't use them for now).
After that area is created, any locally declared variable I pass to the library to get values from the shared memory gets "tainted" with warnings from potential "out-of-bounds" runtime errors or "variable might not be initialized" warnings. The library has already been tested with several different types of data structures (some of them as big as 15kB) and data integrity and performance are satisfying.
Any idea why am I getting these warnings?
Thank you very much, best regards!
c pointers shared-memory void-pointers
As part of my C application for Linux, I wrote a small library to allow different processes to communicate through shared memory areas.
I am having lots of alerts for potential out-of-bounds pointers and potentially uninitialized variables contained in the memory areas I create this way.
Consider the function I wrote to create a shared memory area:
int LayOutShm (const char *shm_key, size_t memory_size, void ** new_memory)
{
int fd_value = 0;
void * pshm = NULL;
int return_value = MEM_MGMT_SHM_ERROR;
/* Force new_buffer pointer to NULL just like we initialize return_value to MEM_MGMT_SHM_ERROR */
*new_memory = NULL;
/* Shared object is created with O_EXCL to prevent two namesake areas from being created*/
fd_value = shm_open(shm_key, O_CREAT | O_RDWR | O_EXCL , S_IRWXU);
if(fd_value > 0){
if(ftruncate(fd_value, memory_size) == 0){
/* Here is where we get the pointer to the created memory area */
pshm = mmap(NULL, memory_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd_value, 0);
if(pshm != MAP_FAILED){
return_value = MEM_MGMT_SHM_OK;
memset(pshm,0,memory_size); /* Initialize the memory area */
*new_memory = pshm; /* Pass the pointer back to the caller */
}
}
}
return return_value;
}/*LayOutShm*/
Now, this is some piece of code were I get warnings:
#define SHM_KEY "my_shm_key"
typedef struct{
pthread_mutex_t shm_mutex;
int shm_var1;
char shm_var2;
union{
int shm_union_var1;
char shm_union_var2;
}shm_union
}t_shm_area;
static t_shm_area * shm_area = NULL;
static int ShmInitialization(void)
{
int return_value = -1;
(void)LayOutShm(SHM_KEY, sizeof(t_shm_area), (void**)&shm_area);
/*Check for errors in shared memory creation*/
if (shm_area == NULL){
syslog(LOG_ERR | LOG_USER, "Error laying out shared memory segmentn");
}
else{
shm_area->var1 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
shm_area->shm_union.shm_union_var2 = 0; /* This assignment gets flagged with a potential out-of-bounds runtime error */
/*Create empty attributes structure*/
pthread_mutexattr_t mutex_attributes;
/*Initialize attributes structures with default values*/
(void)pthread_mutexattr_init(&mutex_attributes);
/*Set attributes structure with shared memory value*/
(void)pthread_mutexattr_setpshared(&mutex_attributes, PTHREAD_PROCESS_SHARED);
/*settype mutex PTHREAD_MUTEX_ERRORCHECK*/
(void)pthread_mutexattr_settype(&mutex_attributes, PTHREAD_MUTEX_ERRORCHECK);
/*Initialize the mutex with all the previous attributes*/
(void)pthread_mutex_init(&shm_area->shm_mutex, &mutex_attributes);
return_value = 0;
}
return return_value;
}/*ShmInitialization*/
This is the first time I try my hand at double pointers, so I wouldn't be surprised if I were screwing things up with either that void ** in the declaration or the way I cast the input double pointer to void.
A previous implementation of the function returned the pointer directly and didn't generate these problems, but I was asked to change it so that we can return a status code (even if we don't use them for now).
After that area is created, any locally declared variable I pass to the library to get values from the shared memory gets "tainted" with warnings from potential "out-of-bounds" runtime errors or "variable might not be initialized" warnings. The library has already been tested with several different types of data structures (some of them as big as 15kB) and data integrity and performance are satisfying.
Any idea why am I getting these warnings?
Thank you very much, best regards!
c pointers shared-memory void-pointers
c pointers shared-memory void-pointers
asked Nov 21 at 10:24
Jorge Juan Torres Quiroga
469
469
1
Probably because there is no such thing as a generic pointer-to-pointer.void**
is not compatible witht_shm_area **
. The only generic pointer type isvoid*
. So you need a step in between:void* v = shm_area;
.LayOutShm(...&v); shm_area = v;
– Lundin
Nov 21 at 10:34
That makes a lot of sense! Should I cast "v" when assigning it back to shm_area? As inshm_area = (t_shm_area *)v;
– Jorge Juan Torres Quiroga
Nov 21 at 11:21
1
No, that's not needed when converting to/from void pointers.
– Lundin
Nov 21 at 11:27
I'll change the code accordingly and check the functionality plus the static analysis reports. I'll be back with them as soon as they are available. Thank you very much!
– Jorge Juan Torres Quiroga
Nov 21 at 11:32
I am glad to report that @Lundin solution worked like a charm! Please, post your comment as an answer so I can give it the green tick ^^ Thank you!
– Jorge Juan Torres Quiroga
Nov 21 at 13:19
add a comment |
1
Probably because there is no such thing as a generic pointer-to-pointer.void**
is not compatible witht_shm_area **
. The only generic pointer type isvoid*
. So you need a step in between:void* v = shm_area;
.LayOutShm(...&v); shm_area = v;
– Lundin
Nov 21 at 10:34
That makes a lot of sense! Should I cast "v" when assigning it back to shm_area? As inshm_area = (t_shm_area *)v;
– Jorge Juan Torres Quiroga
Nov 21 at 11:21
1
No, that's not needed when converting to/from void pointers.
– Lundin
Nov 21 at 11:27
I'll change the code accordingly and check the functionality plus the static analysis reports. I'll be back with them as soon as they are available. Thank you very much!
– Jorge Juan Torres Quiroga
Nov 21 at 11:32
I am glad to report that @Lundin solution worked like a charm! Please, post your comment as an answer so I can give it the green tick ^^ Thank you!
– Jorge Juan Torres Quiroga
Nov 21 at 13:19
1
1
Probably because there is no such thing as a generic pointer-to-pointer.
void**
is not compatible with t_shm_area **
. The only generic pointer type is void*
. So you need a step in between: void* v = shm_area;
. LayOutShm(...&v); shm_area = v;
– Lundin
Nov 21 at 10:34
Probably because there is no such thing as a generic pointer-to-pointer.
void**
is not compatible with t_shm_area **
. The only generic pointer type is void*
. So you need a step in between: void* v = shm_area;
. LayOutShm(...&v); shm_area = v;
– Lundin
Nov 21 at 10:34
That makes a lot of sense! Should I cast "v" when assigning it back to shm_area? As in
shm_area = (t_shm_area *)v;
– Jorge Juan Torres Quiroga
Nov 21 at 11:21
That makes a lot of sense! Should I cast "v" when assigning it back to shm_area? As in
shm_area = (t_shm_area *)v;
– Jorge Juan Torres Quiroga
Nov 21 at 11:21
1
1
No, that's not needed when converting to/from void pointers.
– Lundin
Nov 21 at 11:27
No, that's not needed when converting to/from void pointers.
– Lundin
Nov 21 at 11:27
I'll change the code accordingly and check the functionality plus the static analysis reports. I'll be back with them as soon as they are available. Thank you very much!
– Jorge Juan Torres Quiroga
Nov 21 at 11:32
I'll change the code accordingly and check the functionality plus the static analysis reports. I'll be back with them as soon as they are available. Thank you very much!
– Jorge Juan Torres Quiroga
Nov 21 at 11:32
I am glad to report that @Lundin solution worked like a charm! Please, post your comment as an answer so I can give it the green tick ^^ Thank you!
– Jorge Juan Torres Quiroga
Nov 21 at 13:19
I am glad to report that @Lundin solution worked like a charm! Please, post your comment as an answer so I can give it the green tick ^^ Thank you!
– Jorge Juan Torres Quiroga
Nov 21 at 13:19
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
The generic pointer type in C is void*
. There is however no generic pointer-to-pointer type void**
. So the cast (void**)&shm_area
is a cast between non-compatible pointer types. Technically this is undefined behavior (strict aliasing violation), so anything can happen.
To fix this, use a temporary void*
for the parameter passing:
void* vptr = shm_area;
LayOutShm(...&vptr);
shm_area = vptr;
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The generic pointer type in C is void*
. There is however no generic pointer-to-pointer type void**
. So the cast (void**)&shm_area
is a cast between non-compatible pointer types. Technically this is undefined behavior (strict aliasing violation), so anything can happen.
To fix this, use a temporary void*
for the parameter passing:
void* vptr = shm_area;
LayOutShm(...&vptr);
shm_area = vptr;
add a comment |
up vote
1
down vote
accepted
The generic pointer type in C is void*
. There is however no generic pointer-to-pointer type void**
. So the cast (void**)&shm_area
is a cast between non-compatible pointer types. Technically this is undefined behavior (strict aliasing violation), so anything can happen.
To fix this, use a temporary void*
for the parameter passing:
void* vptr = shm_area;
LayOutShm(...&vptr);
shm_area = vptr;
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The generic pointer type in C is void*
. There is however no generic pointer-to-pointer type void**
. So the cast (void**)&shm_area
is a cast between non-compatible pointer types. Technically this is undefined behavior (strict aliasing violation), so anything can happen.
To fix this, use a temporary void*
for the parameter passing:
void* vptr = shm_area;
LayOutShm(...&vptr);
shm_area = vptr;
The generic pointer type in C is void*
. There is however no generic pointer-to-pointer type void**
. So the cast (void**)&shm_area
is a cast between non-compatible pointer types. Technically this is undefined behavior (strict aliasing violation), so anything can happen.
To fix this, use a temporary void*
for the parameter passing:
void* vptr = shm_area;
LayOutShm(...&vptr);
shm_area = vptr;
answered Nov 21 at 14:04
Lundin
105k17154258
105k17154258
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53409956%2fgetting-out-of-bounds-and-variable-uninitialized-warnings-with-mapped-shared%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vbS pz9xsj,oqH,7UYXHPl wrpkYWUk 1H
1
Probably because there is no such thing as a generic pointer-to-pointer.
void**
is not compatible witht_shm_area **
. The only generic pointer type isvoid*
. So you need a step in between:void* v = shm_area;
.LayOutShm(...&v); shm_area = v;
– Lundin
Nov 21 at 10:34
That makes a lot of sense! Should I cast "v" when assigning it back to shm_area? As in
shm_area = (t_shm_area *)v;
– Jorge Juan Torres Quiroga
Nov 21 at 11:21
1
No, that's not needed when converting to/from void pointers.
– Lundin
Nov 21 at 11:27
I'll change the code accordingly and check the functionality plus the static analysis reports. I'll be back with them as soon as they are available. Thank you very much!
– Jorge Juan Torres Quiroga
Nov 21 at 11:32
I am glad to report that @Lundin solution worked like a charm! Please, post your comment as an answer so I can give it the green tick ^^ Thank you!
– Jorge Juan Torres Quiroga
Nov 21 at 13:19