Failing OpenID Connect middleware - how to debug?
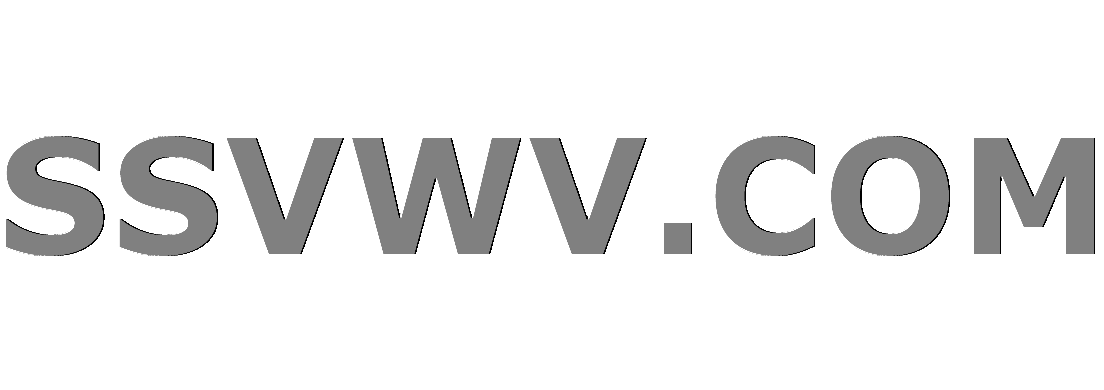
Multi tool use
up vote
2
down vote
favorite
I set up a very simple ASP.NET MVC 5 application that tries to authenticate a user through an OpenID provider in Authorization Code mode.
I'm able to log in and the server returns a code in the redirect URL querystring and a nonce cookie. However, back on the client application the user is not authenticated (User.Identity.IsAuthenticated
false), has no claims and called controller Action that has an Authorize
attribute is never carried out. Browser stays on the redirect URL page which is the home page.
I think something happens during the execution of the OpenID Connect middleware which makes it stop halfway through, but can't quite figure out how to debug it.
No exceptions are thrown even in "break on all CLR exceptions" mode.
When connecting an EventListener to
IdentityModelEventSource.Logger
at Verbose level I only get one logged event that says "Generating nonce for openIdConnect message", once per authentication attempt.No
Notification
hooks are reached exceptRedirectToIdentityProvider
, so it looks like no authorization code or security token is received, but the authentication doesn't fail either.
How can I get more info on what happens so that I can debug my problem?
Here's the code:
public void Configuration(IAppBuilder app)
{
var clientSecret = "secret";
var authenticationOptions = new OpenIdConnectAuthenticationOptions
{
ClientId = "id",
ClientSecret = clientSecret,
Authority = "https://theauthority",
RedirectUri = "https://localhost/MyApp/",
};
authenticationOptions.ResponseType = OpenIdConnectResponseType.Code; // Authorization code
authenticationOptions.TokenValidationParameters.IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(clientSecret));
authenticationOptions.TokenValidationParameters.RequireSignedTokens = true;
authenticationOptions.TokenValidationParameters.ValidAudience = "katanaclient";
authenticationOptions.SignInAsAuthenticationType = "Cookies";
authenticationOptions.Configuration = new OpenIdConnectConfiguration
{
Issuer = "https://theissuer",
AuthorizationEndpoint = "https://theendpoint",
TokenEndpoint = "https://theendpoint/api/v1/token",
UserInfoEndpoint = "https://theendpoint/api/v1/userinfo",
EndSessionEndpoint = "https://theendpoint/api/v1/logout",
ScopesSupported = { "openid", "profile"},
};
authenticationOptions.Notifications = new OpenIdConnectAuthenticationNotifications
{
RedirectToIdentityProvider = async n =>
{
// here it goes
if (n.ProtocolMessage.RequestType == OpenIdConnectRequestType.Authentication)
{
n.ProtocolMessage.EnableTelemetryParameters = false;
}
},
AuthorizationCodeReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
AuthenticationFailed = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenValidated = async n =>
{
// doesn't go through here
Debug.WriteLine($"{n.Response.Body}");
},
MessageReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
}
};
app.UseCookieAuthentication(new CookieAuthenticationOptions()
);
app.UseOpenIdConnectAuthentication(authenticationOptions);
Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger.LogLevel = EventLevel.Verbose;
Microsoft.IdentityModel.Logging.IdentityModelEventSource.ShowPII = true;
var listener = new EventListener();
listener.EnableEvents(Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger, EventLevel.LogAlways);
listener.EventWritten += Listener_EventWritten; // Only thing this ever logs is "generating nonce"
}
[Edit]
I found out that in an ASP.NET Core project with GetClaimsFromUserInfoEndpoint = true
it works perfectly. But that property is sadly missing from the older Microsoft.Owin.Security.OpenIdConnect
implementation...
asp.net-mvc owin openid openid-connect katana
|
show 10 more comments
up vote
2
down vote
favorite
I set up a very simple ASP.NET MVC 5 application that tries to authenticate a user through an OpenID provider in Authorization Code mode.
I'm able to log in and the server returns a code in the redirect URL querystring and a nonce cookie. However, back on the client application the user is not authenticated (User.Identity.IsAuthenticated
false), has no claims and called controller Action that has an Authorize
attribute is never carried out. Browser stays on the redirect URL page which is the home page.
I think something happens during the execution of the OpenID Connect middleware which makes it stop halfway through, but can't quite figure out how to debug it.
No exceptions are thrown even in "break on all CLR exceptions" mode.
When connecting an EventListener to
IdentityModelEventSource.Logger
at Verbose level I only get one logged event that says "Generating nonce for openIdConnect message", once per authentication attempt.No
Notification
hooks are reached exceptRedirectToIdentityProvider
, so it looks like no authorization code or security token is received, but the authentication doesn't fail either.
How can I get more info on what happens so that I can debug my problem?
Here's the code:
public void Configuration(IAppBuilder app)
{
var clientSecret = "secret";
var authenticationOptions = new OpenIdConnectAuthenticationOptions
{
ClientId = "id",
ClientSecret = clientSecret,
Authority = "https://theauthority",
RedirectUri = "https://localhost/MyApp/",
};
authenticationOptions.ResponseType = OpenIdConnectResponseType.Code; // Authorization code
authenticationOptions.TokenValidationParameters.IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(clientSecret));
authenticationOptions.TokenValidationParameters.RequireSignedTokens = true;
authenticationOptions.TokenValidationParameters.ValidAudience = "katanaclient";
authenticationOptions.SignInAsAuthenticationType = "Cookies";
authenticationOptions.Configuration = new OpenIdConnectConfiguration
{
Issuer = "https://theissuer",
AuthorizationEndpoint = "https://theendpoint",
TokenEndpoint = "https://theendpoint/api/v1/token",
UserInfoEndpoint = "https://theendpoint/api/v1/userinfo",
EndSessionEndpoint = "https://theendpoint/api/v1/logout",
ScopesSupported = { "openid", "profile"},
};
authenticationOptions.Notifications = new OpenIdConnectAuthenticationNotifications
{
RedirectToIdentityProvider = async n =>
{
// here it goes
if (n.ProtocolMessage.RequestType == OpenIdConnectRequestType.Authentication)
{
n.ProtocolMessage.EnableTelemetryParameters = false;
}
},
AuthorizationCodeReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
AuthenticationFailed = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenValidated = async n =>
{
// doesn't go through here
Debug.WriteLine($"{n.Response.Body}");
},
MessageReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
}
};
app.UseCookieAuthentication(new CookieAuthenticationOptions()
);
app.UseOpenIdConnectAuthentication(authenticationOptions);
Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger.LogLevel = EventLevel.Verbose;
Microsoft.IdentityModel.Logging.IdentityModelEventSource.ShowPII = true;
var listener = new EventListener();
listener.EnableEvents(Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger, EventLevel.LogAlways);
listener.EventWritten += Listener_EventWritten; // Only thing this ever logs is "generating nonce"
}
[Edit]
I found out that in an ASP.NET Core project with GetClaimsFromUserInfoEndpoint = true
it works perfectly. But that property is sadly missing from the older Microsoft.Owin.Security.OpenIdConnect
implementation...
asp.net-mvc owin openid openid-connect katana
Which OpenID provider are you using?
– Jeshwel
Nov 28 at 14:42
You should use thecode
to get theaccess token
from the token endpoint. It will contain the claims you are looking for.
– RakihthaRR
Dec 3 at 6:12
You can also use OpenIdConnectResponseType.CodeIdToken to cut out the middle man and get it all back in one go. Assuming your OpenId Connect provider allows that response type combination at least.
– Matthew Brubaker
Dec 3 at 20:04
@RakihthaRR Why would the middleware's Configuration expose aTokenEndpoint
setting if you have to manually send a request to the endpoint yourself? What does it use it for?
– guillaume31
Dec 4 at 8:27
@MatthewBrubaker my provider doesn't seem to support Code ID Token:unsupported_response_type
in the redirect URI querystring
– guillaume31
Dec 4 at 8:30
|
show 10 more comments
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I set up a very simple ASP.NET MVC 5 application that tries to authenticate a user through an OpenID provider in Authorization Code mode.
I'm able to log in and the server returns a code in the redirect URL querystring and a nonce cookie. However, back on the client application the user is not authenticated (User.Identity.IsAuthenticated
false), has no claims and called controller Action that has an Authorize
attribute is never carried out. Browser stays on the redirect URL page which is the home page.
I think something happens during the execution of the OpenID Connect middleware which makes it stop halfway through, but can't quite figure out how to debug it.
No exceptions are thrown even in "break on all CLR exceptions" mode.
When connecting an EventListener to
IdentityModelEventSource.Logger
at Verbose level I only get one logged event that says "Generating nonce for openIdConnect message", once per authentication attempt.No
Notification
hooks are reached exceptRedirectToIdentityProvider
, so it looks like no authorization code or security token is received, but the authentication doesn't fail either.
How can I get more info on what happens so that I can debug my problem?
Here's the code:
public void Configuration(IAppBuilder app)
{
var clientSecret = "secret";
var authenticationOptions = new OpenIdConnectAuthenticationOptions
{
ClientId = "id",
ClientSecret = clientSecret,
Authority = "https://theauthority",
RedirectUri = "https://localhost/MyApp/",
};
authenticationOptions.ResponseType = OpenIdConnectResponseType.Code; // Authorization code
authenticationOptions.TokenValidationParameters.IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(clientSecret));
authenticationOptions.TokenValidationParameters.RequireSignedTokens = true;
authenticationOptions.TokenValidationParameters.ValidAudience = "katanaclient";
authenticationOptions.SignInAsAuthenticationType = "Cookies";
authenticationOptions.Configuration = new OpenIdConnectConfiguration
{
Issuer = "https://theissuer",
AuthorizationEndpoint = "https://theendpoint",
TokenEndpoint = "https://theendpoint/api/v1/token",
UserInfoEndpoint = "https://theendpoint/api/v1/userinfo",
EndSessionEndpoint = "https://theendpoint/api/v1/logout",
ScopesSupported = { "openid", "profile"},
};
authenticationOptions.Notifications = new OpenIdConnectAuthenticationNotifications
{
RedirectToIdentityProvider = async n =>
{
// here it goes
if (n.ProtocolMessage.RequestType == OpenIdConnectRequestType.Authentication)
{
n.ProtocolMessage.EnableTelemetryParameters = false;
}
},
AuthorizationCodeReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
AuthenticationFailed = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenValidated = async n =>
{
// doesn't go through here
Debug.WriteLine($"{n.Response.Body}");
},
MessageReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
}
};
app.UseCookieAuthentication(new CookieAuthenticationOptions()
);
app.UseOpenIdConnectAuthentication(authenticationOptions);
Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger.LogLevel = EventLevel.Verbose;
Microsoft.IdentityModel.Logging.IdentityModelEventSource.ShowPII = true;
var listener = new EventListener();
listener.EnableEvents(Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger, EventLevel.LogAlways);
listener.EventWritten += Listener_EventWritten; // Only thing this ever logs is "generating nonce"
}
[Edit]
I found out that in an ASP.NET Core project with GetClaimsFromUserInfoEndpoint = true
it works perfectly. But that property is sadly missing from the older Microsoft.Owin.Security.OpenIdConnect
implementation...
asp.net-mvc owin openid openid-connect katana
I set up a very simple ASP.NET MVC 5 application that tries to authenticate a user through an OpenID provider in Authorization Code mode.
I'm able to log in and the server returns a code in the redirect URL querystring and a nonce cookie. However, back on the client application the user is not authenticated (User.Identity.IsAuthenticated
false), has no claims and called controller Action that has an Authorize
attribute is never carried out. Browser stays on the redirect URL page which is the home page.
I think something happens during the execution of the OpenID Connect middleware which makes it stop halfway through, but can't quite figure out how to debug it.
No exceptions are thrown even in "break on all CLR exceptions" mode.
When connecting an EventListener to
IdentityModelEventSource.Logger
at Verbose level I only get one logged event that says "Generating nonce for openIdConnect message", once per authentication attempt.No
Notification
hooks are reached exceptRedirectToIdentityProvider
, so it looks like no authorization code or security token is received, but the authentication doesn't fail either.
How can I get more info on what happens so that I can debug my problem?
Here's the code:
public void Configuration(IAppBuilder app)
{
var clientSecret = "secret";
var authenticationOptions = new OpenIdConnectAuthenticationOptions
{
ClientId = "id",
ClientSecret = clientSecret,
Authority = "https://theauthority",
RedirectUri = "https://localhost/MyApp/",
};
authenticationOptions.ResponseType = OpenIdConnectResponseType.Code; // Authorization code
authenticationOptions.TokenValidationParameters.IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(clientSecret));
authenticationOptions.TokenValidationParameters.RequireSignedTokens = true;
authenticationOptions.TokenValidationParameters.ValidAudience = "katanaclient";
authenticationOptions.SignInAsAuthenticationType = "Cookies";
authenticationOptions.Configuration = new OpenIdConnectConfiguration
{
Issuer = "https://theissuer",
AuthorizationEndpoint = "https://theendpoint",
TokenEndpoint = "https://theendpoint/api/v1/token",
UserInfoEndpoint = "https://theendpoint/api/v1/userinfo",
EndSessionEndpoint = "https://theendpoint/api/v1/logout",
ScopesSupported = { "openid", "profile"},
};
authenticationOptions.Notifications = new OpenIdConnectAuthenticationNotifications
{
RedirectToIdentityProvider = async n =>
{
// here it goes
if (n.ProtocolMessage.RequestType == OpenIdConnectRequestType.Authentication)
{
n.ProtocolMessage.EnableTelemetryParameters = false;
}
},
AuthorizationCodeReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
AuthenticationFailed = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
},
SecurityTokenValidated = async n =>
{
// doesn't go through here
Debug.WriteLine($"{n.Response.Body}");
},
MessageReceived = async notification =>
{
// doesn't go through here
Debug.WriteLine($"{notification.Response.Body}");
}
};
app.UseCookieAuthentication(new CookieAuthenticationOptions()
);
app.UseOpenIdConnectAuthentication(authenticationOptions);
Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger.LogLevel = EventLevel.Verbose;
Microsoft.IdentityModel.Logging.IdentityModelEventSource.ShowPII = true;
var listener = new EventListener();
listener.EnableEvents(Microsoft.IdentityModel.Logging.IdentityModelEventSource.Logger, EventLevel.LogAlways);
listener.EventWritten += Listener_EventWritten; // Only thing this ever logs is "generating nonce"
}
[Edit]
I found out that in an ASP.NET Core project with GetClaimsFromUserInfoEndpoint = true
it works perfectly. But that property is sadly missing from the older Microsoft.Owin.Security.OpenIdConnect
implementation...
asp.net-mvc owin openid openid-connect katana
asp.net-mvc owin openid openid-connect katana
edited Dec 7 at 14:09
asked Nov 22 at 8:33
guillaume31
11.2k2339
11.2k2339
Which OpenID provider are you using?
– Jeshwel
Nov 28 at 14:42
You should use thecode
to get theaccess token
from the token endpoint. It will contain the claims you are looking for.
– RakihthaRR
Dec 3 at 6:12
You can also use OpenIdConnectResponseType.CodeIdToken to cut out the middle man and get it all back in one go. Assuming your OpenId Connect provider allows that response type combination at least.
– Matthew Brubaker
Dec 3 at 20:04
@RakihthaRR Why would the middleware's Configuration expose aTokenEndpoint
setting if you have to manually send a request to the endpoint yourself? What does it use it for?
– guillaume31
Dec 4 at 8:27
@MatthewBrubaker my provider doesn't seem to support Code ID Token:unsupported_response_type
in the redirect URI querystring
– guillaume31
Dec 4 at 8:30
|
show 10 more comments
Which OpenID provider are you using?
– Jeshwel
Nov 28 at 14:42
You should use thecode
to get theaccess token
from the token endpoint. It will contain the claims you are looking for.
– RakihthaRR
Dec 3 at 6:12
You can also use OpenIdConnectResponseType.CodeIdToken to cut out the middle man and get it all back in one go. Assuming your OpenId Connect provider allows that response type combination at least.
– Matthew Brubaker
Dec 3 at 20:04
@RakihthaRR Why would the middleware's Configuration expose aTokenEndpoint
setting if you have to manually send a request to the endpoint yourself? What does it use it for?
– guillaume31
Dec 4 at 8:27
@MatthewBrubaker my provider doesn't seem to support Code ID Token:unsupported_response_type
in the redirect URI querystring
– guillaume31
Dec 4 at 8:30
Which OpenID provider are you using?
– Jeshwel
Nov 28 at 14:42
Which OpenID provider are you using?
– Jeshwel
Nov 28 at 14:42
You should use the
code
to get the access token
from the token endpoint. It will contain the claims you are looking for.– RakihthaRR
Dec 3 at 6:12
You should use the
code
to get the access token
from the token endpoint. It will contain the claims you are looking for.– RakihthaRR
Dec 3 at 6:12
You can also use OpenIdConnectResponseType.CodeIdToken to cut out the middle man and get it all back in one go. Assuming your OpenId Connect provider allows that response type combination at least.
– Matthew Brubaker
Dec 3 at 20:04
You can also use OpenIdConnectResponseType.CodeIdToken to cut out the middle man and get it all back in one go. Assuming your OpenId Connect provider allows that response type combination at least.
– Matthew Brubaker
Dec 3 at 20:04
@RakihthaRR Why would the middleware's Configuration expose a
TokenEndpoint
setting if you have to manually send a request to the endpoint yourself? What does it use it for?– guillaume31
Dec 4 at 8:27
@RakihthaRR Why would the middleware's Configuration expose a
TokenEndpoint
setting if you have to manually send a request to the endpoint yourself? What does it use it for?– guillaume31
Dec 4 at 8:27
@MatthewBrubaker my provider doesn't seem to support Code ID Token:
unsupported_response_type
in the redirect URI querystring– guillaume31
Dec 4 at 8:30
@MatthewBrubaker my provider doesn't seem to support Code ID Token:
unsupported_response_type
in the redirect URI querystring– guillaume31
Dec 4 at 8:30
|
show 10 more comments
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426753%2ffailing-openid-connect-middleware-how-to-debug%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426753%2ffailing-openid-connect-middleware-how-to-debug%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vynLulB0E
Which OpenID provider are you using?
– Jeshwel
Nov 28 at 14:42
You should use the
code
to get theaccess token
from the token endpoint. It will contain the claims you are looking for.– RakihthaRR
Dec 3 at 6:12
You can also use OpenIdConnectResponseType.CodeIdToken to cut out the middle man and get it all back in one go. Assuming your OpenId Connect provider allows that response type combination at least.
– Matthew Brubaker
Dec 3 at 20:04
@RakihthaRR Why would the middleware's Configuration expose a
TokenEndpoint
setting if you have to manually send a request to the endpoint yourself? What does it use it for?– guillaume31
Dec 4 at 8:27
@MatthewBrubaker my provider doesn't seem to support Code ID Token:
unsupported_response_type
in the redirect URI querystring– guillaume31
Dec 4 at 8:30